一、Django基础知识
1、安装django
pip install django
python目录
- python.exe
- scripts
- pip.exe
- django-admin.exe
- lib
- 内置模块
- site-packages
- openpyxl
- python-docx
- flask
- django
2、创建项目
django中项目会有一些默认的文件和默认的文件夹
2.1:打开终端
django-admin.exe startproject testone
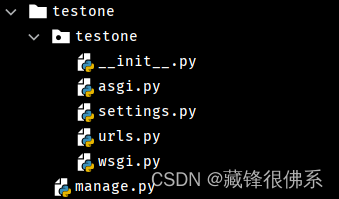
3、项目文件介绍
mysite
- manage.py
- mysite
- __init__.py
- asgi.py
- settings.py
- urls.py
- wsgi.py
4、APP
- 项目
- app,用户管理【表结构、函数、HTML模板、CSS】
- app,订单管理
- app,后台管理
- app,网站
- app,API
- ……
4.1 安装app
python3.9 manage.py startapp [app's name]
4.2 app目录文件说明
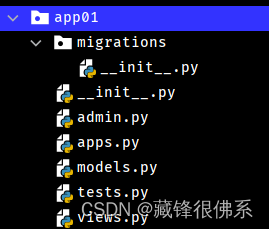
- app01
|- migrations
|- __init__.py
|- __init__.py
|- admin.py
|- apps.py
|- models.py
|- tests.py
|- views.py
5、快速上手django
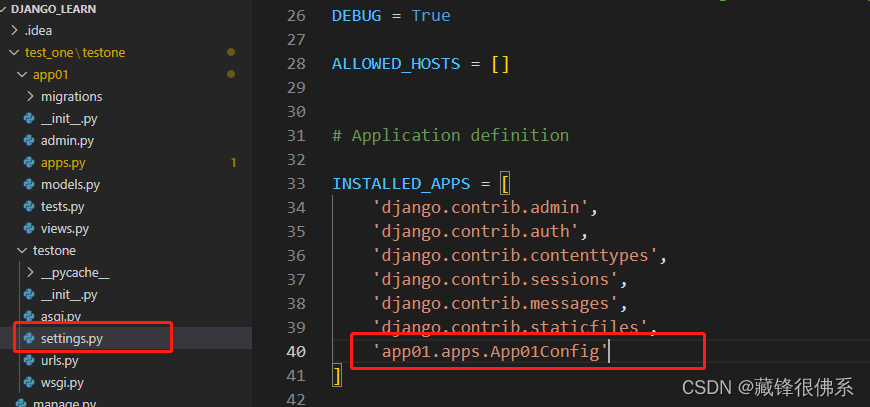
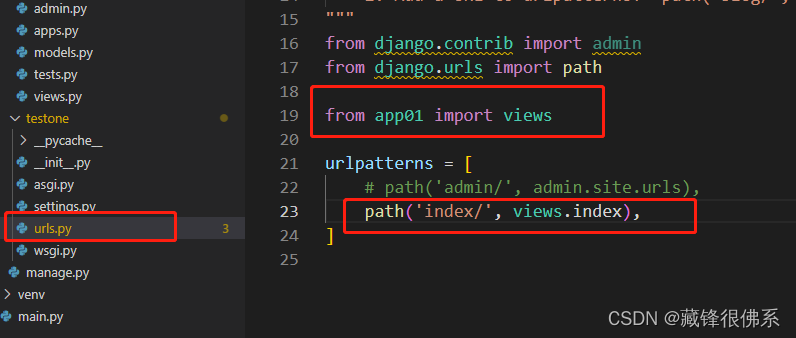
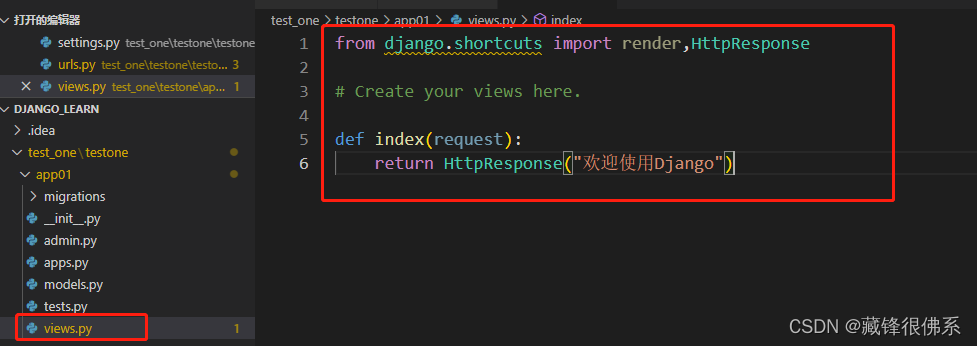
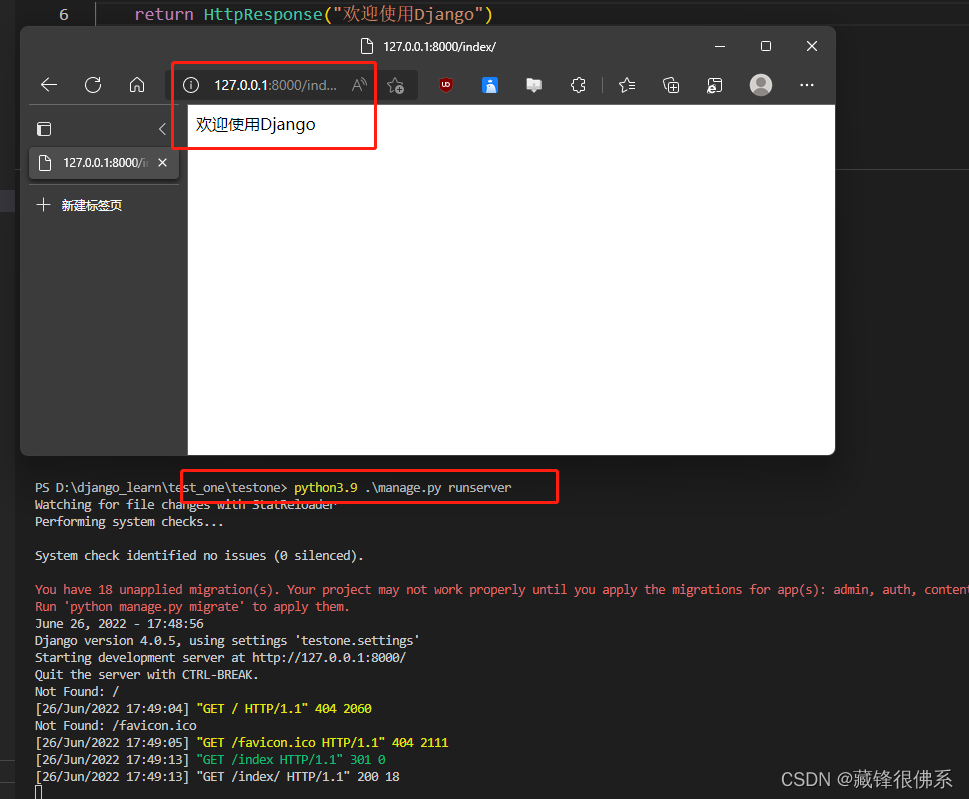
5.1 再写一个页面
```python
- url -> 函数
- 函数
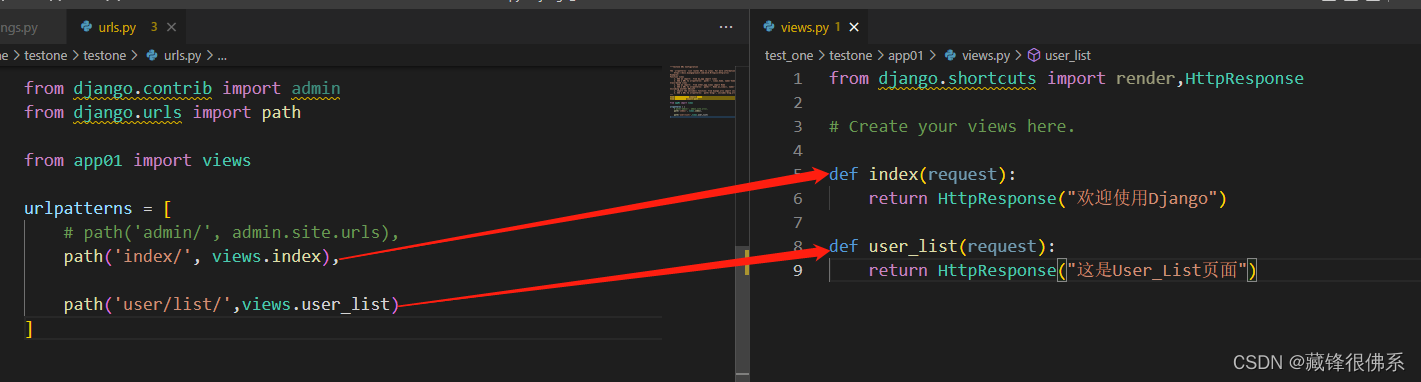
