1、正确python3递归写法
from typing import List
class Solution:
def fourSum(self, nums: List[int], target: int) -> List[List[int]]:
def nSumtarget(nums,n,start,target):
sz = len(nums)
res=[]
if n<2 or sz<n:
return res
if n==2:
lo = start
hi = sz-1
while (lo<hi):
sum = nums[lo]+nums[hi]
left = nums[lo]
right = nums[hi]
if sum<target:
while lo<hi and nums[lo]==left:
lo+=1
elif sum>target:
while lo<hi and nums[hi]==right:
hi-=1
else:
res.append([left,right])
while lo<hi and nums[lo]==left:
lo+=1
while lo<hi and nums[hi]==right:
hi-=1
else:
i=start
while i<sz:
print(i)
sub = nSumtarget(nums,n-1,i+1,target-nums[i])
for arr in sub:
arr.append(nums[i])
res.append(arr)
while (i<sz-1 and nums[i] == nums[i+1]):
i+=1
i=i+1
return res
nums.sort()
print(nums)
return nSumtarget(nums,4,0,target)
if __name__=="__main__":
nums = [1,0,-1,0,-2,2]
target = 0
res = Solution().fourSum(nums,0)
print(res)
①运行结果(注意while循环的输出) 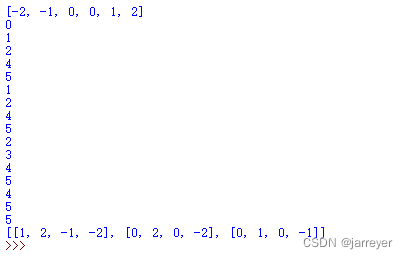
2、错误写法
from typing import List
class Solution:
def fourSum(self, nums: List[int], target: int) -> List[List[int]]:
def nSumtarget(nums,n,start,target):
sz = len(nums)
res=[]
if n<2 or sz<n:
return res
if n==2:
lo = start
hi = sz-1
while (lo<hi):
sum = nums[lo]+nums[hi]
left = nums[lo]
right = nums[hi]
if sum<target:
while lo<hi and nums[lo]==left:
lo+=1
elif sum>target:
while lo<hi and nums[hi]==right:
hi-=1
else:
res.append([left,right])
while lo<hi and nums[lo]==left:
lo+=1
while lo<hi and nums[hi]==right:
hi-=1
else:
for i in range(start,sz):
print(i)
sub = nSumtarget(nums,n-1,i+1,target-nums[i])
for arr in sub:
arr.append(nums[i])
res.append(arr)
while (i<sz-1 and nums[i] == nums[i+1]):
i+=1
return res
nums.sort()
print(nums)
return nSumtarget(nums,4,0,target)
if __name__=="__main__":
nums = [1,0,-1,0,-2,2]
target = 0
res = Solution().fourSum(nums,0)
print(res)
②运行结果(for循环和while循环的不同之处) 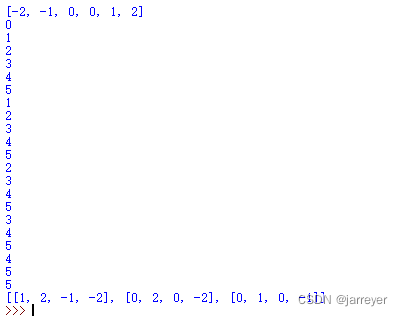
3、c++写法
c++中for循环和python3中存在差别,c++中下面的for循环变量i值可再循环内部中的while循环中更新,是全局的;而python的for循环变量i不会循环内部更新
using namespace std;
class Solution {
public:
vector<vector<int>> fourSum(vector<int>& nums,int target) {
sort(nums.begin(), nums.end());
return nSumTarget(nums, 4, 0, target);
}
vector<vector<int>> nSumTarget(
vector<int>& nums, int n, int start, int target) {
int sz = nums.size();
vector<vector<int>> res;
if (n < 2 || sz < n) return res;
if (n == 2) {
int lo = start, hi = sz - 1;
while (lo < hi) {
int sum = nums[lo] + nums[hi];
int left = nums[lo], right = nums[hi];
if (sum < target) {
while (lo < hi && nums[lo] == left) lo++;
} else if (sum > target) {
while (lo < hi && nums[hi] == right) hi--;
} else {
res.push_back({left, right});
while (lo < hi && nums[lo] == left) lo++;
while (lo < hi && nums[hi] == right) hi--;
}
}
} else {
for (int i = start; i < sz; i++) {
cout<<i<<endl;
vector<vector<int>>
sub = nSumTarget(nums, n - 1, i + 1, target - nums[i]);
for (vector<int>& arr : sub) {
arr.push_back(nums[i]);
res.push_back(arr);
}
while (i < sz - 1 && nums[i] == nums[i + 1]) {
i++;
}
}
}
return res;
}
};
代码来源:https://mp.weixin.qq.com/s/fSyJVvggxHq28a0SdmZm6Q
|