最近写了一个python库叫fastgame ,下载方式:
pip install fastgame -i https://pypi.org/project/
用了下Github Page ,文档在https://stripepython.github.io/fastgame-document/
创建游戏:
from fastgame import FastGame
game = FastGame()
写贪吃蛇:
import random
import fastgame
from fastgame.utils.color import *
SNAKE_WIDTH = 20
WIDTH = 850
HEIGHT = 700
game = fastgame.FastGame('Snake', size=(WIDTH, HEIGHT), fps=10)
canvas = fastgame.Canvas(size=(WIDTH, HEIGHT), bgcolor=WHITE)
pen = canvas.init_pen()
score_text = fastgame.Label('Score: 0', size=36, color=BLUE)
snake = [
[WIDTH // 2, HEIGHT // 2 - SNAKE_WIDTH],
[WIDTH // 2, HEIGHT // 2],
[WIDTH // 2, HEIGHT // 2 + SNAKE_WIDTH],
[WIDTH // 2, HEIGHT // 2 + SNAKE_WIDTH * 2]
]
head = snake[0].copy()
angel = 'left'
score = 0
def get_food():
x = random.randint(SNAKE_WIDTH, WIDTH - SNAKE_WIDTH)
y = random.randint(SNAKE_WIDTH, HEIGHT - SNAKE_WIDTH)
x -= x % SNAKE_WIDTH
y -= y % SNAKE_WIDTH
return [x, y]
def eaten_food():
x0, y0 = food
x1, y1 = head
return abs(x0 - x1) <= SNAKE_WIDTH and abs(y0 - y1) <= SNAKE_WIDTH
def move(move_angel: str = 'left'):
global angel, food, score
angel = move_angel
if eaten_food():
score += 1
food = get_food()
else:
snake.pop()
if move_angel == 'left':
head[0] -= SNAKE_WIDTH
elif move_angel == 'right':
head[0] += SNAKE_WIDTH
elif move_angel == 'up':
head[1] -= SNAKE_WIDTH
elif move_angel == 'down':
head[1] += SNAKE_WIDTH
snake.insert(0, head.copy())
def dead():
body = snake.copy()
del body[0]
return (
(head in body)
or head[0] <= 0
or head[0] >= WIDTH
or head[1] <= 0
or head[1] >= HEIGHT
)
food = get_food()
@game.update
def update(over=False):
pen.fill_screen(PINK)
score_text.set_text(f'Score: {score}')
score_text.update()
pen.set_color(WHITE)
for body in snake:
pen.rectangle(*body, (SNAKE_WIDTH, SNAKE_WIDTH))
pen.set_color(BLACK)
pen.rectangle(*head, (SNAKE_WIDTH, SNAKE_WIDTH))
pen.set_color(GREEN)
pen.rectangle(*food, (SNAKE_WIDTH, SNAKE_WIDTH))
move(angel)
if dead():
if not over:
update(True)
game_over()
def game_over():
over_sprite = fastgame.screenshot()
game_over_text = fastgame.Label('Game Over', size=96, color=WHITE)
game_over_text.position = (0, HEIGHT // 2)
@game.update
def reset_update():
over_sprite.update()
game_over_text.update()
@game.on_key_down
def on_key_down():
global angel
key = game.event.get('key')
if key == fastgame.K_LEFT and angel != 'right':
angel = 'left'
elif key == fastgame.K_RIGHT and angel != 'left':
angel = 'right'
elif key == fastgame.K_UP and angel != 'down':
angel = 'up'
elif key == fastgame.K_DOWN and angel != 'up':
angel = 'down'
if __name__ == '__main__':
game.mainloop()
效果: 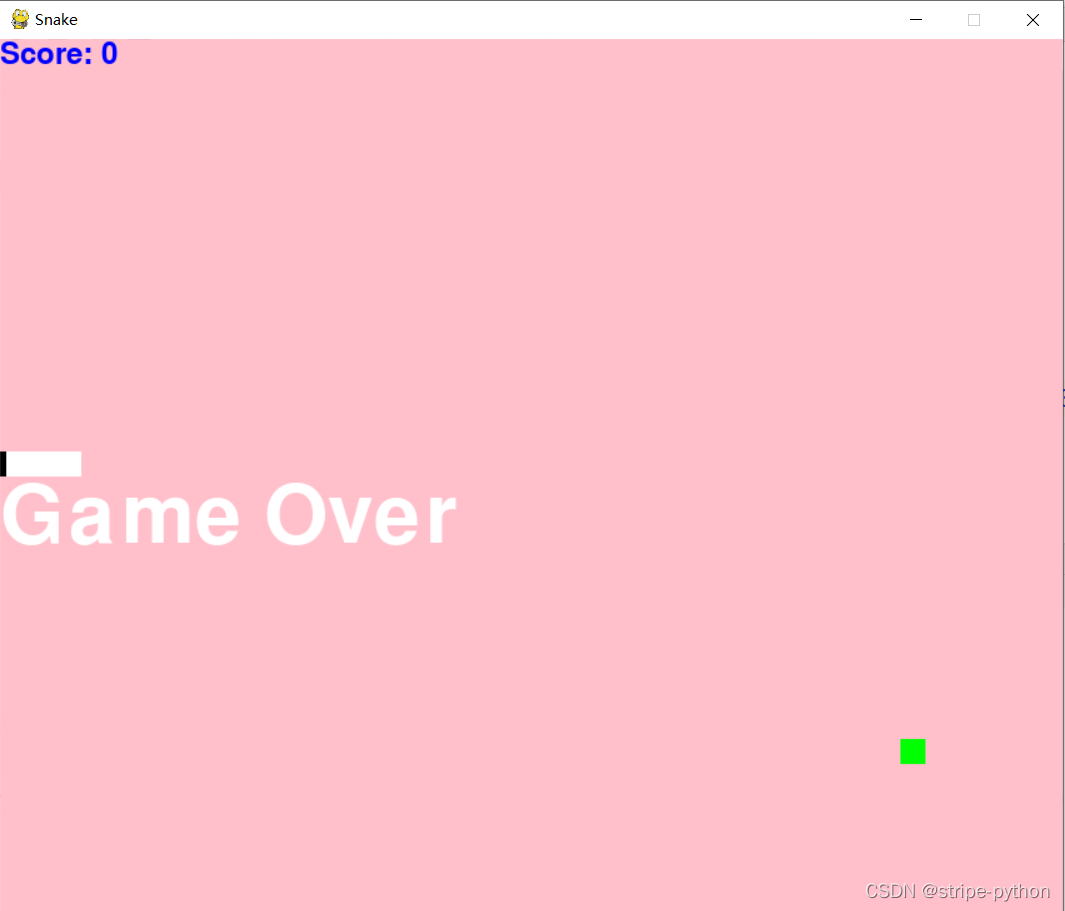
|