NumPy是一个Python包,提供快速,灵活和富有表现力的数据结构,旨在使处理“关系”或“标记”数据既简单又直观。它旨在成为在Python中进行实际的现实世界数据分析的基本高级构建块。
NumPy Basic
1、获取numpy版本并显示numpy构建配置
编写一个NumPy程序来获取numpy版本并显示numpy构建配置。
import numpy as np
print(np.__version__)
print(np.show_config())
'''
1.20.3
blas_mkl_info:
libraries = ['mkl_rt']
library_dirs = ['D:/Anaconda\\Library\\lib']
define_macros = [('SCIPY_MKL_H', None), ('HAVE_CBLAS', None)]
include_dirs = ['D:/Anaconda\\Library\\include']
blas_opt_info:
libraries = ['mkl_rt']
library_dirs = ['D:/Anaconda\\Library\\lib']
define_macros = [('SCIPY_MKL_H', None), ('HAVE_CBLAS', None)]
include_dirs = ['D:/Anaconda\\Library\\include']
lapack_mkl_info:
libraries = ['mkl_rt']
library_dirs = ['D:/Anaconda\\Library\\lib']
define_macros = [('SCIPY_MKL_H', None), ('HAVE_CBLAS', None)]
include_dirs = ['D:/Anaconda\\Library\\include']
lapack_opt_info:
libraries = ['mkl_rt']
library_dirs = ['D:/Anaconda\\Library\\lib']
define_macros = [('SCIPY_MKL_H', None), ('HAVE_CBLAS', None)]
include_dirs = ['D:/Anaconda\\Library\\include']
None
'''
2、获取有关add函数的帮助
编写一个NumPy程序来获取有关add函数的帮助。
import numpy as np
print(np.info(np.add))
3、测试给定数组的任何元素是否都不为零
编写一个NumPy程序来测试给定数组的任何元素是否都不为零。
只要有一个元素为0,则结果就为False
import numpy as np
x = np.array([1, 2, 3, 4])
print("Original array:")
print(x)
print("Test if none of the elements of the said array is zero:")
print(np.all(x))
x = np.array([0, 1, 2, 3])
print("Original array:")
print(x)
print("Test if none of the elements of the said array is zero:")
print(np.all(x))
这里涉及python当中的all函数:
any()实现了或(OR)运算,而all()实现了与(AND)运算。
