一、字符串
·字符串就是一串字符,是编程语言中表示文本的数据类型
·在Python 中可以使用一对双引号”??或者一对’?单引号定义一个字符串
???虽然可以使有\”?或者\’?做字符串的转义,但是在实际开发中:
如果字符串内部需要使用”??可以使用’?定义字符串
如果字符串内部需要使用’??可以使用”?定义字符串
·可以使用 索引 获取一个字符串中 指定位置的字符,索引数从0开始计数
·也可以使用for循环遍历 字符串中每一个字符
(注:大多数编程语言中 都是用”?来定义字符串的)
1.1字符串取索引操作如下:
(注:不论是之前学的列表,元组,字典,还是字符串,取索引 后面都是用[ ])
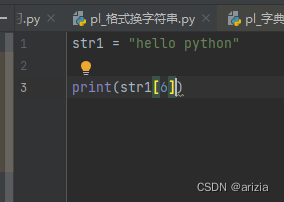
代码:
str1 = "hello python"
print(str1[6])
?输出结果:
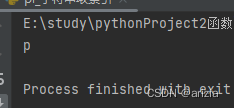
?(注:在Python 中,空格也是要算一个 索引的)
1.2 字符串的遍历
操作如下:
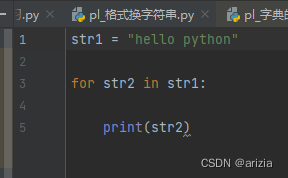
?代码:
str1 = "hello python"
for str2 in str1:
print(str2)
输出结果:
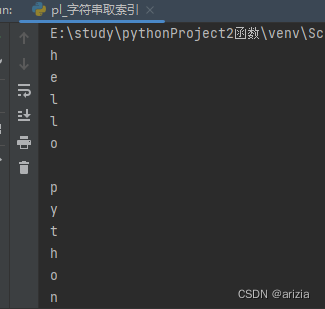
?1.3 字符串的常用操作:
① len ?获取字符串的长度
操作:
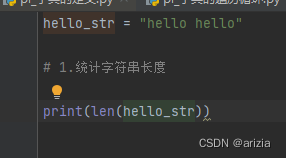
代码:?
hello_str = "hello hello"
print(len(hello_str))
输出结果:
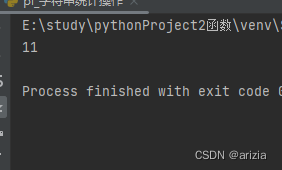
?(注:一定要算上空格键)
② count ?统计大字符串中,某一个小字符串出现的次数
操作:
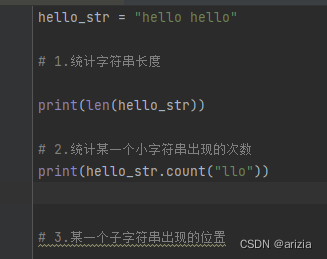
?代码:
hello_str = "hello hello"
print(hello_str.count("llo"))
输出结果:

?
③ index ?获取小字符串 第一次出现的索引
操作:
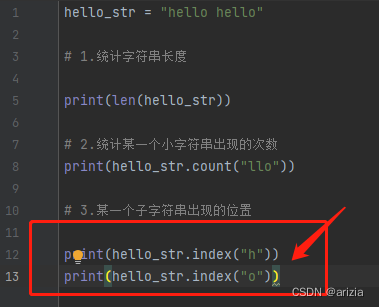
?代码:
hello_str = "hello hello"
# 3.某一个子字符串出现的位置
print(hello_str.index("h"))
print(hello_str.index("o"))
输出结果:

?做了两个子字符串的 代码, h 最开始出现位置是 0 、 o最开始出现的位置是4
1.4 在Python 中 字符串 的 使用方法总结以及归类:
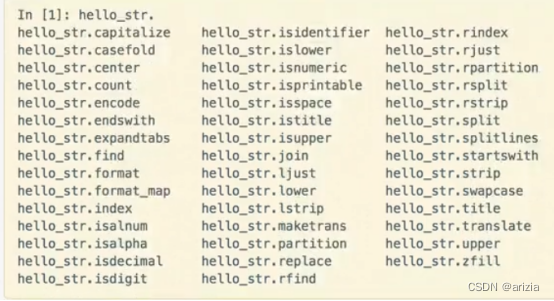
?在Python 中 使用字符串的 方法 是比较多的,下面进行一下归类:
1.4-1
判断类型:凡是以 ?is ?开头的 ?都是表示判断类型的
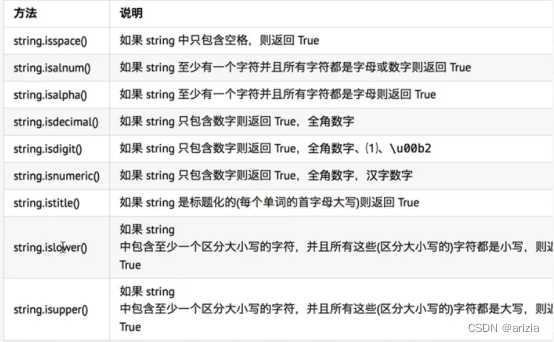
?下面是演练:
演练1:isspace
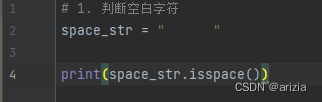
?代码:
space_str = " "
print(space_str.isspace())
输出结果:

