面向对象进阶-多继承和定制对象
多重继承
class Father(object):
def work(self):
print('父亲在工作')
class Mather(object):
def work(self):
print('母亲在工作')
class Child(Father, Mather):
def __init__(self, name):
self._name = name
if __name__ == '__main__':
c = Child('小明')
c.work()
print(Child.__mro__)
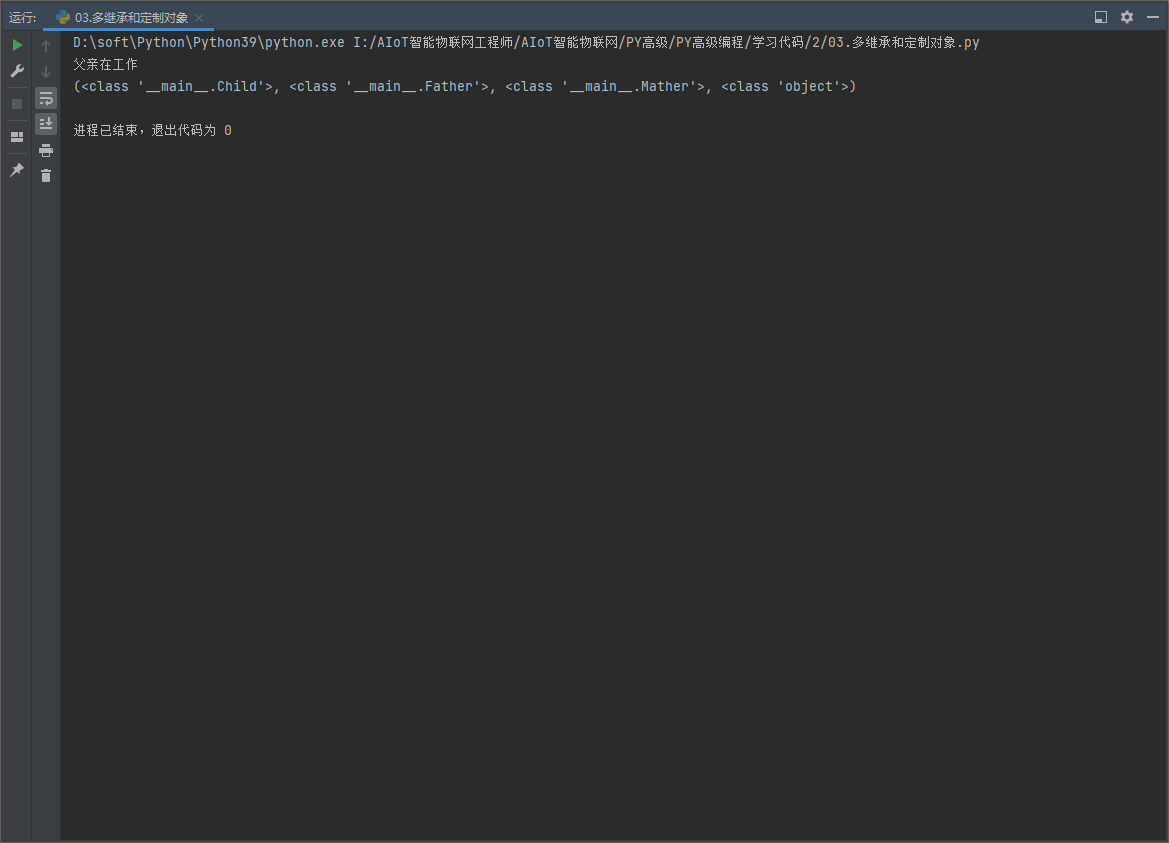
定制类 (魔术方法)
头尾是双下划线的属性或方法, 在Python中是有特殊用途
