同步:超市有5个土豆,而我想买50个土豆
from time import sleep
class Potato:
@classmethod
def make(cls, num, *args, **kws):
potatos = []
for i in range(num):
potatos.append(cls.__new__(cls, *args, **kws))
return potatos
#创建超市列表,放5个土豆
all_potatos = Potato.make(5)
def take_potatos(num):
count = 0
while True:
#判断有无土豆,没有就等待
if len(all_potatos) == 0:
sleep(.1)
else:
#有就删除一个,即在bucket篮子里加入一个
potato = all_potatos.pop()
yield potato
count += 1
#当土豆数买够数量就停
if count == num:
break
#买土豆
def buy_potatos():
bucket = []
for p in take_potatos(50):
bucket.append(p)
print(bucket)
buy_potatos()
上述结局由于是同步的就是只能一直等,因为超市只有5个,而我想买50个,没买够只能等
异步:买土豆
import asyncio
import random
from time import sleep
class Potato:
@classmethod
def make(cls, num, *args, **kws):
potatos = []
for i in range(num):
potatos.append(cls.__new__(cls, *args, **kws))
return potatos
#创建超市列表,放5个土豆
all_potatos = Potato.make(5)
#异步买土豆
async def take_potatos(num):
count = 0
while True:
#判断有无土豆,没有就等待
if len(all_potatos) == 0:
#等待超市采购土豆
await ask_potatos()
else:
#有就删除一个,即在bucket篮子里加入一个
potato = all_potatos.pop()
yield potato
count += 1
#当土豆数买够数量就停
if count == num:
break
#超市去买土豆存货,放到超市列表
async def ask_potatos():
await asyncio.sleep(random.random())
all_potatos.extend(Potato.make(random.randint(1, 10)))
#买土豆
async def buy_potatos():
bucket = []
number = 0
async for p in take_potatos(50):
bucket.append(p)
print('买编号为 %d,第%d个土豆'%(id(p),number+1))
number+=1
def main():
import asyncio
loop = asyncio.get_event_loop()
res = loop.run_until_complete(buy_potatos())
loop.close()
main()
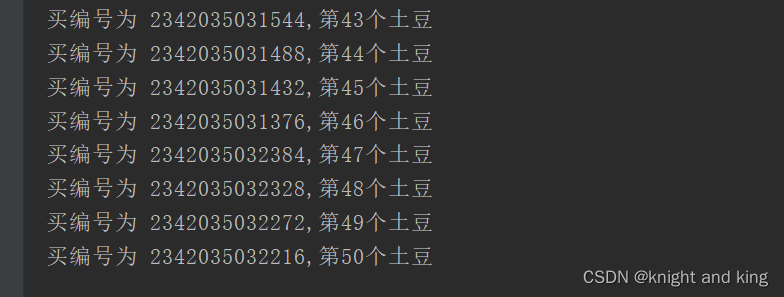
?超市土豆不够了就异步去别的地方采购土豆,我等超市采购完土豆(当生产者完成和返回之后,这是便能从await挂起的地方继续往下跑,完成消费的过程。),然后我再买土豆,买够五十个就停了
因为是异步,所以我们也可以在超市采购土豆的时候,去干别的(如买西红柿)
import asyncio
import random
from time import sleep
class Potato:
@classmethod
def make(cls, num, *args, **kws):
potatos = []
for i in range(num):
potatos.append(cls.__new__(cls, *args, **kws))
return potatos
#创建超市列表,放5个土豆
all_potatos = Potato.make(5)
class Tomatos:
@classmethod
def make(cls, num, *args, **kws):
tomatos = []
for i in range(num):
tomatos.append(cls.__new__(cls, *args, **kws))
return tomatos
#创建超市列表,放10个西红柿
all_tomatos = Tomatos.make(10)
#异步买土豆
async def take_potatos(num):
count = 0
while True:
#判断有无土豆,没有就等待
if len(all_potatos) == 0:
await ask_potatos()
else:
#有就删除一个,即在bucket篮子里加入一个
potato = all_potatos.pop()
yield potato
count += 1
#当土豆数买够数量就停
if count == num:
break
#异步买西红柿
async def take_tomatos(num):
count = 0
while True:
#判断有无西红柿,没有就等待
if len(all_tomatos) == 0:
await ask_tomatos()
else:
#有就删除一个,即在bucket篮子里加入一个
tomato = all_tomatos.pop()
yield tomato
count += 1
#当西红柿数买够数量就停
if count == num:
break
#超市去买土豆存货,放到超市列表
async def ask_potatos():
await asyncio.sleep(random.random())
all_potatos.extend(Potato.make(random.randint(1, 10)))
# 超市去买西红柿存货,放到超市列表
async def ask_tomatos():
await asyncio.sleep(random.random())
all_tomatos.extend(Tomatos.make(random.randint(1, 10)))
#买土豆
async def buy_potatos():
bucket = []
number = 0
async for p in take_potatos(50):
bucket.append(p)
print('买编号为 %d,第%d个土豆'%(id(p),number+1))
number+=1
async def buy_tomatos():
bucket = []
number = 0
async for p in take_tomatos(50):
bucket.append(p)
print('买编号为 %d,第%d个西红柿' % (id(p), number + 1))
number += 1
def main():
import asyncio
loop = asyncio.get_event_loop()
res = loop.run_until_complete(asyncio.wait([buy_potatos(),buy_tomatos()]))
loop.close()
main()
如图:
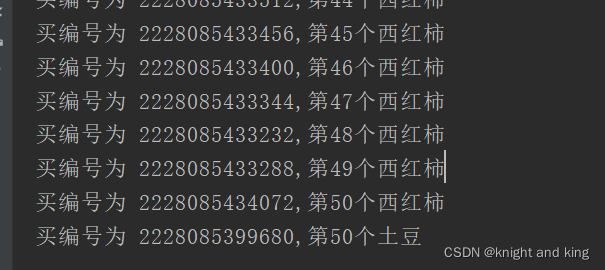
?
|