【1】对于any(iterables),如果可迭代对象iterables中任意存在每一个元素为True则返回True。特例:若可迭代对象为空,比如空列表[],则返回False。
【2】对于all(iterables),如果可迭代对象iterables中所有元素都为True则返回True。特例:若可迭代对象为空,比如空列表[],则返回True。
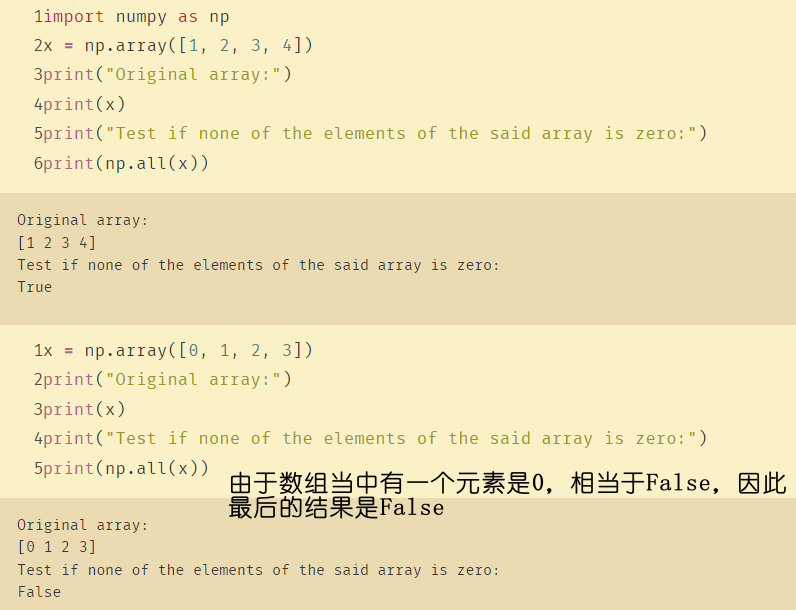
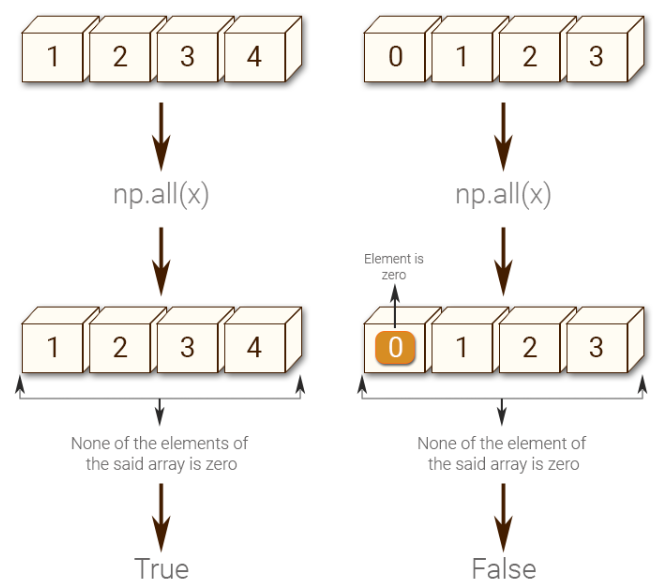
4、测试给定数组的任何元素是否为非零
只要有一个元素非零,则得到的结果为True.
import numpy as np
x = np.array([0, 0, 0, 0])
print("Original array:")
print(x)
print("Test whether any of the elements of a given array is non-zero:")
print(np.any(x))
x = np.array([0, 0, 2, 0])
print("Original array:")
print(x)
print("Test whether any of the elements of a given array is non-zero:")
print(np.any(x))
这里涉及python当中的any函数:
any()实现了或(OR)运算,而all()实现了与(AND)运算。
【1】对于any(iterables),如果可迭代对象iterables中任意存在每一个元素为True则返回True。特例:若可迭代对象为空,比如空列表[],则返回False。
【2】对于all(iterables),如果可迭代对象iterables中所有元素都为True则返回True。特例:若可迭代对象为空,比如空列表[],则返回True。
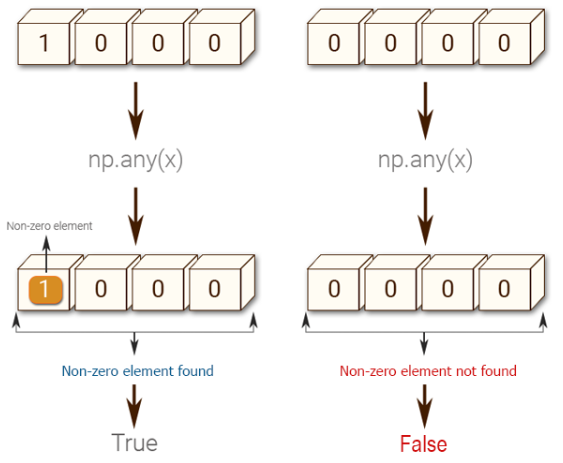
5、逐元素测试给定数组的有限性
编写一个 NumPy 程序来测试给定数组元素的有限性(不是无穷大或不是数字)。
import numpy as np
a = np.array([1, 0, np.nan, np.inf])
print("Original array")
print(a)
print("Test a given array element-wise for finiteness :")
print(np.isfinite(a))
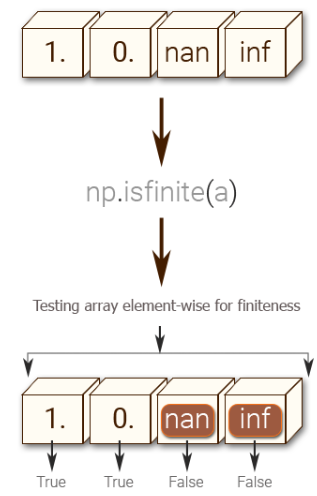
【1】math.isfinite():是数学模块的库方法,用于检查给定数是否为有限数,它接受数字(整数/浮点数,有限,无限或NaN)。如果 number 是有限数字(或可转换为有限数字),那么返回 true。否则,如果 number 是 NaN(非数字),或者是正、负无穷大的数,则返回 false。
【2】np.nan:表示not a number
【3】np.inf :表示+∞,是没有确切的数值的,类型为浮点型
6、逐元素测试正无穷大或负无穷大
编写一个 NumPy 程序来逐个元素测试正无穷大或负无穷大
import numpy as np
a = np.array([1, 0, np.nan, np.inf])
print("Original array")
print(a)
print("Test element-wise for positive or negative infinity:")
