Python:如何查看一个对象有哪些属性、方法以及查询源码中只有pass的方法的参数
1.问题背景
python 是C语言 实现的,尽管有很多标准库是由python 代码实现,但是涉及到底层支撑架构的功能或需要较高计算性能的实现还是会使用C语言 。一些IDE 为了对这些进行友好代码提示,会弄和底层一样的访问接口,而其实现直接过程写 pass 略过。 我们如果要查看某些功能的源码时,往往会发现这个方法上面只写了一个pass ,并带有标识# real signature unknown; restored from __doc__ ,连可输入的参数有哪些都不知道: 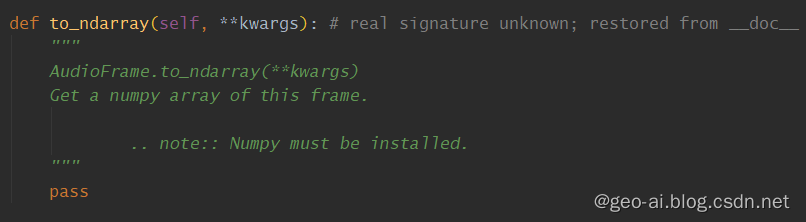 上面的**kwargs 表示关键字参数,如果是比较详细的库还好,会给你列出能输入哪些关键字参数,但大多数情况时你压根不知道可以输入的关键字参数有哪些~~
2.解决思路
2.1.查看对象有哪些属性或方法
这里我们以典型的计算库:numpy 进行举例,因为其中含有大量C 写的代码,而我们无法通过python查看其源代码: 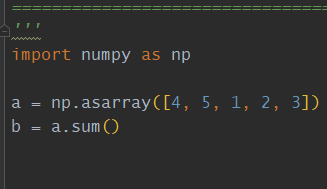 在这里,初始化了一个ndarray :a ,然后对它排序,那么如何知道a 有哪些方法能够调用呢?
2.1.1dir()函数
我们可以使用python 自带的内置函数dir 直接返回模块内定义的所有名称,结果以一个字符串列表的形式返回。:
dir(a)
可以看到得到了a能够调用的所有方法:
[‘T’, ‘abs’, ‘add’, ‘and’, ‘array’, ‘array_finalize’, ‘array_function’, ‘array_interface’, ‘array_prepare’, ‘array_priority’, ‘array_struct’, ‘array_ufunc’, ‘array_wrap’, ‘bool’, ‘class’, ‘complex’, ‘contains’, ‘copy’, ‘deepcopy’, ‘delattr’, ‘delitem’, ‘dir’, ‘divmod’, ‘doc’, ‘eq’, ‘float’, ‘floordiv’, ‘format’, ‘ge’, ‘getattribute’, ‘getitem’, ‘gt’, ‘hash’, ‘iadd’, ‘iand’, ‘ifloordiv’, ‘ilshift’, ‘imatmul’, ‘imod’, ‘imul’, ‘index’, ‘init’, ‘init_subclass’, ‘int’, ‘invert’, ‘ior’, ‘ipow’, ‘irshift’, ‘isub’, ‘iter’, ‘itruediv’, ‘ixor’, ‘le’, ‘len’, ‘lshift’, ‘lt’, ‘matmul’, ‘mod’, ‘mul’, ‘ne’, ‘neg’, ‘new’, ‘or’, ‘pos’, ‘pow’, ‘radd’, ‘rand’, ‘rdivmod’, ‘reduce’, ‘reduce_ex’, ‘repr’, ‘rfloordiv’, ‘rlshift’, ‘rmatmul’, ‘rmod’, ‘rmul’, ‘ror’, ‘rpow’, ‘rrshift’, ‘rshift’, ‘rsub’, ‘rtruediv’, ‘rxor’, ‘setattr’, ‘setitem’, ‘setstate’, ‘sizeof’, ‘str’, ‘sub’, ‘subclasshook’, ‘truediv’, ‘xor’, ‘all’, ‘any’, ‘argmax’, ‘argmin’, ‘argpartition’, ‘argsort’, ‘astype’, ‘base’, ‘byteswap’, ‘choose’, ‘clip’, ‘compress’, ‘conj’, ‘conjugate’, ‘copy’, ‘ctypes’, ‘cumprod’, ‘cumsum’, ‘data’, ‘diagonal’, ‘dot’, ‘dtype’, ‘dump’, ‘dumps’, ‘fill’, ‘flags’, ‘flat’, ‘flatten’, ‘getfield’, ‘imag’, ‘item’, ‘itemset’, ‘itemsize’, ‘max’, ‘mean’, ‘min’, ‘nbytes’, ‘ndim’, ‘newbyteorder’, ‘nonzero’, ‘partition’, ‘prod’, ‘ptp’, ‘put’, ‘ravel’, ‘real’, ‘repeat’, ‘reshape’, ‘resize’, ‘round’, ‘searchsorted’, ‘setfield’, ‘setflags’, ‘shape’, ‘size’, ‘sort’, ‘squeeze’, ‘std’, ‘strides’, ‘sum’, ‘swapaxes’, ‘take’, ‘tobytes’, ‘tofile’, ‘tolist’, ‘tostring’, ‘trace’, ‘transpose’, ‘var’, ‘view’]
2.1.2.help命令
如果我们需要更详细的解释,可以对a使用help 命令,该命令会返回对象的完整帮助文档(由于文档实在太长,只截取了一部分):
help(a)
Help on ndarray object: class ndarray(builtins.object) | ndarray(shape, dtype=float, buffer=None, offset=0, | strides=None, order=None) | | An array object represents a multidimensional, homogeneous array | of fixed-size items. An associated data-type object describes the | format of each element in the array (its byte-order, how many bytes it | occupies in memory, whether it is an integer, a floating point number, | or something else, etc.) | | Arrays should be constructed using array , zeros or empty (refer | to the See Also section below). The parameters given here refer to | a low-level method (ndarray(...) ) for instantiating an array. | | For more information, refer to the numpy module and examine the | methods and attributes of an array. |
