Python学习_day01
初学建议使用IDLE: Integrated Development and Learing Evrionment 快乐码字
永远的 hello world
>>>print("hello world")
hello world
>>>print('hello world')
hello world
>>>print('''hello world''')
hello world
输入 import this ,一起欣赏一下这首Python之诗~
>>>import this
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
1.1 一个猜数字的小游戏
"""用python设计一个小游戏"""
temp = input("猜一下心里想的一个数字:")
guess = int(temp)
if guess == 0:
print("yes, 你猜对了!")
else:
print("no, 你猜错了,正确的数字是0哦!")
print("game over!")
 input() 函数用于接收用户的输入及返回。
tips: Java用户记得不要写 " ; " 哦! 正确使用缩进! 正确拼写函数!
1.2 Python内置函数
输入dir(__builtins__) , 查看Python的内置函数,两个下划线! 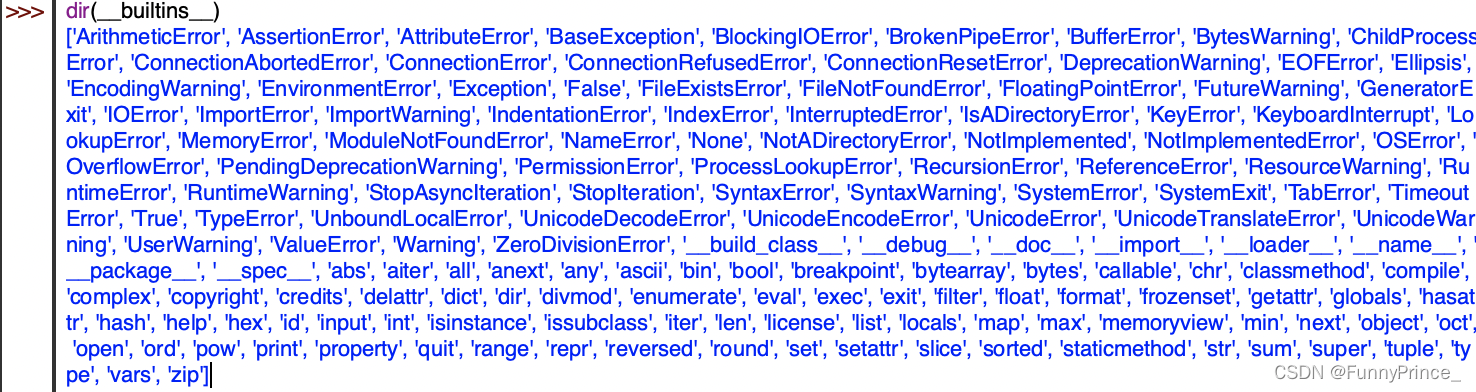
1.3变量 Variable
变量不可直接数字开头! 变量可以使用中文! 变量取最新值! 若要交换两个变量的值,可以不使用临时变量(第三变量),直接 x,y = y,x 即可交换! 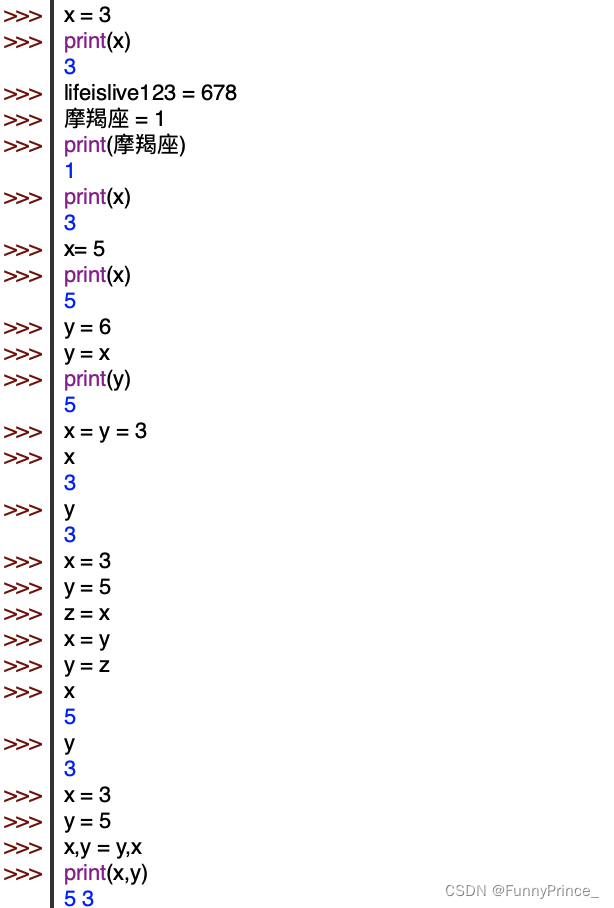
1.4 字符串 String
Single quotes ’ ’ Double quotes " " Triple quoted 三个单引号 ‘’’ ‘’’ 或 三个双引号 “”" “”"
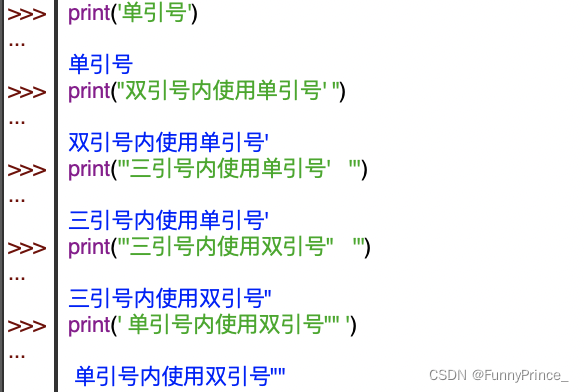
引号必须成双成对!
