帮助文档查看器是很多程序中必备要素,而利用Qt中的QTreeView组件可以很方便的查看文件,而QTextBrowser可以直接显示格式化的MarkDown文本。因此可以利用这两个组件制作一个帮助文件查看器。 本程序的文件结构如下:
.
├── main.py
└── ui
└── docViewer.ui
└── docs --这里放帮助文档
├── a.md
├── b.md
└── c.md
如果使用命令
conda install matplotlib
会自动一起安装上pyqt 5.12 和 pyqtchart 5.12 但是qt 5.12 的文字查看器并没有显示Markdown或Html的功能。 需要用命令升级一下
pip install --upgrade pyqt5
运行界面
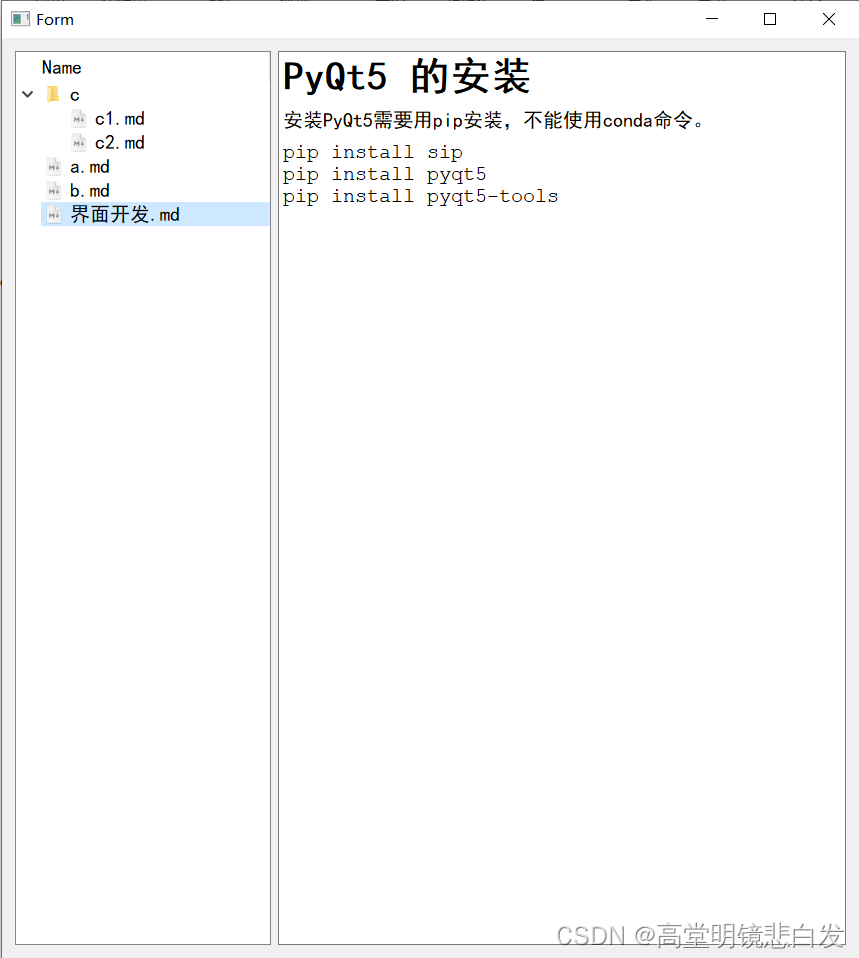
Python代码
from PyQt5.QtWidgets import QApplication,QWidget,QFileSystemModel
from PyQt5.uic import loadUi
from PyQt5.QtGui import QFont
import sys
import os
class Doc_Viewer(QWidget):
def __init__(self,parent=None) -> None:
super().__init__(parent)
loadUi('./ui/docViewer.ui',self)
self.__initUi()
def __initUi(self):
'''
初始化运行逻辑
'''
self.fileModel = QFileSystemModel()
pathIndex=self.fileModel.setRootPath(os.path.abspath("./docs/"))
print(self.fileModel.rootPath())
self.treeView.setModel(self.fileModel)
self.treeView.setRootIndex(pathIndex)
for i in [1,2,3]:
self.treeView.setColumnHidden(i, True)
self.treeView.clicked.connect(self.loadDoc)
def loadDoc(self,QpathIndex):
'''
加载文件
'''
filepath= self.fileModel.filePath(QpathIndex)
print(f"选择了文件【{filepath}】" )
if os.path.isfile(filepath):
markdown=open(filepath,mode="r",encoding="utf-8").read()
self.textBrowser.setMarkdown(markdown)
if __name__=="__main__":
app = QApplication(sys.argv)
app.setFont(QFont("黑体",12))
w = Doc_Viewer()
w.show()
sys.exit(app.exec())
UI文件
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>Form</class>
<widget class="QWidget" name="Form">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>857</width>
<height>920</height>
</rect>
</property>
<property name="windowTitle">
<string>Form</string>
</property>
<layout class="QHBoxLayout" name="horizontalLayout">
<item>
<widget class="QTreeView" name="treeView">
<property name="sizePolicy">
<sizepolicy hsizetype="Fixed" vsizetype="Expanding">
<horstretch>100</horstretch>
<verstretch>0</verstretch>
</sizepolicy>
</property>
</widget>
</item>
<item>
<widget class="QTextBrowser" name="textBrowser">
<property name="documentTitle">
<string/>
</property>
<property name="readOnly">
<bool>true</bool>
</property>
</widget>
</item>
</layout>
</widget>
<resources/>
<connections/>
</ui>
|