print(np.isinf(a))
'''
Original array
[ 1. 0. nan inf]
Test element-wise for positive or negative infinity:
[False False False True]
'''
【1】np.isinf(x):判断x数组当中是否有正无穷大或者负无穷大的数,返回值是布尔类型的list
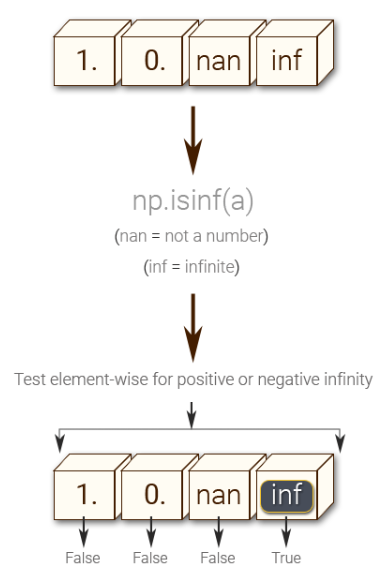
7、逐元素测试给定数组的 NaN
编写一个 NumPy 程序来逐个元素测试给定数组的 NaN
import numpy as np
a = np.array([1, 0, np.nan, np.inf])
print("Original array")
print(a)
print("Test element-wise for NaN:")
print(np.isnan(a))
'''
Original array
[ 1. 0. nan inf]
Test element-wise for NaN:
[False False True False]
'''
【1】np.isnan(x):判断x数组当中是否有Nan值,返回值是布尔类型的list
8、逐元素测试给定数组的复数、实数
编写一个 NumPy 程序来逐个元素测试给定数组的复数、实数。还要测试给定数字是否为标量类型。 【1】np.iscomplex(a):测试a数组当中是否存在复数,返回值是布尔类型的list 【2】np.isreal(a):测试a数组当中是否存在实数,返回值是布尔类型的list 【3】np.isscalar(3.1):测试3.1是否是标量,返回值是布尔类型
import numpy as np
a = np.array([1+1j, 1+0j, 4.5, 3, 2, 2j])
print("Original array")
print(a)
print("Checking for complex number:")
print(np.iscomplex(a))
print("Checking for real number:")
print(np.isreal(a))
print("Checking for scalar type:")
print(np.isscalar(3.1))
print(np.isscalar([3.1]))
9、创建两个给定数组的元素比较
编写一个NumPy程序来创建两个给定数组的元素比较(更大,greater_equal,更少和less_equal)。
import numpy as np
x = np.array([3, 5])
y = np.array([2, 5])
print("Original numbers:")
print(x)
print(y)
print("Comparison - greater")
print(np.greater(x, y))
print("Comparison - greater_equal")
print(np.greater_equal(x, y))
print("Comparison - less")
print(np.less(x, y))
print("Comparison - less_equal")
print(np.less_equal(x, y))
'''
Original numbers:
[3 5]
[2 5]
Comparison - greater
[ True False]
Comparison - greater_equal
[ True True]
