常用操作
基本
- 设置x/y轴的名字:plt.xlabel,ax.set_xlabel。labelpad可以调整文字和坐标轴的距离
- 设置坐标轴刻度:plt.xticks(x,x_自定义), ax.set_xticks。rotation可以调整文字的角度
- 设置title:plt.title(), ax.set_title()
- 绘制多个图:plt.subplot(122)
- 检查字体
import matplotlib as mpl
mpl.font_manager.fontManager.ttflist
- 保存图片
ax.get_figure ().savefig(‘name’,dpi=400) plt.savefig(‘name’,dpi=400) - 显示中文
import matplotlib.pyplot as plt
plt.rcParams['font.sans-serif'] = ['SimHei']
plt.rcParams['axes.unicode_minus'] = False
ax=plt.gca()
ax.spines['right'].set_color('none')
ax.spines['top'].set_color('none')
- 开始设置图像的大小
plt.figure(figsize=(10, 5), dpi=400) - 设置图注:
plt.legend() 在画图的时候每个图加一个label 就可以 另一种方式
line1, = plt.plot(xxx, label='女生购物欲望')
line2, = plt.plot(xxx, label='男生购物欲望')
plt.legend(handles=[line1, line2], labels=['girl购物欲望','boy购物欲望'], loc='best',title="图例标题")
颜色 colormap
详见https://blog.csdn.net/sinat_32570141/article/details/105226330
各种图
饼状图
参考https://blog.csdn.net/weixin_46649052/article/details/115321326
import matplotlib.pyplot as plt
labels = 'Frogs', 'Hogs', 'Dogs', 'Logs'
sizes = [15, 30, 45, 10]
colors = ['yellowgreen', 'gold', 'lightskyblue', 'lightcoral']
