代码简单明了, 在python 2.7中运行
代码
import json
import traceback
if __name__ == '__main__':
key1 = "key1"
key2 = "key2"
dict1 = {key1: 123, key2: "v2"}
dict2 = {"all_params": dict1}
jsonStr1 = json.dumps(dict2["all_params"])
print "originalDict:%s, json str1:%s" % (dict2["all_params"], jsonStr1)
print "\n"
dict2["param_values"] = json.dumps([dict2["all_params"]])
dict2["param_values2"] = dict2["all_params"]
print "add param_values(value is json str) and param_values2(value is a dict) to dict"
print "dict2:%s" % dict2
print "\n"
print 'dict2["param_values"] is str and is json array str, it can be converted into dict'
tmp1 = json.loads(dict2["param_values"])
print "tmp1 Type:%s" % type(tmp1)
valueFromJsonKeyOne1 = tmp1[0]
print "valueFromJsonKeyOne1:%s" % valueFromJsonKeyOne1
print "\n"
print 'dict2["param_values2"] is not str and it is a json, it can\'t be converted into dict'
try:
tmp2 = json.loads(dict2["param_values2"])
print "tmp2 Type:%s" % type(tmp2)
print "tmp2:%s" % tmp2
print "\n"
except Exception as ex:
print'when converting json, ex:', ex
print 'traceback.format_exc():\n%s' % traceback.format_exc()
dict3 = {}
dict3.update(valueFromJsonKeyOne1)
print "new_dict is empty, update new_dict by valueFromJsonKeyOne"
print "new_dict:%s" % dict3
for k, v in dict3.items():
print "%s:%s" % (k, v)
print "jsonStr1 is json str, it can be converted into dict"
tmpDict2 = json.loads(jsonStr1)
print "tmpDict2:%s" % tmpDict2
print "tmpDict2[0]:%s" % tmpDict2[0]
print "\n"
print "done"
调试结果
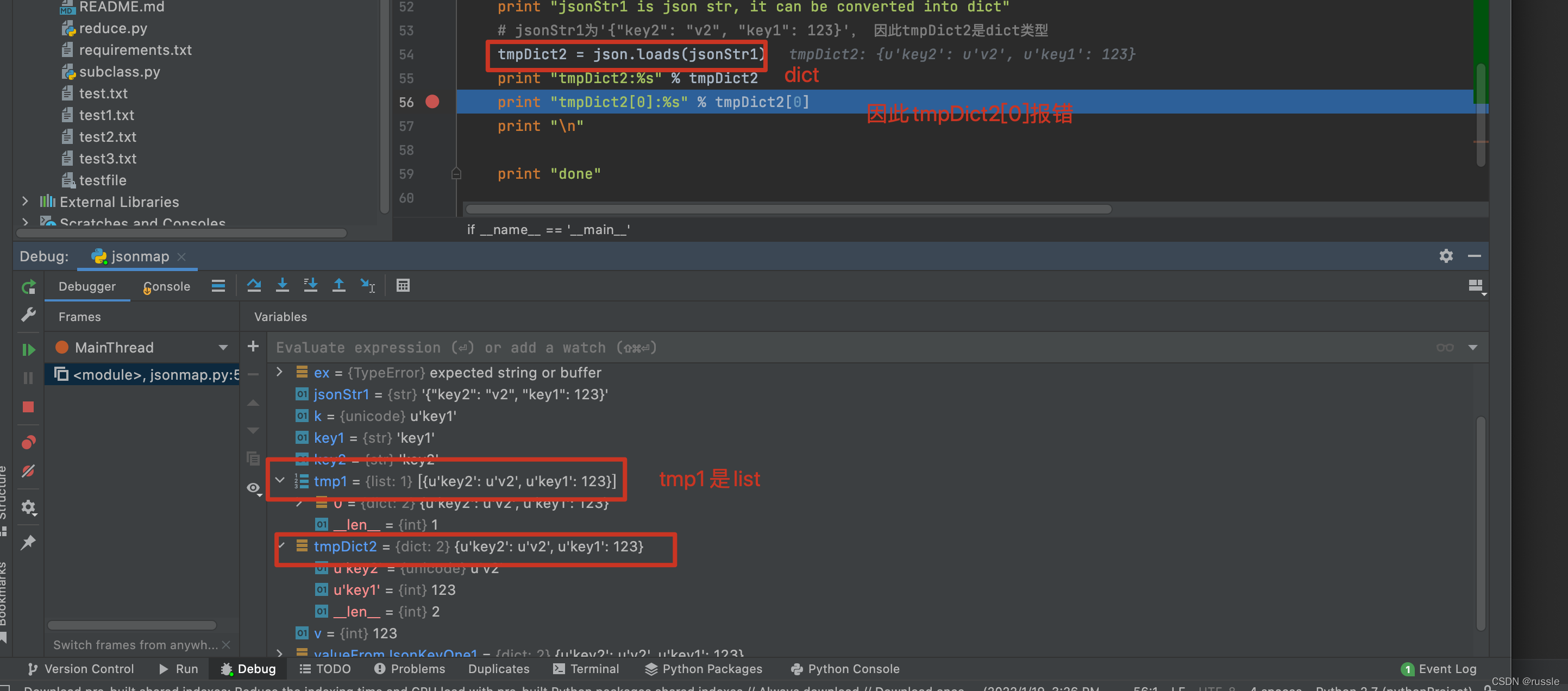
运行结果
Traceback (most recent call last):
File "/Users/ericyang/PycharmProjects/pythonProject/jsonmap.py", line 56, in <module>
print "tmpDict2[0]:%s" % tmpDict2[0]
KeyError: 0
originalDict:{'key2': 'v2', 'key1': 123}, json str1:{"key2": "v2", "key1": 123}
add param_values(value is json str) and param_values2(value is a dict) to dict
dict2:{'param_values': '[{"key2": "v2", "key1": 123}]', 'all_params': {'key2': 'v2', 'key1': 123}, 'param_values2': {'key2': 'v2', 'key1': 123}}
dict2["param_values"] is str and is json array str, it can be converted into dict
tmp1 Type:<type 'list'>
valueFromJsonKeyOne1:{u'key2': u'v2', u'key1': 123}
dict2["param_values2"] is not str and it is a json, it can't be converted into dict
when converting json, ex: expected string or buffer
traceback.format_exc():
Traceback (most recent call last):
File "/Users/ericyang/PycharmProjects/pythonProject/jsonmap.py", line 36, in <module>
tmp2 = json.loads(dict2["param_values2"])
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/json/__init__.py", line 339, in loads
return _default_decoder.decode(s)
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/json/decoder.py", line 364, in decode
obj, end = self.raw_decode(s, idx=_w(s, 0).end())
TypeError: expected string or buffer
new_dict is empty, update new_dict by valueFromJsonKeyOne
new_dict:{u'key2': u'v2', u'key1': 123}
key2:v2
key1:123
jsonStr1 is json str, it can be converted into dict
tmpDict2:{u'key2': u'v2', u'key1': 123}
Process finished with exit code 1
|