一、os.path
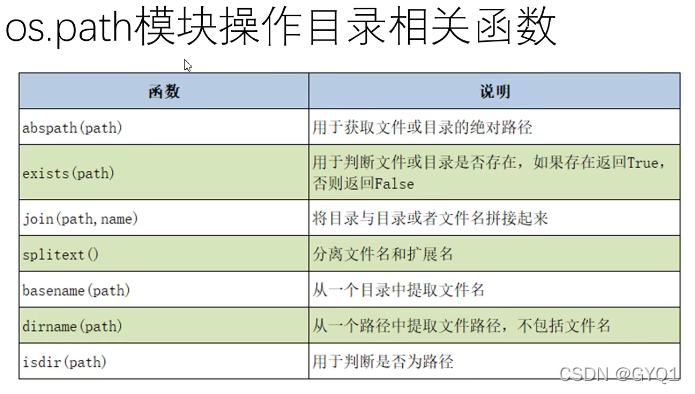
import os.path
print(os.path.abspath('day2.py')) # 返回绝对路径
print(os.path.exists('a.txt')) # 返回文件是否存在
print(os.path.join('D:\\Py file\\pythonProject', 'day2.py')) # 将目录和文件名拼接起来
print(os.path.split('D:\\Py file\\pythonProject\\day2.py')) # 将目录和文件拆分
print(os.path.splitext('day2.py')) # 将文件名拆分
print(os.path.basename('D:\\Py file\\pythonProject\\day2.py')) # 提取文件名
print(os.path.dirname('D:\\Py file\\pythonProject\\day2.py')) # 提取目录
print(os.path.isdir('D:\\Py file\\pythonProject\\day2.py')) # 判断是否是目录
获取当前目录下所有.py文件
import os.path
path = os.getcwd()
flist = os.listdir(path)
for i in flist:
if i.endswith('.py'):
print(i, end='\t')
os.walk(path)#返回一个可迭代对象,path中所有目录,文件
二、学生管理系统(写bug)
import os
import re
def menu():
print('菜单')
print('-------------------------------------------------------')
print('1.录入学生信息')
print('2.查找学生信息')
print('3.删除学生信息')
print('4.修改学生信息')
print('5.统计学生总人数')
print('6.显示所有学生信息')
print('0.退出系统')
print('------------------------------------------------------')
def insert():
id = input('添加的学生id为:')
name = input('学生姓名为:')
english = int(input(name + '的英语成绩为:'))
python = int(input(name + '的python成绩为:'))
java = int(input(name + '的java成绩为:'))
dict = {'id': id, 'name': name, 'english': english, 'python': python, 'java': java}
with open('./student.txt', 'a') as fp:
fp.write(str(dict) + '\n')
answer = input('信息录入成功是否继续Y/N')
if answer == 'y' or answer == 'Y':
insert()
else:
pass
def show_student(lst):
if len(lst) == 0:
print('没有查询到学生信息')
return
format_title = '{:^6}\t{:^12}\t{:^8}\t{:^10}\t{:^10}\t{:^8}'
print(format_title.format('ID', '姓名', '英语成绩', 'Python成绩', 'java成绩', '总成绩'))
format_data = '{:^6}\t{:^12}\t{:^8}\t{:^10}\t{:^10}\t{:^8}'
for dic in lst:
print(format_data.format(dic.get('id'),
dic.get('name'),
dic.get('english'),
dic.get('python'),
dic.get('java'),
int(dic.get('english')) + int(dic.get('python')) + int(dic.get('java'))
))
def search():
while True:
with open('./student.txt', 'r') as fp:
res = fp.readlines()
id = ''
list = []
name = ''
if os.path.exists('./student.txt'):
choice = int(input('按id查找请输入0,按姓名查找请按1:'))
if choice in [0, 1]:
if choice == 0:
id = str(input('请输入要查找的id:'))
if choice == 1:
name = input('请输入要查找的学生姓名:')
for item in res:
d = dict(eval(item))
if id != '':
if d['id'] == id:
list.append(d)
else:
if d['name'] == name:
list.append(d)
if len(list) == 0:
print('没有查询到结果!')
answer = input(('是否继续Y/N'))
if answer == 'Y' or answer == 'y':
search()
else:
return
else:
show_student(list)
list = ''
print('查询结束')
answer = input(('是否继续Y/N'))
if answer == 'Y' or answer == 'y':
search()
else:
break
else:
print('选错了!')
continue
else:
print('暂未保存学员信息!')
return
def delete():
while True:
flag = False
stu_id = input('请输入要删除的学生的ID:')
if stu_id != '':
with open('./student.txt', 'r') as fp:
stu_all = fp.readlines()
if stu_all:
with open('./student.txt', 'w') as fp:
for item in stu_all:
a = dict(eval(item))
if a['id'] != stu_id:
fp.write(str(a) + '\n')
else:
flag = True
if flag:
print('id为' + stu_id + '的学生信息已删除!')