?(注:如果有其他字符,返还结果则是 false)
演练二、判断数字(这个里面有三种判断方法,光看不好理解,所以做一下实际演练)
下面直接三种类型,做一起的演练,方便比较
①全是数字:(注:这三种方法都是不能判断小数的)
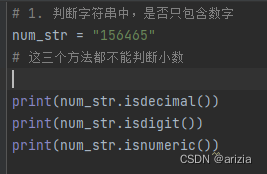
?代码:
num_str = "156465"
print(num_str.isdecimal())
print(num_str.isdigit())
print(num_str.isnumeric())
输出结果:
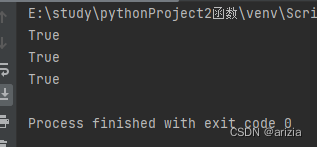
?结论:三种方法都是可以判断纯数字的(注:在我们开发中,最常用的还是第一种,判断纯阿拉伯数字比较常用)
②特殊数字:“①”这个数字是表示我们平时在键盘中无法直接输入,但是可以通过其他的输入法或者特殊方式输入的字符串 ?一般统称为 unicode 字符串
操作:
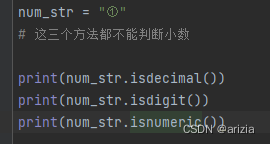
?代码:
num_str = "①"
print(num_str.isdecimal())
print(num_str.isdigit())
print(num_str.isnumeric())
输出结果:
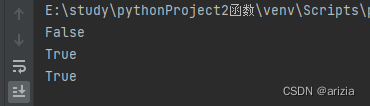
?结论:str.isdigit、str.isnumeric 可以判断特殊字符串
③中文数字:
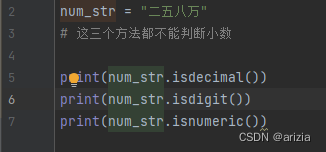
?代码:
num_str = "二五八万"
print(num_str.isdecimal())
print(num_str.isdigit())
print(num_str.isnumeric())
输出结果:

结论:str.isnumeric,不仅可以判断特殊字符还可以判断中文数字?
1.4.2 查找和替换类型:
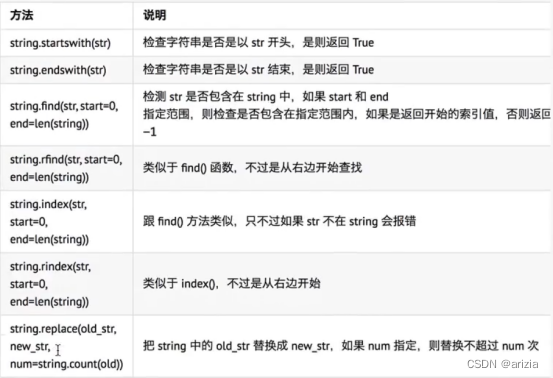
?
下面是演练:

?代码:
hello_str = "hello world"
print(hello_str.startswith("hello"))
输出结果:

?
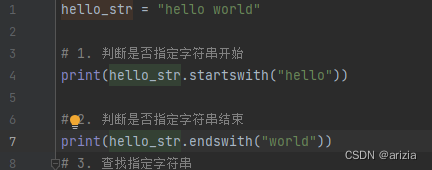
?代码:
hello_str = "hello world"
print(hello_str.endswith("world"))
输出结果:

