一、介绍:
??模型类被创建在"应用目录/models.py" 文件中。模型类必须继承自Model类,位于包dango.db.models 中。接下来首先以"影片-人物"管理为例进行演示。 1定义在models.py文件中定义模型类。
from django.db import models
# Create your models here.
#影片信息模型类
class FilmInfo(models.Model):
"""fimInfo model"""
fid = models.AutoField(primary_key=True, verbose_name='影片编号')
fname = models.CharField(max_length=20, verbose_name="影片名称")
playcount = models.IntegerField(default=0, verbose_name="播放量")
commentcount = models.IntegerField(default=8, verbose_name="评论量")
pub_date = models.DateField(null=True, verbose_name="发布日期")
is_delete = models.BooleanField(default=False,verbose_name="逻辑删除")
class Meta:
db_table = "filminfo" #指明数据库表名
verbose_name ="影片信息" #在admin站点中显示的名称
#优化模型类输出
def __str__(self):
return self.fname
class PeopleInfo(models.Model):
GENDER_CHOICES=(
(0,'男'),
(1,'女')
)
uid=models.AutoField(primary_key=True,verbose_name='编号')
uname=models.CharField(max_length=10,verbose_name='编号')
gender=models.SmallIntegerField(choices=GENDER_CHOICES,default=0,verbose_name='性别')
desc =models.CharField(max_length=2000,null=True,verbose_name='简介')
# 外键约束,人物属于那部影片
film=models.ForeignKey(FilmInfo,on_delete=models.CASCADE,verbose_name='影片')
is_delete = models.BooleanField(default=False,verbose_name='逻辑删除')
class Meta:
db_table='t_peopleinfo' #指名数据库表名
verbose_name='人物信息' #在admin 站点中显示的名称
#优化模型类输出
def __str__(self):
return self.uname
下面结果会报错,
