1.首先引入Maven依赖 可以登陆Maven网站,查找jython-standalone 的依赖,选中使用较多使用的版本即可,此处以2.7.0为例:
<dependency>
<groupId>org.python</groupId>
<artifactId>jython-standalone</artifactId>
<version>2.7.0</version>
</dependency>
2.编写python函数,此处以pycharm为例,写一个快排,并经过测试争取无误后即可使用
def partition(arr, low, high):
i = (low-1)
pivot = arr[high]
for j in range(low, high):
if arr[j] <= pivot:
i = i + 1
arr[i], arr[j] = arr[j], arr[i]
arr[i+1], arr[high] = arr[high], arr[i+1]
return i + 1
def quick_sort(arr, low, high):
if low < high:
pi = partition(arr, low, high)
quick_sort(arr, low, pi-1)
quick_sort(arr, pi + 1, high)
def do_sort(nums):
arr = nums
n = len(arr)
quick_sort(arr, 0, n-1)
return arr
3.编写Java代码对上述快排进行调用
package com.example.graduation.controller;
import com.example.graduation.bean.data;
import com.example.graduation.service.DataService;
import org.python.core.PyFunction;
import org.python.core.PyList;
import org.python.core.PyObject;
import org.python.util.PythonInterpreter;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
@Controller
public class DataController {
@RequestMapping("/test")
@ResponseBody
public String test(){
PythonInterpreter interpreter = new PythonInterpreter();
Random random = new Random();
List<Integer> list = new ArrayList<>();
for (int i = 0; i < 10; i++) {
list.add(random.nextInt(100));
}
interpreter.execfile("F:\\WK\\Python\\experiment\\graduation\\test.py");
PyFunction function = interpreter.get("do_sort", PyFunction.class);
PyObject obj = function.__call__(new PyList(list));
return obj.toString();
}
}
4.启动springboot项目 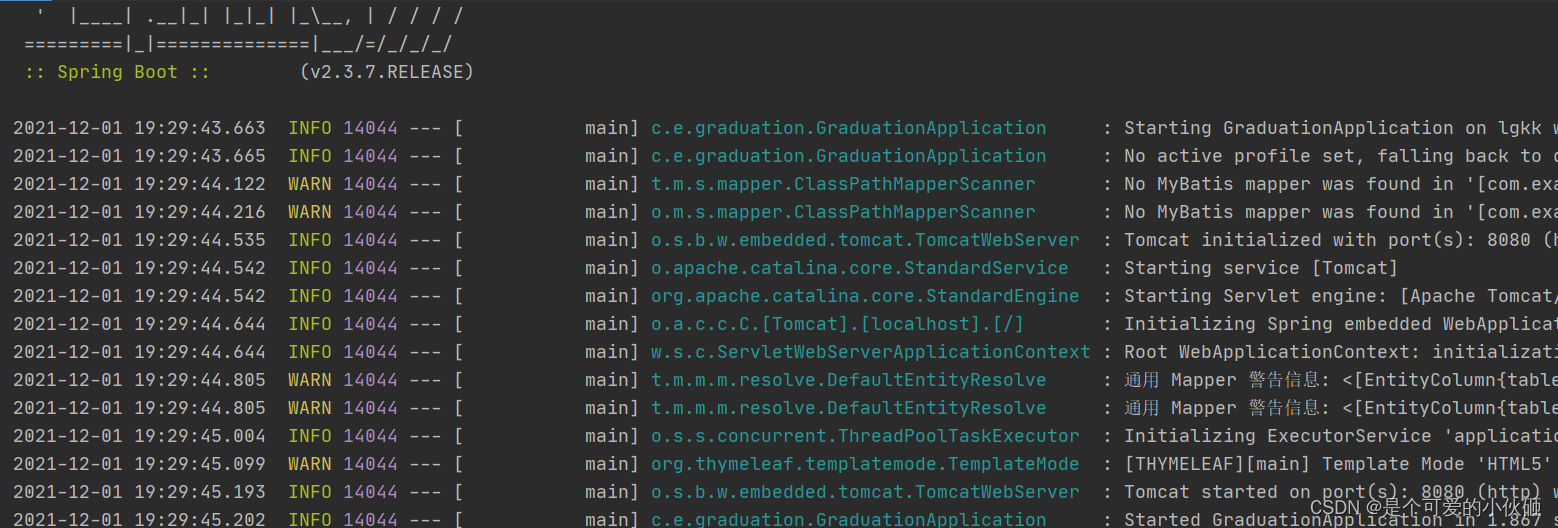 5.浏览器访问http://localhost:8080/test 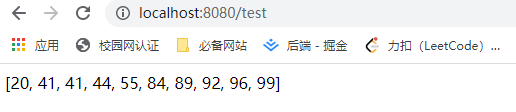
|