5.2 templates模板
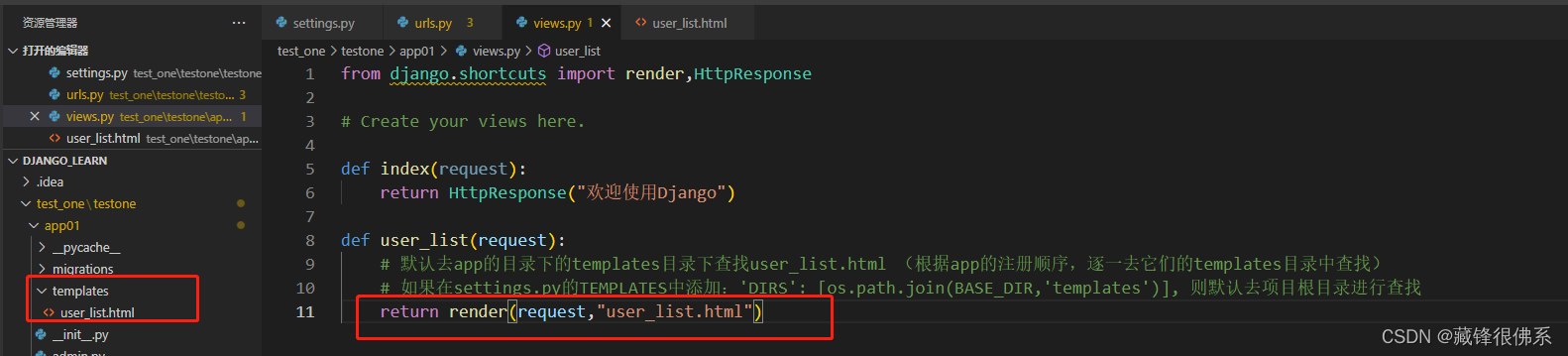
5.3 静态文件
包括:图片,css,js
5.3.1 static目录
- 在app目录下创建static文件夹
- 在html中 load static 文件夹
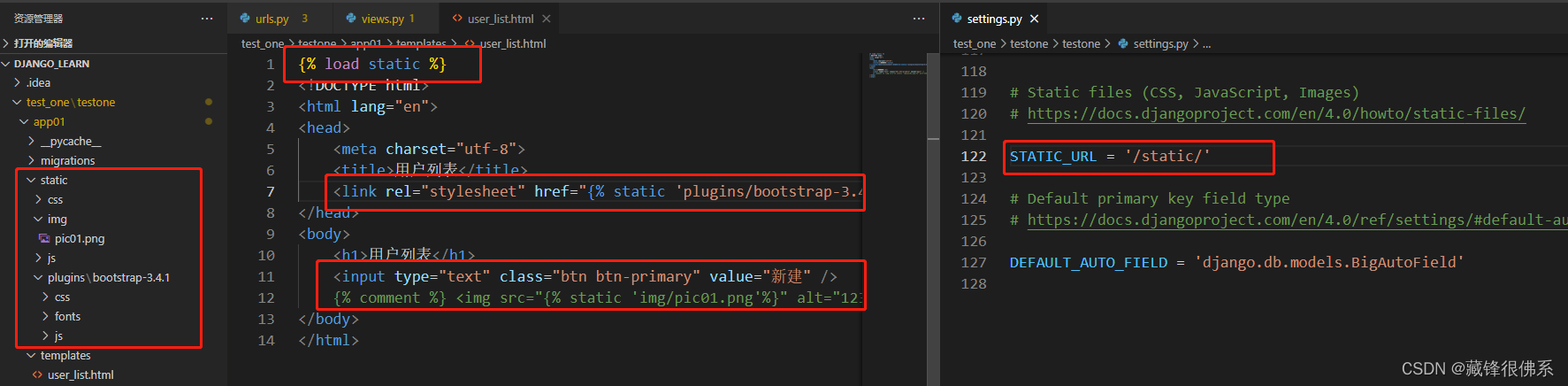
{% load static %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>用户列表</title>
<link rel="stylesheet" href="{% static 'plugins/bootstrap-3.4.1/css/bootstrap.css' %}">
</head>
<body>
<h1>用户列表</h1>
<input type="text" class="btn btn-primary" value="新建" />
{% comment %} <img src="{% static 'img/pic01.png'%}" alt="123"> {% endcomment %}
</body>
</html>
```
```python
# settings.py
# Internationalization
# https://docs.djangoproject.com/en/4.0/topics/i18n/
LANGUAGE_CODE = 'en-us'
TIME_ZONE = 'UTC'
USE_I18N = True
USE_TZ = True
# Static files (CSS, JavaScript, Images)
# https://docs.djangoproject.com/en/4.0/howto/static-files/
STATIC_URL = '/static/'
