外包 | A Menu-Driven Proram
1. Question
Write a menu-driven program for De Anza College Food Court. (You need to use functions!)
-
Display the food menu to a user (Just show the 5 options’ names and prices - No need to show the Combos or the details!) 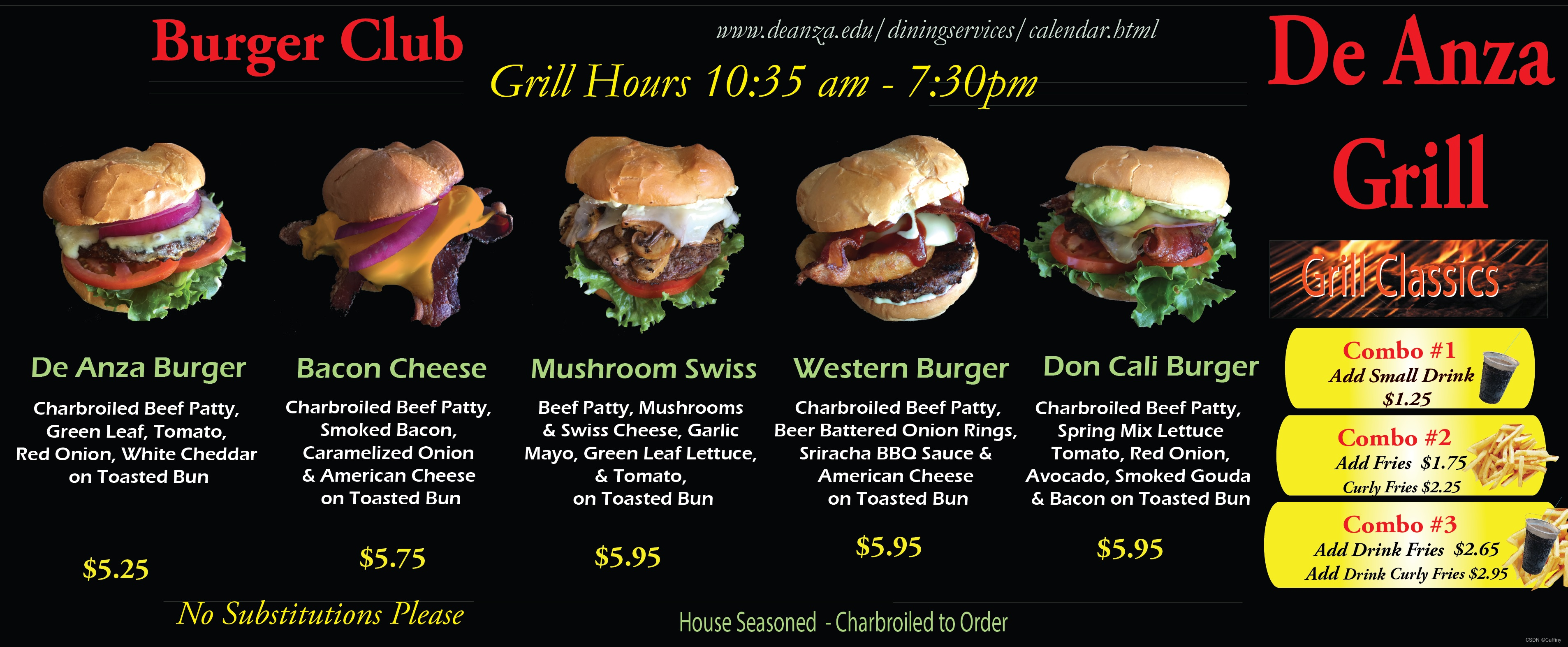 -
Ask the user what he/she wants and how many of it. (Check the user inputs) AND use strip() function to strip your inputs(if needed) -
Keep asking the user until he/she chooses the exit option. -
Calculate the price. -
Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price. -
Display the bill to the user. The bill includes:
- The food items
- The quantities
- The cost of them
- The total before tax
- Tax amount
- Total price after tax
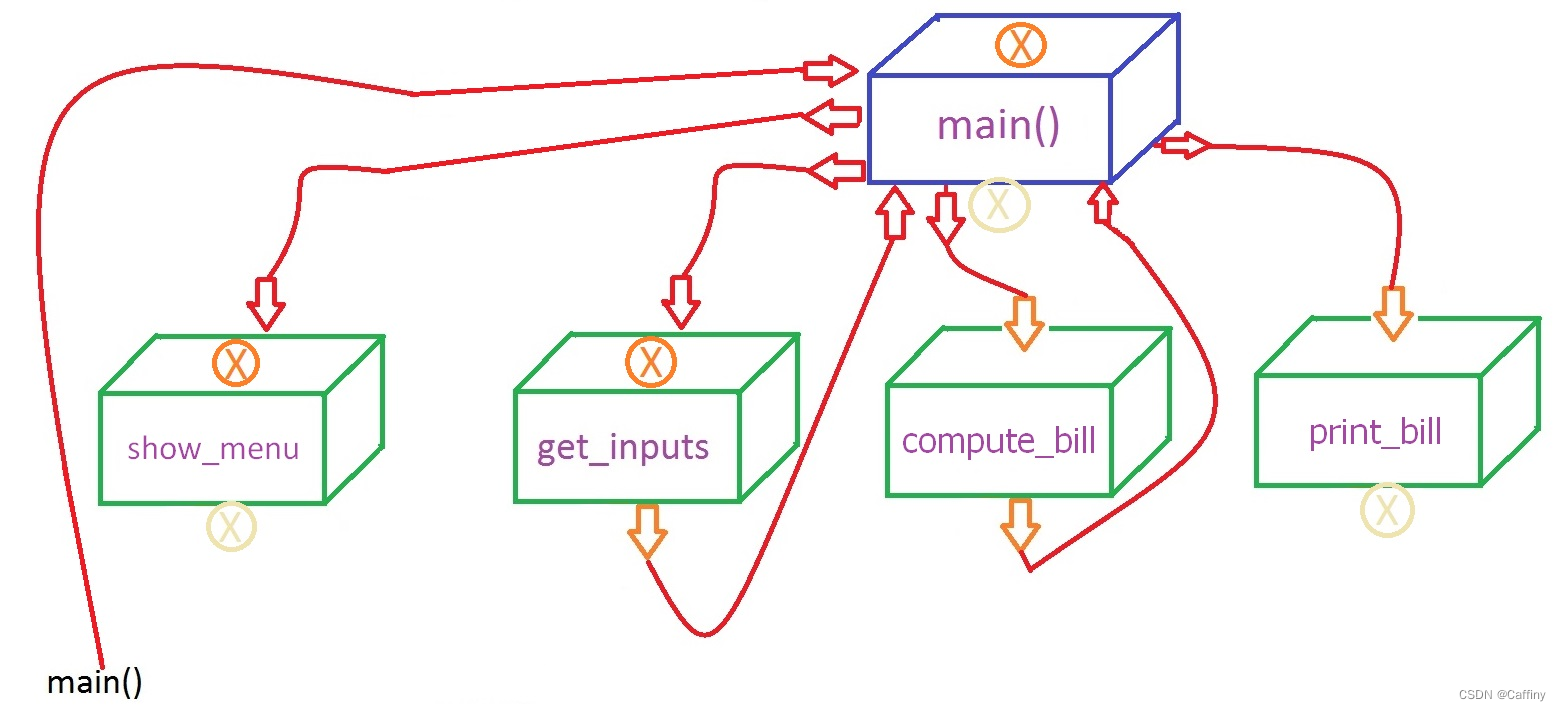 ?
2. Code
- Burger Menu
burger_Menu = [
(5.25, 'De Anza Burger'), (5.75, 'Bacon Cheese'),
(5.95, 'Mushroom Swiss'), (5.95, 'Western Burger'), (5.95, 'Don Cali Burger')
]
- show_menu()
def show_menu():
print('*' * 59)
print('*%10s%s%10s*' % ('', 'Welcome to De Anza College Food Court', ''))
print('*' * 59)
print('%10s%+10s%+20s' % ('', 'Price', 'Item'))
for i in range(len(burger_Menu)):
print('%10s%d%+9s%+20s' % ('', i+1, '$'+str(burger_Menu[i][0]), burger_Menu[i][1]))
print()
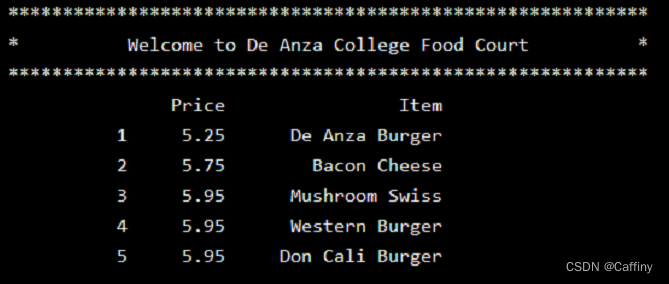 - get_input()
def get_input():
food_Items = [0 for _ in range(len(burger_Menu))]
while 1:
input_Option = input('(6 for exit)Input your Option: ').strip()
if input_Option not in '123456':
print('*Excuse Me*: Please Input Option from 1 to 6')
