while 的用法
while作用:只要…条件成立,就一直做…。永远为True的循环,俗称死循环。但在循环过程中,制造某种可以使循环停下来的条件,就可以停止它。要免编写无限循环,务必对每个while环进行测试,确保它像预期那样结束。
1.使用while计数,while的意思就是当条件成立时,执行while内的判断。
count = 0
while True:
print("count:",count)
count=count+1
2.猜年龄游戏,每个人允许猜3次,如果猜对直接退出
count = 0
age_of_oldboy = 56
while True:
if count ==3:
break
guess_age = int(input("guess age:"))
if guess_age ==age_of_oldboy:
print("yes,you got it.")
break
elif guess_age > age_of_oldboy:
print("think smaller...")
else:
print("think bigger!")
count+=1
?? ??
3、略优化上述循环,使用while count 小于3 与if count ==3 break 执行结果一致
count = 0
age_of_oldboy = 56
while count<3:
guess_age = int(input("guess age:"))
if guess_age ==age_of_oldboy:
print("yes,you got it.")
break
elif guess_age > age_of_oldboy:
print("think smaller...")
else:
print("think bigger!")
count+=1
?? ??
4、输入错误答案3次后,需要提示用户不能尝试了
count = 0
age_of_oldboy = 56
while count<3:
guess_age = int(input("guess age:"))
if guess_age ==age_of_oldboy:
print("yes,you got it.")
break
elif guess_age > age_of_oldboy:
print("think smaller...")
else:
print("think bigger!")
count+=1
else:
print("you have tried too many times..fuck off")
?? ??
5、任性玩游戏,当3次跑完后,询问是否继续玩游戏,输入n代表结束,输入任何其他数代表继续
count = 0
age_of_oldboy = 56
while count <3:
guess_age = int(input("guess age:"))
if guess_age ==age_of_oldboy:
print("yes,you got it.")
break
elif guess_age > age_of_oldboy:
print("think smaller...")
else:
print("think bigger!")
count +=1
if count ==3:
countine_confirm = input("do you want to keep guessing..?")
if countine_confirm != "n":
count = 0
else:
print("you have tried too many times..fuck off")
break的意思
break可以理解为破坏的意思,当条件成立执行break后,后面的条件不会在执行。 简单点说,break就是结束整个循环的意思。
for循环
1、简单的for使用方法
for i in range(10):
print("loop",i)
2、使用步长为2,打印10以内的偶数
步长默认为1,可以为2,或者3…
for i in range(0,10,2):
print("loop",i)
在这里插入代码片
3、用for循环执行上段猜年龄游戏
age_of_oldboy = 56
for i in range(3):
guess_age = int(input("guess age:"))
if guess_age ==age_of_oldboy:
print("yes,you got it.")
break
elif guess_age > age_of_oldboy:
print("think smaller...")
else:
print("think bigger!")
else:
print("you have tried too many times..fuck off")
4、双层循环
每个大循环执行1次,小循环就得执行10次
for i in range(10):
print('-------------',i)
for j in range(10):
print(j)
continue用法
1、continue意思:跳出本次循环,继续到下一次循环。
for i in range(0,10):
if i <3:
print("loop",i)
else:
continue
print("hahaha...")
?? ??
2、pycharm打断点调试
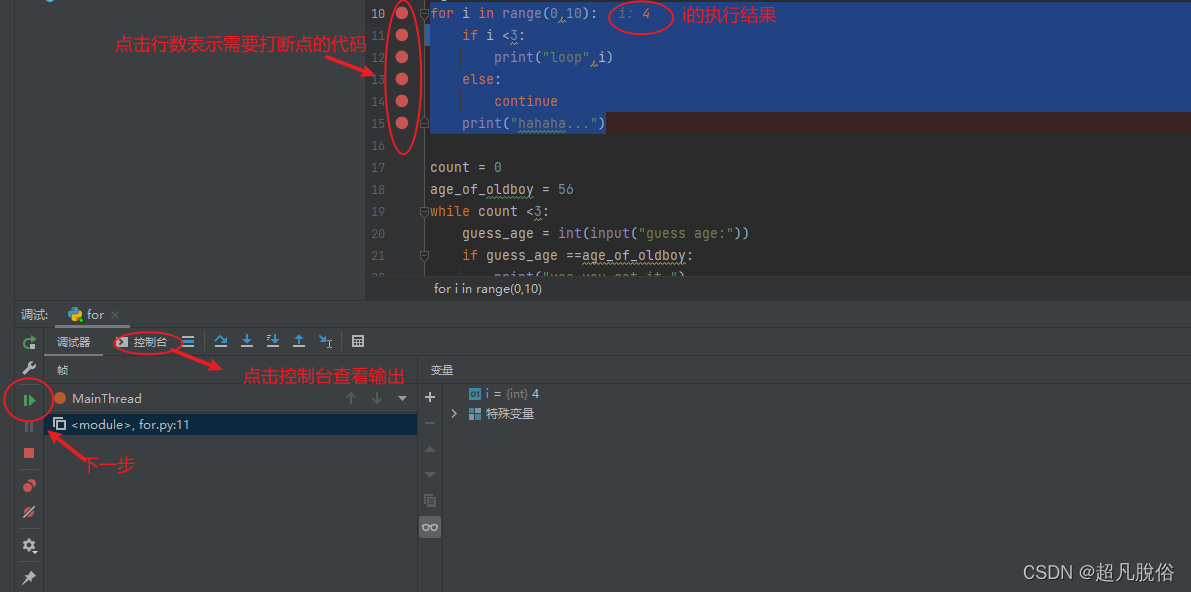
插入一个if else 简单判断,当输入用户账号密码登录,使用if else判断
import getpass
_username = "josh"
_password = "josh123"
username = input("username:")
password = input("password:")
if _username == username and _password == password:
print("Welcome user {name} login...".format(name=username))
else:
print("Invalid username or password!")
|