#创建牵引文件连接数据库,Mysql ,下面连接数据库会报错。
(py3_001) boot@boot-virtual-machine:~/Desktop/djprojects/film_manager$ python manage.py makemigrations film
Traceback (most recent call last):
File "/home/boot/Desktop/djprojects/film_manager/manage.py", line 22, in <module>
main()
...........................................................
File "/home/boot/.virtualenvs/py3_001/lib/python3.9/site-packages/pymysql/_auth.py", line 265, in caching_sha2_password_auth
data = sha2_rsa_encrypt(conn.password, conn.salt, conn.server_public_key)
File "/home/boot/.virtualenvs/py3_001/lib/python3.9/site-packages/pymysql/_auth.py", line 143, in sha2_rsa_encrypt
raise RuntimeError(
RuntimeError: 'cryptography' package is required for sha256_password or caching_sha2_password auth methods
??由上面的错误提示可知,连接数据库时候,cryptography 包 是required 。 解决办法,需要安装 :pip install cryptography 。即可成功解决.结果如下图所示:
(py3_001) boot@boot-virtual-machine:~/Desktop/djprojects/film_manager$ pip install cryptography
Collecting cryptography
Downloading cryptography-35.0.0-cp36-abi3-manylinux_2_24_x86_64.whl (3.5 MB)
|████████████████████████████████| 3.5 MB 1.4 MB/s
Collecting cffi>=1.12
Downloading cffi-1.15.0-cp39-cp39-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (444 kB)
|████████████████████████████████| 444 kB 190 kB/s
Collecting pycparser
Downloading pycparser-2.21-py2.py3-none-any.whl (118 kB)
