Kalman滤波器真的太复杂了,因此整理了这篇文章,来记录一下对自己有用的一些参考链接。 如果用我自己的话来总结kalman滤波器(可能不准确):
- 要观测的目标自身存在一个运动状态(状态方程);
- 在这个目标身上我安装了一些传感器(观测方程);
- 我可以通过它上一个的运动状态,来预测此时刻的位置;
- 我也可以通过传感器来直接检测出它此时刻的位置;
- 但是这个世界存在着误差,我无论预测还是检测都可能不准;
- 因此我打算将预测值和检测值数据融合一下;
- 在数据融合过程中,我更相信预测值还是检测值,那就用到卡尔曼增益(Kk);
- Kalman帮我决定更相信预测值还是检测值进行了量化,Kalman根据协方差矩阵、状态观测矩阵等写出了一个方程,让估计误差最小。
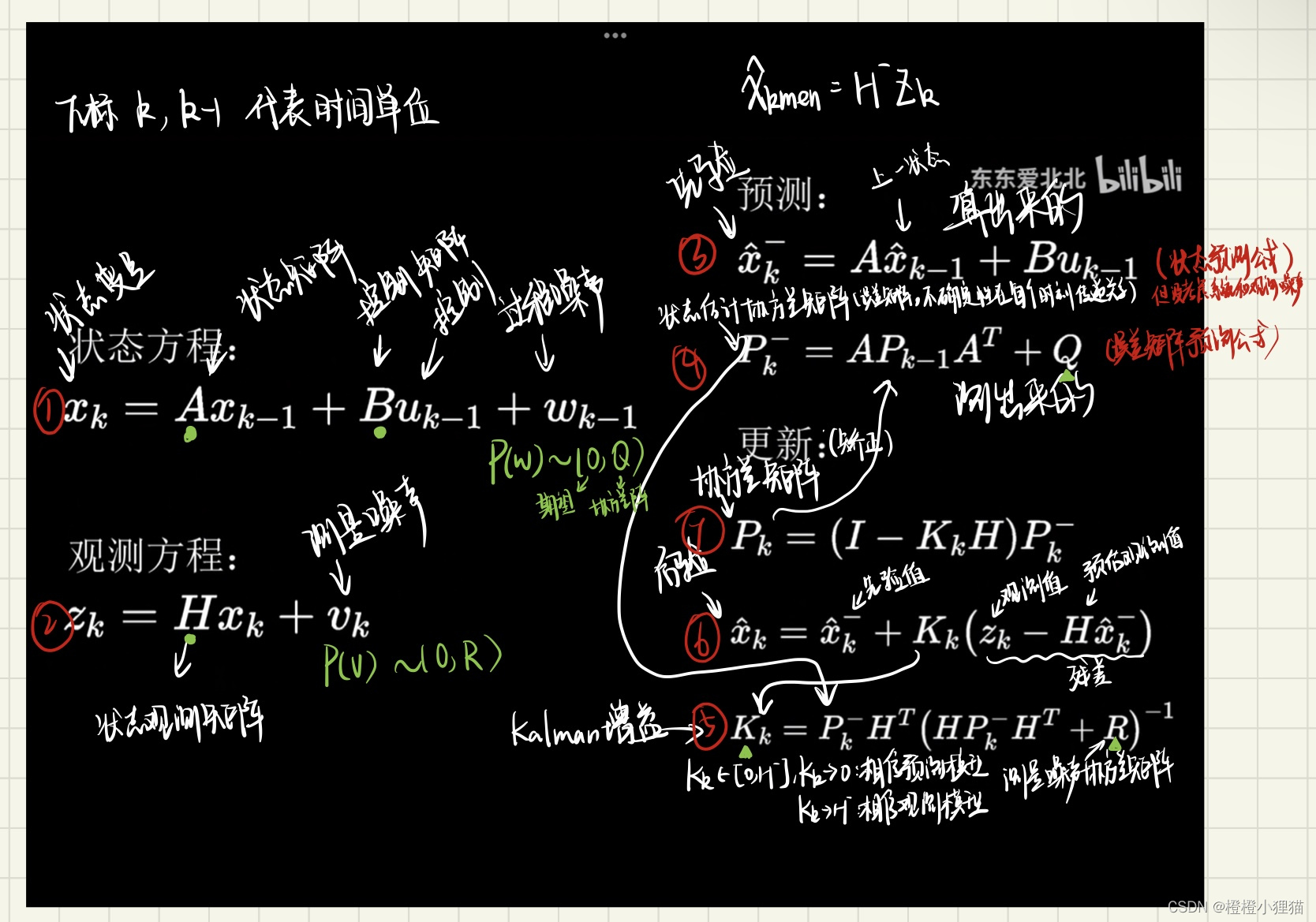
Kalman跟踪直观感受
Kalman原理
Kalman应用
opencv官方kalman函数说明 cv::KalmanFilter Class Reference
自己写了个类 输入值为目标的中心坐标和长宽,输入预测的坐标位置和速度,可能存在部分错误,需后期修正。
import cv2
import numpy as np
class KalmanTrack:
def __init__(self):
self.kalman = cv2.KalmanFilter(6, 4)
A = np.array([[1, 0, 0, 0, 1, 0],
[0, 1, 0, 0, 0, 1],
[0, 0, 1, 0, 0, 0],
[0, 0, 0, 1, 0, 0],
[0, 0, 0, 0, 1, 0],
[0, 0, 0, 0, 0, 1]], np.float32)
self.kalman.transitionMatrix = A
B = None
self.kalman.controlMatrix = B
H = np.array([[1, 0, 0, 0, 0, 0],
[0, 1, 0, 0, 0, 0],
[0, 0, 1, 0, 0, 0],
[0, 0, 0, 1, 0, 0]], np.float32)
self.kalman.measurementMatrix = H
R = np.array([[1, 0, 0, 0],
[0, 1, 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1]], np.float32)
self.kalman.measurementNoiseCov = R
Q = np.eye(6, dtype=np.float32) * 0.1
self.kalman.processNoiseCov = Q
P = np.eye(6, dtype=np.float32)
self.kalman.errorCovPre = P
self.cur_measurement = np.nan
self.cur_prediction = np.nan
self.pre_measurement = np.nan
self.pre_prediction = np.nan
def get_cur_state(self, target_box):
"""获取初始值状态测量值"""
self.cur_measurement = target_box
self.cur_measurement = np.array(
[[np.float32(self.cur_measurement[0]), np.float32(self.cur_measurement[1]),
np.float32(self.cur_measurement[2]), np.float32(self.cur_measurement[3])]]).T
return self.cur_measurement
def get_initial_state(self, target_box):
self.cur_measurement = self.get_cur_state(target_box)
self.pre_measurement = self.cur_measurement
self.cur_prediction = self.cur_measurement
self.pre_prediction = self.cur_measurement
def correct_and_predict(self, target_box):
self.pre_measurement = self.cur_measurement
self.pre_prediction = self.cur_prediction
self.cur_measurement = self.get_cur_state(target_box)
self.kalman.correct(self.cur_measurement)
self.cur_prediction = self.kalman.predict()
return self.cur_prediction
if __name__ == '__main__':
kalman_tracker = KalmanTrack()
kalman_tracker.get_initial_state([729, 288, 35, 101])
while True:
data = list([kalman_tracker.pre_prediction[0][0], kalman_tracker.pre_prediction[1][0],
kalman_tracker.pre_prediction[2][0], kalman_tracker.pre_prediction[3][0]])
print(kalman_tracker.correct_and_predict(data))
|