str 打印这个对象的描述
def __str__(self):
print(super(Person, self).__str__())
print('-' * 80)
return ', '.join([
self.__class__.__module__,
self.__class__.__name__,
self._name,
hex(id(self.__class__)),
hex(id(self))
])
p = Person('张三')
print(p)
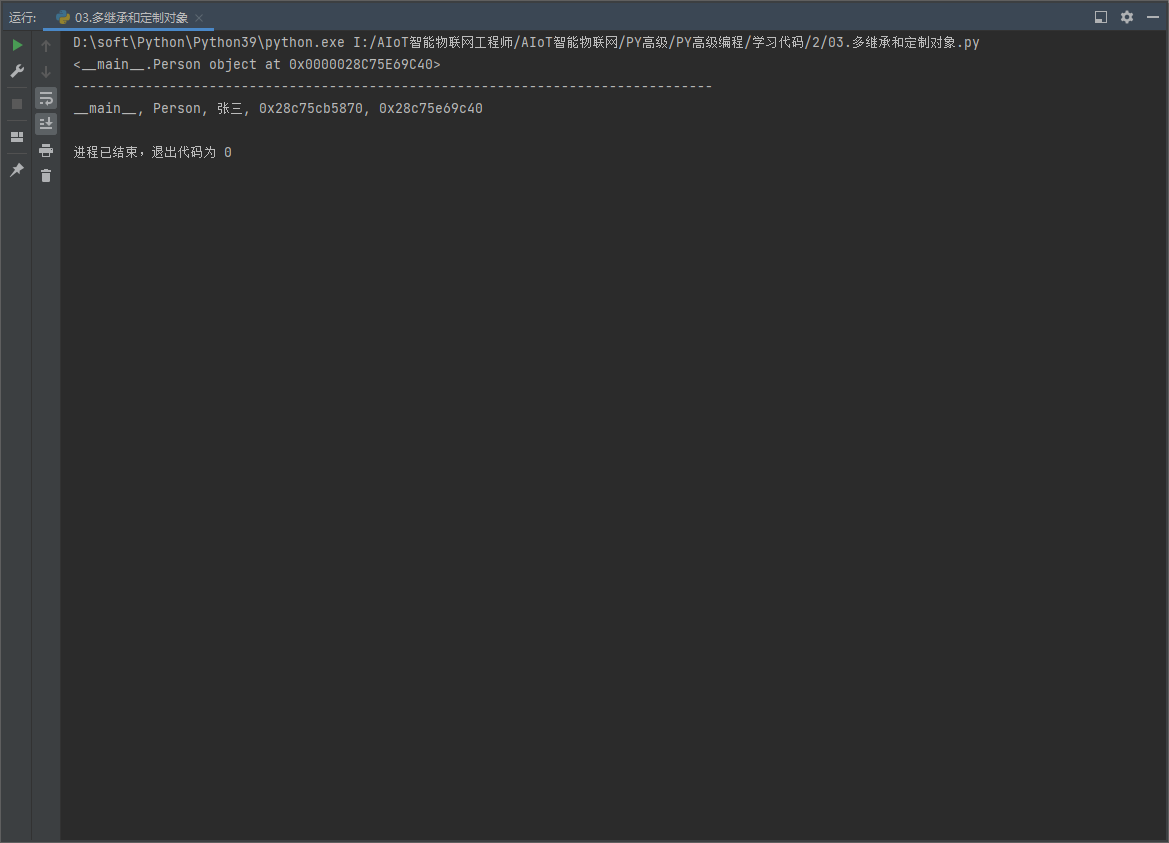
iter 与 next 定义可迭代对象与迭代器对象
from collections.abc import Iterable
from collections.abc import Iterator
