1. 三角形
import turtle as t
t.speed(1)
t.fillcolor('red')
t.begin_fill()
t.fd(180)
t.left(90)
t.forward(180)
t.goto(0,0)
t.end_fill()
t.fillcolor('yellow')
t.begin_fill()
t.forward(180)
t.right(90)
t.forward(180)
t.goto(0,0)
t.end_fill()
t.hideturtle()
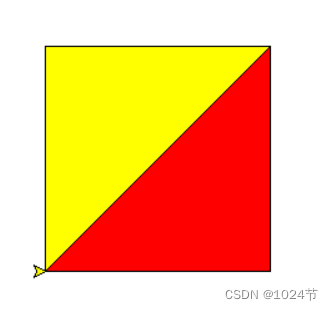
2. 扇形
import turtle as t
t.speed(0)
list = [0,90,180,270]
colors = ['red','yellow','blue','green']
for i in range(4):
t.seth(list[i])
t.fillcolor(colors[i])
t.begin_fill()
t.forward(100)
t.right(90)
t.circle(-100,45)
t.right(90)
t.forward(100)
t.end_fill()
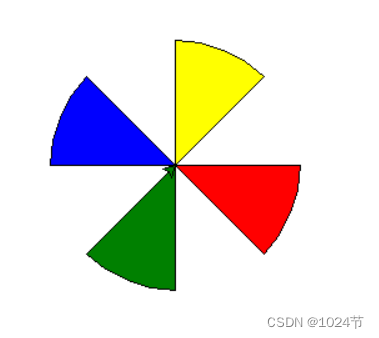
3. 正方形内切填充圆
import turtle as t
t.hideturtle()
t.penup()
t.goto(0,-50)
t.pendown()
t.color('red','yellow')
t.begin_fill()
t.circle(50)
t.end_fill()
t.back(50)
for i in range(4):
t.forward(100)
t.left(90)
t.done()
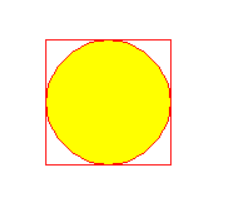
4. 绘制六边形
绘制图形要求:
- 背景为白色,正六边形和三角形的填充颜色分别为红色、绿色;
- 图形中间为边长为150的正六边形,周围为六个等边三角形;
- 正六边形的上下两条边要求与X轴方向平行;
- 绘图过程中隐藏画笔,并能清楚地看到图形绘制过程。
import turtle as t
t.fillcolor('red')
t.hideturtle()
t.begin_fill()
for i in range(6):
t.forward(150)
t.fillcolor('green')
t.begin_fill()
for j in range(3):
t.right(120)
t.forward(150)
t.left(60)
t.end_fill()
t.end_fill()
t.fillcolor('red')
t.begin_fill()
for i in range(6):
t.forward(150)
t.left(60)
t.end_fill()
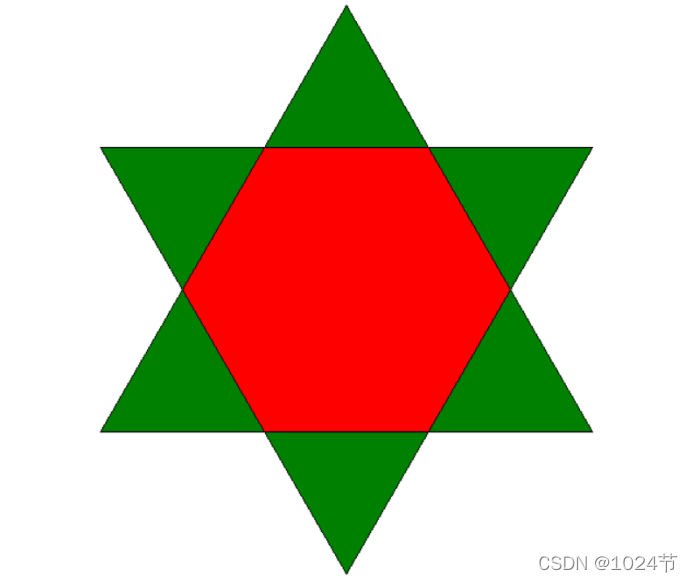
5. 奥运五环
import turtle as t
t.speed(0)
t.pensize(6)
t.color('black')
t.circle(80)
t.penup()
t.goto(-180,0)
t.pendown()
