🍰 个人主页:__Aurora__ 🍞如果文章有什么需要改进的地方还请各位大佬指正。 🍉如果我的文章对你有帮助?? 关注🙏🏻 点赞👍 收藏??

一、python自定义异常
1.自定义一个CustomException类,继承Exception 类  2.编写CustomException类 输入一个大于0小于100的正整数数,否则报异常
class CustomException(Exception):
min_e=0;
max_e=100;
def __init__(self,e,*args):
super().__init__(args)
self.e=e
def __str__(self):
return f"This {self.e} does not meet the regulations"
3.编写触发异常的方法 当输入的正整数 大于100或者小于0 触发 CustomException异常
def customException_to(numberInput):
if numberInput>CustomException.max_e or numberInput<CustomException.min_e:
raise CustomException(numberInput)
最终代码
class CustomException(Exception):
min_e=0;
max_e=100;
def __init__(self,e,*args):
super().__init__(args)
self.e=e
def __str__(self):
return f"This {self.e} does not meet the regulations"
def customException_to(numberInput):
if numberInput>CustomException.max_e or numberInput<CustomException.min_e:
raise CustomException(numberInput)
if __name__ == '__main__':
numberInput = input('Enter a temperature in Fahrenheit:')
try:
numberInput=int(numberInput)
try:
customException_to(numberInput)
except CustomException as e:
print(e)
except ValueError as e:
print(e)
测试结果 
二、python自定义异常分析
1、字符串前的f
格式化 {} 内容,不在 {} 内的照常展示输出,如果你想输出 {},那就用双层 {{}} 将想输出的内容包起来。
lists = [1,2]
print(lists, f'lists length {len(lists)}.')
print(lists, f'lists length {{len(lists)}}.')
print(lists, f'has a length of {{{len(lists)}}}.')
dics ={"name":"张三","age":18}
print(f'name of value {dics.get("name")}.')
2、 __init__(self,e,*args):
__init__ 方法接收参数e(可定义不同类型的多个形参)作为CustomException 的属性
3、__str__ 属性
当触发异常时,需要异常信息。__str__ 方法返回自定义字符串
4、customException_to方法
customException_to 方法的目的是判断达到报错条件,如果达到条件则通关键字raise 触发CustomException
输入999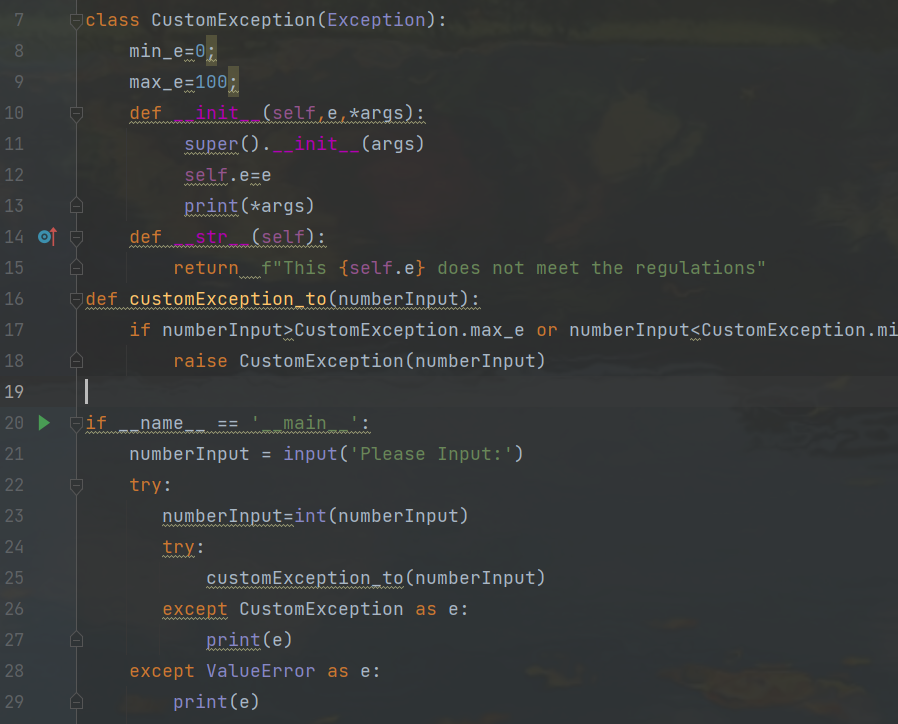 21行 接收999 23行 合法字符,整数 25行 执行customException_to 方法 16-18行 判断输入numberInput ,大于100则 触发CustomException 异常 10-13行 初始化CustomException 异常类 26行 捕捉异常,准备打印异常信息 14-15行获取异常信息 27行打印 
参考:https://www.pythontutorial.net/python-oop/python-custom-exception/
|