Comparison - less
[False False]
Comparison - less_equal
[False True]
'''
【1】np.greater(x, y):判断x是否大于y 【2】np.greater_equal(x, y):判断x是否大于等于y 【3】np.less(x, y):判断x是否小于y 【4】np.less_equal(x, y):判断x是否小于等于y
10、创建数组,并确定数组占用的内存大小
编写一个NumPy程序来创建一个值为1,7,13,105的数组,并确定数组占用的内存大小。
import numpy as np
X = np.array([1, 7, 13, 105])
print("Original array:")
print(X)
print("Size of the memory occupied by the said array:")
print("%d bytes" % (X.size * X.itemsize))
'''
Original array:
[ 1 7 13 105]
Size of the memory occupied by the said array:
32 bytes
'''
【1】X.size:求数组X当中的元素个数 【2】X.itemsize:X数组中一个元素的长度(字节)
11、创建从 30 到 70 的整数数组
import numpy as np
array=np.arange(30,71)
print("Array of the integers from 30 to70")
print(array)
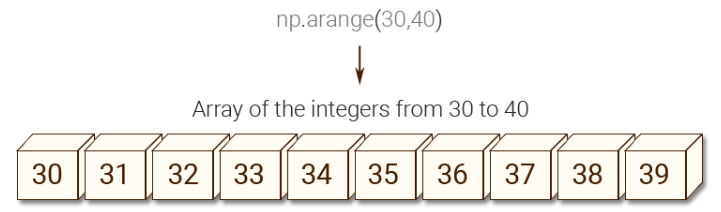
12、创建3x3单位矩阵
编写一个NumPy程序来创建一个3x3单位矩阵。
import numpy as np
array_2D=np.identity(3)
print('3x3 matrix:')
print(array_2D)
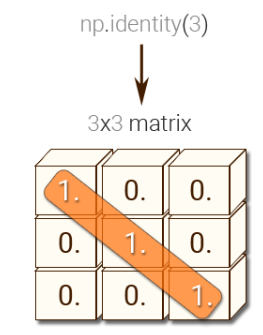
13、创建包含从30到70的所有偶数整数的数组
编写一个NumPy程序来创建一个包含从30到70的所有偶数整数的数组。
import numpy as np
array=np.arange(30,71,2)
print("Array of all the even integers from 30 to 70")
print(array)
14、创建由10个0,10个1,10个5组成的数组
编写一个NumPy程序来分别创建一个包含10个0,10个1,10个5的数组。
import numpy as np
array=np.zeros(10)
print("An array of 10 zeros:")
print(array)
array=np.ones(10)
print("An array of 10 ones:")
print(array)
array=np.ones(10)*5
print("An array of 10 fives:")
print(array)
'''
An array of 10 zeros:
[ 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.]
An array of 10 ones:
[ 1. 1. 1. 1. 1. 1. 1. 1. 1. 1.]
An array of 10 fives:
[ 5. 5. 5. 5. 5. 5. 5. 5. 5. 5.]
