整体思维导图
1、变量赋值
2、容器序列的深浅拷贝
3、字典与collections扩展内置数据类型
4、函数调用
5、变量作用域(命名空间)
6、高阶函数
7、闭包、装饰器
8、对象协议与鸭子类型
9、yield语句
10、异步协程
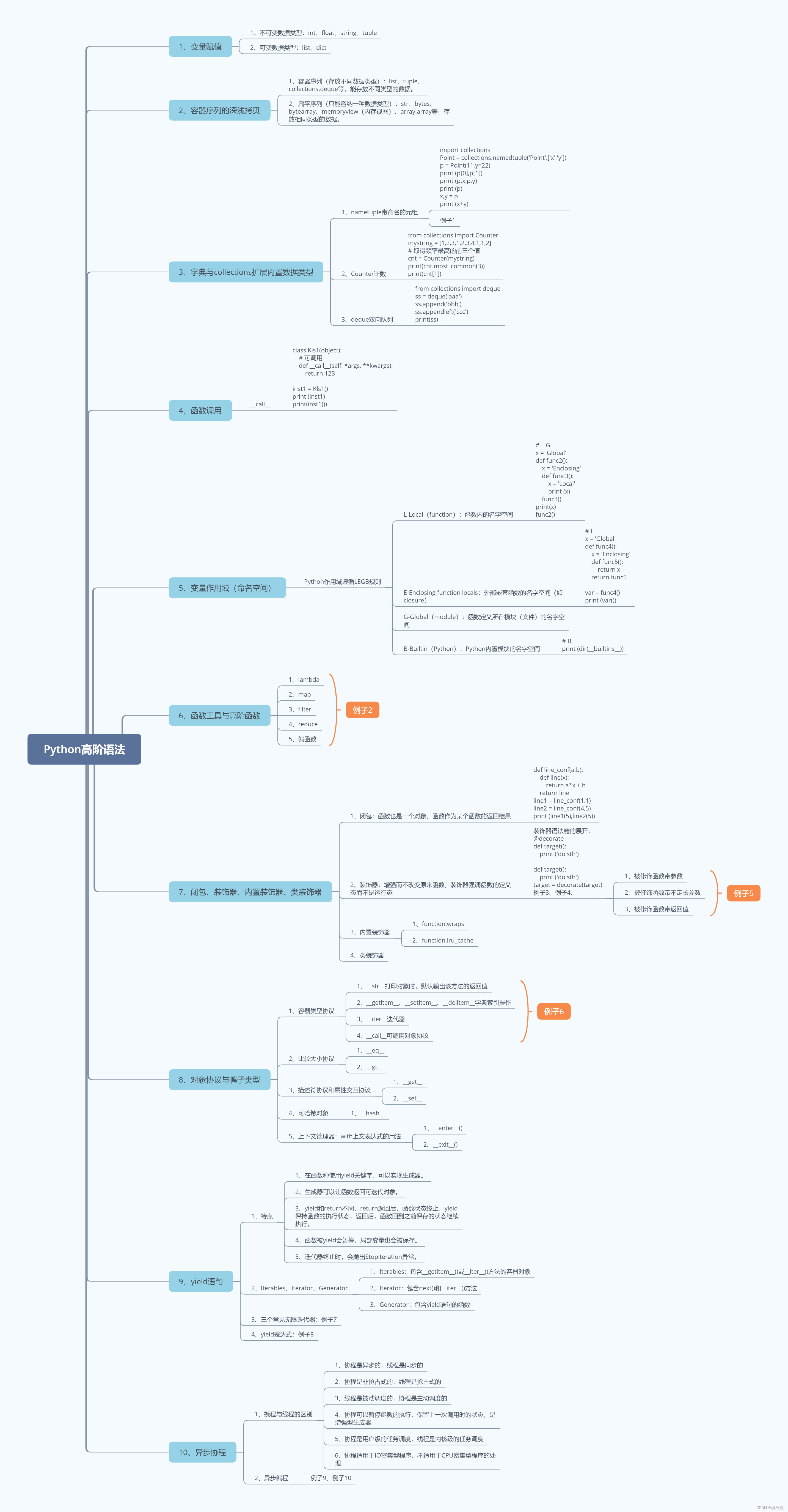
代码示例
- 例子1
"""
计算三维点的欧氏距离
"""
import numpy as np
vector1 = np.array([1,2,3])
vector2 = np.array([4,5,6])
op1 = np.sqrt(np.sum(np.square(vector1-vector2)))
op2 = np.linalg.norm(vector1-vector2)
print(f'op1:{op1},op2:{op2}')
from collections import namedtuple
from math import sqrt
Point = namedtuple('Point',['x','y','z'])
class Vector(Point):
def __init__(self,p1,p2,p3):
self.p1 = p1
self.p2 = p2
self.p3 = p3
def __sub__(self, other):
tmp = (self.p1-other.p1)**2 + (self.p2-other.p2)**2 + (self.p3-other.p3)**2
return sqrt(tmp)
p1 = Vector(1,2,3)
p2 = Vector(4,5,6)
print (p1-p2)
2.例子2
k = lambda x : x +1
print (k(1))
def square(x):
return x**2
m = map(square,range(5))
print(next(m))
print (next(m))
print(list(m))
li = [square(x) for x in range(5)]
print (li)
from functools import reduce
def add(x,y):
return x+y
ad = reduce(add,[0,1,2,3,4,5,6,7,8,9,10])
print(ad)
def is_odd(n):
return n%2 == 1
li = list(filter(is_odd,[1,2,3,4,5,6]))
print (li)
def add(x,y):
return x+y
from functools import partial
add_2 = partial(add,1)
res = add_2(9)
print (res)
3.例子3
def decorate(func):
print ('running...')
