外层加引号(repr)/去掉外层引号(eval)
s = 'abdcf'
print eval('['+','.join([repr(i) for i in s])+']')
['a', 'b', 'd', 'c', 'f']
序列与字符串互相转换(join)
join:字符串序列使用指定字符拼接,被拼接的序列必须是字符串
>>> str1='()'
>
>>> str2=['ab','c','d']
>>> str1.join(str2)
'ab()c()d'
>>> str3=('x','y','z')
>>> str1.join(str3)
'x()y()z'
>>> s = {'t1','t2','t3','t4'}
>>> str1.join(s)
't3()t4()t2()t1'
>>> Dict = {'I': 'I', 'Love': 'love', 'You': 'you'}
>>> str1.join(Dict)
'I()Love()You'
>>> str4='mnkj'
>>> str1.join(str4)
'm()n()k()j'
简单条件判断在同一行处理
直接看例子更有说服力:
a = [1,2,3]
b = a if len(a) != 0 else ""
print(b)
c=[]
d = c if len(c) != 0 else "c 是一个空列表"
print(d)
没有switch case
没有自增++、自减–
while 与 else 组合 、for 与 else 组合
x = 3
while (x >0):
print x
while (x > 0):
print x
else :
print x
x = 8
for i in range(3,x):
print i
else:
print i + 1
空语句 pass
pass 本身不做任何事情,只是用于占位,保持结构完整性, 那么问题来了,为啥要占位呢? 可能是某些语句不需要做任何事情,但又必须要有内容,或者某些条件成立不需要做任何事情,但为了阅读上的便利;
class A:
pass
for letter in 'Python':
if letter == 'h':
pass
print '这是 pass 块'
print '当前字母 :', letter
print "Good bye!"
import 和 dir
使用import引入包,就可以使用包中的方法; dir可以查看包中有哪些内容;
>>> import math
>>> dir(math)
['__doc__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos', 'cosh', 'degrees', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'pi', 'pow', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc']
常用数据函数
