1. 代码(假数据)
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.QtCore import *
from threading import Thread
import time, sys, os
import qdarkstyle
os.chdir(os.path.dirname(os.path.abspath(__file__)))
class MySignals(QObject):
updateTempSingle = pyqtSignal(QProgressBar, float)
global_ms = MySignals()
class Temp_Gui(QWidget):
def __init__(self):
super(Temp_Gui, self).__init__()
QFontDatabase.addApplicationFont("./font/simkai.ttf")
font = QFont()
font.setFamily("Arial")
font.setPointSize(18)
QGroupBox("mywin").setFont(font)
self.setWindowTitle("温度监测")
self.setWindowIcon(QIcon('./images/boy.png'))
self.resize(250, 100)
StyleSheet = '''
QWidget{
text-align: center;
border: 2px solid #2196F3;
border-radius: 5px;
background-color: #E0E0E0;
},
'''
self.setStyleSheet(qdarkstyle.load_stylesheet_pyqt5())
formLayout = QFormLayout()
self.Label_temp_name1 = QLabel('热电偶1:')
self.Label_temp_name2 = QLabel('热电偶2:')
self.Label_temp_name3 = QLabel('热电偶3:')
self.LineEdit_temp_value1 = QLineEdit("0 °C")
self.LineEdit_temp_value2 = QLineEdit("0 °C")
self.LineEdit_temp_value3 = QLineEdit("0 °C")
self.LineEdit_temp_value1.setReadOnly(True)
self.LineEdit_temp_value2.setReadOnly(True)
self.LineEdit_temp_value3.setReadOnly(True)
self.LineEdit_temp_value1.setAlignment(Qt.AlignCenter)
self.LineEdit_temp_value2.setAlignment(Qt.AlignCenter)
self.LineEdit_temp_value3.setAlignment(Qt.AlignCenter)
formLayout.addRow(self.Label_temp_name1, self.LineEdit_temp_value1)
formLayout.addRow(self.Label_temp_name2, self.LineEdit_temp_value2)
formLayout.addRow(self.Label_temp_name3, self.LineEdit_temp_value3)
self.setLayout(formLayout)
global_ms.updateTempSingle.connect(self.updataTemperature)
def updataTemperature(self, object, value):
object.setText(str(value) + " °C")
def readTempTask():
def threadFunc():
while True:
for i in range(0, 101, 1):
time.sleep(0.2)
global_ms.updateTempSingle.emit(temp_gui.LineEdit_temp_value1, i + 0.1 * i)
global_ms.updateTempSingle.emit(temp_gui.LineEdit_temp_value2, i + 0.2 * i)
global_ms.updateTempSingle.emit(temp_gui.LineEdit_temp_value3, i + 0.3 * i)
thread = Thread(target=threadFunc, daemon=True)
thread.start()
if __name__ == '__main__':
app = QApplication(sys.argv)
temp_gui = Temp_Gui()
temp_gui.show()
readTempTask()
sys.exit(app.exec_())
2. 代码(真数据)
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.QtCore import *
from threading import Thread
import time, sys, os
import qdarkstyle
import busio
import digitalio
import board
from adafruit_bus_device.spi_device import SPIDevice
os.chdir(os.path.dirname(os.path.abspath(__file__)))
class MySignals(QObject):
updateTempSingle = pyqtSignal(QProgressBar, float)
global_ms = MySignals()
class Temp_Gui(QWidget):
def __init__(self):
super(Temp_Gui, self).__init__()
QFontDatabase.addApplicationFont("./font/simkai.ttf")
font = QFont()
font.setFamily("Arial")
font.setPointSize(18)
QGroupBox("mywin").setFont(font)
self.setWindowTitle("温度监测")
self.setWindowIcon(QIcon('./images/boy.png'))
self.resize(250, 100)
StyleSheet = '''
QWidget{
text-align: center;
border: 2px solid #2196F3;
border-radius: 5px;
background-color: #E0E0E0;
},
'''
self.setStyleSheet(qdarkstyle.load_stylesheet_pyqt5())
formLayout = QFormLayout()
self.Label_temp_name1 = QLabel('热电偶1:')
self.Label_temp_name2 = QLabel('热电偶2:')
self.Label_temp_name3 = QLabel('热电偶3:')
self.LineEdit_temp_value1 = QLineEdit("0 °C")
self.LineEdit_temp_value2 = QLineEdit("0 °C")
self.LineEdit_temp_value3 = QLineEdit("0 °C")
self.LineEdit_temp_value1.setReadOnly(True)
self.LineEdit_temp_value2.setReadOnly(True)
self.LineEdit_temp_value3.setReadOnly(True)
self.LineEdit_temp_value1.setAlignment(Qt.AlignCenter)
self.LineEdit_temp_value2.setAlignment(Qt.AlignCenter)
self.LineEdit_temp_value3.setAlignment(Qt.AlignCenter)
formLayout.addRow(self.Label_temp_name1, self.LineEdit_temp_value1)
formLayout.addRow(self.Label_temp_name2, self.LineEdit_temp_value2)
formLayout.addRow(self.Label_temp_name3, self.LineEdit_temp_value3)
self.setLayout(formLayout)
global_ms.updateTempSingle.connect(self.updataTemperature)
def updataTemperature(self, object, value):
object.setText(str(value) + " °C")
def readTempTask():
def threadFunc():
with busio.SPI(board.SCLK, board.MOSI, board.MISO) as spi_bus:
cs1_pin = digitalio.DigitalInOut(board.SPI1_CS)
cs2_pin = digitalio.DigitalInOut(board.PC7)
cs3_pin = digitalio.DigitalInOut(board.PC10)
MAX6675_1 = SPIDevice(spi_bus, cs1_pin, baudrate=1_000_000)
MAX6675_2 = SPIDevice(spi_bus, cs2_pin, baudrate=1_000_000)
MAX6675_3 = SPIDevice(spi_bus, cs3_pin, baudrate=1_000_000)
while True:
bytes_read1 = bytearray(2)
bytes_read2 = bytearray(2)
bytes_read3 = bytearray(2)
with MAX6675_1 as spi:
spi.readinto(bytes_read1)
high_bit = bytes_read1[0] * 32
low_bit = (bytes_read1[1] & 0b11111000) >> 3
Temp1 = (high_bit + low_bit) * 0.25
with MAX6675_2 as spi:
spi.readinto(bytes_read2)
high_bit = bytes_read2[0] * 32
low_bit = (bytes_read2[1] & 0b11111000) >> 3
Temp2 = (high_bit + low_bit) * 0.25
with MAX6675_3 as spi:
spi.readinto(bytes_read3)
high_bit = bytes_read3[0] * 32
low_bit = (bytes_read3[1] & 0b11111000) >> 3
Temp3 = (high_bit + low_bit) * 0.25
global_ms.updateTempSingle.emit(temp_gui.LineEdit_temp_value1, Temp1)
global_ms.updateTempSingle.emit(temp_gui.LineEdit_temp_value2, Temp2)
global_ms.updateTempSingle.emit(temp_gui.LineEdit_temp_value3, Temp3)
time.sleep(0.22)
thread = Thread(target=threadFunc, daemon=True)
thread.start()
if __name__ == '__main__':
app = QApplication(sys.argv)
temp_gui = Temp_Gui()
temp_gui.show()
readTempTask()
sys.exit(app.exec_())
3. 运行图片
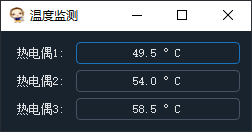
|