网络编程学习(11)/ FTP项目(5) ——文件上传和上传断点续存功能
服务端 lib 文件夹下的 main.py 状态码的变化
STATUS_CODE = {
200: "User login succeeded !",
201: "Error: wrong username or wrong password !",
202: "Action_type does not exist !",
300: "Check Dir !",
301: "Dir does not exist !",
400: "Ready to send file !",
401: "File does not exist !",
402: "Ready to re_send !",
500: "File upload done !",
501: "File upload failed !",
502: "File exist !"
}
文件上传功能
服务端 lib 文件夹下的 main.py
def _put(self, header_dic):
"""从客户端接收文件"""
filename = header_dic["filename"]
total_size = header_dic["file_size"]
res_szie = 0
with open(self.current_dir + "\\%s.upload" % filename, "wb") as f:
while res_szie < total_size:
data = self.conn.recv(self.MSG_SIZE)
res_szie += len(data)
f.write(data)
if os.path.isfile(self.current_dir + "\\" + filename):
os.rename(self.current_dir + "\\%s.upload" % filename, self.current_dir + "\\" + filename + "." +str(time.time()))
else:
os.rename(self.current_dir + "\\%s.upload" % filename, self.current_dir + "\\" + filename)
self.send_response(500)
客户端 client 文件夹下的 FTPClient.py
def put(self, command):
"""向服务端上传文件"""
if self.parameter_length_judgment(command=command, min_size=2):
filename = command[0]
file_path = command[1]
if os.path.isfile(file_path):
file_size = os.path.getsize(file_path)
self.create_header_send(action_type="put", filename=filename, file_size=file_size)
print(self.current_dir)
self.shelve_obj_upload[filename] = (file_path, file_size, self.current_dir)
progress_bar = self.progress_bar(total_size=file_size)
progress_bar.__next__()
send_size = 0
with open(file_path, "rb") as f:
for line in f:
send_size += len(line)
progress_bar.send(send_size)
self.client.send(line)
response = self.get_response()
if response.get("status_code") == 500:
print(response.get("status_msg"))
del self.shelve_obj_upload[filename]
else:
print("File does not exist !")
文件上传断点续存功能
服务端 lib 文件夹下的 main.py
def _re_put(self, header_dic):
"""重新上传"""
filename = header_dic.get("filename")
total_size = header_dic.get("total_size")
server_path = header_dic.get("server_path")
if os.path.isfile("%s\\%s.upload" % (server_path, filename)):
recv_size = os.path.getsize("%s\\%s.upload" % (server_path, filename))
self.send_response(status_code=502, recv_size=recv_size)
with open("%s\\%s.upload" % (server_path, filename), "ab") as f:
while recv_size < total_size:
data = self.conn.recv(self.MSG_SIZE)
recv_size += len(data)
f.write(data)
if os.path.isfile("%s\\%s" % (server_path, filename)):
os.rename("%s\\%s.upload" % (server_path, filename), "%s\\%s.%s" % (server_path, filename, str(time.time())))
else:
os.rename("%s\\%s.upload" % (server_path, filename), "%s\\%s" % (server_path, filename))
else:
self.send_response(status_code=401)
客户端 client 文件夹下的 FTPClient.py
def interactive(self):
"""交互指令"""
if self.auth():
self.unfinished_download_check()
self.unfinished_upload_check()
........
def unfinished_upload_check(self):
"""上传断点续存"""
if list(self.shelve_obj_upload.keys()):
print("-------Unfinished upload list------")
for index, filename in enumerate(list(self.shelve_obj_upload.keys())):
print("%s %s %s" % (index, filename,
self.shelve_obj_upload[filename][0]))
while True:
choice = input("[select file index to re-put]>>: ").strip()
if not choice: continue
if choice == "back": break
if choice.isdigit():
choice = int(choice)
if choice <= index:
filename = list(self.shelve_obj_upload.keys())[choice]
file_path = self.shelve_obj_upload[filename][0]
total_size = self.shelve_obj_upload[filename][1]
server_path = self.shelve_obj_upload[filename][2]
if os.path.isfile(file_path) and total_size == os.path.getsize(file_path):
self.create_header_send(action_type="re_put", total_size=total_size, server_path=server_path, filename=filename)
response = self.get_response()
if response.get("status_code") == 401:
print(response.get("status_msg"))
del self.shelve_obj_upload[filename]
else:
recv_size = response.get("recv_size")
progress_bar = self.progress_bar(total_size=total_size, res_size=recv_size, last_size=recv_size)
progress_bar.__next__()
with open(file_path, "rb") as f:
f.seek(recv_size)
for line in f:
self.client.send(line)
recv_size += len(line)
progress_bar.send(recv_size)
print("File re_upload done !")
del self.shelve_obj_upload[filename]
else:
print("File does not exist !")