|████████████████████████████████| 118 kB 611 kB/s
Installing collected packages: pycparser, cffi, cryptography
Successfully installed cffi-1.15.0 cryptography-35.0.0 pycparser-2.21
(py3_001) boot@boot-virtual-machine:~/Desktop/djprojects/film_manager$ python manage.py makemigrations film
Migrations for 'film':
film/migrations/0001_initial.py
- Create model FilmInfo
- Create model PeopleInfo
(py3_001) boot@boot-virtual-machine:~/Desktop/djprojects/film_manager$
二、根据迁移文件生成映射书库据表。
??所以可以看到我们创建的两个模型类已经运行成功。分别是:Create model FilmInfo ,Create model PeopleInfo 。接下来我们去生成映射书库据表。
(py3_001) boot@boot-virtual-machine:~/Desktop/djprojects/film_manager$ python manage.py migrate #生成映射书库据表。
Operations to perform:
Apply all migrations: admin, auth, contenttypes, film, sessions
Running migrations:
Applying contenttypes.0001_initial... OK
Applying auth.0001_initial... OK
Applying admin.0001_initial... OK
Applying admin.0002_logentry_remove_auto_add... OK
Applying admin.0003_logentry_add_action_flag_choices... OK
Applying contenttypes.0002_remove_content_type_name... OK
Applying auth.0002_alter_permission_name_max_length... OK
Applying auth.0003_alter_user_email_max_length... OK
Applying auth.0004_alter_user_username_opts... OK
Applying auth.0005_alter_user_last_login_null... OK
Applying auth.0006_require_contenttypes_0002... OK
Applying auth.0007_alter_validators_add_error_messages... OK
