flask框架(点点点工程师自我代码修养)
1. 实例项目–鱼书
1. 思维导图
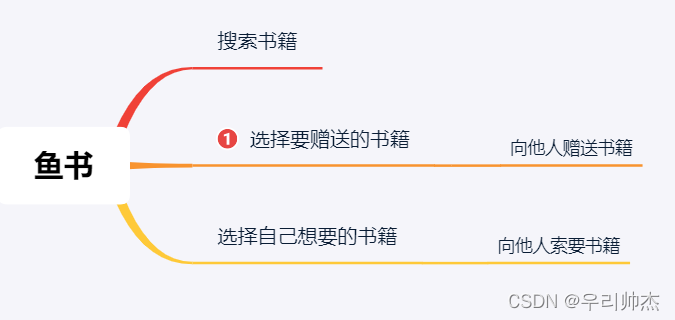 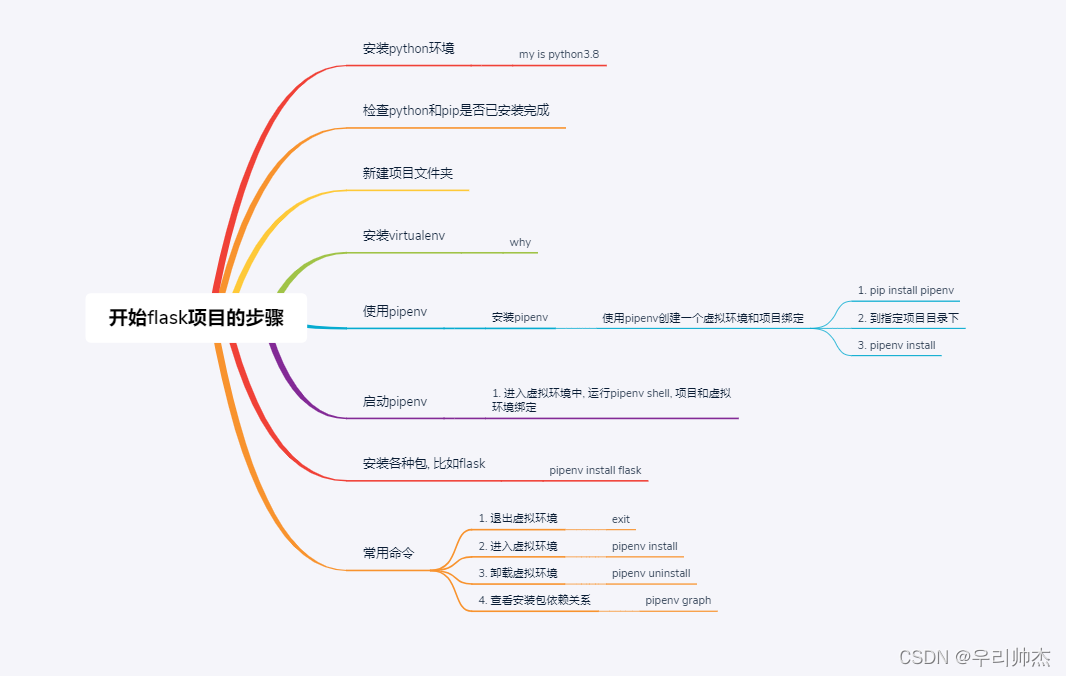
2. 开发工具 – 根据喜欢
- pycharm
- mysql
- navaicat
3. 编写一个最小的flask应用
- 如何在pycharm中设置虚拟环境:
File – settings – Project: fisher – Project Interpreter选择虚拟目录的路径 查看路径方式: 进入项目 – pipenv venv
1. 初步服务器启动
from flask import Flask
app = Flask(__name__)
app.run()
2. flask最小原型与唯一URL原则
from flask import Flask
app = Flask(__name__)
@app.route('/hello')
def hello():
return "Hello"
app.run()
3. 路由的另一种注册方法
- 自动重启服务器(解决每次修改代码都需要重启的问题)----开启调试模式
from flask import Flask
app = Flask(__name__)
@app.route('/hello')
def hello():
return 'hello'
app.run(debug=True)
- 另一种注册方式
- 即插视图必须得使用add_url_rule来注册
import flask from Flak
app = Flak(__name__)
def hello():
return "hello"
app.add_url_rule("hello", view_func=hello)
app.run(debug=True)
4. app.run相关参数与flask配置文件
from flask import Flask
app = Flask(__name__)
@app.route('/hello')
def hello():
return "hello"
app.run(debug=True, host="0.0.0.0", port=81)
- flask配置文件
fisher.py
from flask import Flask
app = Flask(__name__)
app.config.from_object('config')
@app.route('/hello')
def hello():
return "hello"
if__name__ == '__main__'
app.run(debug=app.config['DEBUG'], host='0.0.0.0', port=81)
5. 响应对象response
- 视图函数返回结果
????不仅仅返回字符串,还有以下 ????????(1)status code 200, 404, 500 ????????(2)content-type = text/html, http, headers ????????(3)Response - 创建response对象方法
(1)第一种方式
from flask import Flask, make_response
app = Flask(__name__)
app.config.from_object('config')
@app.route('/hello')
def hello():
headers = {
'content-type': 'application/json',
'location': 'http://www.bing.com'
}
response = make_response('<html></html>', 301)
response.headers = headers
return response
if __name__ == '__main__'
app.run(host='0.0.0.0', port=81, debug=app.config['DEBUG'])
(2)第二种方式
from flask import Flask
app = Flask(__name__)
app.config.from_object('config')
@app.route('/hello')
def hello():
headers = {
'content-type': 'application/json',
'location': 'http://www.bing.com'
}
return '<html></hrml>', 301, headers
if __name__ == '__main__'
app.run(host='0.0.0.0', port=81, debug=app.config["DEBUG"])
6. requests发送http请求以及代码优化
- fisher.py文件
from flask import Flask
from helper import is_isbn_or_key
app = Flask(__name__)
app.config.from_object('config')
@app.route('/book/search/<q>/<page>')
def search(q, page):
isbn_or_key = is_isbn_or_key(q)
if __name__ == 'main':
app.run(host='0.0.0.0', port=81, debug=app.config['DEBUG'])
- helper.py文件
def is_isbn_or_key(work):
isbn_or_key = 'key'
if len(word)==13 and word.isdisgit():
isbn_or_key = 'isbn'
if '-' in word and len(word)==10 and word.replace('-', '').isdisgit():
isbn_or_key = 'isbn'
return isbn_or_key
- config.py文件
DEBUG = True
- http.py文件
import requests
class HTTP:
def get(self, url, return_json=True):
r = requests.get(url)
if r.statu_code !=200:
return {} if return_json else ''
return r.json() if return_json else r.text
|