观看视频 本次课,我们将实现小球在碰到窗口的边缘时发生反弹,先来看一下图。 参考:pygame中的Rect对象 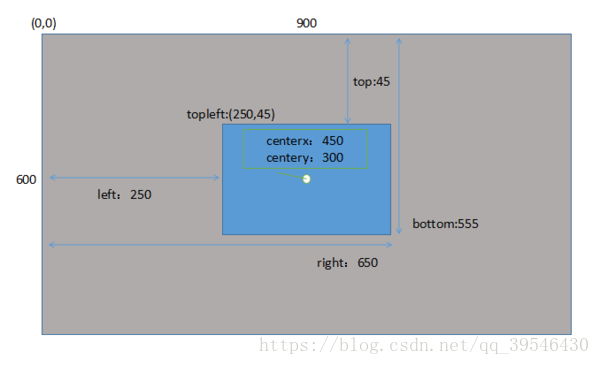
示例1:让小球动起来
下面的代码,球没有移动的原因就是move() 方法错误,move(x,y) 方法:返回按给定偏移量移动后的新矩形。x和y参数可以是任何整数值(正数或负数)。而原来的Rect对象并没有移动,需要将图像绘制在新的Rect对象中,这样才能产生动画
import pygame, sys
pygame.init()
screen = pygame.display.set_mode((600,480))
pygame.display.set_caption("小小工坊")
ball = pygame.image.load('pygame/images/ball.gif')
ball_rect = ball.get_rect()
speed = 1
while True:
for e in pygame.event.get():
if e.type == pygame.QUIT:
pygame.quit()
sys.exit()
ball_rect.move(speed, speed)
screen.fill((0,0,255))
screen.blit(ball,ball_rect)
pygame.display.update()
因此,程序中ball_rect.move(speed, speed) 这句应改成:ball_rect = ball_rect.move(speed, speed)
import pygame, sys
pygame.init()
screen = pygame.display.set_mode((600,480))
pygame.display.set_caption("小小工坊")
ball = pygame.image.load('pygame/images/ball.gif')
ball_rect = ball.get_rect()
speed = 1
while True:
for e in pygame.event.get():
if e.type == pygame.QUIT:
pygame.quit()
sys.exit()
ball_rect = ball_rect.move(speed, speed)
screen.fill((0,0,255))
screen.blit(ball,ball_rect)
pygame.display.update()
通过修改,球一闪而过,速度太快,这时,我们需要用到time模块,设定每秒钟游戏的刷新频率,因此,应该添加:
fclock = pygame.time.clock()
……
fclock.tick(60)
示例2:让小球反弹
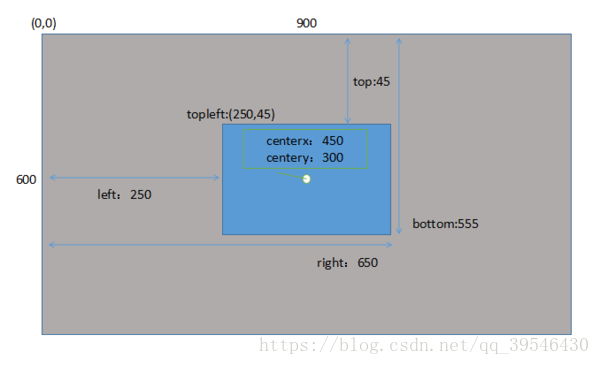 当小球碰到边缘的情况,就是绘制小球所在的Rect对象碰到边缘,有四种情况:
- top < =0:竖直方向的速度变为负值
- bottom > screenHeight: 竖直方向的速度变为正值
- left <= 0 :水平方向的速度变为正值
- right >= screenWidth:水平方向的速度变为负值
因此,程序需要改为:
import pygame, sys
pygame.init()
screen = pygame.display.set_mode((600,480))
pygame.display.set_caption("小小工坊")
ball = pygame.image.load('pygame/images/ball.gif')
ball_rect = ball.get_rect()
speed = 1
fclock = pygame.time.Clock()
while True:
for e in pygame.event.get():
if e.type == pygame.QUIT:
pygame.quit()
sys.exit()
ball_rect = ball_rect.move(speed, speed)
if ball_rect.right >= 600:
speed = -1
ball_rect.right = 600
if ball_rect.left <= 0:
speed = 1
ball_rect.left = 0
if ball_rect.bottom >= 480:
speed = -1
ball_rect.bottom = 480
if ball_rect.top <= 0:
speed = 1
ball_rect.top = 0
screen.fill((0,0,255))
screen.blit(ball,ball_rect)
pygame.display.update()
fclock.tick(200)
但是此时,小球一直在一条对角线上来回运动,这是因为:不论在水平或竖直方向,只要超出屏幕的边界,speed的值就会改变,而speed是水平和竖直两个方向的速度。这时就要考虑用两个值分别表示水平和竖直方向上的速度,这时可以考虑用列表:speed = [1, 1] 。这里能否用元组speed = (1, 1) 呢?
import pygame, sys
pygame.init()
screen = pygame.display.set_mode((600,480))
pygame.display.set_caption("小小工坊")
ball = pygame.image.load('pygame/images/ball.gif')
ball_rect = ball.get_rect()
speed = [1,1]
fclock = pygame.time.Clock()
while True:
for e in pygame.event.get():
if e.type == pygame.QUIT:
pygame.quit()
sys.exit()
ball_rect = ball_rect.move(speed[0], speed[1])
if ball_rect.right >= 600:
speed[0] = -1
ball_rect.right = 600
if ball_rect.left <= 0:
speed[0] = 1
ball_rect.left = 0
if ball_rect.bottom >= 480:
speed[1] = -1
ball_rect.bottom = 480
if ball_rect.top <= 0:
speed[1] = 1
ball_rect.top = 0
screen.fill((0,0,255))
screen.blit(ball,ball_rect)
pygame.display.update()
fclock.tick(200)
|