场景
- 文件传输大小限制时,需要将文件切小,分别发送,合并还原
- 文件发送限制(比如:破解软件、小电影…)
切分原理图
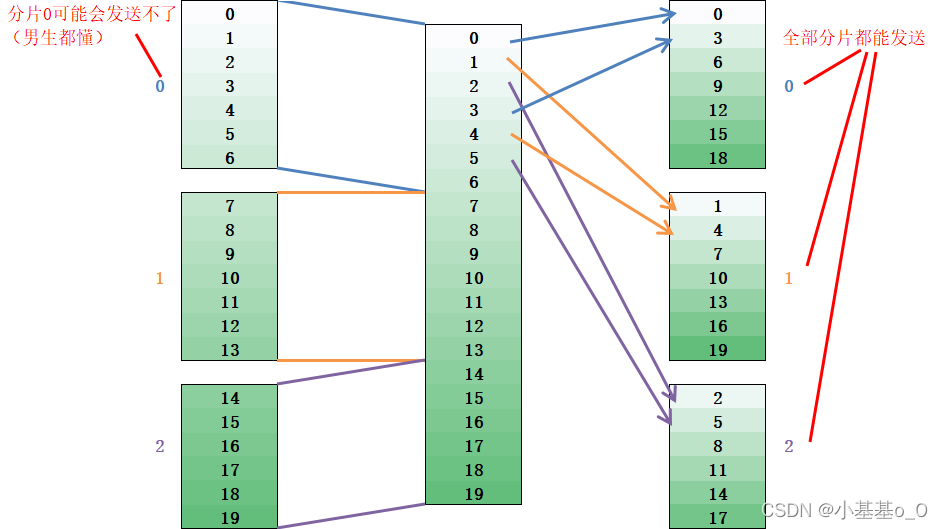
代码实现
切分方式(图左)
SOURCE = 'scala-intellij-bin-2020.3.1.zip'
N = 9
restore = 'restore_' + SOURCE
def rb(abs_path):
"""二进制方式读取文件"""
with open(abs_path, 'rb') as f:
return f.read()
def wb(abs_path, s):
"""二进制方式写文件"""
with open(abs_path, 'wb') as f:
f.write(s)
def split():
"""文件切分"""
frb = rb(SOURCE)
each = int(len(frb) // N) + 1
for i in range(N):
wb(str(i), frb[i * each: (i + 1) * each])
def combine():
"""合并分片,还原文件"""
with open(restore, 'wb') as f:
for i in range(N):
f.write(rb(str(i)))
if __name__ == '__main__':
split()
combine()
切分方式(图右)
import os
SOURCE = '待切文件'
N = 9
path = os.path.dirname(__file__)
source = os.path.join(path, SOURCE)
fragment = os.path.join(path, 'fragment/')
if not os.path.exists(fragment):
os.mkdir(fragment)
restore = os.path.join(fragment, SOURCE)
def rb(abs_path):
"""二进制方式读取文件"""
with open(abs_path, 'rb') as f:
return f.read()
def split():
"""文件切分"""
frb = rb(source)
f_ls = [open(os.path.join(fragment, str(i)), 'wb') for i in range(N)]
for i in range(len(frb)):
if i % 2 == 0:
f_ls[i % N].write(frb[i: i + 2])
for f in f_ls:
f.close()
def combine():
"""合并分片,还原文件"""
f = open(restore, 'wb')
fb_ls = [rb(os.path.join(fragment, str(i))) for i in range(N)]
le = sum(len(frb) for frb in fb_ls)
for i in range(le):
if i % 2 == 0:
f.write(fb_ls[i % N][int(((i / 2) // N) * 2): int(((i / 2) // N) * 2) + 2])
f.close()
def checkout():
"""计算还原率"""
o = rb(source)
t = sum(i == j for i, j in zip(rb(restore), o))
print('还原率', t / len(o))
if __name__ == '__main__':
split()
combine()
checkout()
|