def inner():
return func()
return inner
@decorate
def func2():
pass
4.例子4
def html(func):
def decorate():
return f'<html>{func()}</html>'
return decorate
def body(func):
def decorate():
return f'<body>{func()}</body>'
return decorate
@html
@body
def content():
return 'hello world'
con = content()
print (con)
5.例子5
"""
例子5
"""
def outer(func):
def inner(a,b):
print (f'inner:{func.__name__}')
print (a,b)
func(a,b)
return inner
@outer
def foo(a,b):
print (a+b)
print (f'foo:{foo.__name__}')
foo(1,2)
foo.__name__
def outer2(func):
def inner2(*args,**kwargs):
func(*args,**kwargs)
return inner2
@outer2
def foo2(a,b,c):
print (a+b+c)
foo2(1,2,3)
def outer3(func):
def inner3(*args,**kwargs):
ret = func(*args,**kwargs)
return ret
return inner3
@outer3
def foo3(a,b,c):
return (a+b+c)
r = foo3(1,1,3)
print (r)
def outer_arg(bar):
def outer(func):
def inner(*args,**kwargs):
ret = func(*args,**kwargs)
print (bar)
return ret
return inner
return outer
@outer_arg('foo_arg')
def foo(a,b,c):
return (a+b+c)
print (foo(1,1,1))
6.例子6
"""
例子6
"""
class Foo(object):
def __str__(self):
return '__str__ is called'
def __getitem__(self, key):
print (f'__getitem__ {key}')
def __setitem__(self, key, value):
print (f'__setitem__ {key},{value}')
def __delitem__(self, key):
print (f'__delitem__ {key}')
def __iter__(self):
return iter([i for i in range(5)])
bar = Foo()
print (bar)
bar['key1']
bar['key1'] = 'value1'
del bar['key1']
for i in bar:
print (i)
7.例子7
""""
例子7
"""
import itertools
count = itertools.count()
next(count)
next(count)
next(count)
cycle = itertools.cycle(('yes','no'))
next(cycle)
next(cycle)
next(cycle)
repeat = itertools.repeat(10,times=2)
next(repeat)
8.例子8
""""
例子8
"""
def jumping_range(up_to):
index = 0
while index < up_to:
jump = yield index
print (f'jump is {jump}')
if jump is None:
jump = 1
index += 1
print (f'index is {index}')
iterator = jumping_range(5)
print (next(iterator))
print (iterator.send(2))
print (next(iterator))
print (iterator.send(-1))
for x in iterator:
print (x)
9.例子9
"""
例子9
await接收的对象必须是awaitable对象
awaitable对象定义了__await__()方法
awaitable对象有三类:1、协程 coroutine 2、任务 Task 3、未来对象 Future
"""
import asyncio
async def main():
print ('hello')
await asyncio.sleep(3)
print ('world')
asyncio.run(main())
10.例子10
from multiprocessing import Pool
import asyncio
import time
async def test(time):
await asyncio.sleep(time)
async def main(num):
start_time = time.time()
tasks = [asyncio.create_task(test(1)) for proxy in range(num)]
[await t for t in tasks]
print (time.time() - start_time)
def run(num):
asyncio.run(main(num))
if __name__ == '__main__':
start_time = time.time()
p = Pool()
for i in range(4):
p.apply_async(run,args=(2500,))
p.close()
p.join()
print (f'total {time.time()-start_time}')
|