explode = (0, 0.1, 0, 0)
plt.pie(sizes, explode=explode, labels=labels, colors=colors, autopct='%1.1f%%', shadow=True, startangle=90)
plt.axis('equal')
plt.show()
折线图
参考https://zhuanlan.zhihu.com/p/110656183
import matplotlib.pyplot as plt
n = [0, 1, 2, 3, 4]
m = [2, 3, -1, 1, -2]
plt.plot(n, m,
color = 'k',
linestyle = '-.',
linewidth = 3,
marker = 'p',
markersize = 15,
markeredgecolor = 'b',
markerfacecolor = 'r')
plt.show()
柱状图
参考https://zhuanlan.zhihu.com/p/25128216
import numpy as np
import matplotlib.pyplot as plt
size = 5
x = np.arange(size)
a = np.random.random(size)
b = np.random.random(size)
c = np.random.random(size)
total_width, n = 0.8, 3
width = total_width / n
x = x - (total_width - width) / 2
plt.bar(x, a, width=width, label='a')
plt.bar(x + width, b, width=width, label='b')
plt.bar(x + 2 * width, c, width=width, label='c')
plt.legend()
plt.show()
横过来的柱状图
import numpy as np
import matplotlib.pyplot as plt
a = np.array([5, 20, 15, 25, 10])
b = np.array([10, 15, 20, 15, 5])
plt.barh(range(len(a)), a)
plt.barh(range(len(b)), -b)
plt.show()
极坐标柱状图
import numpy as np
import matplotlib.pyplot as plt
