新建子应用
在现在的后台项目里再创建一个goods子应用 再settings.py文件里注册子应用 路由分发,新建urls.py文件 配置序列化器,创建serializers.py
创建模型类
在子应用下的models.py文件中创建
from django.db import models
class Brand(models.Model):
first_name=models.CharField('品牌首字母',max_length=10,default=None)
name=models.CharField('品牌名称',max_length=20)
logo=models.CharField('品牌logo',max_length=200)
def __str__(self):
return self.name
class Meta:
db_table='brand'
verbose_name_plural='品牌'
生成迁移文件进行迁移
往里添加几条数据 例如:  需要配置静态文件 在根目录创建static文件夹 在项目根目录中的settings.py里进行配置
STATIC_URL = '/static/'
STATICFILES_DIRS=(
os.path.join(BASE_DIR,'static'),
)
编写序列化器
在子应用下创建serializers.py文件中创建
from rest_framework import serializers
from .models import *
class BrandSer(serializers.ModelSerializer):
logo=serializers.CharField(allow_null=True,allow_blank=True)
class Meta:
model=Brand
fields='__all__'
read_only_fields=['id']
编写视图类
from django.shortcuts import render
from rest_framework.viewsets import ModelViewSet
from rest_framework.views import APIView
from rest_framework.response import Response
from p6_306 import settings
from .models import *
from .serializers import *
import os
from rest_framework.pagination import PageNumberPagination
class MyPagination(PageNumberPagination):
page_size = 3
max_page_size = 5
page_query_param = 'page'
page_size_query_param = 'pageSize'
class BrandViewSet(ModelViewSet):
queryset = Brand.objects.all()
serializer_class = BrandSer
lookup_field = 'pk'
lookup_url_kwarg = 'pk'
pagination_class = MyPagination
class UploadLogoAPIView(APIView):
def post(self,request):
file=request.data.get('file')
static_path='static/images/logos'
file_path=os.path.join(settings.BASE_DIR,static_path)
file_name=os.path.join(file_path,file.name)
with open(file_name,'wb')as f:
f.write(file.file.read())
return Response({
'code':200,
'msg':'图片上传成功',
'static_path':static_path
})
配置路由
from django.urls import path
from rest_framework import routers
from .views import *
urlpatterns = [
path('upload/logo/',UploadLogoAPIView.as_view())
]
router=routers.SimpleRouter()
router.register('brands',BrandViewSet,'brands')
urlpatterns+=router.urls
前端
找到对应的vue组件进行配置 展示全部品牌信息 组件例如:Brands.vue
<script>
import BreadCrumb from '@/components/widget/BreadCrumb'
import Addbrands from '@/components/widget/AddBrands'
import BrandsTable from '@/components/widget/BrandsTable'
export default {
name: 'Brands',
data () {
return {
page:1,
total:0,
pagesize:2,
aBrandsList:[]
}
},
components:{
BreadCrumb,
Addbrands,
BrandsTable
},
mounted(){
this.fnGetData(1);
},
methods:{
fnGetData(num){
this.axios.get('/v1/goods/brands/',{
params:{
page:num,
pageSize:this.pageSize
},
headers:{
Authorization:'JWT '+localStorage.token
},
responseType:'json'
}).then((result) => {
console.log('加载所有品牌的响应',result)
if (result.status==200){
this.aBrandsList=result.data.results
this.total=result.data.count
}else{
this.$message({
type:'error',
message:'加载品牌错误'
})
}
}).catch((err) => {
console.log('加载失败的响应',err)
});
},
fnGetPage(page){
this.page=page
this.fnGetData(page)
},
}
}
</script>
