1.Requests以json形式发送post请求
client.py
import requests
import json
# Requests以json形式发送post请求
data_json = json.dumps({"1":"a", "2":"b", "3":"c", "4":"d"})
res = requests.post(url="http://localhost:8000/",data=data_json)
server.go
package main
import (
"fmt"
"io/ioutil"
"net/http"
)
func main() {
http.HandleFunc("/", myHandle)
http.ListenAndServe(":8000", nil)
}
func myHandle(w http.ResponseWriter, r *http.Request) {
defer r.Body.Close()
con, _ := ioutil.ReadAll(r.Body) //获取post的数据
fmt.Println(con)
fmt.Println("-------------------")
fmt.Println(string(con))
}


注:必须先运行服务端,再运行客户端,否则会报错?requests.exceptions.ConnectionError: HTTPConnectionPool(host='localhost', port=8000): Max retries exceeded with url: / (Caused by NewConnectionError('<urllib3.connection.HTTPConnection object at 0x102d822e0>: Failed to establish a new connection: [Errno 61] Connection refused'))
2.Requests以form表单形式发送post请求
client.py
import requests
data = {"1":"a", "2":"b", "3":"c", "4":"d"}
res = requests.post(url="http://localhost:8000/",data=data)

例1
client.py
import requests
import json
data_json = json.dumps({"k1":"a", "k2":"b", "k3":"c", "k4":"d"})
res = requests.post(url="http://localhost:8000/",data=data_json)
server.go
/*
* @Author: your name
* @Date: 2021-12-22 10:55:07
* @LastEditTime: 2021-12-23 21:25:00
* @LastEditors: Please set LastEditors
* @Description: 打开koroFileHeader查看配置 进行设置: https://github.com/OBKoro1/koro1FileHeader/wiki/%E9%85%8D%E7%BD%AE
* @FilePath: /script/t0.go
*/
package main
import (
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
)
type Data struct {
K1 string `json:"k1"`
K2 string `json:"k2"`
K3 string `json:"k3"`
K4 string `json:"k4"`
}
func main() {
http.HandleFunc("/", myHandle)
http.ListenAndServe(":8000", nil)
}
func myHandle(w http.ResponseWriter, r *http.Request) {
defer r.Body.Close()
con, _ := ioutil.ReadAll(r.Body) //获取post的数据
conStr := string(con)
// fmt.Println(con)
// fmt.Println("----------------------------")
fmt.Println(conStr)
fmt.Println("----------------------------")
var data Data
// 字节数组(con) 转 json(Data)
if err := json.Unmarshal(con, &data); err == nil {
fmt.Println(data)
fmt.Println(data.K1)
fmt.Println(data.K2)
fmt.Println(data.K3)
fmt.Println(data.K4)
} else {
fmt.Println(err)
}
}
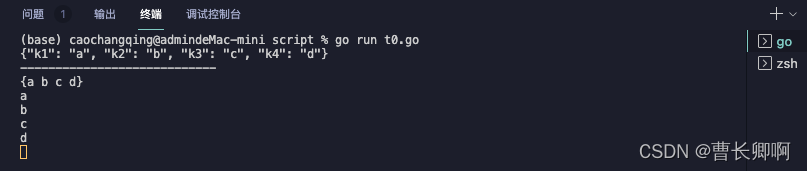
注:如需string转json,则如下
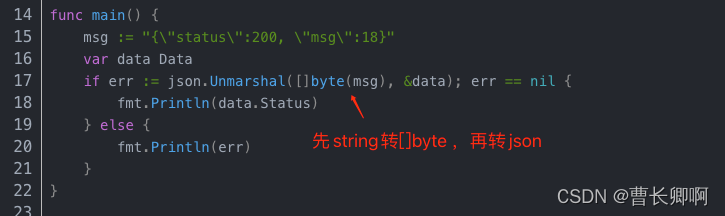
?
|