N = 8
theta = np.linspace(0.0, 2 * np.pi, N, endpoint=False)
radii = np.random.randint(3,15,size = N)
width = np.pi / 4
colors = np.random.rand(8,3)
ax = plt.subplot(111, projection='polar')
ax.bar(
x=theta,
height=radii,
width=width,
bottom=bottom,
linewidth=2,
edgecolor='white',
color=colors
)
箱线图
别人博客学的骚操作
参考https://www.cnblogs.com/Big-Big-Watermelon/p/14052165.html
marker
普通marker 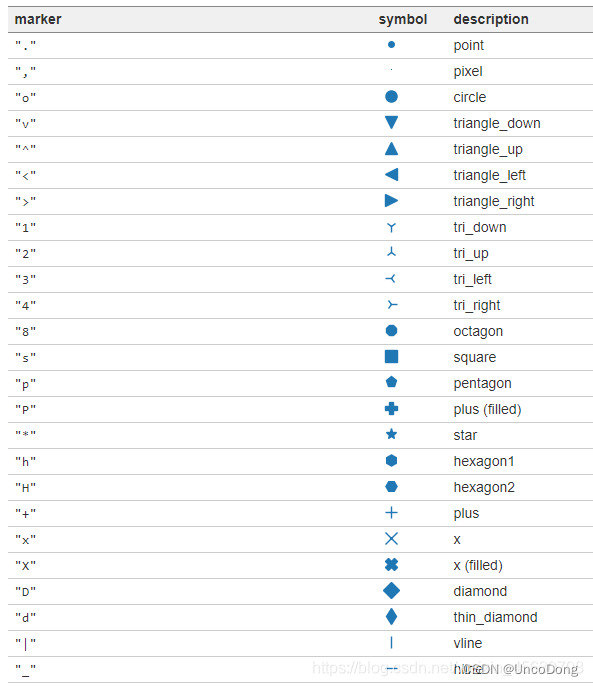 数学符号marker
可以显示的形状 marker名称
? \varpi
? \varrho
? \varsigma
? \vartheta
ξ \xi
ζ \zeta
Δ \Delta
Γ \Gamma
Λ \Lambda
Ω \Omega
Φ \Phi
Π \Pi
Ψ \Psi
Σ \Sigma
Θ \Theta
Υ \Upsilon
Ξ \Xi
? \mho
? \nabla
? \aleph
? \beth
? \daleth
? \gimel
/ /
[ [
? \Downarrow
? \Uparrow
‖ \Vert
↓ \downarrow
? \langle
? \lceil
? \lfloor
? \llcorner
? \lrcorner
? \rangle
? \rceil
? \rfloor
? \ulcorner
↑ \uparrow
? \urcorner
\vert
{ \{
\|
} \}
] ]
|
? \bigcap
? \bigcup
? \bigodot
? \bigoplus
? \bigotimes
? \biguplus
? \bigvee
? \bigwedge
? \coprod
∫ \int
∮ \oint
∏ \prod
∑ \sum
自定义marker
import matplotlib.pyplot as plt
plt.rcParams['font.sans-serif']=['SimHei']
plt.rcParams['axes.unicode_minus'] = False
plt.figure(dpi=200)
