背景是在工作中,需要给业务方提供一堆明细数据,从数据库里取出来的明细数据超过csv文件打开的上限了,业务方没法用,所以就需要对其进行拆分
python读写csv文件测试
先配置相关包并定义一个结果文件的存储路径
import csv
import os
result_path = 'D:\Python_Project\CSV文件拆分\结果文件'
往csv文件写入数据的时候有两种方式,writerow 和writerows
with open(result_path + '\三国.csv','w', newline='') as file:
writer = csv.writer(file)
writer.writerow(["刘备", "玄德", "蜀"])
writer.writerow(["曹操", "孟德", "魏"])
writer.writerow(["孙权", "仲谋", "吴"])
writer.writerow(["董卓", "仲颖", "其它"])
一行一行的往里面添加,结果如下图 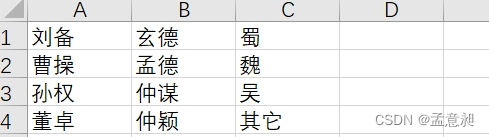
list = [["关羽", "云长", "蜀"],
["夏侯惇", "元让", "魏"],
["甘宁", "兴霸", "吴"],
["吕布", "奉先", "其他"]]
with open(result_path + '\三国.csv','a', newline='') as file:
writer = csv.writer(file)
writer.writerows(list)
writerows 则是准备一个列表,一次直接插入,结果如下图 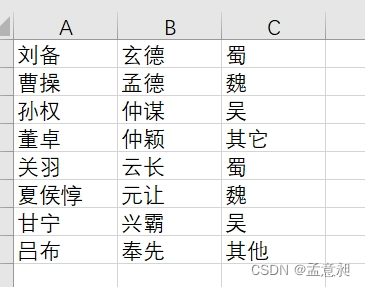
准备一个需要切分的csv文件
新建一个csv文件,并直接准备10000行,后续每1000行拆分1次 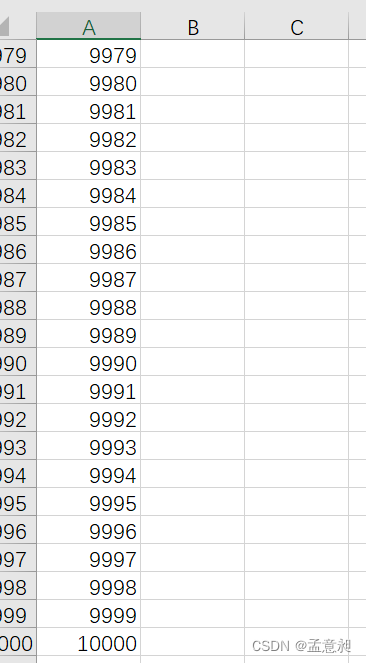 确定输入文件路径和输出文件路径,其中i是文件的第i行,j是用来表示第j个输出的文件
example_path = 'D:\Python_Project\CSV文件拆分\example.csv'
example_result_dir = 'D:\Python_Project\CSV文件拆分'
with open(example_path, 'r', newline='') as example_file:
example = csv.reader(example_file)
i = j = 1
for row in example:
print(row)
print(f'i 等于 {i}, j 等于 {j}')
if i % 1000 == 0:
j += 1
print(f'第{j}个文件生成完成')
example_result_path = example_result_dir + '\example_result' + str(j) + '.csv'
print(example_result_path)
对于没有输出文件的情况,就新创建一个
if not os.path.exists(example_result_path):
with open(example_result_path, 'w', newline='') as file:
csvwriter = csv.writer(file)
csvwriter.writerow(['这是一个表头'])
csvwriter.writerow(row)
i += 1
如果存在输出文件的话,则直接追加内容
else:
with open(example_result_path, 'a', newline='') as file:
csvwriter = csv.writer(file)
csvwriter.writerow(row)
i += 1
输出结果如下 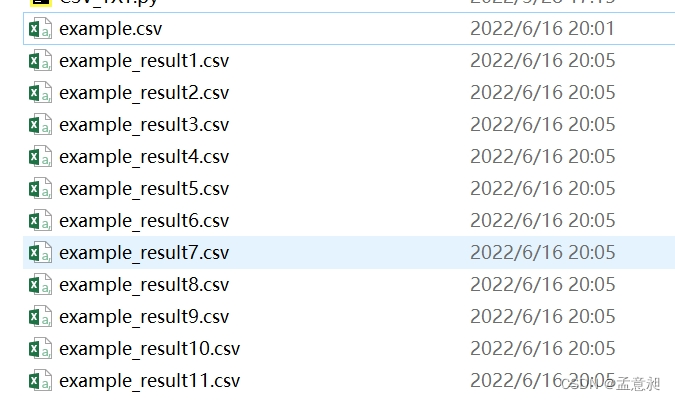 完整代码如下:
import csv
import os
result_path = 'D:\Python_Project\CSV文件拆分\结果文件'
with open(result_path + '\三国.csv','w', newline='') as file:
writer = csv.writer(file)
writer.writerow(["刘备", "玄德", "蜀"])
writer.writerow(["曹操", "孟德", "魏"])
writer.writerow(["孙权", "仲谋", "吴"])
writer.writerow(["董卓", "仲颖", "其它"])
list = [["关羽", "云长", "蜀"],
["夏侯惇", "元让", "魏"],
["甘宁", "兴霸", "吴"],
["吕布", "奉先", "其他"]]
with open(result_path + '\三国.csv','a', newline='') as file:
writer = csv.writer(file)
writer.writerows(list)
example_path = 'D:\Python_Project\CSV文件拆分\example.csv'
example_result_dir = 'D:\Python_Project\CSV文件拆分'
with open(example_path, 'r', newline='') as example_file:
example = csv.reader(example_file)
i = j = 1
for row in example:
print(row)
print(f'i 等于 {i}, j 等于 {j}')
if i % 1000 == 0:
j += 1
print(f'第{j}个文件生成完成')
example_result_path = example_result_dir + '\example_result' + str(j) + '.csv'
print(example_result_path)
if not os.path.exists(example_result_path):
with open(example_result_path, 'w', newline='') as file:
csvwriter = csv.writer(file)
csvwriter.writerow(['这是一个表头'])
csvwriter.writerow(row)
i += 1
else:
with open(example_result_path, 'a', newline='') as file:
csvwriter = csv.writer(file)
csvwriter.writerow(row)
i += 1
|