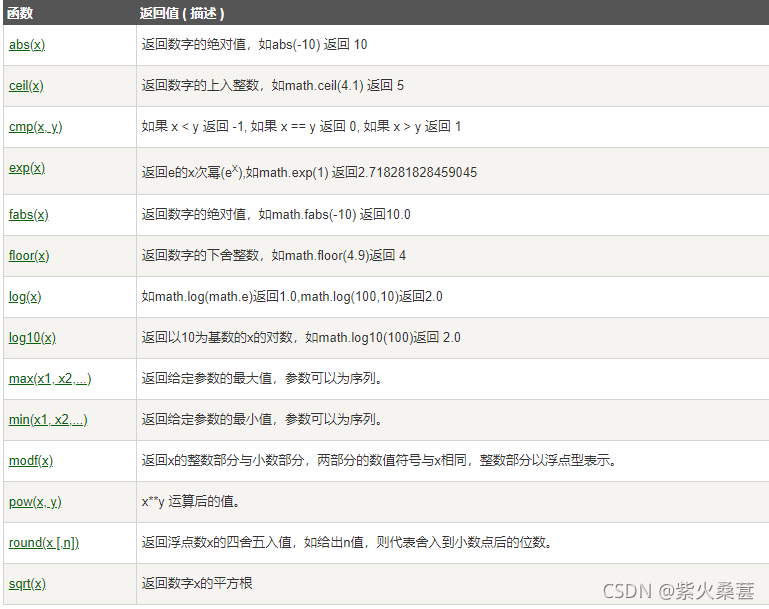
随机函数
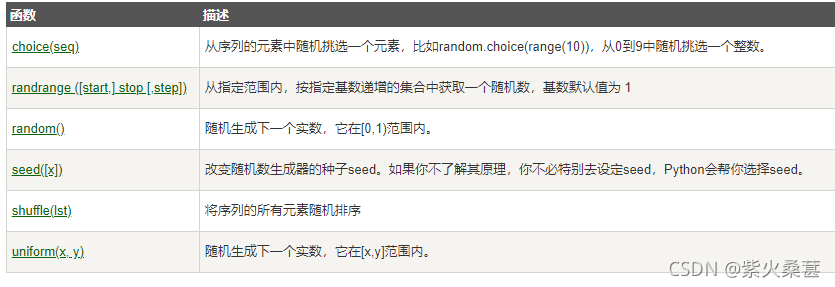
range 函数(左闭右开)
print range(1,5)
日期、时间
import time 模块
>>> import time
>>> time.time()
1637573856.7712119
>>> time.localtime()
time.struct_time(tm_year=2021, tm_mon=11, tm_mday=22, tm_hour=17, tm_min=37, tm_sec=44, tm_wday=0, tm_yday=326, tm_isdst=0)
>>> time.asctime()
'Mon Nov 22 17:39:16 2021'
>>> time.asctime(time.localtime(time.time()))
'Mon Nov 22 17:39:47 2021'
日历
>>> import calendar
>>> dir(calendar)
['Calendar', 'EPOCH', 'FRIDAY', 'February', 'HTMLCalendar', 'IllegalMonthError', 'IllegalWeekdayError', 'January', 'LocaleHTMLCalendar', 'LocaleTextCalendar', 'MONDAY', 'SATURDAY', 'SUNDAY', 'THURSDAY', 'TUESDAY', 'TextCalendar', 'WEDNESDAY', '_EPOCH_ORD', '__all__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', '_colwidth', '_locale', '_localized_day', '_localized_month', '_spacing', 'c', 'calendar', 'datetime', 'day_abbr', 'day_name', 'different_locale', 'error', 'firstweekday', 'format', 'formatstring', 'isleap', 'leapdays', 'main', 'mdays', 'month', 'month_abbr', 'month_name', 'monthcalendar', 'monthlen', 'monthrange', 'nextmonth', 'prcal', 'prevmonth', 'prmonth', 'prweek', 'repeat', 'setfirstweekday', 'sys', 'timegm', 'week', 'weekday', 'weekheader']
>>>
time 模块
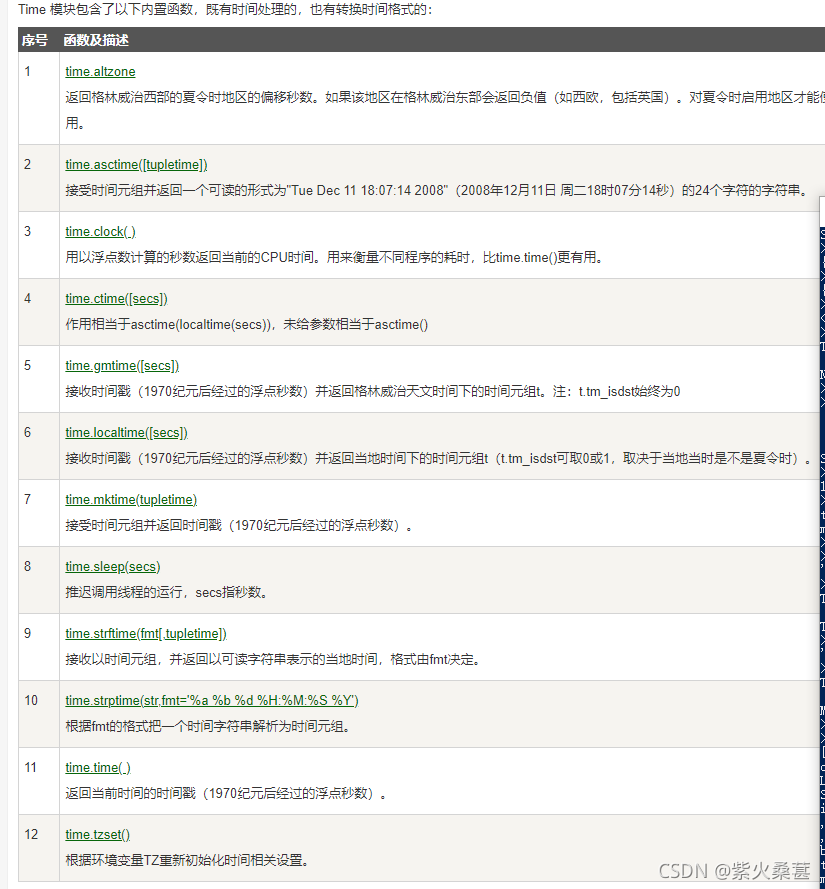
|