'''
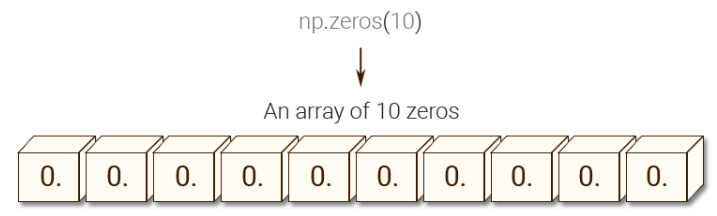 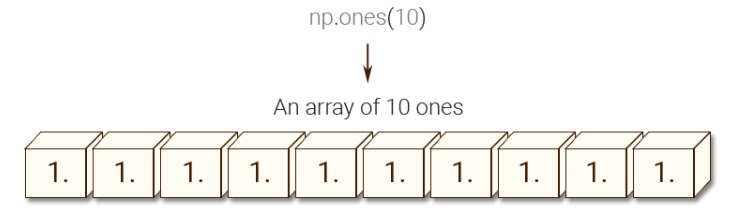 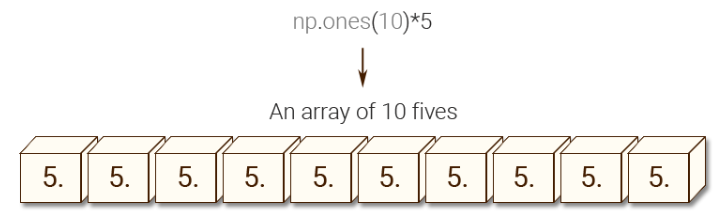
15、使用并迭代创建一个3X4数组
编写一个NumPy程序来创建一个3x4矩阵,其中填充了从10到21的值。
import numpy as np
a = np.arange(10,22).reshape((3, 4))
print("Original array:")
print(a)
print("Each element of the array is:")
for x in np.nditer(a):
print(x,end=" ")
'''
Original array:
[[10 11 12 13]
[14 15 16 17]
[18 19 20 21]]
Each element of the array is:
10 11 12 13 14 15 16 17 18 19 20 21
'''
【1】nditer:使用nditer可以完成逐个访问数组中的元素。 【2】end=" ":表示循环输出的元素后面以空格进行分割。 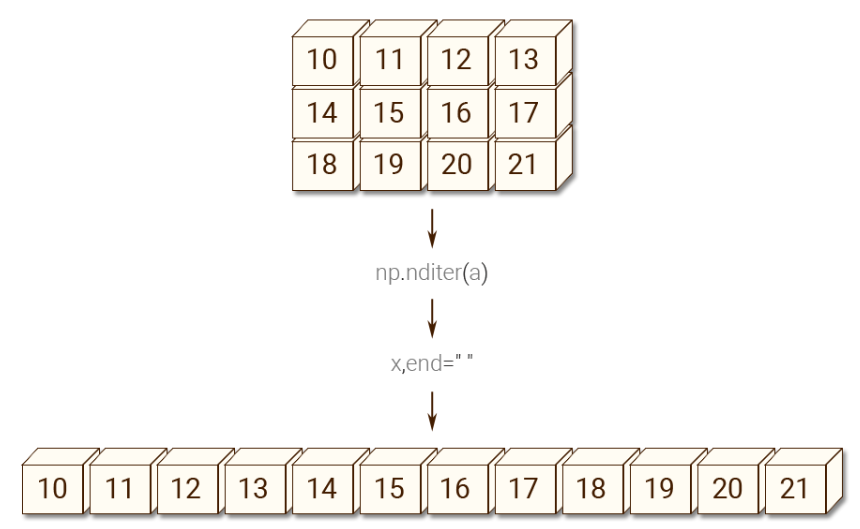
16、计算正弦曲线上点的x和y坐标并绘制
编写一个 NumPy 程序来计算正弦曲线上点的 x 和 y 坐标,并使用 matplotlib 绘制这些点。
import numpy as np
import matplotlib.pyplot as plt
x = np.arange(0, 3 * np.pi, 0.2)
y = np.sin(x)
print("Plot the points using matplotlib:")
plt.plot(x, y)
plt.show()
[0. 0.2 0.4 0.6 0.8 1. 1.2 1.4 1.6 1.8 2. 2.2 2.4 2.6 2.8 3. 3.2 3.4
3.6 3.8 4. 4.2 4.4 4.6 4.8 5. 5.2 5.4 5.6 5.8 6. 6.2 6.4 6.6 6.8 7.