t.color('blue')
t.circle(80)
t.penup()
t.goto(180,0)
t.pendown()
t.color('red')
t.circle(80)
t.penup()
t.goto(92,-95)
t.pendown()
t.color('green')
t.circle(80)
t.penup()
t.goto(-92,-95)
t.pendown()
t.color('yellow')
t.circle(80)
t.color('black')
t.penup()
t.goto(-200,200)
t.pendown()
t.write('奥运五环',font=('楷体',50))
6. 五角星
import random
import turtle as t
t.speed(0)
t.hideturtle()
def a(x,y):
z = random.randint(50,100)
t.penup()
t.goto(x,y)
t.pendown()
t.color('red')
t.begin_fill()
for i in range(5):
t.forward(z)
t.right(144)
t.end_fill()
count = random.randint(1,10)
for i in range(count):
x = random.randint(-300,300)
y = random.randint(-300,300)
a(x,y)
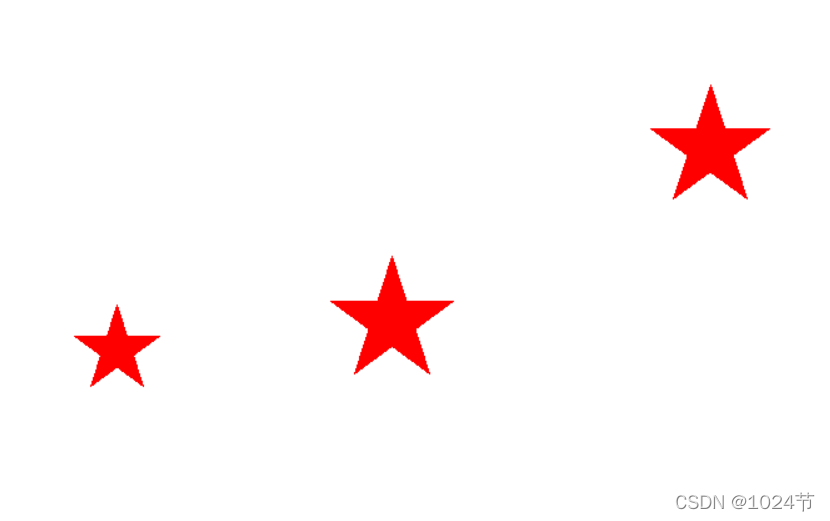 2.
import turtle as t
t.speed(0)
t.hideturtle()
t.pensize(6)
t.color('yellow','red')
t.begin_fill()
for i in range(5):
t.forward(150)
t.right(144)
t.end_fill()
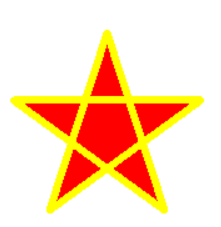
7. 斐波那契螺旋线
前两项都是1,从第3项开始,每一项都等于前两项之和。
a = [1,1]
for i in range(2,9):
a.append(a[-2] + a[-1])
for i in a:
t.circle(i*5,90)
def fun(n):
if n <= 2:
return 1
else:
return fun(n - 1)+ fun(n - 2)
for i in range(1,9):
t.circle(fun(i)* 6,90)
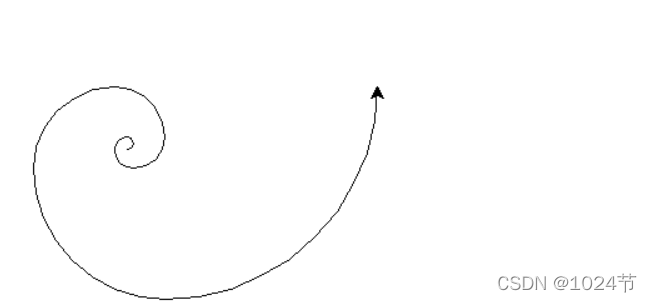
def fun(n):
if n <= 2:
return 1
else:
return fun(n - 1)+ fun(n - 2)
a = []
for i in range(1,9):
a.append(fun(i))
for x in a:
t.color('red')
for i in range(4):
t.forward(x * 6)
t.right(90)
t.color('black')
t.circle(-x * 6,90)
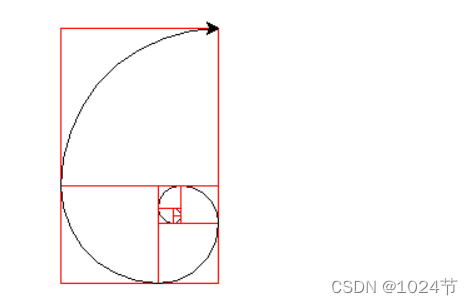
海归图一
|