class Fib:
a = 0
b = 1
i = 0
maxSize = 20
def __iter__(self):
return self
def __next__(self):
self.i += 1
self.a, self.b = self.b, self.a + self.b
if self.i > self.maxSize:
raise StopIteration
return self.a
f = Fib()
print(isinstance(f, Iterable))
print(isinstance(f, Iterator))
for k, _ in enumerate(f):
print(str(k).zfill(3), _)
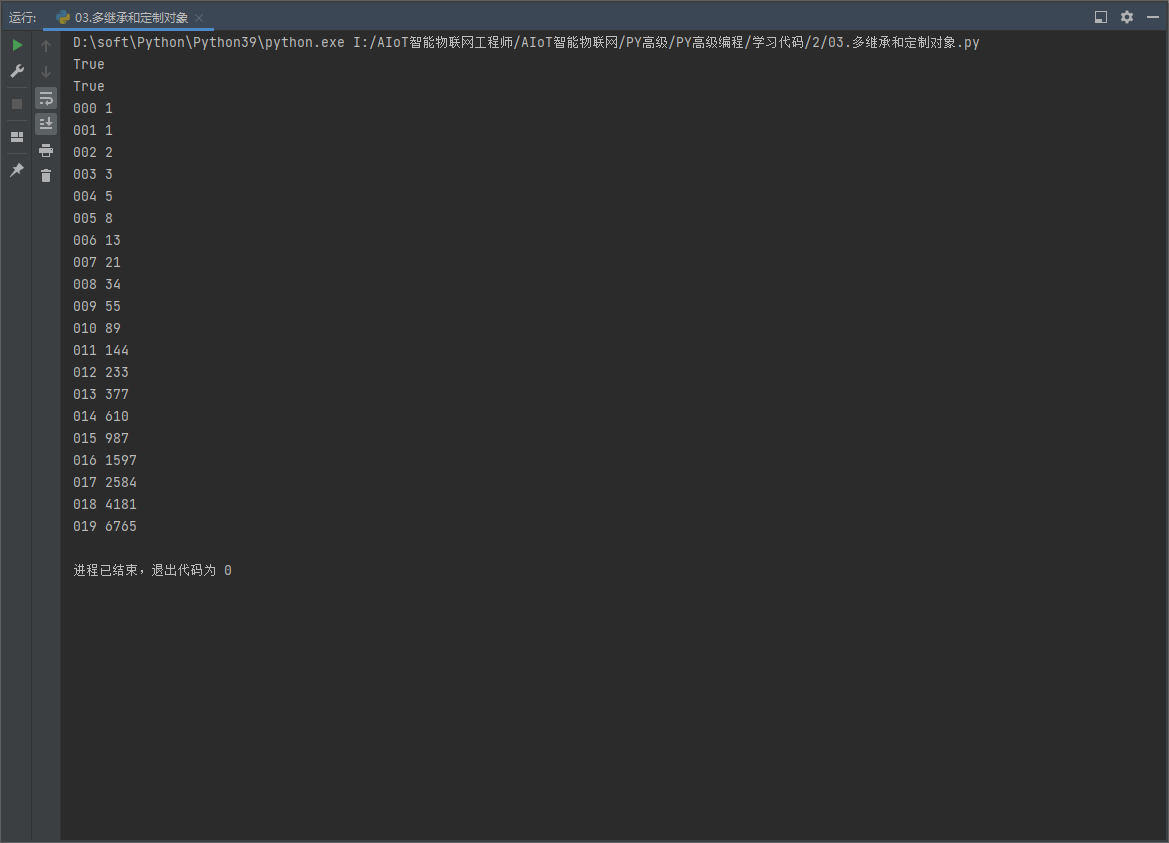
getitem 使迭代对象更像list 可以下标访问、切片
def __getitem__(self, item):
a, b, c, i = 1, 1, [], 0
start, stop, step = 0, self.maxSize, 1
if isinstance(item, int):
start, stop, step = 0, item, 1
elif isinstance(item, slice):
start, stop, step = item.start, item.stop, item.step
if start is None:
start = 0
if stop is None:
start = self.maxSize
