绝对导入与相对导入 包的概念 编程思维的转变 软件开发目录规范 常见内置模块
昨日内容概要
- 索引取值与迭代取值的差异
- 模块简介
- 导入模块的两种句式
- 导入模块的补充知识
- 循环导入问题
- 模块的查找顺序
今日内容详细
绝对导入与相对导入
所有涉及模块的导入sys.path都参照执行文件为准
绝对导入(推荐使用)
from ccc import b
from ccc.ddd.eee import b
相对导入(module only)
只能在模块文件中使用 不能在执行文件中使用 当项目比较复杂的时候 相对导入比较容易出错
def index():
from . import b
from .. import b
from ../.. import b
包的概念
什么是包
一个含有__init__.py文件的文件夹就是包
为什么有包
为更高效的管理py文件(模块文件)
如何使用
1.创建python package
2.内部放有多个同类型的模块
3. import python package
4. 在__init__.py文件内用相对导入的方式导入包内的模块
在python2中文件夹内必须要有__init__.py文件才会识别为包 而python3中没有严格要求
编程思想的转变
小白阶段>>>函数阶段>>>模块阶段 按需求从上往下堆叠代码>>>根据不同的功能将代码封装成不同的函数>>>根据不同的功能该分成不同的模块文件 单文件>>>多文件 一切都是为了能高效便捷地进行资源管理
软件开发目录规范
程序目录规范
1.bin文件夹 用于存储程序的启动文件 start.py 2.conf文件夹configuration 用于存储程序的配置文件 settings.py 3.core文件夹 用于存储程序的核心逻辑 src.py(source) 4.lib文件夹library 用于存储程序的公共功能 common.py 5.db文件夹database 用于存储程序的数据文件 userinfo.txt 6.log文件夹 用于存储程序的日志文件 log.log 7.interface文件夹(核心的延伸) 用于存储程序的接口文件 user.py order.py goods.py 8.readme文件(文本文件) 用于编写程序的说明、介绍、广告 类似于产品说明书 9.requirements.txt文件 用于存储程序所需的第三方模块名称和版本 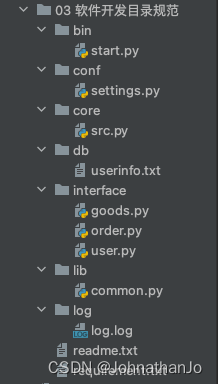
常见内置模块
collection模块(提供了更多的数据类型)
namedtuple 命名元组
from collections import namedtuple
Point = namedtuple('二维坐标系', ['x', 'y'])
res1 = Point(1, 3)
res2 = Point(10, 49)
print(res1, res2)
print(res1.x)
print(res1.y)
Point = namedtuple('三维坐标系', 'x y z')
deque 双端队列
from collections import deque
q = deque()
q.append(111)
q.append(222)
q.append(333)
q.append(444)
q.appendleft(555)
print(q)
OrderedDict 有序字典
from collections import OrderedDict
od = OrderedDict([('a', 1), ('b', 2), ('c', 3)])
print(od)
Counter 计数器
res = 'abcdeabcdabcaba'
new_dict = {}
for i in res:
if i not in new_dict:
new_dict[i] = 1
else:
new_dict[i] += 1
print(new_dict)
from collections import Counter
res1 = Counter(res)
print(res1)
time模块
1. time() 时间戳
2. time.gmtime() 结构化时间
3. time.strftime() 格式化时间
import time
print(time.time())
print(time.gmtime())
print(time.strftime('%Y-%m-%d %H:%M:%S'))
print(time.strftime('%Y-%m-%d'))
print(time.strftime('%H:%M:%S'))
print(time.strftime('%Y-%m-%d %X'))
print(time.strptime('2022-07-14 12:25:33','%Y-%m-%d %H:%M:%S'))
print(time.localtime())
三种时间格式的转换示意图 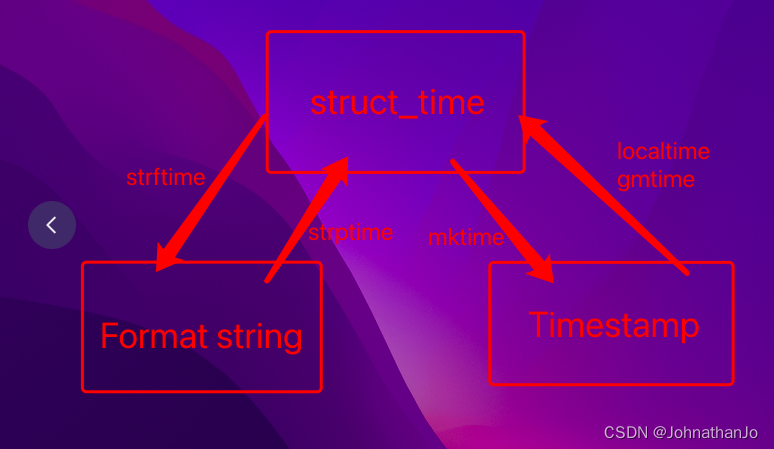
|