PyQt5 message box
默认情况下,如果我们点击标题栏上的 x 按钮,QWidget 会关闭。有时我们想修改这个默认行为。例如,如果我们在编辑器中打开了一个文件,我们对其进行了一些更改。我们显示一个消息框来确认操作。
messagebox.py
#!/usr/bin/python
"""
ZetCode PyQt5 tutorial
This program shows a confirmation
message box when we click on the close
button of the application window.
Author: Jan Bodnar
Website: zetcode.com
"""
import sys
from PyQt5.QtWidgets import QWidget, QMessageBox, QApplication
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(300, 300, 250, 150)
self.setWindowTitle('Message box')
self.show()
def closeEvent(self, event):
reply = QMessageBox.question(self, 'Message',
"Are you sure to quit?", QMessageBox.Yes |
QMessageBox.No, QMessageBox.No)
if reply == QMessageBox.Yes:
event.accept()
else:
event.ignore()
def main():
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
如果我们关闭 QWidget,则会生成 QCloseEvent。要修改小部件行为,我们需要重新实现 closeEvent 事件处理程序。
reply = QMessageBox.question(self, 'Message',
"Are you sure to quit?", QMessageBox.Yes |
QMessageBox.No, QMessageBox.No)
我们显示一个带有两个按钮的消息框:是和否。第一个字符串出现在标题栏上。第二个字符串是对话框显示的消息文本。第三个参数指定出现在对话框中的按钮组合。最后一个参数是默认按钮。它是最初具有键盘焦点的按钮。返回值存储在回复变量中。
if reply == QtGui.QMessageBox.Yes:
event.accept()
else:
event.ignore()
这里我们测试返回值。如果我们单击 Yes 按钮,我们将接受导致窗口小部件关闭和应用程序终止的事件。否则我们忽略关闭事件。
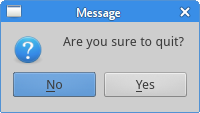
Figure: Message box
Centering window on the screen
以下脚本显示了我们如何在桌面屏幕上居中放置一个窗口。
center.py
#!/usr/bin/python
"""
ZetCode PyQt5 tutorial
This program centers a window
on the screen.
Author: Jan Bodnar
Website: zetcode.com
"""
import sys
from PyQt5.QtWidgets import QWidget, QDesktopWidget, QApplication
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.resize(250, 150)
self.center()
self.setWindowTitle('Center')
self.show()
def center(self):
qr = self.frameGeometry()
cp = QDesktopWidget().availableGeometry().center()
qr.moveCenter(cp)
self.move(qr.topLeft())
def main():
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
QDesktopWidget 类提供有关用户桌面的信息,包括屏幕大小。
self.center()
将窗口居中的代码放置在自定义中心方法中
qr = self.frameGeometry()
我们得到一个指定主窗口几何形状的矩形。这包括任何窗框。
cp = QDesktopWidget().availableGeometry().center()
我们计算出显示器的屏幕分辨率。从这个分辨率,我们得到中心点。
qr.moveCenter(cp)
我们的矩形已经有了它的宽度和高度。现在我们将矩形的中心设置为屏幕的中心。矩形的大小不变。
self.move(qr.topLeft())
我们将应用程序窗口的左上角移动到 qr 矩形的左上角,从而使窗口在屏幕上居中。
在 PyQt5 教程的这一部分中,我们在 PyQt5 中创建了简单的代码示例。
|