Applying auth.0008_alter_user_username_max_length... OK
Applying auth.0009_alter_user_last_name_max_length... OK
Applying auth.0010_alter_group_name_max_length... OK
Applying auth.0011_update_proxy_permissions... OK
Applying auth.0012_alter_user_first_name_max_length... OK
Applying film.0001_initial... OK
Applying sessions.0001_initial... OK
(py3_001) boot@boot-virtual-machine:~/Desktop/djprojects/film_manager$
三、查看数据库是否根据牵引文件的需求生成数据库,因此返回终端去连接filmdatabase 数据库。
boot@boot-virtual-machine:~$ mysql -h 192.168.86.128 -u luyu -p #以 luyu 的身份进入数据库
Enter password:
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 25
Server version: 8.0.27-0ubuntu0.21.10.1 (Ubuntu)
Copyright (c) 2000, 2021, Oracle and/or its affiliates.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql> use filmdatabase; #开始使用我们在pycharm 创建的数据库模型类
Reading table information for completion of table and column names
You can turn off this feature to get a quicker startup with -A
Database changed
mysql> show tables; #显示filmdatabase工程下的所有数据库明细
+----------------------------+
| Tables_in_filmdatabase |
+----------------------------+
| auth_group |
| auth_group_permissions |
| auth_permission |
| auth_user |
| auth_user_groups |
| auth_user_user_permissions |
| django_admin_log |
| django_content_type |
| django_migrations |
| django_session |
| t_filminfo |
| t_peopleinfo |
+----------------------------+