else:
print('没有id为:' + stu_id + '的学生数据')
else:
print('没有一条数据,先录入学生信息吧')
insert()
return
show()
answer = input(('是否继续Y/N'))
if answer == 'Y' or answer == 'y':
delete()
else:
break
def modify():
list = []
show()
with open('./student.txt', 'r') as fp:
stu_all = fp.readlines()
id = input('请输入要修改的学员id:')
for item in stu_all:
a = dict(eval(item))
list.append(a['id'])
if a['id'] == id:
print('找到该学生信息,可以修改了!')
a['name'] = input('请输入姓名')
a['english'] = input('请输入英语成绩')
a['python'] = input('请输入python成绩')
a['java'] = input('请输入java成绩')
with open('./student.txt', 'w') as fp:
fp.write(str(a) + '\n')
fp.close()
print('修改成功')
else:
with open('./student.txt', 'w') as fp1:
fp1.write(str(a) + '\n')
fp1.close()
if list:
if id not in list:
print('没有此id对应学生信息,请重新输入')
modify()
else:
print('没有数据,请添加!')
insert()
return
answer = input(('是否继续修改Y/N'))
if answer == 'Y' or answer == 'y':
modify()
else:
return
def total():
with open('./student.txt', 'r') as fp:
res = fp.readlines()
if len(res) > 0:
print('共有{}名学生'.format(len(res)))
else:
print('还没有录入学生数据')
def show():
stu_list = []
with open('./student.txt', 'r') as fp:
students = fp.readlines()
for item in students:
d = dict(eval(item))
stu_list.append(d)
if stu_list:
show_student(stu_list)
else:
print('没有数据,录入一些吧!')
if __name__ == '__main__':
while True:
menu()
choice = int(input('请输入您的选择:'))
if choice in [0, 1, 2, 3, 4, 5, 6, 7]:
if choice == 0:
answer = input('您确定要退出系统吗?Y/N')
if answer == 'Y' or answer == 'y':
print('拜拜了!')
break
else:
continue
elif choice == 1:
insert()
elif choice == 2:
search()
elif choice == 3:
delete()
elif choice == 4:
modify()
elif choice == 5:
total()
elif choice == 6:
show()
else:
print('选择已有的功能!')
continue
三、项目打包让其可以在没有python环境的电脑运行
安装第三方模块:
win+R->cmd->pip install Pyinstaller
打包:
在命令行窗口输入:pyinstaller -F? D:\Py_flie\pythonProject\day5.py(py文件绝对路径)
结果如下:
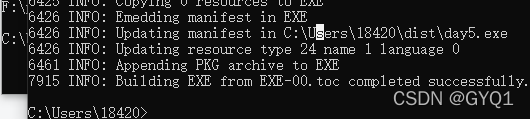
在倒数第4行可以看到生成的.exe文件的目录
找到运行即可:
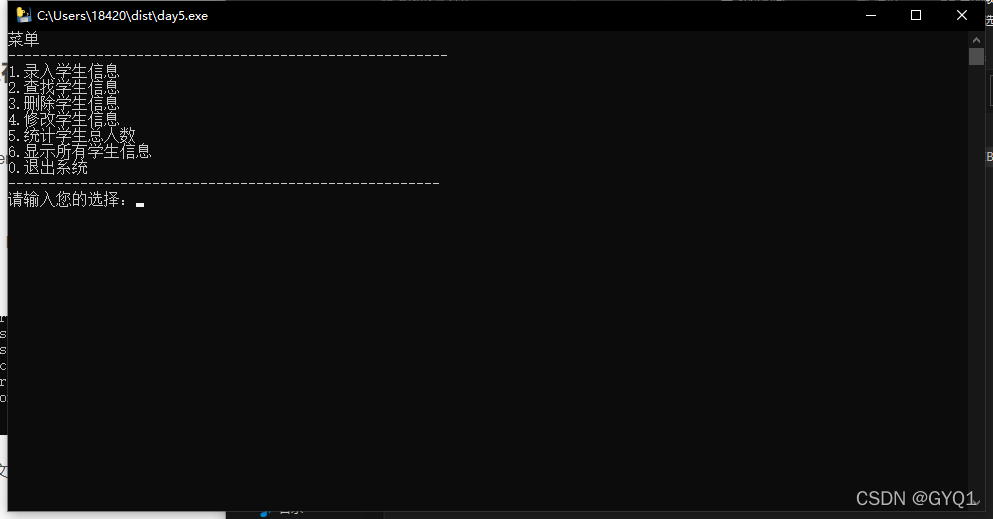
?完成
?
|