plt.plot([1,2,3],[1,2,3],marker=4, markersize=15, color='lightblue',label='常规marker')
plt.plot([1.8,2.8,3.8],[1,2,3],marker='2', markersize=15, color='#ec2d7a',label='常规marker')
plt.plot([1,2,3],[4,5,6],marker='$\circledR$', markersize=15, color='r', alpha = 0.5 ,label='非常规marker')
plt.plot([1.5,2.5,3.5],[1.25,2.1,6.5],marker='$\heartsuit$', markersize=15, color='#f19790', alpha=0.5,label='非常规marker')
plt.plot([1,2,3],[2.5,6.2,8],marker='$\clubsuit$', markersize=15, color='g', alpha=0.5,label='非常规marker')
plt.plot([1.2,2.2,3.2],[1,2,3],marker='$666$', markersize=15, color='#2d0c13',label='自定义marker')
plt.legend(loc='upper left')
for i in ['top','right']:
plt.gca().spines[i].set_visible(False)
线的形状
字符型linestyle
linestyle_str = [
('solid', 'solid'),
('dotted', 'dotted'),
('dashed', 'dashed'),
('dashdot', 'dashdot')]
元组型linestyle 可以通过修改元组中的数字来呈现出不同的线型,因此可以构造出无数种线型。
linestyle_tuple = [
('loosely dotted', (0, (1, 10))),
('dotted', (0, (1, 1))),
('densely dotted', (0, (1, 2))), ('loosely dashed', (0, (5, 10))),
('dashed', (0, (5, 5))),
('densely dashed', (0, (5, 1))), ('loosely dashdotted', (0, (3, 10, 1, 10))),
