import pandas as pd
data = pd.read_csv(r"D:\本科\kaggle数据挖掘\titanic\train.csv", index_col = 0)
data.head()
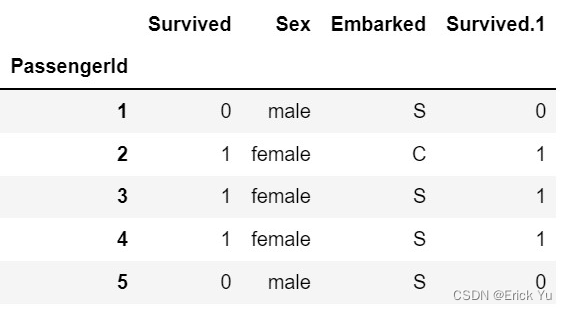
?处理缺失值:
from sklearn.impute import SimpleImputer
Embarked = data.loc[:, "Embarked"].values.reshape(-1,1)
imp_mode = SimpleImputer(strategy = "most_frequent") #most_frequent == 众数
data.loc[:,"Embarked"] = imp_mode.fit_transform(Embarked)
data.info()
?
<class 'pandas.core.frame.DataFrame'>
Int64Index: 891 entries, 1 to 891
Data columns (total 4 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 Survived 891 non-null int64
1 Sex 891 non-null object
2 Embarked 891 non-null object
3 Survived.1 891 non-null int64
dtypes: int64(2), object(2)
memory usage: 34.8+ KB
from sklearn.preprocessing import OneHotEncoder
X = data.iloc[:,0:-1]
X
?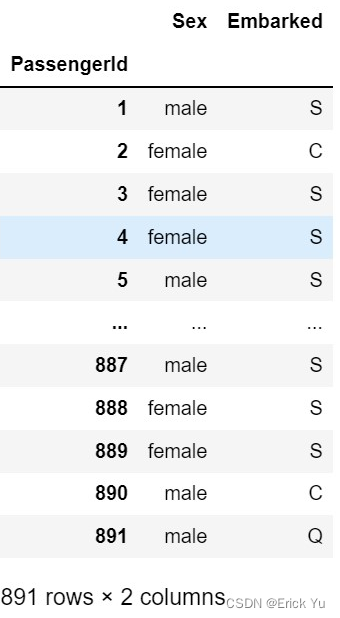
enc = OneHotEncoder(categories = 'auto').fit(X) #categories是自动属性——不用人为输入有什么属性(男、女),自动识别
result = enc.transform(X).toarray() #to array 转化成array
result
array([[0., 1., 0., 0., 1.],
[1., 0., 1., 0., 0.],
[1., 0., 0., 0., 1.],
...,
[1., 0., 0., 0., 1.],
[0., 1., 1., 0., 0.],
[0., 1., 0., 1., 0.]])
result.shape #查看result的shape
(891, 5)
891行,5列(3+2:
男:10
女:01
S:100
C:010
Q:001
result = pd.DataFrame(result)
result
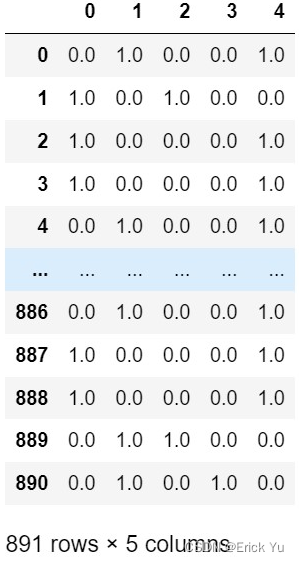
newdata = pd.concat([X,result], axis = 1)
newdata.head()
?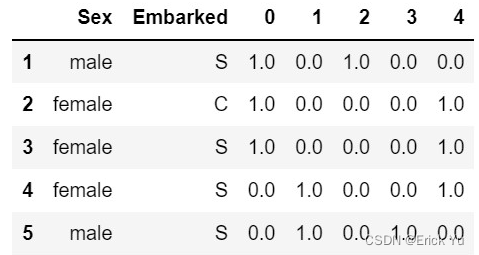
newdata.drop(["Sex", "Embarked"], axis = 1, inplace = True) #删除掉Sex和Embarked列
newdata.columns = ["Female", "Male", "Embarked_C", "Embarked_Q", "Embarked_S"]
newdata.head()
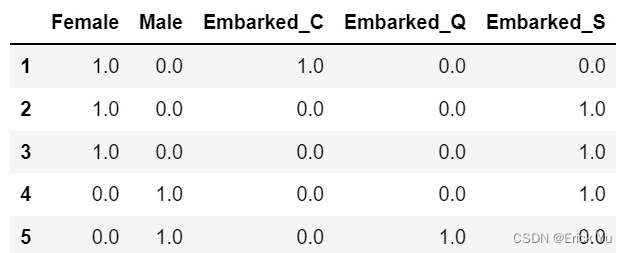
|