continue
else:
input_Option = int(input_Option)
if input_Option == 6:
print("Thank you, hope to see you again!\n")
break
else:
input_Num = input('How many you want: ').strip()
if input_Num.isdigit():
food_Items[input_Option-1] += int(input_Num)
else:
print('*Excuse Me*: Please enter an integer')
continue
return food_Items
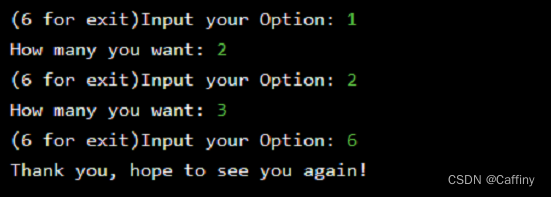 - compute_bill()
def compute_bill(food_Items):
food_bill = [0 for _ in range(len(burger_Menu))]
for i in range(len(food_Items)):
food_bill[i] = burger_Menu[i][0] * food_Items[i]
total_noTax = sum(food_bill)
is_Student = input('Are you a student?(y/n): ').strip().lower()
if is_Student == 'y' or is_Student == 'yes':
tax = 0
else:
tax = total_noTax * 0.09
total_withTax = total_noTax + tax
return food_bill, total_noTax, tax, total_withTax
- print_bill()
def print_bill(food_Items, food_bill, total_noTax, tax, total_withTax):
print('\n*%20sHere is your bill%20s*' % ('', ''))
print('%7s%-20s%-16s%-9s%10s' % ('', 'Item', 'Quantities', 'Cost', ''))
for i in range(len(food_Items)):
if food_Items[i] != 0:
print('%7s%-20s%-16s%-9s%10s' % ('', burger_Menu[i][1], food_Items[i], '$'+str(food_bill[i]), ''))
else:
continue
print()
print('The total before tax: $%.2f' % total_noTax)
print('Tax amount: $%.2f' % tax)
print('Total price after tax: $%.2f' % total_withTax)
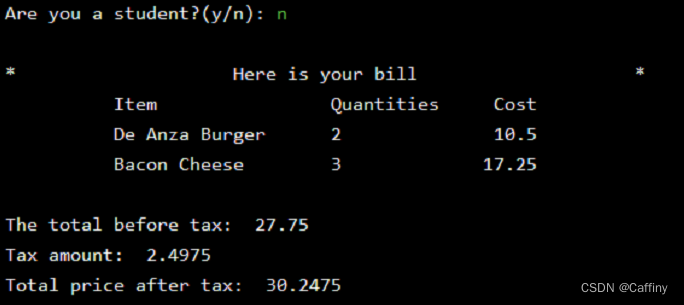 - main()
def main():
show_menu()
food_Items = get_input()
if sum(food_Items) != 0:
food_bill, total_noTax, tax, total_withTax = compute_bill(food_Items)