('dashdotted', (0, (3, 5, 1, 5))),
('densely dashdotted', (0, (3, 1, 1, 1))), ('dashdotdotted', (0, (3, 5, 1, 5, 1, 5))),
('loosely dashdotdotted', (0, (3, 10, 1, 10, 1, 10))),
('densely dashdotdotted', (0, (3, 1, 1, 1, 1, 1)))]
线型使用代码:
import matplotlib.pyplot as plt
plt.figure(dpi=120)
plt.plot([1,2,3],[1,2,13],linestyle='dotted', color='#1661ab', linewidth=5, label='字符型线型:dotted')
plt.plot([0.8,0.9,1.5],[0.8,0.9,21.5],linestyle=(0,(3, 1, 1, 1, 1, 1)), color='#ec2d7a', linewidth=5, label='元组型线型:(0,(3, 1, 1, 1, 1, 1)')
for i in ['top','right']:
plt.gca().spines[i].set_visible(False)
plt.plot([1.5,2.5,3.5],[1,2,13],linestyle=(0,(1,2,3,4,2,2)), color='black', linewidth=5, label='自定义线型:(0,(1,2,3,4,2,2)))')
plt.plot([2.5,3.5,4.5],[1,2,13],linestyle=(2,(1,2,3,4,2,2)), color='g', linewidth=5, label='自定义线型:(1,(1,2,3,4,2,2)))')
plt.legend()
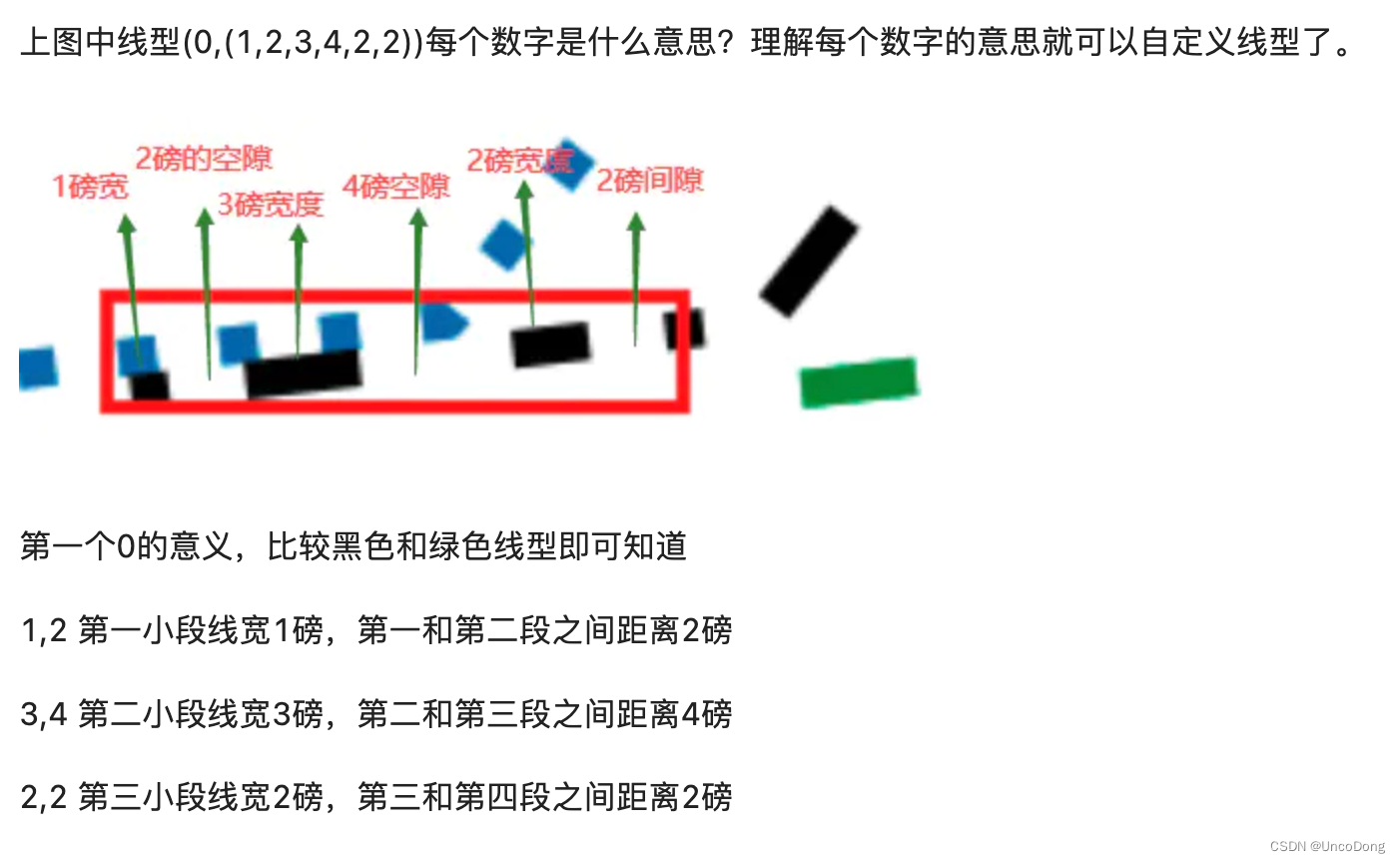 不得不说这哥们写的真好
常用颜色
图片都是我从人家博客复制来的,csdn这个beyond自己打了水印   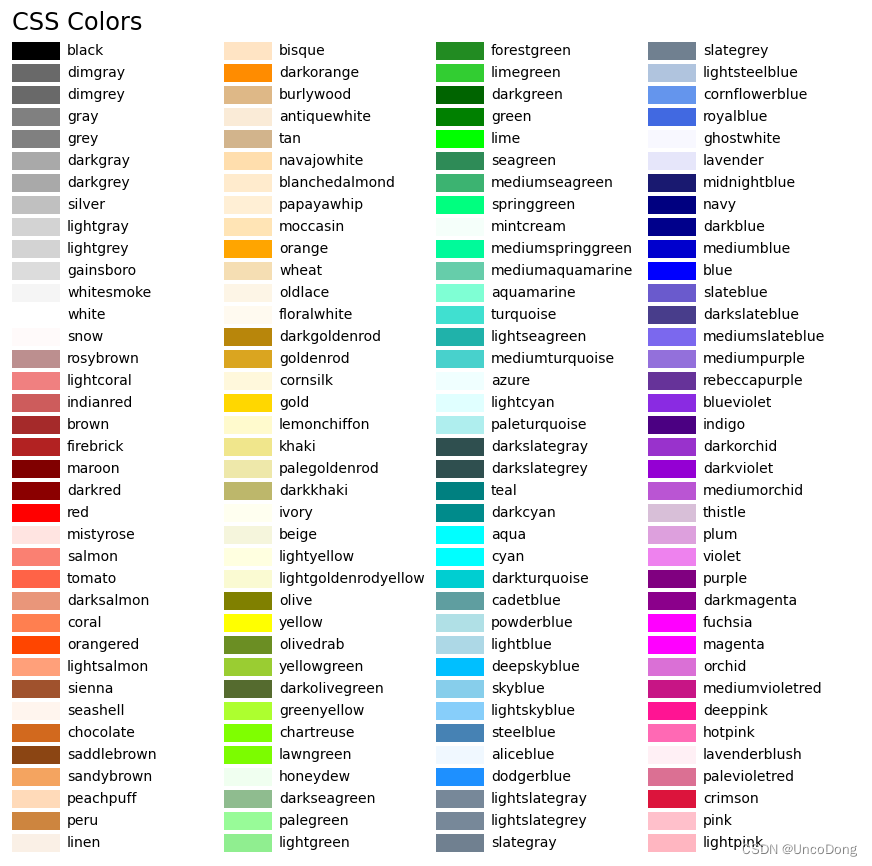 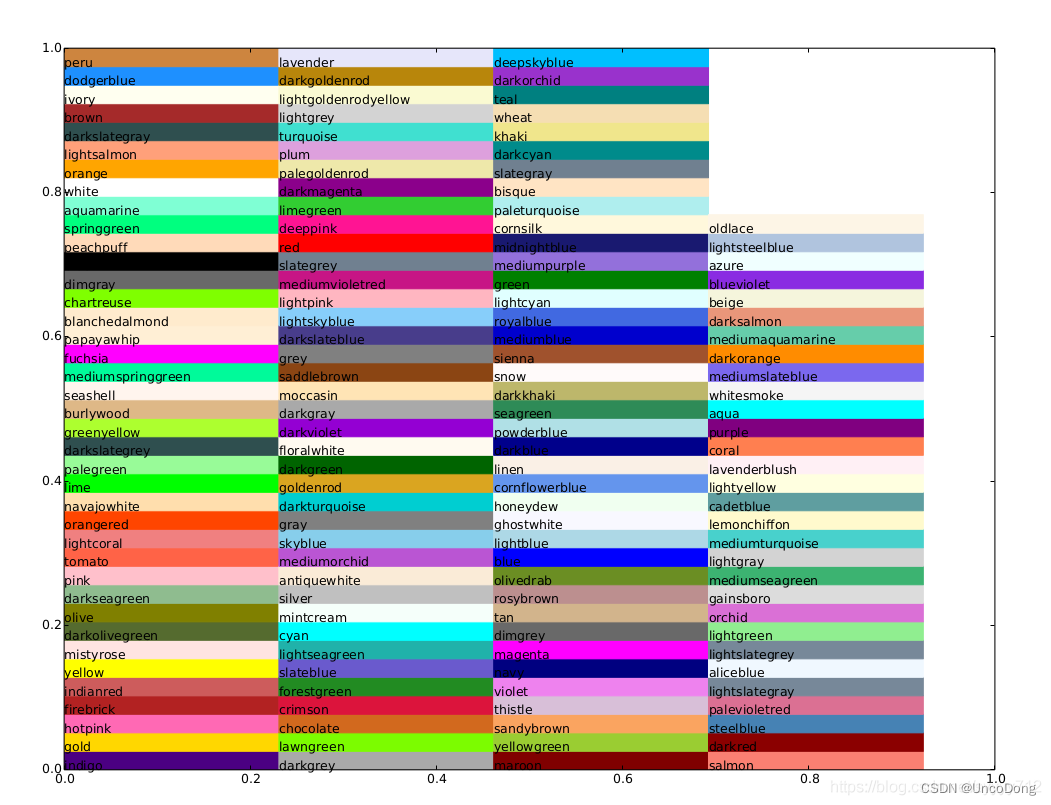 装了seaborn扩展的话,在字典seaborn.xkcd_rgb中包含所有的xkcd crowdsourced color names。 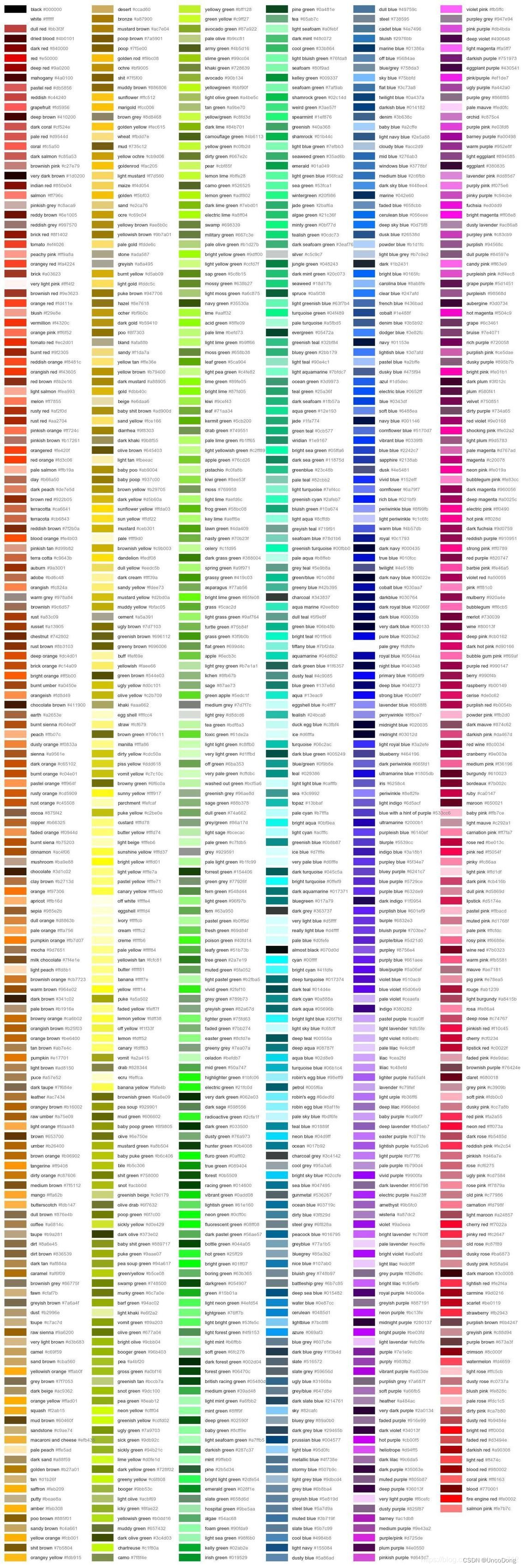
|