QWebEngineView是PyQt5(5.4版本之后)扩展库中的类,需要单独安装
pip install PyQtWebEngine
使用示例
? 需求:解析百度坐标拾取系统中用户选取的坐标点;
? 分析:用户在地图选点后,网页数据会更新,获取更新后的html并解析出坐标值即可;
? 效果图示
? 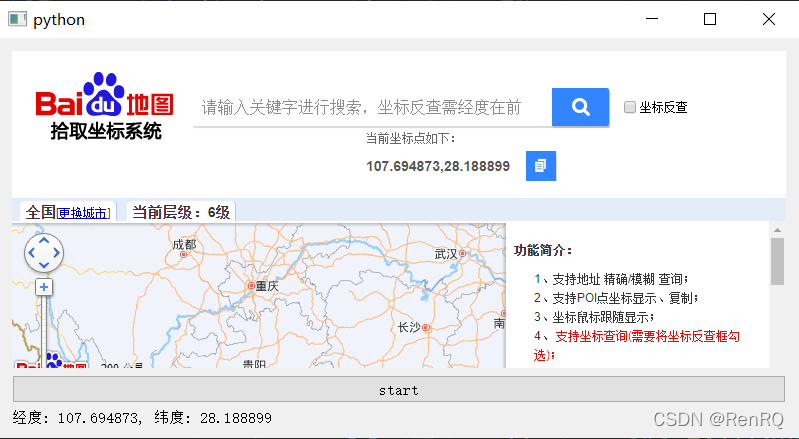
? 关键代码:
? 1.获取html
browser.page().toHtml(parse_html) # 这里的callback异步执行,默认传递html给parse_html
? 2.解析出坐标
def parse_html(html_doc):
longitude, latitude = None, None
soup = bs(html_doc, 'html.parser')
for i in soup.find_all('input'):
if i.get('data-clipboard-text'):
print(i.get('data-clipboard-text').split(','))
longitude, latitude = i.get('data-clipboard-text').split(',')
return longitude, latitude
其他
# 需要bs4解析html
from bs4 import BeautifulSoup as bs
demo参考
import sys
from PyQt5.QtWebEngineWidgets import QWebEngineView
from PyQt5.QtCore import QUrl
from PyQt5.QtWidgets import QVBoxLayout, QPushButton, QApplication, QWidget, QLabel
from bs4 import BeautifulSoup as bs
class coordinate(QWidget):
def __init__(self, url):
super(coordinate, self).__init__()
self.url = url
self.setup_ui()
def setup_ui(self):
"""init ui : include webEngineView and a button"""
btn = QPushButton('start', self)
self.label1 = QLabel()
self.browser = QWebEngineView(self)
self.browser.resize(600, 400)
self.browser.load(QUrl(self.url))
self.browser.show()
layout = QVBoxLayout(self)
layout.addWidget(self.browser)
layout.addWidget(btn)
layout.addWidget(self.label1)
btn.clicked.connect(self.get_coordinate)
def get_coordinate(self):
self.browser.page().toHtml(self.parse_html)
print('上面是异步执行打印验证')
def parse_html(self, html_doc):
longitude, latitude = None, None
try:
soup = bs(html_doc, 'html.parser')
for i in soup.find_all('input'):
if i.get('data-clipboard-text'):
longitude, latitude = i.get('data-clipboard-text').split(',')
except Exception as e:
print(e)
self.label1.setText(f'经度: {longitude}, 纬度: {latitude}')
print(longitude, latitude)
if __name__ == "__main__":
url = 'http://api.map.baidu.com/lbsapi/getpoint/index.html'
app = QApplication(sys.argv)
form = coordinate(url)
form.setGeometry(300,300,800,400)
form.show()
sys.exit(app.exec_())
|