1.5 转义字符
符号 | Notes |
---|
\\ | 反斜杠(\) | \’ | 单引号(’) | \" | 双引号(") | \a | 响铃(BEL) | \b | 退格符(BS) | \n | 换行符(LF) | \t | 水平制表符(TAB) | \v | 垂直制表符(VT) | \r | 回车符(CR) | \f | 换页符(FF) | \ooo | ooo 为八进制数 | \xhh | hh 为十六进制数 |
 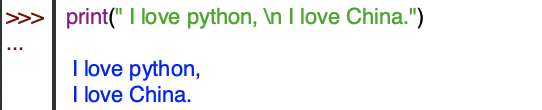
1.6 原始字符串 raw Strings
要输出原始字符串,在反斜杠前再加一个反斜杠,类似此笔记:  若要输出的东西被误以为是转义字符,只需在输出前加 r 即可: 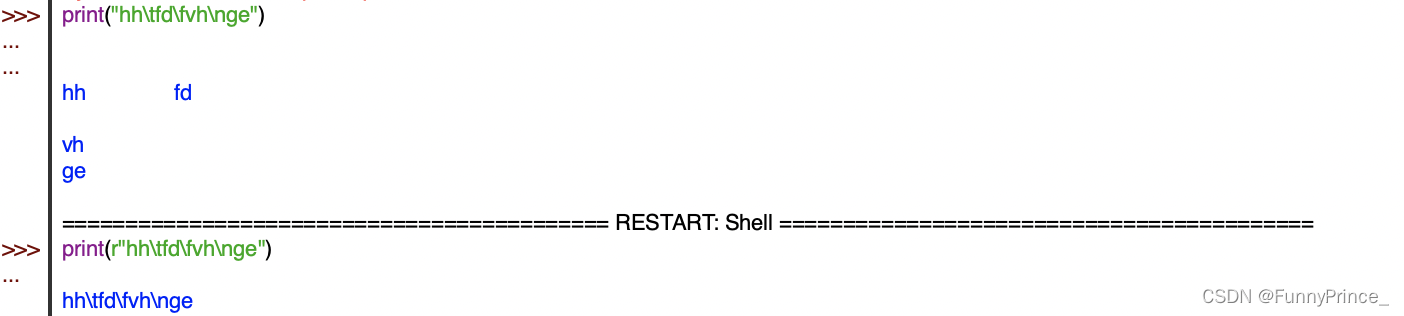
?? 反斜杠不可放在字符串末尾!反斜杠放在字符串的末尾,表示还没结束!  使用三引号,中间可无限换行,替代使用末尾反斜杠。 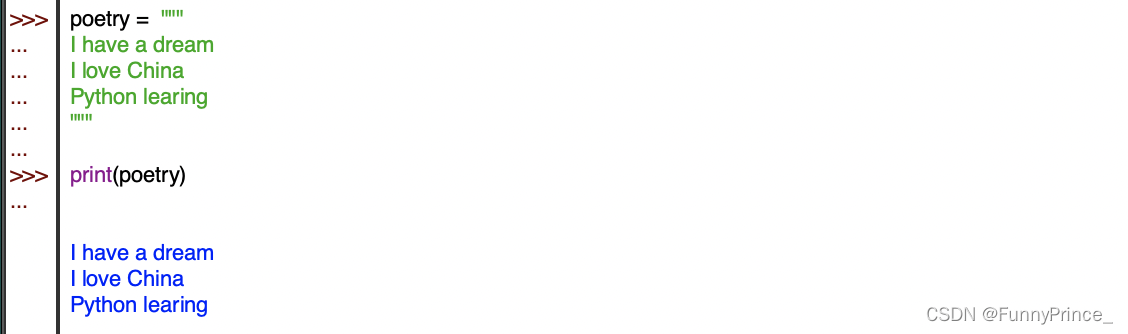
1.7 字符串的加法和乘法 Concatenation and multiplication of Strings
