零基础学python day03 python基础
掌握python基础中的必备语法知识
1.循环语句
1.1 while循环
while 条件:
...
...
...
1.2 break
break 用于在while循环中终止循环 例
print("start")
while True:
print("1")
break
print("2")
print("end")
'''
start
1
end
'''
1.3continue
continue 用于结束本次循环,直接开始进行下一次循环 例
print("start")
while True:
print(1)
continue
print(2)
print(3)
print("end")
执行结果: start 1 1 1 …
1.5 while else
当while后的条件不成立时,else中的代码就会执行
while 条件:
代码
else:
代码
2. 字符串格式化
字符串格式化,使用更便捷的形式实现字符串的拼接
2.1 %
2.1.1 基本格式化操作
name = "水饺"
text = "我叫%s,今年18岁" %name
2.1.2 百分比的处理
text = "%s,下载进度90%%" %"您好"
使用两个%%
2.2 format(推荐)
text = "%s,下载进度90%%" %"您好"
text = "我叫{0},今年28岁".format("水饺")
text = "我叫{0},今年{1}岁".format("水饺", 18)
text = "我叫{0},今年{1}岁,真实的姓名是{0}".format("水饺", 18)
text = "我叫{},今年28岁".format("水饺")
text = "我叫{},今年{}岁".format("水饺", 18)
text = "我叫{},今年{}岁,真实的姓名是{}".format("水饺", 18, "水饺")
text = "我叫{name},今年28岁".format(name="水饺")
text = "我叫{name},今年{age}岁,真实的姓名是{name}".format(name="水饺", age=18)
text = "我叫{0},今年{1}岁"
data1 = text.format("水饺", 18)
2.3 f
python3.6及以上版本才能使用,更便捷
name = "shuijiao"
age = 18
text = f"my name is {name},{age} year's old"
print(text)
运行结果 
name = "shuijiao"
age = 18
text = f"my name is {name},{age+2} year's old"
print(text)
运行结果 
python3.8引入新功能
name = "shuijiao"
age = 18
text = f"my name is {name},{age+2=} year's old"
print(text)
运行结果  ** 进制转换**
v1 = f"我今年{22}岁"
v2= f"我今年{22:#b}岁"
v3 = f"我今年{22:#o}岁"
v4 = f"我今年{22:#x}岁"
print("v1="+v1)
print("v2="+v2)
print("v3="+v3)
print("v4="+v4)
运行结果 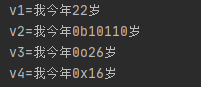
3.运算符
** 幂 // 取整数 != 不等 in 包含? not in 不包含?
3.1 运算符优先级
- 算数优先级大于比较优先级
- 比较运算符优先级大于逻辑运算符
- 逻辑运算符内部三个优先级 not >and >or
加减乘除>比较> not and or
3.2 面试题
逻辑运算中and or
例
v5 = "shuijiap" and "水饺"
print("v5="+v5)
运行结果  原因 运行第一步,将and前后的值转换为布尔值 True and True 第二步判断本次操作的值取决于谁,由于前面的是True,所以取决于后面的值, 所以后面的值是多少最终的值就是多少
面对多个not\and和or的情况,先计算not、再计算and,再计算or 例 0 or 4 and 3 or 7 or9 and 6 = 3 not 8 or 3 and 4 or 2 = 4
|