print_bill(food_Items, food_bill, total_noTax, tax, total_withTax)
3. Sample Run 1
***********************************************************
* Welcome to De Anza College Food Court *
***********************************************************
Price Item
1 $5.25 De Anza Burger
2 $5.75 Bacon Cheese
3 $5.95 Mushroom Swiss
4 $5.95 Western Burger
5 $5.95 Don Cali Burger
(6 for exit)Input your Option: 1
How many you want: 2
(6 for exit)Input your Option: 5
How many you want: 2
(6 for exit)Input your Option: 6
Thank you, hope to see you again!
Are you a student?(y/n): n
* Here is your bill *
Item Quantities Cost
De Anza Burger 2 $10.5
Don Cali Burger 2 $11.9
The total before tax: $22.40
Tax amount: $2.02
Total price after tax: $24.42
Process finished with exit code 0
?
4. Sample Run 2
***********************************************************
* Welcome to De Anza College Food Court *
***********************************************************
Price Item
1 $5.25 De Anza Burger
2 $5.75 Bacon Cheese
3 $5.95 Mushroom Swiss
4 $5.95 Western Burger
5 $5.95 Don Cali Burger
(6 for exit)Input your Option: abc
*Excuse Me*: Please Input Option from 1 to 6
(6 for exit)Input your Option: 9
*Excuse Me*: Please Input Option from 1 to 6
(6 for exit)Input your Option: 1
How many you want: 1.25
*Excuse Me*: Please enter an integer
(6 for exit)Input your Option: 1
How many you want: abc
*Excuse Me*: Please enter an integer
(6 for exit)Input your Option: 6
Thank you, hope to see you again!
Process finished with exit code 0
|