与其他语言一样,字符串的加法是拼接 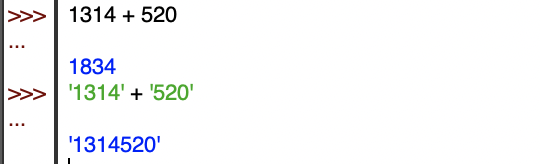 乘法:可多倍输出
print("Love u 3000 tims everyday\n" * 3000)
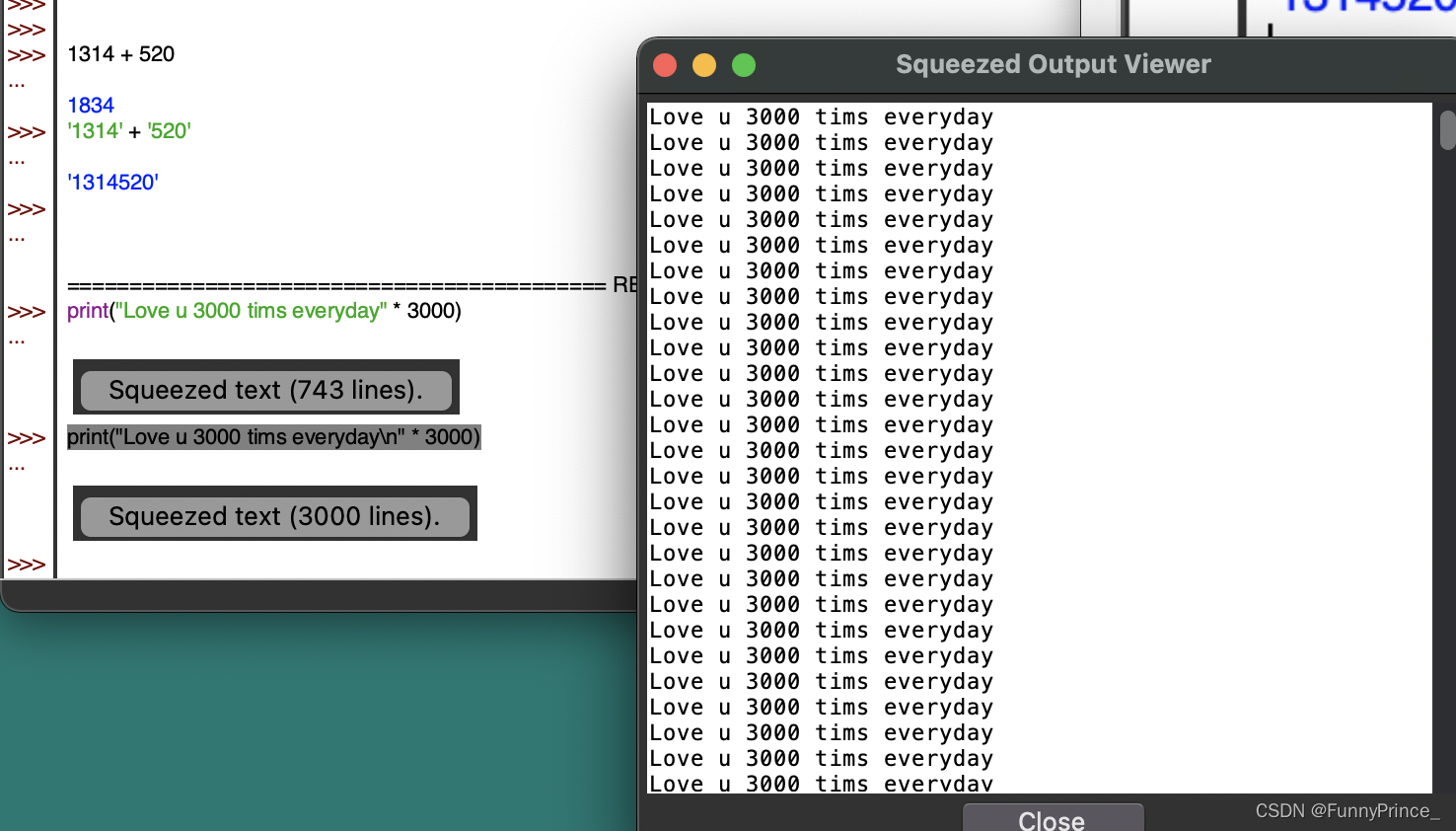
运算符: > \ < \ == \ >= \ <= \ != \ is \ is not
1.8 循环结构
While 条件: 如果条件为真 (true) 执行这里的语句 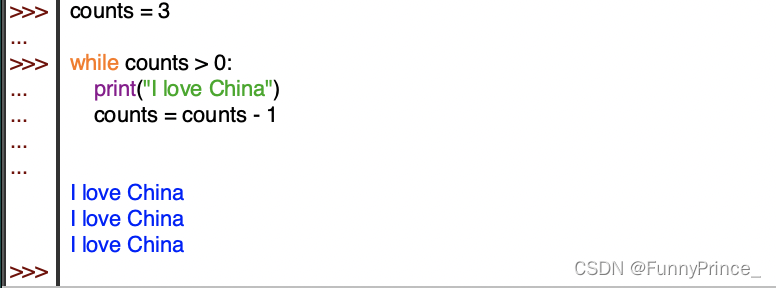
1.9 改进小游戏
"""用python设计一个小游戏"""
"""用python设计一个小游戏"""
import random
counts =3
answer = random.randint(0,10)
while counts > 0:
temp = input("猜一下心里想的一个数字:")
guess = int(temp)
if guess == answer:
print("yes, 你猜对了!")
