首先明白一个知识点。 Python代码用的是解释器,c语言代码用的是编译器,java解释器编译器都可以。
标准库和模块
- 像python的开发者,会在python安装包中直接集成一些功能模块,使用他们的时候使用者不需要进行import导入便可直接使用,例如(数字,字符串,列表,元组等)都可以在编写的时候直接使用。
- 而随python安装程序在lib文件夹里面一般会自带一些标准库和模块,他们需要使用时得先import导入才可使用。例如:时间(time),随机数(random),正则(re)等,需要import 包名 才可以使用
- 第三方公司开发的库和模块,使用前需要下载和安装,安装完成会出现在lib文件夹的子文件包里面,在正常import调用即可。例如:pygame,matplotlib
- 自己写的功能和模块,也可以通过import进行调用。
库?模块?包?
介绍一下包和模块吧。大致就可以比作,我们写过的程序都可以被另一个程序调用,他在被调用的时候,对于调用他的程序来说他就是模块,放他的文件夹就是包。不管是模块还是包,我们都可以把他叫做某某库。其实就是工程文件之间的调用。例如:a程序调用b程序的一些功能,对于a而言,b程序就是模块,而b程序的文件夹,就是包。
操作对象
元组:(对象1,对象2,对象3,对象4,对象5) 列表:[对象1,对象2,对象3,对象4,对象5] 集合:{对象1,对象2,对象3,对象4,对象5} 字典:{k1:v1,K2:v2,k3:v3,k4:v4,k5:v5} 这里只举例了装数据的形式,其中元组是不可变得,比较死板,下标从零开始;2?列表相比于元组更加的灵活,基本可以添加任何形式能被定义的数据,下标也是从零开始。但是集合里的对象不可重复,而且没有序号,顺序是随机的。字典的话就是key:value的形式。
举例
包导入举例:
- 内部功能模块
arr = [1, 2, 3, 4, 5, 6, 7, 8]
print(arr)
可以看到,定义一个列表不用进行导包,就可以在程序中使用
- python自带模块
import time
print(time.time())
这里,import time指导入了import包 time.time()调用time()方法 然后控制台进行了输出
- 外部第三方模块
import pygame
import sys
import personModule
pygame.init()
screen_image = pygame.display.set_mode((800, 600))
screen_rect = screen_image.get_rect()
pygame.display.set_caption("invasion")
ship_image = pygame.image.load("src/lanlan.bmp")