展示删除修改的vue组件 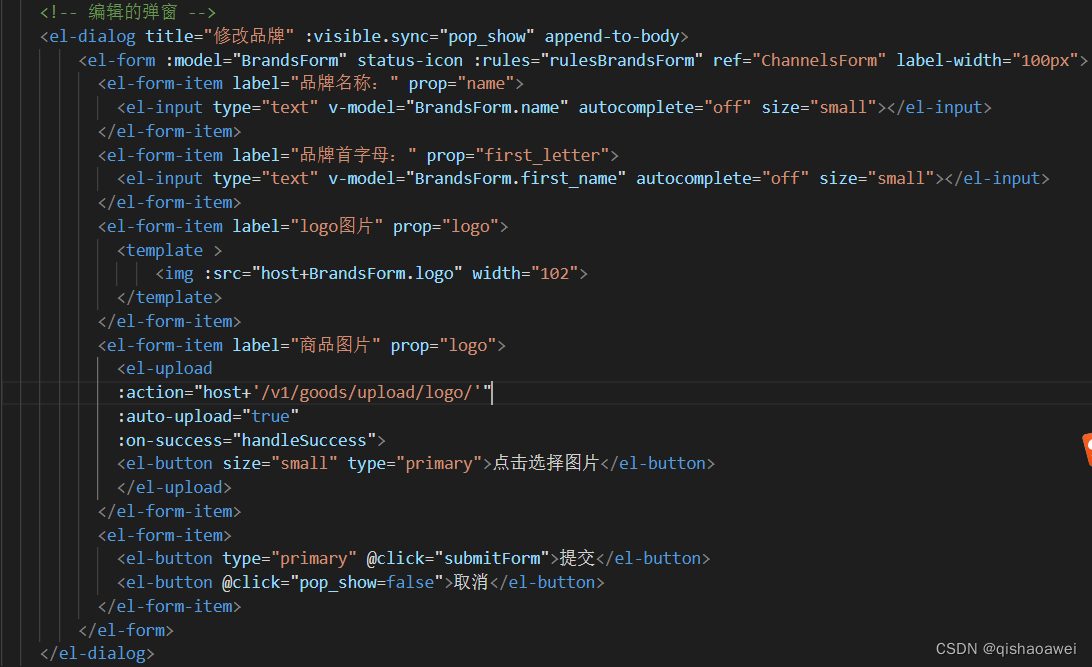
<script>
import cons from '../constant';
let token = localStorage.token;
export default {
name: 'OptionsTable',
props:['brands'],
data () {
return {
host:'http://127.0.0.1:8000',
pop_show:false,
group_type_list:[],
category_list:[],
BrandsForm:{
name:'',
first_name:'',
logo:''
},
fileList:[],
rulesBrandsForm:{}
}
},
methods:{
handleSuccess(res,files){
console.log('上传成功的响应',res)
this.BrandsForm.logo='/'+res.static_path+'/'+files.name
},
fnPopShow(id){
this.pop_show = true;
this.edit_id = id;
this.axios.get('/v1/goods/brands/'+this.edit_id+'/', {
headers: {
'Authorization': 'JWT ' + token
},
responseType: 'json',
})
.then(res=>{
this.BrandsForm.name = res.data.name;
this.BrandsForm.logo = res.data.logo;
this.BrandsForm.first_name = res.data.first_name;
}).catch(err=>{
console.log(err.response);
});
},
submitForm(){
this.axios.put('/v1/goods/brands/'+this.edit_id+'/', this.BrandsForm, {
headers: {
'Authorization': 'JWT ' + token
},
responseType: 'json'
}).then(res=>{
this.$message({
type: 'success',
message: '品牌修改成功!'
});
this.pop_show = false;
this.resetForm('ChannelsForm');
this.$emit('fnResetTable');
}).catch(err=>{
console.log(err.response);
})
},
fnDelBrands(id){
this.edit_id = id;
this.$confirm('此操作将删除该品牌, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
this.axios.delete('/v1/goods/brands/'+this.edit_id+'/',{
headers: {
'Authorization': 'JWT ' + token
},
responseType:'json'
}).then(res=>{
console.log('删除品牌的响应',res)
if (res.status==204){
this.$message({
type: 'success',
message: '删除品牌成功!'
});
this.$emit('fnResetTable');
}else{
this.$message({
type: 'error',
message: '删除品牌失败!'
});
}
}).catch(err=>{
if(err.response.status==404){
this.$message({
type:'info',
message:'图片未找到!'
});
}
})
}).catch(() => {
this.$message({
type: 'info',
message: '已取消删除'
});
});
},
resetForm(formName){
this.$refs[formName].resetFields();
}
},
mounted(){
this.fnGetChannelType();
this.fnGetCategories();
}
}
</script>
添加的组件
<script>
let token = localStorage.token;
export default {
name: 'AddChannels',
data () {
return {
daka:false,
host:'http://127.0.0.1:8000',
pop_show:false,
group_type_list:[],
category_list:[],
BrandsForm:{
name:'',
first_name:'',
logo:''
},
fileList:[],
rulesBrandsForm:{}
}
},
methods:{
handleSuccess(res,files){
console.log('上传成功的响应',res)
this.BrandsForm.logo='/'+res.static_path+'/'+files.name
this.daka=true
},
submitForm(){
this.axios.post('/v1/goods/brands/',this.BrandsForm,{
headers:{
Authorization:'JWT '+token
},
responseType:'json'
}).then((result) => {
if (result.status==201){
this.$message({
type:'success',
message:'添加品牌成功'
})
this.pop_show=false
this.resetForm('BrandsForm')
this.$emit('fnResetTable')
}else{
this.$message({
type:'error',
message:'添加品牌失败'
})
}
}).catch((err) => {
console.log('添加品牌错误',err)
});
},
fnGetChannelType(){},
fnGetCategories(){},
resetForm(formName){
this.$refs[formName].resetFields();
this.daka=false
}
},
mounted(){
this.fnGetChannelType();
this.fnGetCategories();
}
}
</script>
|