Django admin后台管理页面的常用设置
选择列表选项choices
# filename: models.py
from django.db import models
# 例1 int类型:
STATUS = (
(0, 0),
(1, 1)
)
class Team1(models.Model):
status = models.IntegerField('状态', choices=STATUS, default=1) #default状态默认为1
# 例2 字符类型:
class Team2(models.Model):
team_name = models.CharField(max_length=40)
TEAM_LEVELS = (
('U09', 'Under 09s'),
('U10', 'Under 10s'),
('U11', 'Under 11s'),
... #list other team levels
)
team_level = models.CharField(max_length=3, choices=TEAM_LEVELS, default='U11')
输入框
name = models.CharField('用户名', max_length=200, unique=True)
remark = models.TextField('备注', null=True, blank=True)
num = models.IntegerField('数字', null=True, blank=True, default=0)
时间
accountcreated = models.DateField('时间', null=True, blank=True) # 自己选时间
updated = models.DateField('更新时间', auto_now=True) # 自动更新时间
created = models.DateField('创建时间', auto_now_add=True) # 自动更新时间
主键
id = models.AutoField(primary_key=True)
显示在页面
## filename: views.py
from django.shortcuts import render
from .models import Team
def index(request):
list_teams = Team.objects.filter(team_level__exact="U09")
context = {'youngest_teams': list_teams}
return render(request, '/best/index.html', context)
## filename: best/templates/best/index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Home page</title>
</head>
<body>
{% if youngest_teams %}
<ul>
{% for team in youngest_teams %}
<li>{{ team.team_name }}</li>
{% endfor %}
</ul>
{% else %}
<p>No teams are available.</p>
{% endif %}
</body>
</html>
?以format_html动态方式返回结果的函数
# 例1:
def Job_detail(self):
href = f'https://www.zhipin.com/job_detail/?query={self.job_name}&city={self.job_add}/'
return format_html(f'<a href={href}>job</a>')
Job_detail.short_description = 'Job_detail'
#例2:
class ImageColumn(tables.Column):
def render(self, value):
return format_html('<a href="/media/{0}" download>{0}</a>', value)
-
只写模型类、不写__str__: 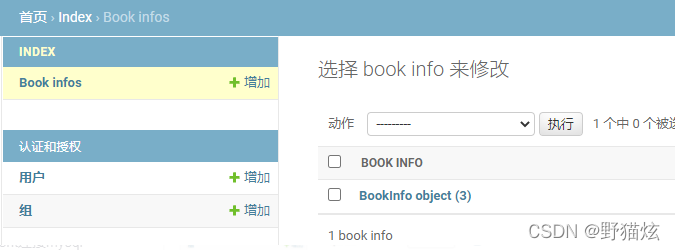 -
写模型类、写__str__: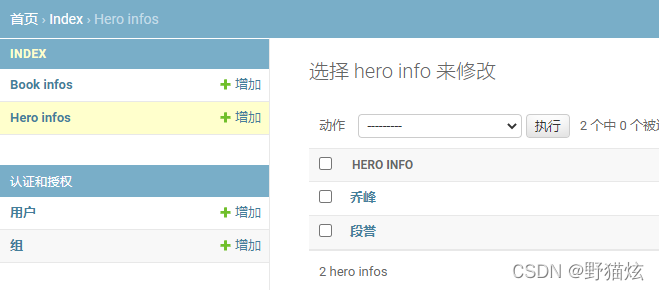
def __str__(self):
return self.name
?字段名默认为app_name, 而表明默认为app名+_类名,如果不想要默认的就要指定,如下:
class ItemForSale(models.Model):
app_id = models.ForeignKey(App)
app_name = models.CharField(verbose_name='应用名', max_length=32, db_column='对应的字段名')
class Meta:
db_table = 'ItemForSale' #对应的表名
verbose_name = '用户名' #指定在admin管理界面中显示中文,单数形式的显示
verbose_name_plural = '用户名' #复数形式的显示
整体代码如下:
from django.db import models
from django.utils.html import format_html
STATUS = (
(0, 0),
(1, 1)
)
class Accounts(models.Model):
id = models.AutoField(primary_key=True)
name = models.CharField('用户名', max_length=200, unique=True)
job_add = models.CharField('地址', max_length=200, unique=True)
status = models.IntegerField('状态', choices=STATUS, default=1)
def __str__(self):
return self.name
def Job_detail(self):
href = f'https://www.zhipin.com/job_detail/?query={self.name}&city={self.job_add}/'
return format_html(f'<a href={href}>Job_detail</a>')
Job_detail.short_description = 'Job_detail'
后台顶部标题的修改:app/admin.py中设置
admin.site.site_title ='site_title'
admin.site.site_header = 'site_header'
admin.site.index_title='index_title'
?更改后
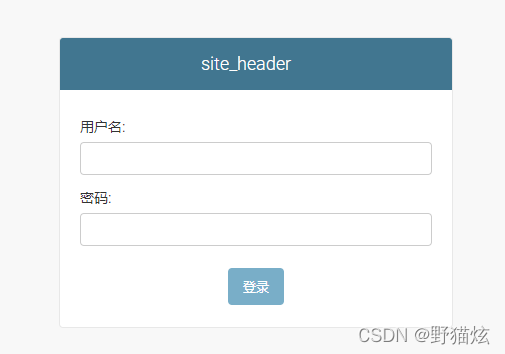
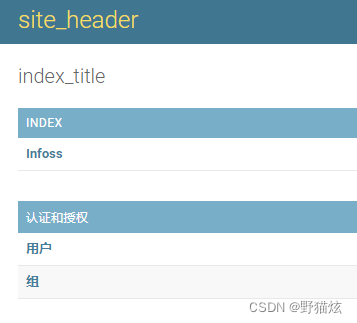
?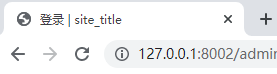
|