if step is None:
step = 1
for _ in range(stop):
if _ >= start:
if i % step == 0:
c.append(a)
i += 1
a, b = b, a + b
if isinstance(item, int):
return a
elif isinstance(item, slice):
return c
print('-' * 80)
print(f[5])
print(f[5:10:2])
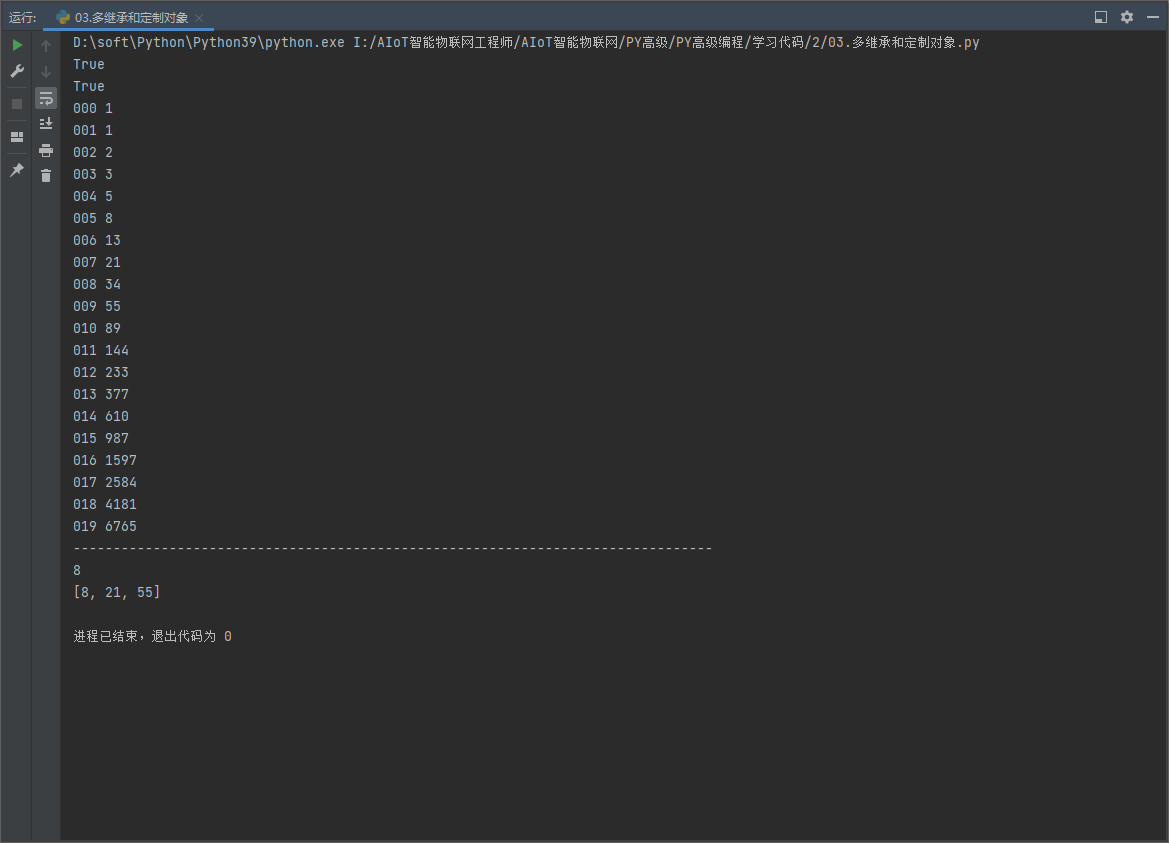
当访问一个不存在的属性或方法 会报错 该如何防止错误?? getattr
def __getattr__(self, item):
prop = '_' + item