# Default primary key field type
# https://docs.djangoproject.com/en/4.0/ref/settings/#default-auto-field
DEFAULT_AUTO_FIELD = 'django.db.models.BigAutoField'
```
6、模板语法
本质上:在HTML中写一些占位符,由数据对这些占位符进行替换和处理
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>TPL</title>
</head>
<body>
<h1>模板语法学习</h1>
<div>{{ n1 }}</div>
<div>{{ n2.0 }}</div>
<div>{{ n2.1 }}</div>
<div>{{ n2.2 }}</div>
<div>
{% for item in n2 %}
<span>{{item}}</span>
{% endfor %}
</div>
<hr/>
<ul>
{% for k,v in n3.items %}
<li>{{k}}:{{v}}</li>
{% endfor %}
</ul>
<hr/>
{{n4}}
{% for item in n4%}
<div>{{item.name}} {{item.salary}}</div>
{% endfor %}
{% comment %} {{n5}}
{% for item in n5 %}
<span>{{item.fund_name}}:{{item.fund_type}},{{item.fund_rlevel}},{{item.syl_1n}}</span>
{% endfor %} {% endcomment %}
<hr/>
{% if n1 == 'Hello Django' %}
<h1>233333</h1>
{% else %}
<h1>344444</h1>
{% endif %}
</body>
</html>
```
7、请求和响应
def something(req):
print(req.method)
print(req.GET)
print(req.POST)
return redirect("https://www.baidu.com")
|