7.2 7.4 7.6 7.8 8. 8.2 8.4 8.6 8.8 9. 9.2 9.4]
【1】np.sin(x): 表示对x元素取正弦 【2】我们做出来的x是离散的点,可以看到这里用plt.plot(x, y) 把离散的点变成了光滑的曲线 【3】np.pi:就是π(3.1415926…)
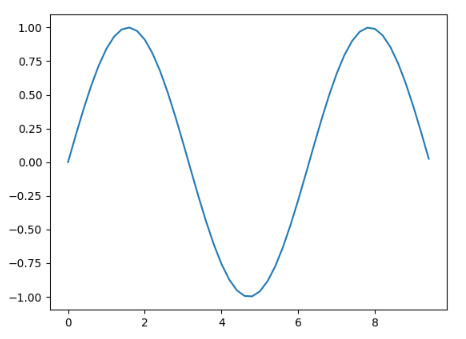
17、查找给定数组中缺少的数据
编写一个NumPy程序来查找给定数组中缺少的数据。这题和第七题是一样的,都是使用isnan函数。
import numpy as np
nums = np.array([[3, 2, np.nan, 1],
[10, 12, 10, 9],
[5, np.nan, 1, np.nan]])
print("Original array:")
print(nums)
print("\nFind the missing data of the said array:")
print(np.isnan(nums))
'''
Original array:
[[ 3. 2. nan 1.]
[10. 12. 10. 9.]
[ 5. nan 1. nan]]
Find the missing data of the said array:
[[False False True False]
[False False False False]
[False True False True]]
'''
18、检查两个数组是否相等
编写一个NumPy程序来检查两个数组是否相等。
import numpy as np
nums1 = np.array([0.5, 1.5, 0.2])
nums2 = np.array([0.4999999999, 1.500000000, 0.2])
np.set_printoptions(precision=15)
print("\nTest said two arrays are equal (element wise) or not:?")
print(nums1 == nums2)
'''
Test said two arrays are equal (element wise) or not:?
[False True True]
'''
nums1 = np.array([0.5, 1.5, 0.23])
nums2 = np.array([0.4999999999, 1.5000000001, 0.23])
print("\nOriginal arrays:")
np.set_printoptions(precision=15)
print("\nTest said two arrays are equal (element wise) or not:?")
print(np.equal(nums1, nums2))
'''
Test said two arrays are equal (element wise) or not:?
[False False True]
'''
【1】nums1 == nums2:可以直接比较各个元素是否相等 【2】np.set_printoptions:用于控制Python中小数的显示精度 【3】np.equal(nums1, nums2):equals()方法用于验证数据是否等效
==和equal的区别
【1】这题当中可以看到,使用==和equals返回值都是一个布尔类型的list 【2】关于在pandas中的区别,看这篇文章: ==和equal的区别
19、创建从正态分布当中选的随机数组
创建一个形状为(10,4)的随机数的二维数组,从正态分布(200,7)中选择随机数。
import numpy as np
np.random.seed(20)
cbrt = np.cbrt(7)
nd1 = 200
print(cbrt * np.random.randn(10, 4) + nd1)
'''
[[201.6908267 200.37467631 200.68394275 195.51750123]
[197.92478992 201.07066048 201.79714021 198.1282331 ]
[200.96238963 200.77744291 200.61875865 199.05613894]
[198.48492638 198.38860811 197.55239946 200.47003621]
[199.91545839 202.99877319 202.01069857 200.77735483]
[199.67739161 193.89831807 202.14273593 202.54951299]