break
else:
if guess < answer:
print("猜小啦")
else:
print("猜大啦")
counts = counts -1
print("game over!")

 1.使用break语句组织部输入正确后的无效循环。
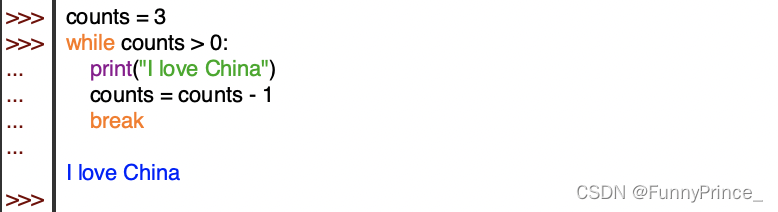
2.random函数 使用 import 导入函数 random.randint(m,n) 随机打印 m 到 n 的一个数。 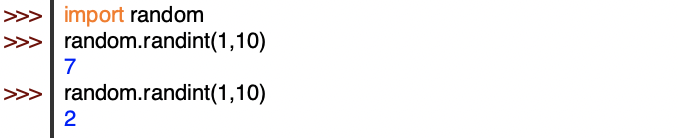
3.random 随机数重现 使用 random.setstate() 来存储随机数,使用 random.getstate() 来获取随机数。
random.getstate() 只表示取出随机数:  若先将第一次读取出来的随机数使用 random.setstate()保存起来再读取,便可复现上次的随机数。 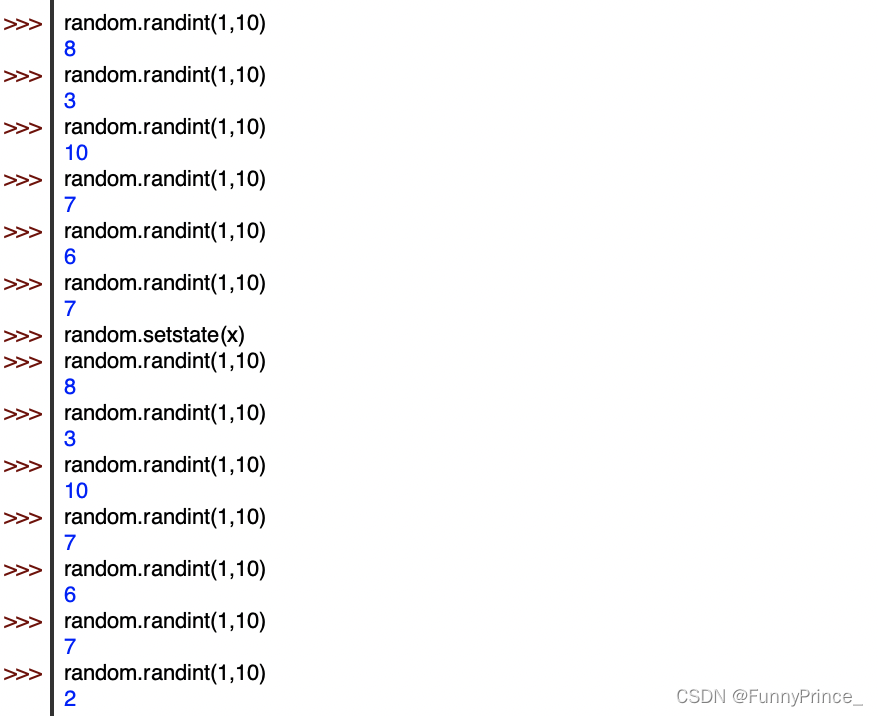 抽奖黑幕系统原理大概如此!
Mac快捷键 Control + P:上一行 学会使用help-- Python Docs进行查找文档
|