if prop in self.__dict__:
return self.__dict__.get(prop)
return lambda: print('%s 方法不存在' % item)
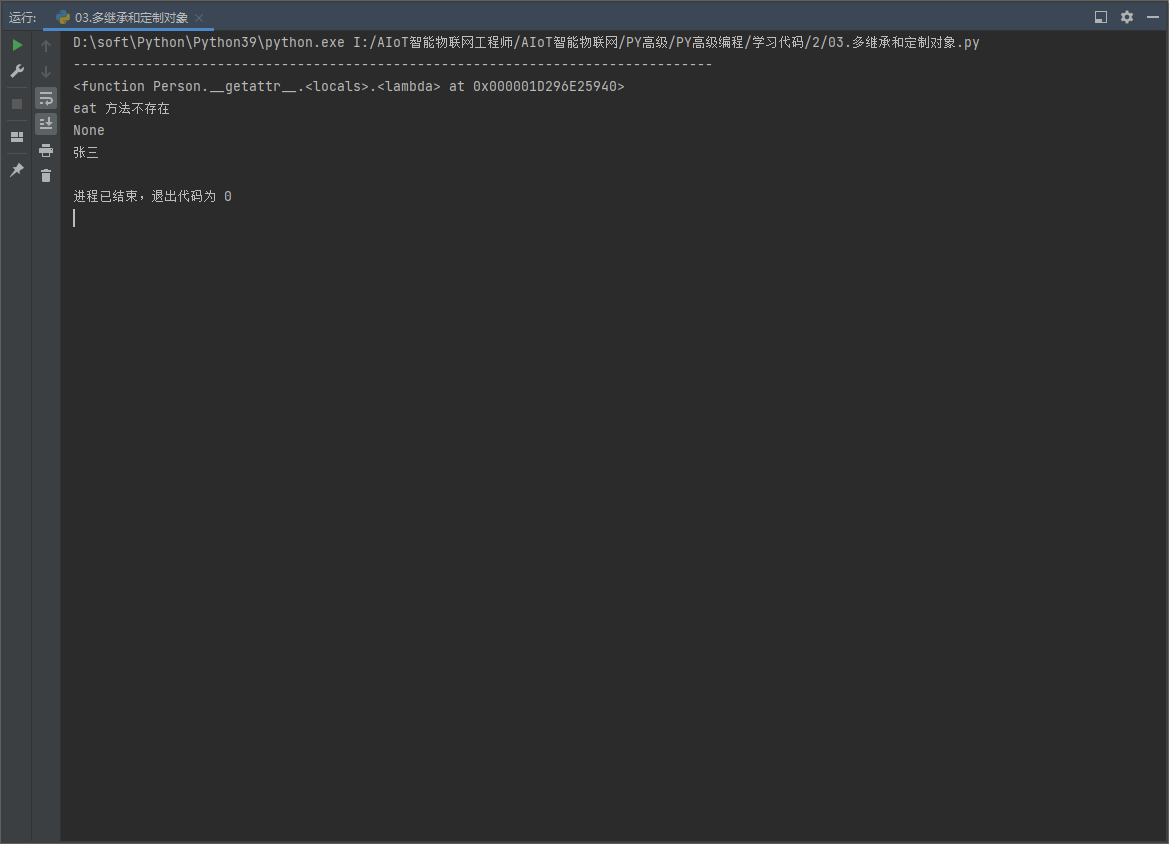
把实例对象当成函数调用 call
def __call__(self, *args, **kwargs):
print('这个实例对象可以当成函数调用')
pass
print(p())
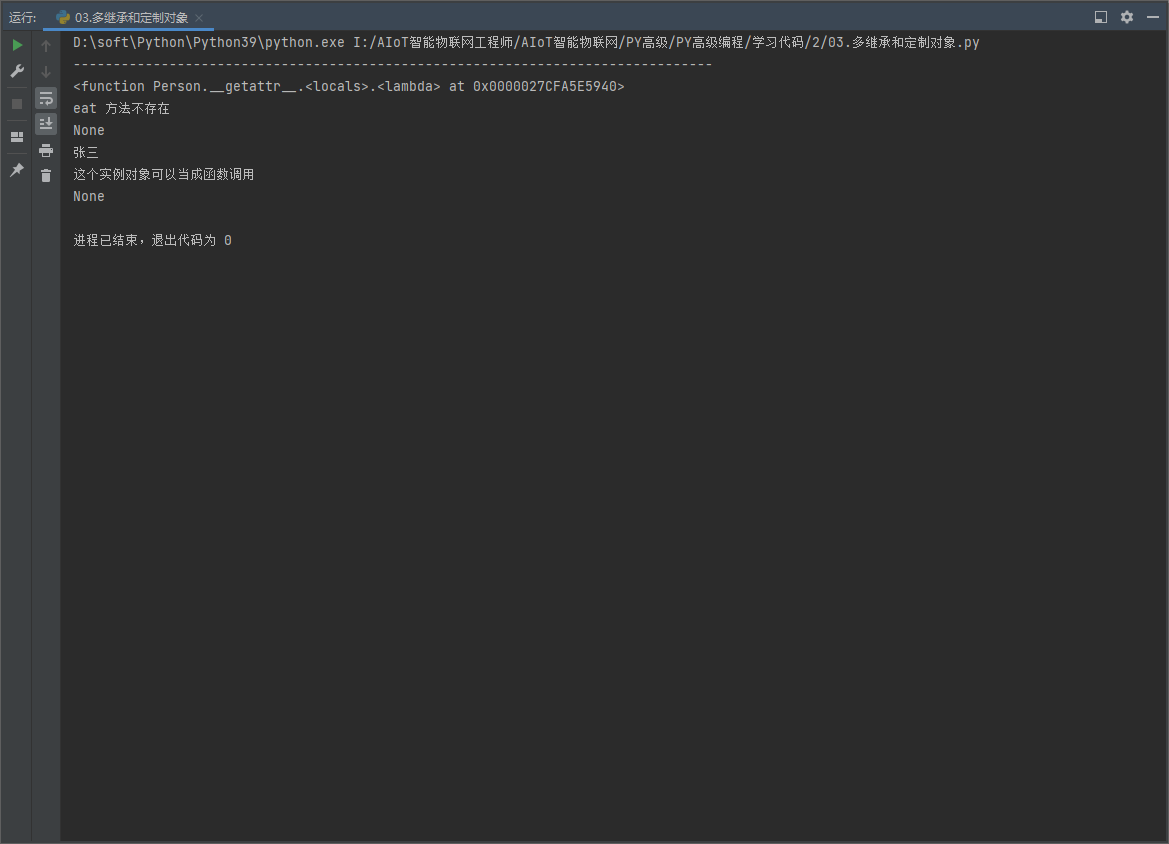
判断一个变量是对象还是函数 | 是否可以调用
print(callable(p))
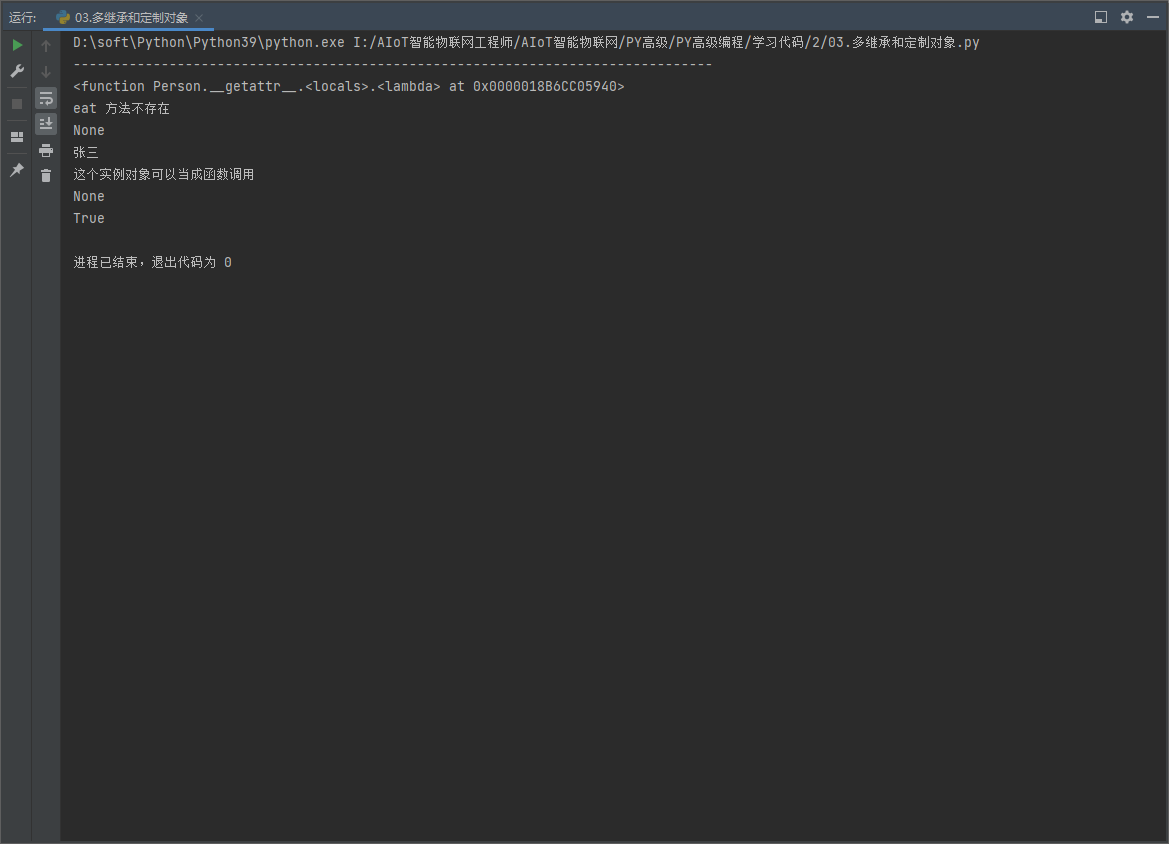
|