1,构造和析构
1,
class fileobject:
def __init__(self,filename='example.txt'):
self.new_file=open(filename,'r+')
def __del__(self):
self.new_file.close()
del self.new_file
2,
- 定义一个类实现摄氏度到华氏度的转换(转换公式:华氏度 = 摄氏度*1.8+32)?
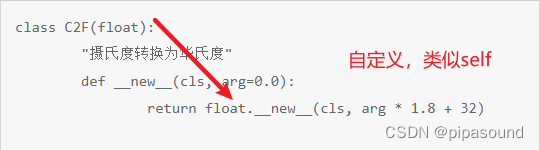
class C2F(float):
def __new__(cls,age=0.0):
return float.__new__(cls,age*1.8+32)
3,?
- ord()函数主要用来返回对应字符的ascii码
- 当传入的参数是字符串的时候,返回该字符串中所有字符的 ASCII 码的和(使用 ord() 获得一个字符的 ASCII 码值)
class Nint(int):
def __new__(cls,age=0):
if isinstance(age,str):
total=0
for each in age:
total+=ord(each)
age=total
return int.__new__(cls,age)
2,算术运算?
- 工厂函数,其实就是一个类对象。当你调用他们的时候事实上就是创建一个相应的实例对象
- 类的属性名和方法名绝对不能相同
- 鸭子类型:关注点在于对象的行为能做什么,而不是关注对象所属的类型。? ? ? ? ? in_the_forest() 函数对参数 duck 只有一个要求:就是可以实现 quack() 和 feathers() 方法。然而 Duck 类和 Person 类都实现了 quack() 和 feathers() 方法,因此它们的实例对象 donald 和 john 都可以用作 in_the_forest() 的参数。这就是鸭子类型。
# I love FishC.com!
class Duck:
def quack(self):
print("呱呱呱!")
def feathers(self):
print("这个鸭子拥有灰白灰白的羽毛。")
class Person:
def quack(self):
print("你才是鸭子你们全家人是鸭子!")
def feathers(self):
print("这个人穿着一件鸭绒大衣。")
def in_the_forest(duck):
duck.quack()
duck.feathers()
def game():
donald = Duck()
john = Person()
in_the_forest(donald)
in_the_forest(john)
>>>game() 呱呱呱! 这个鸭子拥有灰白灰白的羽毛。 你才是鸭子你们全家人是鸭子! 这个人穿着一件鸭绒大衣。?
这里我有个疑问,给类写方法时必须加上self函数,而方法函数中的变量名前有的加了self,有的没加。这里一位师傅的文章写的很清晰--->Python类和对象的self?
- 我们都知道在 Python 中,两个字符串相加会自动拼接字符串,但遗憾的是两个字符串相减却抛出异常。因此,现在我们要求定义一个 Nstr 类,支持字符串的相减操作:A – B,从 A 中去除所有 B 的子字符串,如下
>>> a = Nstr('I love FishC.com!iiiiiiii')
>>> b = Nstr('i')
>>> a - b
'I love FshC.com!'
?class Nstr(str): ? ? def __sub__(self,other): ? ? ? ? return self.replace(other,'')#''替换走other ? ? #记得前面的self.replace里的self,是Nstr类自己的属性变量
- ??移位操作符是应用于二进制操作数的,现在需要你定义一个新的类 Nstr,也支持移位操作符的运算:
>>> a = Nstr('I love FishC.com!')
>>> a << 3
'ove FishC.com!I l'
>>> a >> 3
'om!I love FishC.c'
class Nstr(str): ? ? def __lshift__(self, other): ? ? ? ? return self[other:] + self[:other]?
// << __lshift__? ? ? >>__rshift__
? ? def __rshift__(self, other): ? ? ? ? return self[-other:] + self[:-other]
- ?定义一个类 Nstr,当该类的实例对象间发生的加、减、乘、除运算时,将该对象的所有字符串的 ASCII 码之和进行计算
>>> a = Nstr('FishC') >>> b = Nstr('love') >>> a + b 899 >>> a - b 23 >>> a * b 201918 >>> a / b 1.052511415525114 >>> a // b 1
class Nstr:
def __init__(self,arg=''):#定义空字符串
if isinstance(arg,str):
self.total=0
for each in arg:
self.total+=ord(each)#转换成ASCII码
else:
print("参数错误")
def __add__(self,other):
return self.total+other.total #self,other两个参数
def __sub__(self,other):
return self.total-other.total
def __mul__(self,other):
return self.total*other.total
def __truediv__(self,other):
return self.total/other.total
def __floordiv__(self,other):
return self.total//other.total
- python什么时候会用到反运算魔法方法 ?例如 a + b,如果 a 对象的 __add__ 方法没有实现或者不支持相应的操作,那么 Python 就会自动调用 b 的 __radd__ 方法。
class Nint(int):
def __radd__(self, other):
print("__radd__ 被调用了!")
return int.__add__(self, other)
>>> a = Nint(5) >>> b = Nint(3) >>> a + b 8 >>> 1 + b __radd__ 被调用了! 4
不断更新~?
?
?
|