del self.shelve_obj_upload[filename]
运行结果
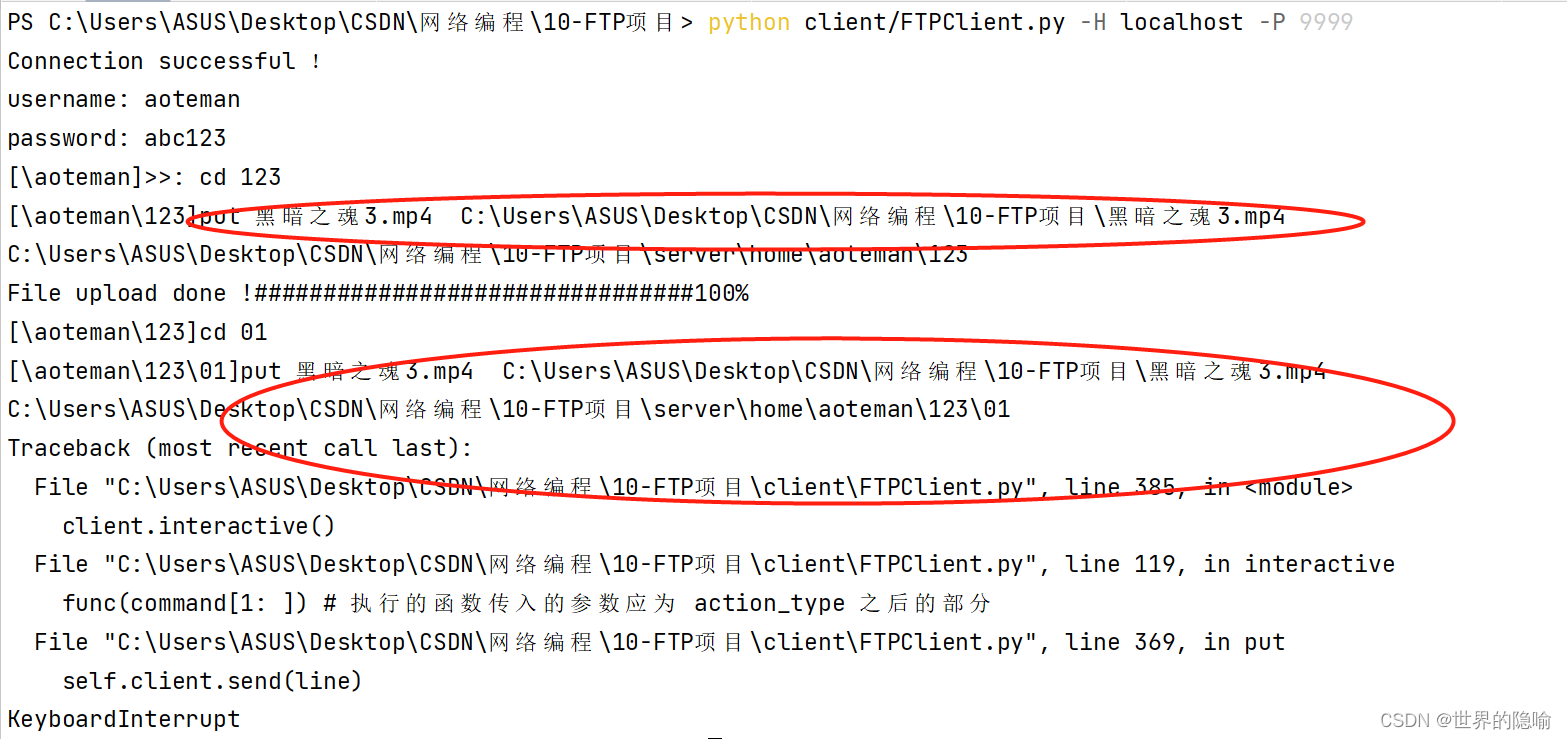 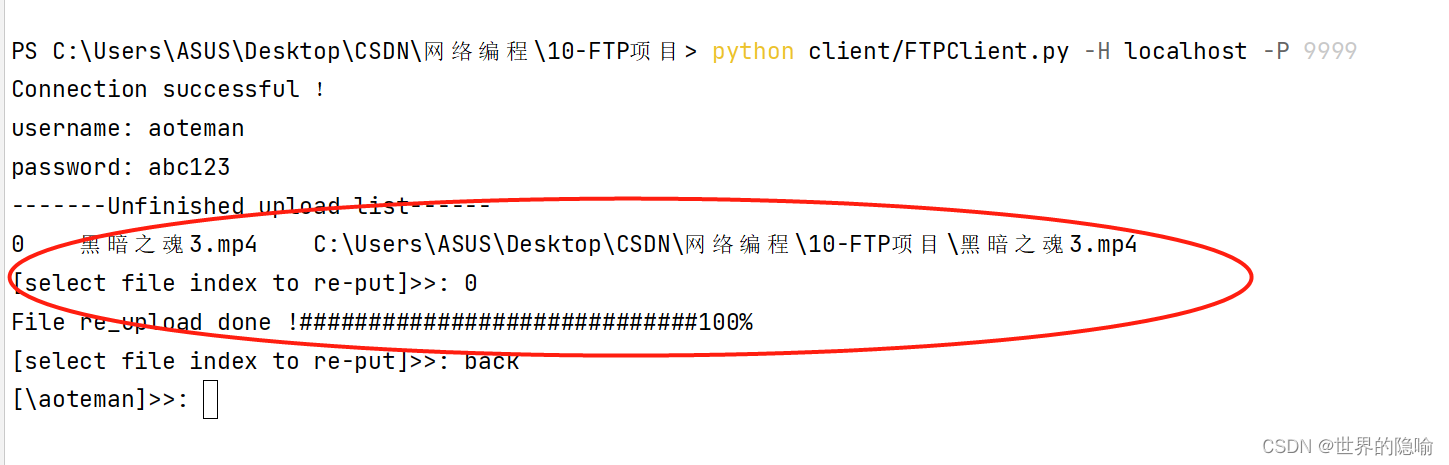
|