[199.53450969 199.7512602 199.79145727 202.97687757]
[200.24634413 196.04606934 198.30611253 197.88701546]
[201.78450912 203.94032834 198.21152803 196.91446071]
[201.0082481 197.03285104 200.63052763 197.82590294]]
'''
【1】np.random.seed(int):用于制定随机数生成时所用算法开始的整数值,使得随机数据可预测。如果使用相同的seed()值,则每次产生的随机数都相同;如果不设置这个值,则系统根据时间来自己选择这个值,因此每次生成的随机数会因为时间差异而不同。 【2】numpy.random.rand(d0,d1,…,dn):randn函数返回一个或一组样本,具有标准正态分布。dn表示每个维度,返回值为指定维度的array。np.random.randn(10, 4) 表示生成二维数组,有10行4列。 【3】numpy.cbrt(x):立方根。
20、按行和列升序对给定数组进行排序
编写一个NumPy程序,按行和列升序对给定数组进行排序。
import numpy as np
nums = np.array([[5.54, 3.38, 7.99],
[3.54, 4.38, 6.99],
[1.54, 2.39, 9.29]])
print("Original array:")
print(nums)
print("\nSort the said array by row in ascending order:")
print(np.sort(nums))
print("\nSort the said array by column in ascending order:")
print(np.sort(nums, axis=0))
'''
Original array:
[[5.54 3.38 7.99]
[3.54 4.38 6.99]
[1.54 2.39 9.29]]
Sort the said array by row in ascending order:
[[3.38 5.54 7.99]
[3.54 4.38 6.99]
[1.54 2.39 9.29]]
Sort the said array by column in ascending order:
[[1.54 2.39 6.99]
[3.54 3.38 7.99]
[5.54 4.38 9.29]]
'''
【1】np.sort(a, axis, kind, order) :对数组进行排序。 axis=1 是按照最后一个轴排序,axis=0 每一列各元素排序 axis=1 每一行各元素排序,默认-1 是按最后一个轴排序。
21、从给定数组中提取小于和大于指定数的数字
编写一个NumPy程序,从给定数组中提取所有小于和大于指定数字的数字。
import numpy as np
nums = np.array([[5.54, 3.38, 7.99],
[3.54, 4.38, 6.99],
[1.54, 2.39, 9.29]])
print("Original array:")
print(nums)
n = 5
print("\nElements of the said array greater than",n)
print(nums[nums > n])
n = 6
print("\nElements of the said array less than",n)
print(nums[nums < n])
'''
Original array:
[[5.54 3.38 7.99]
[3.54 4.38 6.99]
[1.54 2.39 9.29]]
Elements of the said array greater than 5
[5.54 7.99 6.99 9.29]
Elements of the said array less than 6
[5.54 3.38 3.54 4.38 1.54 2.39]
'''
这里应该也是使用了布尔索引
22、创建与原数组相同形状和数据类型的数组
编写一个NumPy程序来创建给定数组的相同形状和数据类型的数组。用 0.0 填充新数组。
import numpy as np
nums = np.array([[5.54, 3.38, 7.99],
[3.54, 8.32, 6.99],
[1.54, 2.39, 9.29]])
print("Original array:")
print(nums)