?
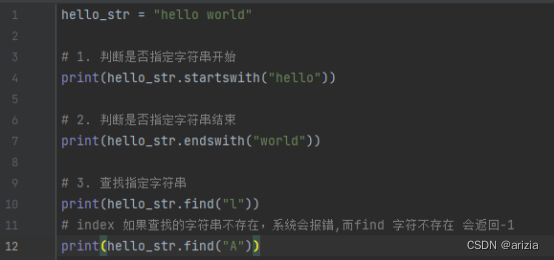
?代码:
hello_str = "hello world"
print(hello_str.find("l"))
print(hello_str.find("A"))
输出结果:

?注: index 如果查找的字符串不存在,系统会报错,而find 查找字符不存在 会返回-1
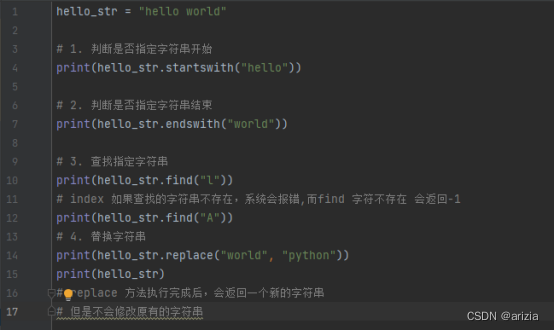
?代码:
hello_str = "hello world"
print(hello_str.replace("world", "python"))
print(hello_str)
输出结果:

(注:#replace 方法执行完成后,会返回一个新的字符串,但是不会修改原有的字符串,所以输出结果会不一样)?
1.4.3?大小写转换类型:
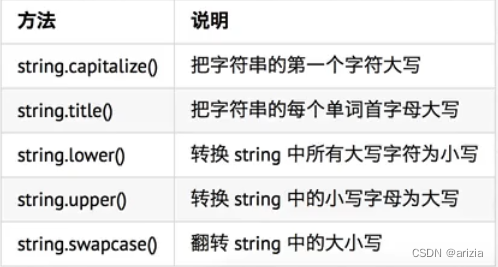
?
1.4.4?文本对其类型:
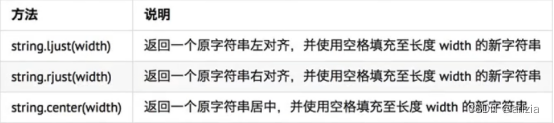
?下面演练的是居中:(其他类型都是类似的)
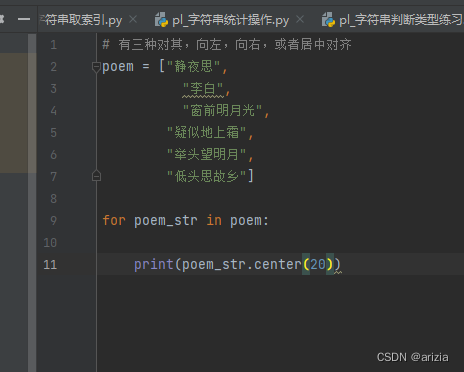
?代码:
poem = ["静夜思",
"李白",
"窗前明月光",
"疑似地上霜",
"举头望明月",
"低头思故乡"]
for poem_str in poem:
print(poem_str.center(20))
(注:poem_str.center(20) 这个括号里面的20 表示宽度,要填写,要不然系统会报错)
输出结果:
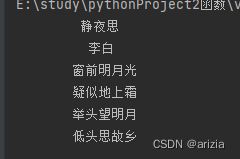
?
1.4.5?去除空白字符类型:
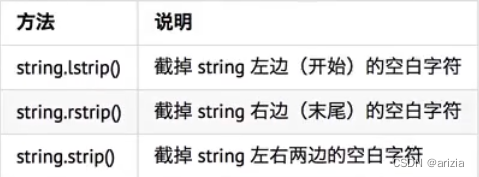
练习:
这个是没有做去除空白的代码和输出结果:
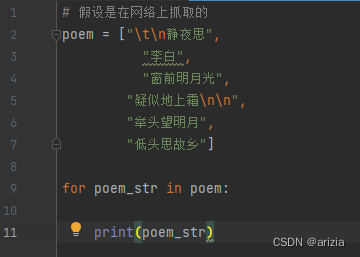
?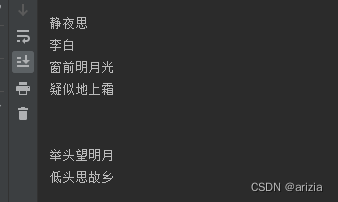
?显示很乱
?下面是做去除空白的演练:
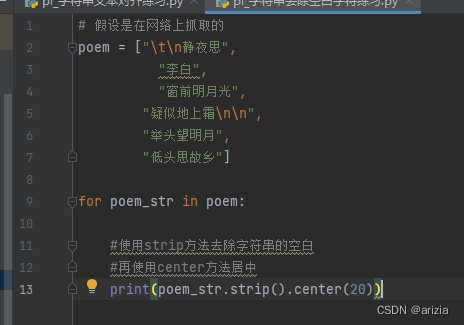
?代码:
poem = ["\t\n静夜思",
"李白",
"窗前明月光",
"疑似地上霜\n\n",
"举头望明月",
"低头思故乡"]
for poem_str in poem:
#使用strip方法去除字符串的空白
#再使用center方法居中
print(poem_str.strip().center(20))
输出结果:
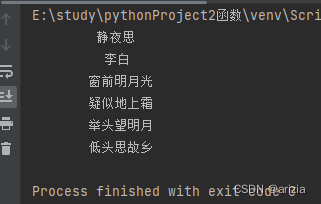
?(注:上面是把去空白和居中对齐一起操作的)
1.4.6?拆分和连接类型:
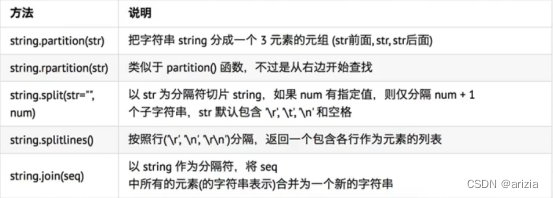
?演练:
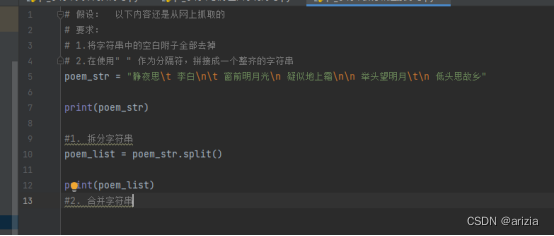
代码:?
poem_str = "静夜思\t 李白\n\t 窗前明月光\n 疑似地上霜\n\n 举头望明月\t\n 低头思故乡"
print(poem_str)
poem_list = poem_str.split()
print(poem_list)
输出结果:
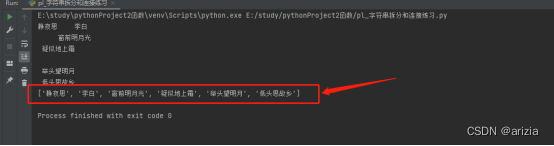
?
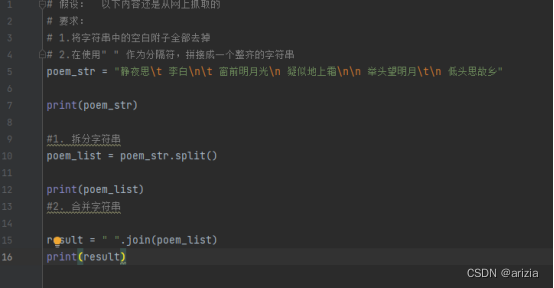
?代码:
poem_str = "静夜思\t 李白\n\t 窗前明月光\n 疑似地上霜\n\n 举头望明月\t\n 低头思故乡"
print(poem_str)
poem_list = poem_str.split()
print(poem_list)
result = " ".join(poem_list)
print(result)
输出结果:
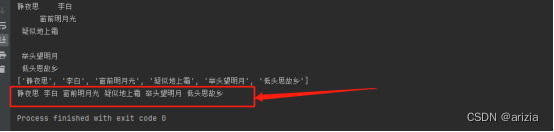
?
1.5 字符串的切片
·切片 方法适用于 字符串、列表、元组
???切片 使用 索引值 来限定范围,从一个大的 字符串 中 切出 小的字符串
???列表 和 元组 都是 有序的 集合,都能够通过索引值 获取到相对应的数据
??字典 是一个无序的集合,使用舰队之 保存数据的
(注:切片时,是不包含结束时,索引值的字符的,切片分为两种 1.顺序:从左往右从 0 开始 2.倒序:一般是针对比较长的字符串 从右往左 从-1 开始)
下面是在 Python 中的操作:
字符串为:0123456798

?(注:要求是2-5?位置 所以结束位置应该就是在6)

?

?

?

?

?

?

?

?

?
总结
今天学习的 字符串 用法比较多,但是也不需要死记硬背,归类了几个大类,每一个大类里面都分的很清楚的。平时用的时候 pycharm 里面 点字符串用法 再按ctrl + Q 是可以看见提示的
|