Parameters |
---|
(for the new method; see Notes below) | | shape : tuple of ints | Shape of created array. | dtype : data-type, optional | Any object that can be interpreted as a numpy data type. | buffer : object exposing buffer interface, optional | Used to fill the array with data. | offset : int, optional |
2.2.查看方法的参数有哪些?
2.2.1.inspect模块
这个模块可以查看可以查看函数的代码,参数,以及路径:
- 查看函数的路径 inspect.getabsfile(…)
- 查看全部代码 inspect.getsource(模块.函数)或者(模块.类.函数)
- 查看函数参数 inspect.getfullargspec(…) 查看类的参数,则括号里为(模块.类._init _)
'''
===========================================
@author: jayce
@file: inspect_args.py
@time: 2022/4/20 18:42
===========================================
'''
import numpy as np
import inspect
def inspect_args(num1, num2):
'''
:param num1:
:param num2:
:return:
'''
return num1 + num2
a = np.asarray([4, 5, 1, 2, 3])
print('查看函数路径:\n{}'.format(inspect.getabsfile(inspect_args)))
print('查看函数完整代码:\n{}'.format(inspect.getsource(inspect_args)))
print('查看函数有哪些参数:\n{}'.format(inspect.getfullargspec(inspect_args)))
可以看到inspect_args函数的相关信息被完整展示了出来:
查看函数路径:
e:\code\python\demo代码\inspect_args.py
查看函数完整代码:
def inspect_args(num1, num2):
'''
:param num1:
:param num2:
:return:
'''
return num1 + num2
查看函数有哪些参数:
FullArgSpec(args=['num1', 'num2'], varargs=None, varkw=None, defaults=None, kwonlyargs=[], kwonlydefaults=None, annotations={})
这里面需要重点关注 的是getfullargspec ,他返回了函数的具体参数,如下:
args :是位置参数名称的列表varargs :是*参数的名称,None 表示不接受任意位置参数varkw :是**参数的名称,None 表示不接受任意的关键字参数。defaults :是与n个位置参数相对应的默认参数值的n元组,None 表示没有默认值kwonlyargs :是关键字参数名称的列表。kwonlydefaults :是一个映射到kwonlyargs参数默认值字典,None 表示没有annotations :是将参数名称映射到注释的字典。该特殊键返回用于报告函数返回值注释(如果有的话)
但需要注意的是,getfullargspec 只能查询由python 编写的代码,如果查询其他语言写的代码,会报错:TypeError: unsupported callable
Traceback (most recent call last):
File "C:\Users\Jayce\Anaconda3\envs\tf1.7\lib\inspect.py", line 1116, in getfullargspec
sigcls=Signature)
File "C:\Users\Jayce\Anaconda3\envs\tf1.7\lib\inspect.py", line 2183, in _signature_from_callable
raise TypeError('{!r} is not a callable object'.format(obj))
TypeError: array([4, 5, 1, 2, 3]) is not a callable object
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "C:\Users\Jayce\Anaconda3\envs\tf1.7\lib\site-packages\IPython\core\interactiveshell.py", line 3343, in run_code
exec(code_obj, self.user_global_ns, self.user_ns)
File "<ipython-input-3-6fd2d9db0386>", line 1, in <module>
inspect.getfullargspec(a)
File "C:\Users\Jayce\Anaconda3\envs\tf1.7\lib\inspect.py", line 1122, in getfullargspec
raise TypeError('unsupported callable') from ex
TypeError: unsupported callable
2.2.2.help命令
help命令当然也可以查询函数的参数,如我们查询numpy.sum 的参数有哪些:
>>>help(np.sum)
Help on function sum in module numpy:
sum(a, axis=None, dtype=None, out=None, keepdims=<no value>, initial=<no value>, where=<no value>)
Sum of array elements over a given axis.