image = pygame.transform.scale(ship_image, (80, 80))
ship_rect = image.get_rect()
ship_rect.center = screen_rect.center
bullet_rect = pygame.Rect(0, 0, 3, 15)
bullet_rect.midbottom = ship_rect.midtop
txt_font = pygame.font.SysFont(None, 48)
txt_image = txt_font.render("lanlan", True, personModule.bg_color_two, personModule.bg_color_three)
txt_rect = txt_image.get_rect()
txt_rect.x = 740
txt_rect.y = 20
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.type == pygame.K_LEFT:
ship_rect.x -= 10
if event.type == pygame.K_RIGHT:
ship_rect.x += 10
if event.type == pygame.K_UP:
ship_rect.y -= 10
if event.type == pygame.K_DOWN:
ship_rect.y += 10
else:
ship_rect.y += 10
bullet_rect.y -= 1
screen_image.fill(personModule.bg_color_one)
screen_image.blit(image, ship_rect)
pygame.draw.rect(screen_image, personModule.bg_color_two, bullet_rect)
screen_image.blit(txt_image, txt_rect)
pygame.display.flip()
这里举例了一个pygame库写的使图像上下左右移动的例子: 使用前请调用下面一条的模块 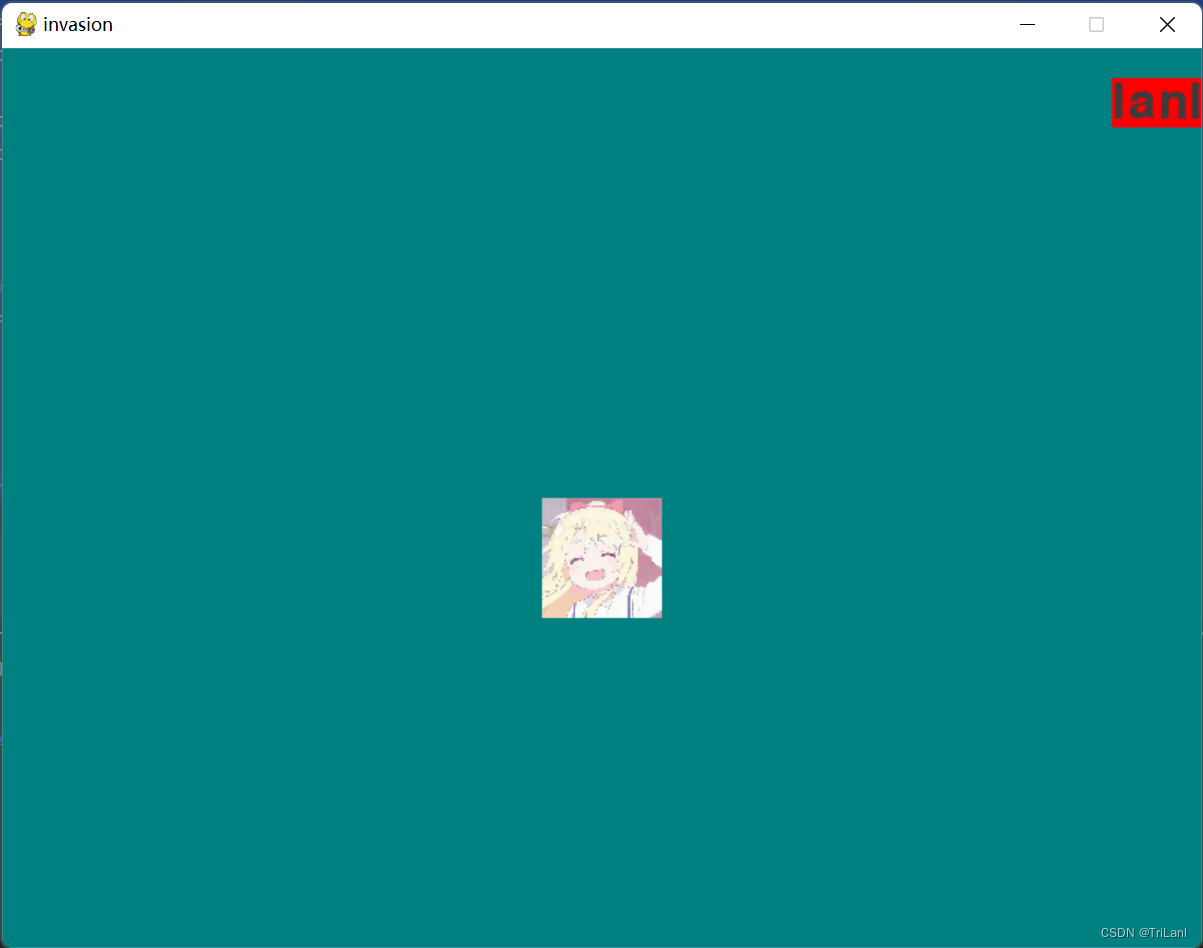 像这样调用第三方模块import pygame 的就是用外部模块。下载完后直接使用。
- 个人书写的模块
bg_color_one = (0, 128, 128)
bg_color_two = (60, 60, 60)
bg_color_three = (255, 0, 0)
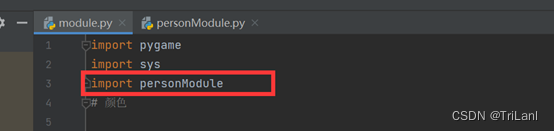 调用完成以后,使用模块名进行调用 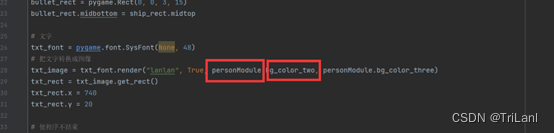
|