每篇前言:
Python面向对象(四)
认真看了前面文章的小伙伴可能会有疑问,我前面不是讲过单例模式了吗?这是不是孤寒者给弄混了。 其实不然,有很多重要的知识点,我都是一遍又一遍的穿插讲解,就是想要引起大家的重视,让大家牢牢记在心中! 所以看这个知识点之前凭借自己的印象,看看能不能自己码出一个单例类!看看自己还能记住多少!
1.1 单例模式
- 确保某一个类只有一个实例,而且自行实例化并向整个系统提供这个实例,这个类称为单例类,单例模式是一种对象创建型模式。
"""
__author__ = 小小明-代码实体
"""
class Singleton(object):
__instance = None
def __new__(cls, age, name):
if not cls.__instance:
cls.__instance = object.__new__(cls)
return cls.__instance
a = Singleton(18, "dongGe")
b = Singleton(8, "dongGe")
print(id(a), id(b))
a.age = 19
print(b.age)
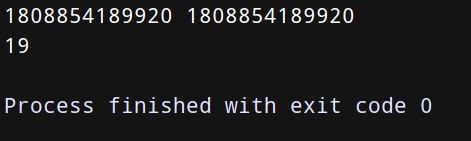
"""
__author__ = 小小明-代码实体
"""
class Singleton(object):
__instance = None
__first_init = False
def __new__(cls, age, name):
if not cls.__instance:
cls.__instance = object.__new__(cls)
return cls.__instance
def __init__(self, age, name):
if not self.__first_init:
self.age = age
self.name = name
Singleton.__first_init = True
def __str__(self):
return "Singleton[%s,%s]" % (self.name, self.age)
a = Singleton(18, "dongGe")
b = Singleton(8, "dongGe")
print(id(a), id(b))
print(a.age, b.age)
print(a, b)

2.1 Enum枚举类
from enum import Enum
Month = Enum('Month', ('Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'))
- 这样我们就获得了Month类型的枚举类,可以直接使用Month.Jan来引用一个常量,或者枚举它的所有成员:
for name, member in Month.__members__.items():
print(name, '=>', member, ',', member.value)
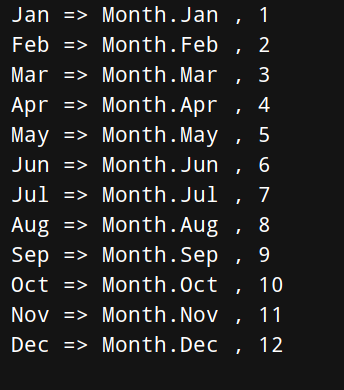
- value属性则是自动赋给成员的int常量,默认从1开始计数。
- 如果需要更精确地控制枚举类型,可以从Enum派生出自定义类:
"""
__author__ = 小小明-代码实体
"""
from enum import Enum, unique
@unique
class Weekday(Enum):
Sun = 0
Mon = 1
Tue = 2
Wed = 3
Thu = 4
Fri = 5
Sat = 6
for name, member in Weekday.__members__.items():
print(name, '=>', member, ',', member.value)
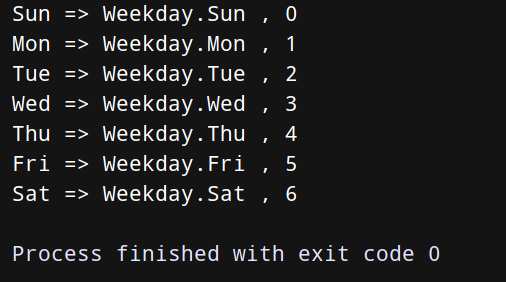
- @unique装饰器可以帮助我们检查保证没有重复值。
- 访问这些枚举类型可以有若干种方法:
Weekday = Enum('Weekday', ("Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"))
for i in range(1,8):
print(i,Weekday(i),Weekday(i).name)
print(Weekday.Tue)
print(Weekday['Tue'])
print(Weekday.Tue.value)
print(Weekday(1))
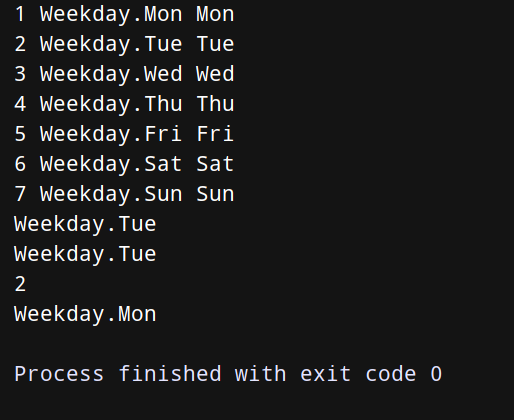
"""
__author__ = 小小明-代码实体
"""
from enum import Enum, unique
@unique
class TrafficLamp(Enum):
RED = 60
GREEN = 45
YELLOW = 5
def next(self):
if self.name == 'RED':
return TrafficLamp.GREEN
elif self.name == 'GREEN':
return TrafficLamp.YELLOW
elif self.name == 'YELLOW':
return TrafficLamp.RED
current = TrafficLamp.RED
for i in range(10):
print(current, current.value)
current = current.next()
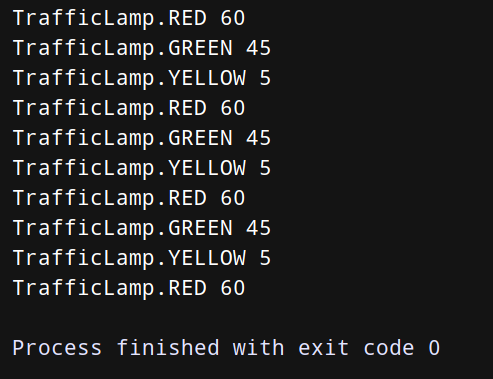
|