?不是什么高科技,网上可以搜到很多,不过 MicroPython 比较乱,不同的开发板有些细微的差别,库也是千差万别。以下为实测通过的。
# 闪灯 (on off 电平反的)
import machine
import time
led = machine.Pin(2, machine.Pin.OUT)
while True:
led.off()
time.sleep(0.1)
led.on()
time.sleep(0.1)
led.off()
time.sleep(0.1)
led.on()
time.sleep(1)
# DHT11
import dht
import machine
d = dht.DHT11(machine.Pin(16))
d.measure()
print(d.temperature() ,d.humidity())
# DHT22
import dht
import machine
d = dht.DHT22(machine.Pin(16))
d.measure()
print(d.temperature() ,d.humidity())
# AHT10(I2C) (配合库 ahtx0.py 来源 https://pypi.org/project/micropython-ahtx0/#description )
import utime
from machine import Pin, I2C
import ahtx0
# I2C for the Wemos D1 Mini with ESP8266
i2c = I2C(scl=Pin(4), sda=Pin(5)) # 注意例程居然是反的,可能是针对其他开发板吧
# Create the sensor object using I2C
sensor = ahtx0.AHT10(i2c)
while True:
print("\nTemperature: %0.2f C" % sensor.temperature)
print("Humidity: %0.2f %%" % sensor.relative_humidity)
utime.sleep(5)
# OLED 默认是 8x8 字体,暂时还没办法改!
from machine import I2C,Pin
from ssd1306 import SSD1306_I2C
import time
i2c = I2C(scl = Pin(4),sda = Pin(5),freq = 10000) #软件I2C
oled = SSD1306_I2C(128, 64, i2c) #创建oled对象
oled.rect(0,0,127,63,1)
oled.show()
oled.text("Hello World!",15,25)
oled.show()
time.sleep(1)
oled.fill(0) # clear
oled.show()
### OLED 直插 GPIO16开始的4个脚
from machine import I2C,Pin
from ssd1306 import SSD1306_I2C
import time
p16 = Pin(16, Pin.OUT) # OLED Vcc
p16.value(1)
p5 = Pin(5, Pin.OUT) # OLED Gnd
p5.value(0)
i2c = I2C(scl = Pin(4),sda = Pin(0)) #软件I2C
oled = SSD1306_I2C(128, 64, i2c) #创建oled对象
oled.rect(0,0,127,63,1)
oled.show()
oled.text("Hello World!",15,30)
oled.show()
?能不接线就不接嘛,条件是买 NodeMCU 要买没有焊排针的,自己焊排母。这样就有一些 UNO 的便利了。
?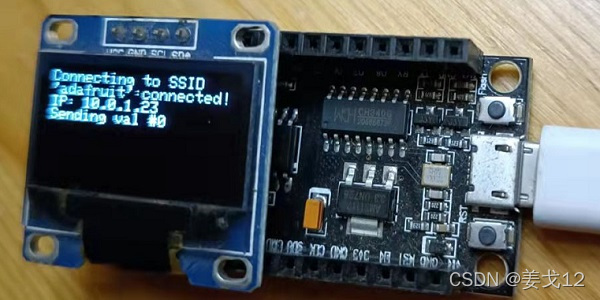
?
# OLED + AHT10 胶水代码
from machine import Pin, I2C
from ssd1306 import SSD1306_I2C
import ahtx0
import utime
import time
i2c = I2C(scl=Pin(4), sda=Pin(5))
print('I2C devices',i2c.scan())
sensor = ahtx0.AHT10(i2c)
oled = SSD1306_I2C(128, 64, i2c)
while True:
tmp = int(sensor.temperature * 100)/100
hum = int(sensor.relative_humidity * 100)/100
print("\nTemperature: %0.2f C" % tmp)
oled.invert(1) # 有这一行全屏反白显示,注释掉取消反白
print("Humidity: %0.2f %%" % hum)
oled.fill(0) # clear
oled.show()
oled.text(str(tmp),15,10) # 浮点转字符串str()
oled.text(str(hum),15,40)
oled.show()
utime.sleep(5)
|