一、工具截图
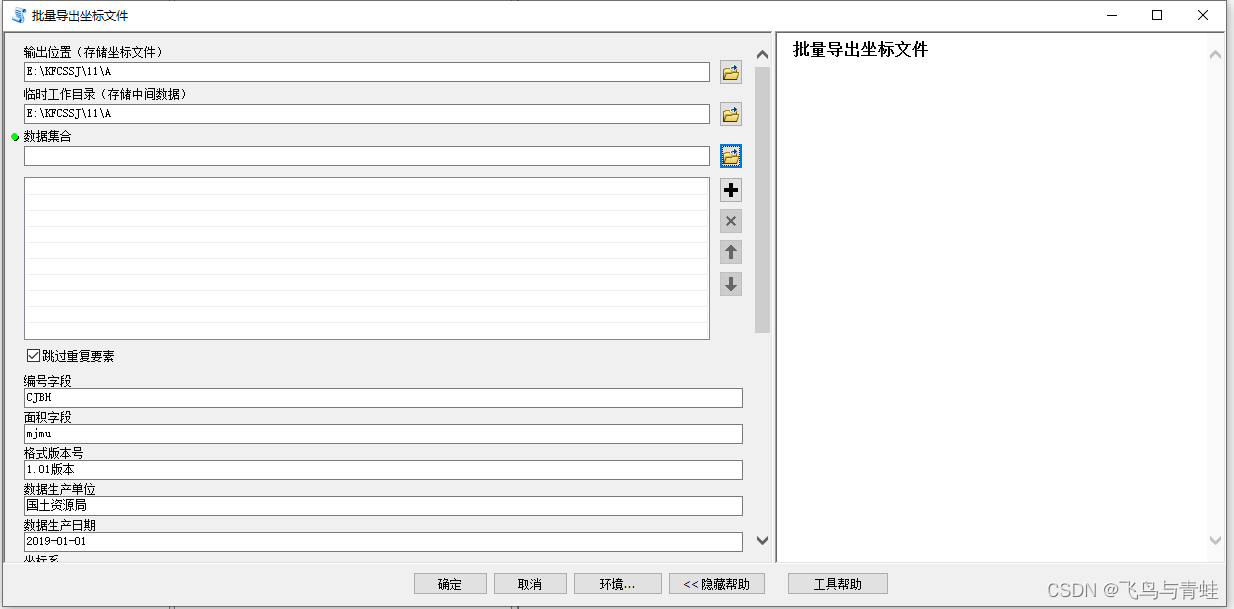 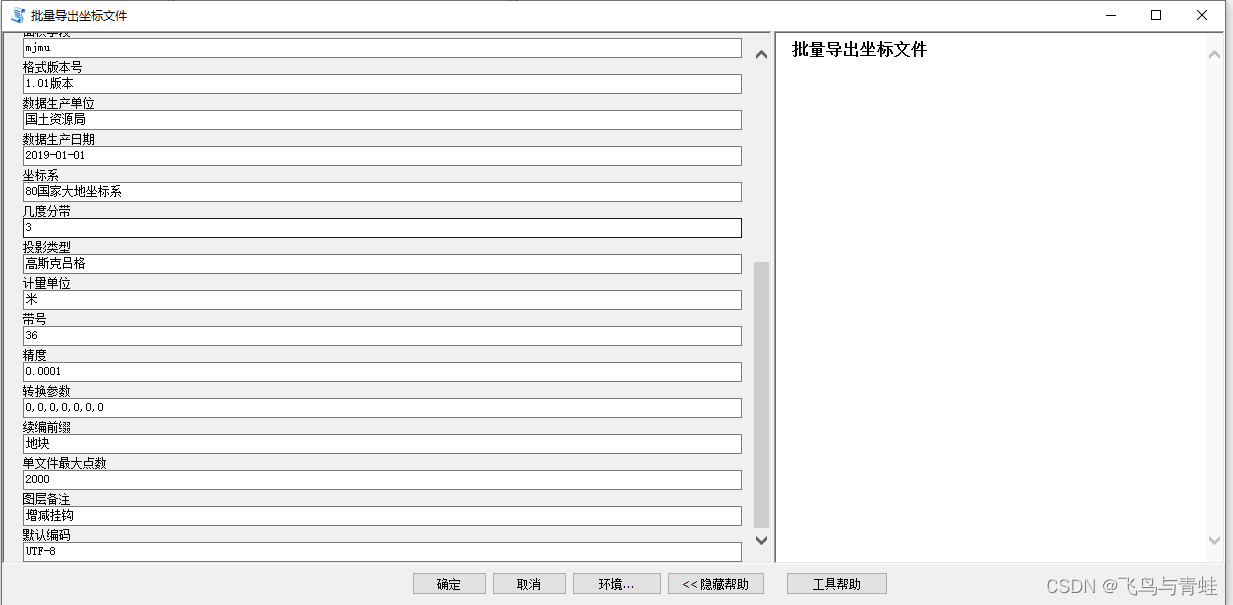 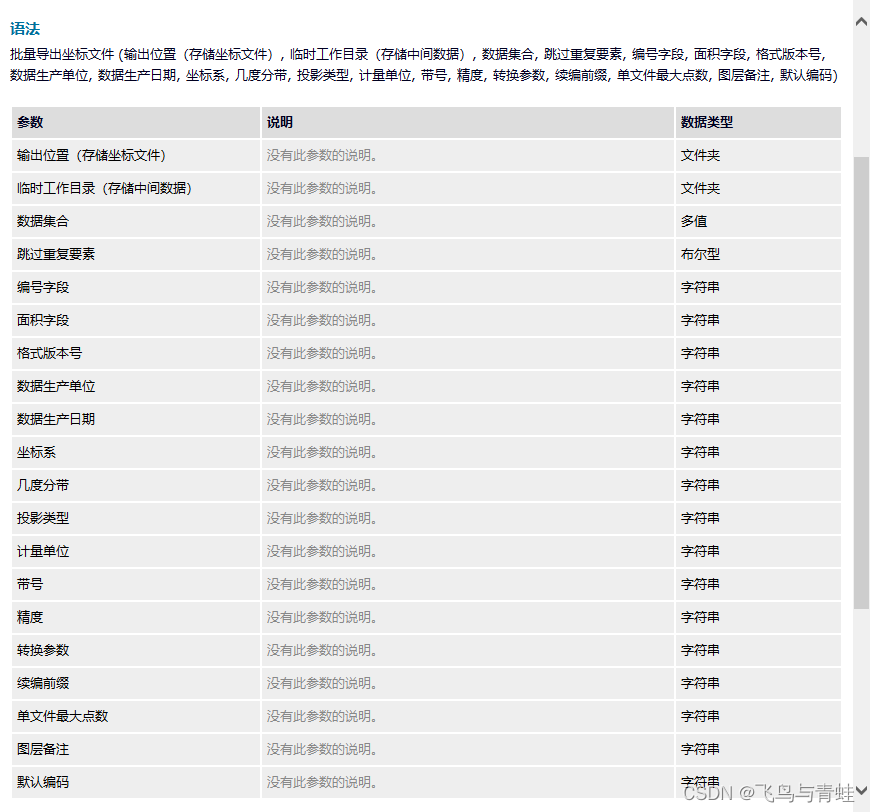
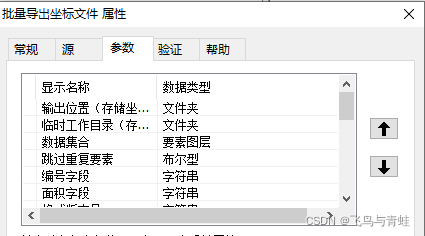 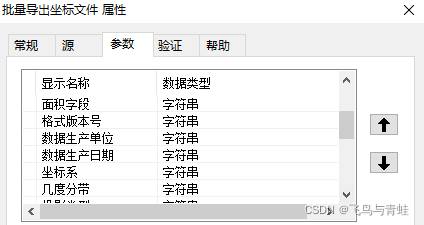 