一、元素定位
1、通过id属性定位
driver.find_element_by_id(' ')
2、通过class属性定位
driver.find_elements_by_class_name()
3、通过xpath属性定位
driver.find_elements_by_xpath()
driver.find_elements_by_xpath(‘//*[@text="全程班" and @id=" " ’) 通过标签中的某属性去定位
4、通过Uiselector定位
(1)定位表达式写法:new UiSelector().方法(参数) (2)多种方式定位:new UISelector().方法(参数).方法(参数) (3)可调用方法的API文档:https://www.apiref.com/android-zh/android/support/test/uiautomator/UiSelector.html
driver.find_elements_by_android_uiautomator('new UiSelector().text("xxx")').click #通过文本定位,比xpath更高效
常用的方法:
description():对应的是content-desc属性
resourceID():同by_id()的用法
text():通过text值定位
textContains():文本比较长的时候,可以用textContains模糊匹配,只要文本包含匹配内容就可以
textStartsWith():是以某个文本开头的匹配
textMatches():正则匹配,这个需要配合正则表达式
5、通过description属性定位
driver.find_element_by_accessibility_id():对应的字段是content-desc
二、元素的基本操作方式
1、点击:click() 2、输入:send_keys() 3、获取元素文本:text() 4、获取元素属性:get_attribute() 5、清空输入框:clear()
例如:获取元素文本
ele = driver.find_element_by_id('id属性值')
r1 = ele.text()
三、三大等待
1、隐式等待
driver.implicitly_wait(100000)
2、显示等待
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from appium.webdriver.common.mobileby import MobileBy #需要导的包
locator = (MobileBy.ANDROID_UIAUTOMATOR,'new UiSelect().text("")')
locator = (By.ANDROID_UIAUTOMATOR,'new UiSelect().text("")')
locator = (MobileBy.ANDROID_UIAUTOMATOR,'new UiSelect().text("")')
WebDriverWait(driver,20,0.5).until(
EC.visibility_of_element_located(locator)
)
解释:
WebDriverWait(driver, timeout, poll_frequency=0.5, ignored_exceptions=None)
driver:WebDriver 的驱动程序(Ie, Firefox, Chrome 或远程)
timeout:最长超时时间,默认以秒为单位
poll_frequency:休眠时间的间隔(步长)时间,默认为 0.5 秒
ignored_exceptions:超时后的异常信息,默认情况下抛
MobileBy和By是继承关系,MobileBy包含了By
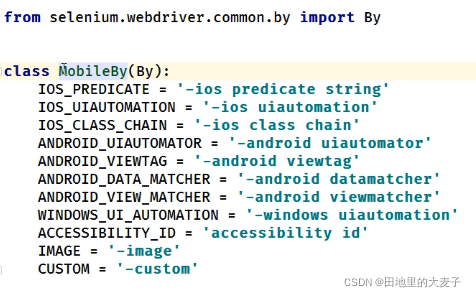 3、强制等待
import time
time.sleep(5)
|