print("\nNew array of equal shape and data type of the said array filled by 0:")
print(np.zeros_like(nums))
【1】np.zeros_like(nums)函数:主要是想实现构造一个矩阵W_update,其维度与矩阵W一致,并为其初始化为全0;这个函数方便的构造了新矩阵,无需参数指定shape大小。
23、以相反的顺序交换给定数组的行和列
编写一个NumPy程序,以相反的顺序交换给定数组的行和列。
import numpy as np
nums = np.array([[[1, 2, 3, 4],
[0, 1, 3, 4],
[90, 91, 93, 94],
[5, 0, 3, 2]]])
print("Original array:")
print(nums)
print("\nSwap rows and columns of the said array in reverse order:")
new_nums = print(nums[::-1, ::-1])
print(new_nums)
'''
Original array:
[[[ 1 2 3 4]
[ 0 1 3 4]
[90 91 93 94]
[ 5 0 3 2]]]
Swap rows and columns of the said array in reverse order:
[[[ 5 0 3 2]
[90 91 93 94]
[ 0 1 3 4]
[ 1 2 3 4]]]
None
'''
【1】关于[::-1] 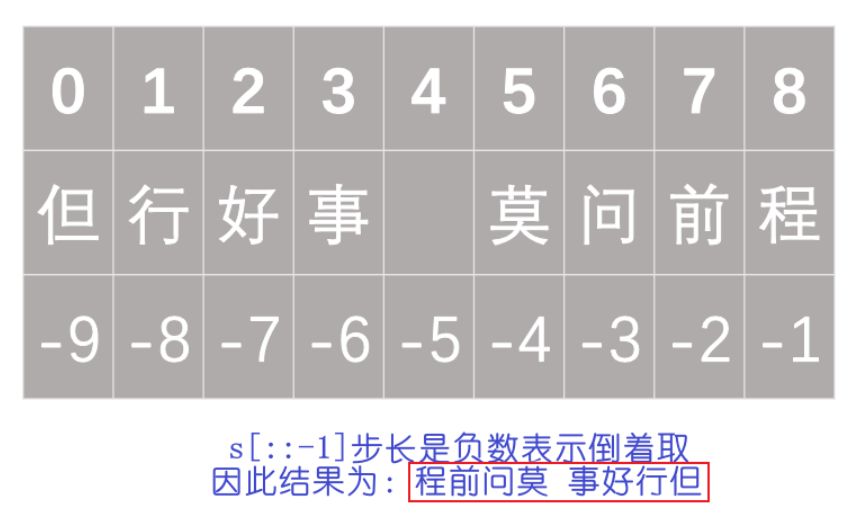 【2】关于[::-1, ::-1]
import numpy as np
a=np.random.rand(5)
print(a)
[ 0.64061262 0.8451399 0.965673 0.89256687 0.48518743]
print(a[-1])
[0.48518743]
print(a[:-1])
[ 0.64061262 0.8451399 0.965673 0.89256687]
print(a[::-1])
[ 0.48518743 0.89256687 0.965673 0.8451399 0.64061262]
print(a[2::-1])
[ 0.965673 0.8451399 0.64061262]
24、将两个相同大小的给定数组逐个元素相乘
编写一个NumPy程序,将两个相同大小的给定数组逐个元素相乘。
import numpy as np
nums1 = np.array([[2, 5, 2],
[1, 5, 5]])
nums2 = np.array([[5, 3, 4],
[3, 2, 5]])
print("Array1:")
print(nums1)
print("Array2:")
print(nums2)
print("\nMultiply said arrays of same size element-by-element:")
print(np.multiply(nums1, nums2))
【1】multiply(a,b):表示乘法,如果a,b是两个数组,那么对应元素相乘
25、转换给定数组
编写一个NumPy程序来创建一个给定形状(5,6)和类型的新数组,并用零填充。 更改以下格式的所述数组:
给定数组:
[[0 0 0 0 0 0 0]
[0 0 0 0 0 0]
[0 0 0 0 0 0]
[0 0 0 0 0 0]
[0 0 0 0 0 0]]
新数组:
[[3 0 3 0 3 0]
[7 0 7 0 7 0]
[3 0 3 0 3 0]
[7 0 7 0 7 0]
[3 0 3 0 3 0]]
import numpy as np
nums = np.zeros(shape=(5, 6), dtype='int')
print("Original array:")
print(nums)
nums[::2, ::2] = 3
nums[1::2, ::2] = 7
print("\nNew array:")
print(nums)
'''
Original array:
[[0 0 0 0 0 0]
[0 0 0 0 0 0]
[0 0 0 0 0 0]
[0 0 0 0 0 0]
[0 0 0 0 0 0]]
New array:
[[3 0 3 0 3 0]
[7 0 7 0 7 0]
[3 0 3 0 3 0]
[7 0 7 0 7 0]
[3 0 3 0 3 0]]
'''
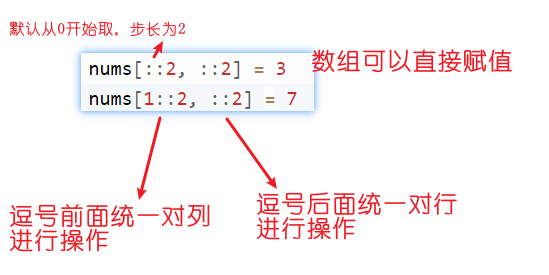 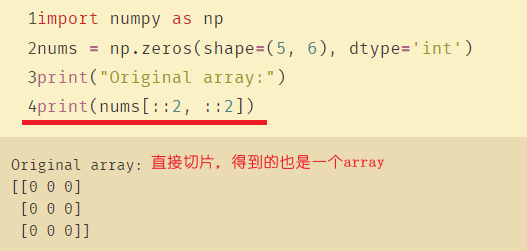
|