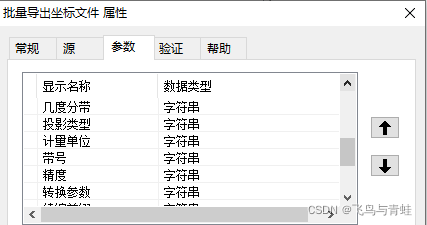 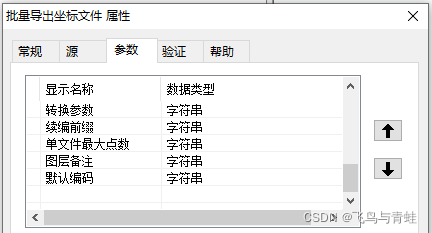
二、导出文件
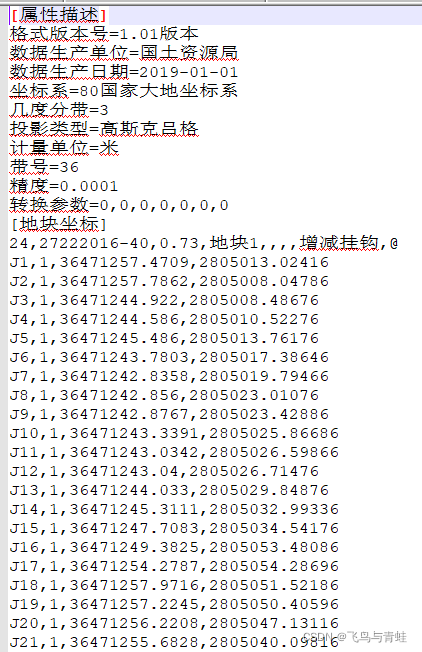
三、脚本
import json
import sys
import arcpy
import os
def set_encoding():
"""
set system encoding.