12 rows in set (0.00 sec)
mysql> desc t_filminfo; #查看 t_filminfo数据库字段情况
+--------------+-------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+--------------+-------------+------+-----+---------+----------------+
| fid | int | NO | PRI | NULL | auto_increment |
| fname | varchar(20) | NO | | NULL | |
| playcount | int | NO | | NULL | |
| commentcount | int | NO | | NULL | |
| pub_date | date | YES | | NULL | |
| is_delete | tinyint(1) | NO | | NULL | |
+--------------+-------------+------+-----+---------+----------------+
6 rows in set (0.03 sec)
mysql> desc t_peopleinfo; #查看t_peopleinfo数据库字段情况
+-----------+---------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-----------+---------------+------+-----+---------+----------------+
| uid | int | NO | PRI | NULL | auto_increment |
| uname | varchar(10) | NO | | NULL | |
| gender | smallint | NO | | NULL | |
| desc | varchar(2000) | YES | | NULL | |
| is_delete | tinyint(1) | NO | | NULL | |
| film_id | int | NO | MUL | NULL | |
+-----------+---------------+------+-----+---------+----------------+
6 rows in set (0.00 sec)
mysql>
四、最后,了解一些数据库的知识说明。
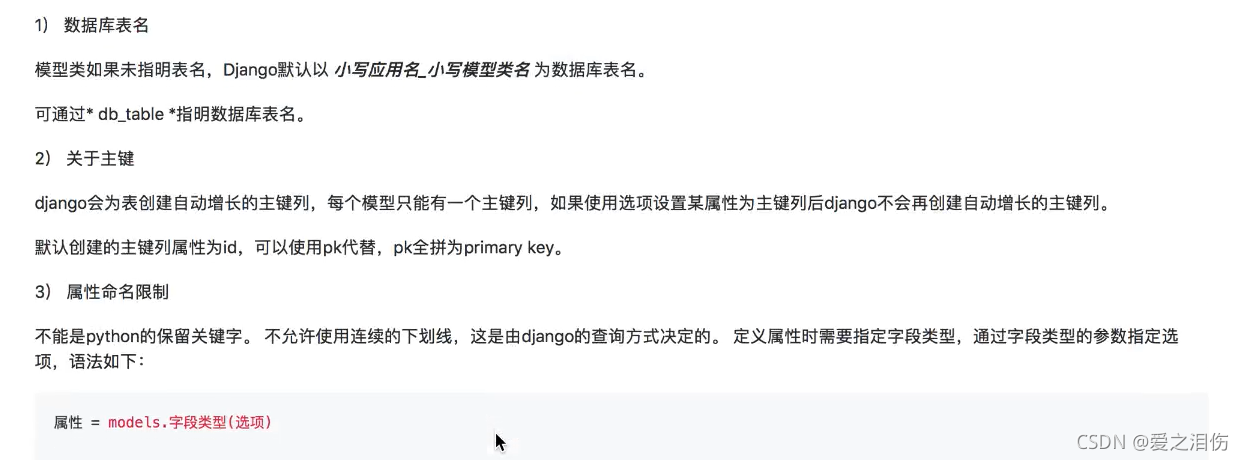 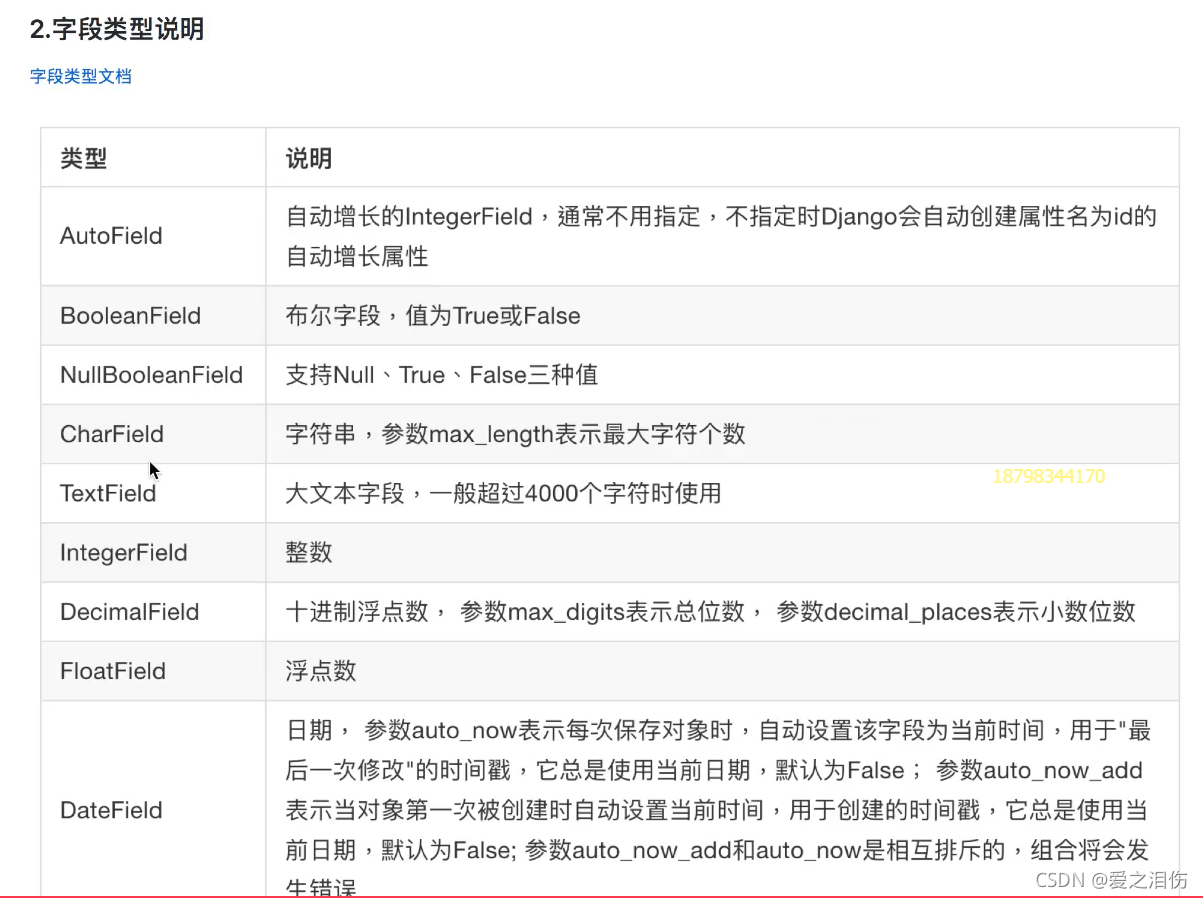 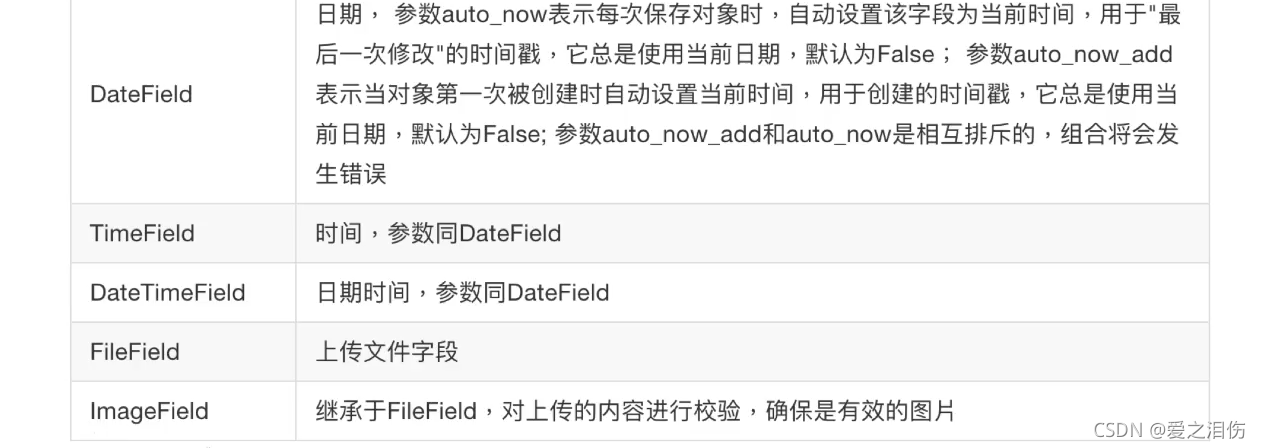 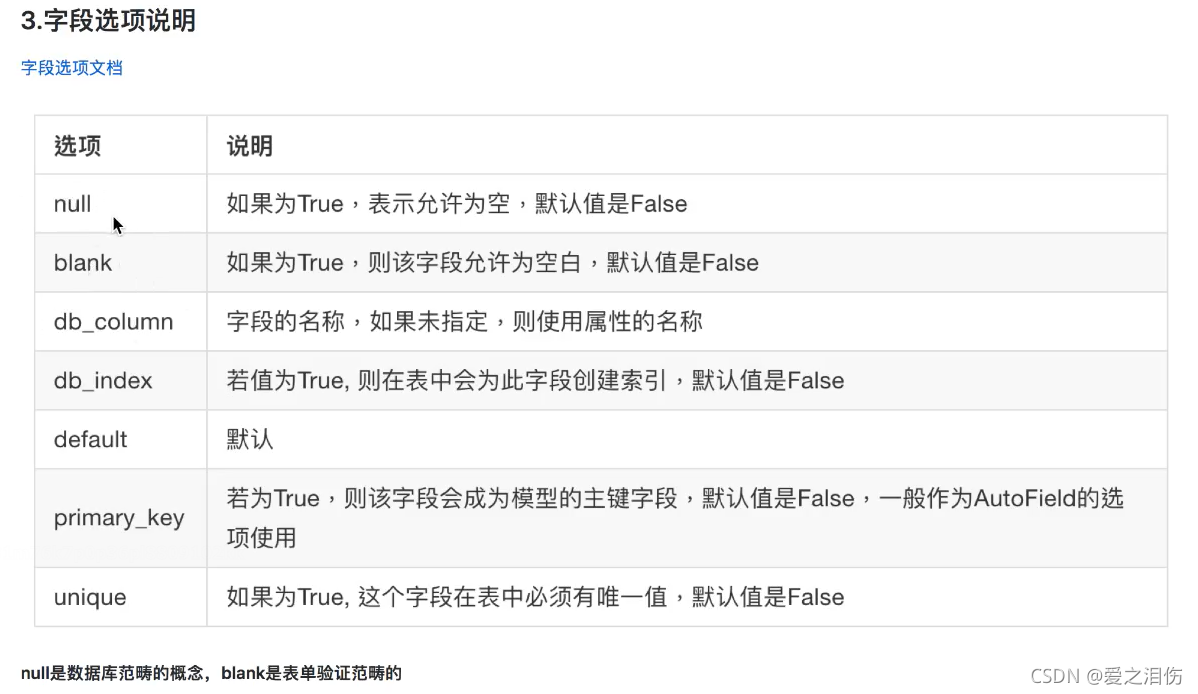 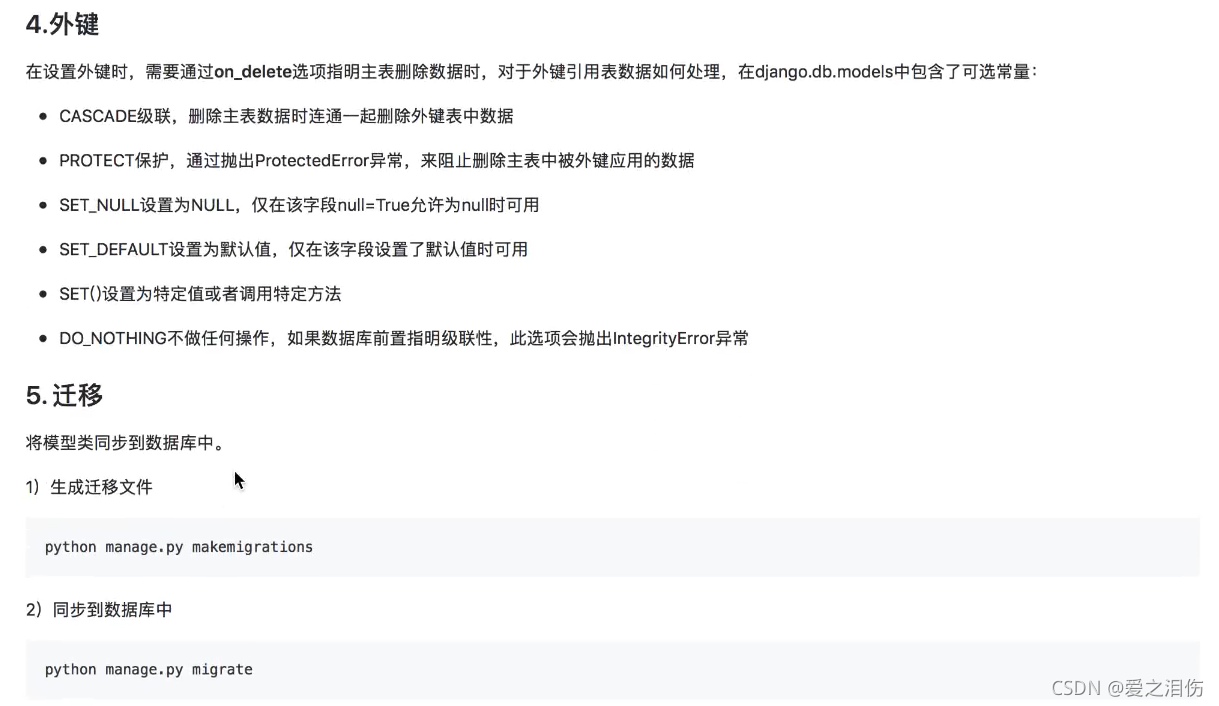 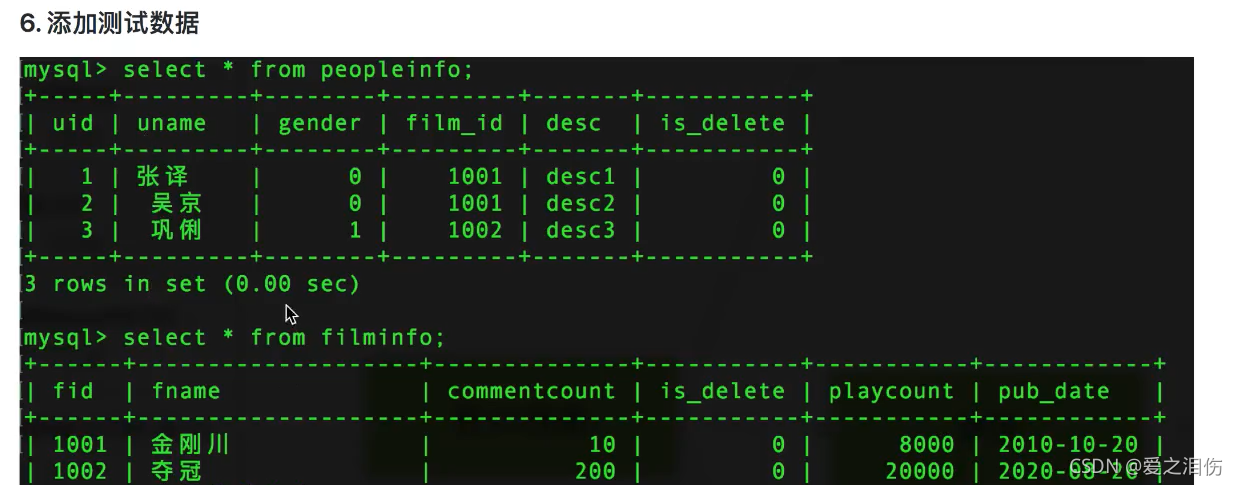
|