Parameters
----------
a : array_like
Elements to sum.
axis : None or int or tuple of ints, optional
Axis or axes along which a sum is performed. The default,
axis=None, will sum all of the elements of the input array. If
axis is negative it counts from the last to the first axis.
.. versionadded:: 1.7.0
If axis is a tuple of ints, a sum is performed on all of the axes
specified in the tuple instead of a single axis or all the axes as
before.
dtype : dtype, optional
The type of the returned array and of the accumulator in which the
elements are summed. The dtype of `a` is used by default unless `a`
has an integer dtype of less precision than the default platform
integer. In that case, if `a` is signed then the platform integer
is used while if `a` is unsigned then an unsigned integer of the
same precision as the platform integer is used.
out : ndarray, optional
Alternative output array in which to place the result. It must have
the same shape as the expected output, but the type of the output
values will be cast if necessary.
keepdims : bool, optional
If this is set to True, the axes which are reduced are left
in the result as dimensions with size one. With this option,
the result will broadcast correctly against the input array.
If the default value is passed, then `keepdims` will not be
passed through to the `sum` method of sub-classes of
`ndarray`, however any non-default value will be. If the
sub-class' method does not implement `keepdims` any
exceptions will be raised.
initial : scalar, optional
Starting value for the sum. See `~numpy.ufunc.reduce` for details.
.. versionadded:: 1.15.0
where : array_like of bool, optional
Elements to include in the sum. See `~numpy.ufunc.reduce` for details.
.. versionadded:: 1.17.0
Returns
-------
sum_along_axis : ndarray
An array with the same shape as `a`, with the specified
axis removed. If `a` is a 0-d array, or if `axis` is None, a scalar
is returned. If an output array is specified, a reference to
`out` is returned.
See Also
--------
ndarray.sum : Equivalent method.
add.reduce : Equivalent functionality of `add`.
cumsum : Cumulative sum of array elements.
trapz : Integration of array values using the composite trapezoidal rule.
mean, average
Notes
-----
Arithmetic is modular when using integer types, and no error is
raised on overflow.
The sum of an empty array is the neutral element 0:
>>> np.sum([])
0.0
For floating point numbers the numerical precision of sum (and
``np.add.reduce``) is in general limited by directly adding each number
individually to the result causing rounding errors in every step.
However, often numpy will use a numerically better approach (partial
pairwise summation) leading to improved precision in many use-cases.
This improved precision is always provided when no ``axis`` is given.
When ``axis`` is given, it will depend on which axis is summed.
Technically, to provide the best speed possible, the improved precision
is only used when the summation is along the fast axis in memory.
Note that the exact precision may vary depending on other parameters.
In contrast to NumPy, Python's ``math.fsum`` function uses a slower but
more precise approach to summation.
Especially when summing a large number of lower precision floating point
numbers, such as ``float32``, numerical errors can become significant.
In such cases it can be advisable to use `dtype="float64"` to use a higher
precision for the output.
Examples
--------
>>> np.sum([0.5, 1.5])
2.0
>>> np.sum([0.5, 0.7, 0.2, 1.5], dtype=np.int32)
1
>>> np.sum([[0, 1], [0, 5]])
6
>>> np.sum([[0, 1], [0, 5]], axis=0)
array([0, 6])
>>> np.sum([[0, 1], [0, 5]], axis=1)
array([1, 5])
>>> np.sum([[0, 1], [np.nan, 5]], where=[False, True], axis=1)
array([1., 5.])
If the accumulator is too small, overflow occurs:
>>> np.ones(128, dtype=np.int8).sum(dtype=np.int8)
-128
You can also start the sum with a value other than zero:
>>> np.sum([10], initial=5)
15
不同于inspect 只能查询由python 编写的代码,help 也可以查询其他代码编译后的函数参数,如C 编译后的代码也能成功查询。
|