"""
a, b, c = sys.stdin, sys.stdout, sys.stderr
reload(sys)
sys.setdefaultencoding("utf-8")
sys.stdin, sys.stdout, sys.stderr = a, b, c
def ring_point_count(feature):
"""
count point number.
:type feature: dict
:param feature:
:return:
"""
count = 0
for ring in feature["geometry"]["rings"]:
count += len(ring)
return count
def reset_num(coordinate):
"""
reset last one serial number.
:type coordinate: list
:param coordinate:
"""
coordinate[-1][0] = "J1"
def load_json(path):
"""
load json file from disk.
:type path: str
:param path:
:return:
"""
with open(path.decode("utf-8"), "r") as f:
return json.load(f)
def feature_maps(features, configure):
"""
get a new feature json of list.
:param features:
:type features: dict
:param configure:
:type configure: dict
:return: feature json of list
"""
maps = []
feature_map = []
point_max = int(configure["attr-desc"]["point-max-num"])
count = 0
index = 1
for feature in features:
rp_count = ring_point_count(feature)
if (count + rp_count) > point_max:
maps.append(feature_map)
feature_map = []
count = 0
index = 1
index_feature = {
"index": str(index),
"rp_count": str(rp_count),
"attributes": feature["attributes"],
"rings": feature["geometry"]["rings"]
}
feature_map.append(index_feature)
count += rp_count
index += 1
maps.append(feature_map)
return maps
def analyse_overview(feature, configure):
"""
get a overview info.
:type configure: dict
:type feature: dict
:param feature:
:param configure:
:return:
"""
attributes = feature["attributes"]
return "{coordinate_count},{area},{plot_num},{plot_name},{attribute},{sheet_designation},{land_use},{remark},@".format(
coordinate_count=feature["rp_count"],
area=attributes[configure["layer-meta"]["serial-num-field"]],
plot_num=attributes[configure["layer-meta"]["area-field"]],
plot_name=configure["attr-desc"]["prefix"] + feature["index"],
attribute="",
sheet_designation="",
land_use="",
remark=configure["attr-desc"]["layer-remark"]
)
def analyse_coordinates(feature):
"""
get coordinates from feature.
:type feature: dict
:param feature:
:return:
"""
rings = feature["rings"]
coordinates = [
[["J" + str(index + 1), str(ring_index + 1), ",".join(list(map(str, coordinate[:])))] for index, coordinate in
enumerate(ring)] for ring_index, ring in enumerate(rings)]
return coordinates
def create_dir(path):
"""
:type path: str
:param path:
"""
if not os.path.exists(path.decode("utf-8")):
os.makedirs(path.decode("utf-8"))
else:
arcpy.AddError(path.decode("utf-8") + "目录已存在")
def write_header(f, configure):
attr_desc = configure["attr-desc"]
f.write("[属性描述]\n")
f.write("格式版本号=" + attr_desc["version"] + "\n")
f.write("数据生产单位=" + attr_desc["producer"] + "\n")
f.write("数据生产日期=" + attr_desc["date"] + "\n")
f.write("坐标系=" + attr_desc["coordinate"] + "\n")
f.write("几度分带=" + attr_desc["degree"] + "\n")
f.write("投影类型=" + attr_desc["projection"] + "\n")
f.write("计量单位=" + attr_desc["unit"] + "\n")
f.write("带号=" + attr_desc["degree-num"] + "\n")
f.write("精度=" + attr_desc["precision"] + "\n")
f.write("转换参数=" + attr_desc["conversion-parameter"] + "\n")
f.write("[地块坐标]\n")
def convert(export_info, configure):
"""
create a new file of coordinates.
:type export_info: dict
:param export_info:
"""
geojson = load_json(export_info["jsonfile"])
features = geojson["features"]
create_dir(export_info["export_dir"])
for index, feature_map in enumerate(feature_maps(features, configure)):
out_filename = "{0}\\{1}-{2}.txt".format(export_info["export_dir"], export_info["filename"], str(index))
with open(out_filename.decode("utf-8"), "w") as f:
write_header(f, configure)
for feature in feature_map:
overview = analyse_overview(feature, configure)
f.write(overview)
f.write("\n")
for coordinate in analyse_coordinates(feature):
reset_num(coordinate)
for item in coordinate:
f.write(",".join(item) + "\n")
def analyse_path(workspace, temp_workspace, layer):
"""
get export information.
:type layer: str
:type temp_workspace: str
:type workspace: str
:param workspace:
:param temp_workspace:
:param layer:
:return:
"""
filename = os.path.basename(layer).split(".")[0]
export_info = {
"layer": layer,
"filename": filename,
"jsonfile": "{root}\\{filename}.json".format(root=temp_workspace, filename=filename),
"export_dir": "{root}\\{layer_dir}".format(root=workspace, layer_dir=filename)
}
return export_info
def check_path(workspace, temp_workspace, layers, skip_repeat):
"""
check whether the path exists.
:param workspace:
:param temp_workspace:
:param layers:
:return:
"""
export_infos = []
for layer in layers:
export_info = analyse_path(workspace, temp_workspace, layer)
if skip_repeat == "true":
if os.path.exists(export_info["jsonfile"].decode("utf-8")):
continue
elif os.path.exists(export_info["export_dir"].decode("utf-8")):
continue
else:
export_infos.append(export_info)
else:
if os.path.exists(export_info["jsonfile"].decode("utf-8")):
arcpy.AddError(export_info["jsonfile"] + "目录已存在")
elif os.path.exists(export_info["export_dir"].decode("utf-8")):
arcpy.AddError(export_info["export_dir"] + "目录已存在")
else:
export_infos.append(export_info)
return export_infos
def export_json(export_infos):
"""
export all json file
:param export_infos:
"""
for export_info in export_infos:
arcpy.FeaturesToJSON_conversion(export_info["layer"], export_info["jsonfile"])
def export_all_file(export_infos, configure):
"""
export file
:param export_infos:
:param configure:
"""
for export_info in export_infos:
convert(export_info, configure)
def export_file(workspace, temp_workspace, layers, configure, skip_repeat):
"""
export file.
:type configure: dict
:type layers: list
:type temp_workspace: str
:type workspace: str
:param workspace:
:param temp_workspace:
:param layers:
:param configure:
"""
arcpy.AddMessage("检查路径是否重复......")
export_infos = check_path(workspace, temp_workspace, layers, skip_repeat)
arcpy.AddMessage("导出JSON格式......")
export_json(export_infos)
arcpy.AddMessage("导出标准格式......")
export_all_file(export_infos, configure)
arcpy.AddMessage("导出完毕")
if __name__ == "__main__":
set_encoding()
workspace = arcpy.GetParameterAsText(0)
temp_workspace = arcpy.GetParameterAsText(1)
layers = arcpy.GetParameterAsText(2).split(";")
skip_repeat = arcpy.GetParameterAsText(3)
configure = {
"layer-meta": {
"serial-num-field": arcpy.GetParameterAsText(4),
"area-field": arcpy.GetParameterAsText(5),
},
"attr-desc": {
"version": arcpy.GetParameterAsText(6),
"producer": arcpy.GetParameterAsText(7),
"date": arcpy.GetParameterAsText(8),
"coordinate": arcpy.GetParameterAsText(9),
"degree": arcpy.GetParameterAsText(10),
"projection": arcpy.GetParameterAsText(11),
"unit": arcpy.GetParameterAsText(12),
"degree-num": arcpy.GetParameterAsText(13),
"precision": arcpy.GetParameterAsText(14),
"conversion-parameter": arcpy.GetParameterAsText(15),
"prefix": arcpy.GetParameterAsText(16),
"point-max-num": arcpy.GetParameterAsText(17),
"layer-remark": arcpy.GetParameterAsText(18)
},
"encode": arcpy.GetParameterAsText(19)
}
export_file(workspace, temp_workspace, layers, configure, skip_repeat)
|