1. 方法补充
1.1 私有方法
class Person:
__age = 18
def __run(self):
print("pao")
p = Person()
print(Person.__dict__)
p.__run()
执行结果: 私有化方法后,方法名称同私有化属性进行了自动变换 
类内部定义了一个 __run 的私有方法,自动从命名了 _Person__run ,然后内部又重写了 _Person__run 方法,从而实现了将 __run 进行覆盖
class Person:
__age = 18
def __run(self):
print("pao")
def _Person__run(self):
print("xxx")
p = Person()
p._Person__run()
执行结果: 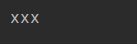
2. 其他内置方法
2.1 __str__ 与 str() 信息格式化
从写 __str__ ,当打印 p1,就可以实现自己想要的逻辑
class Person:
def __init__(self, n, a):
self.name = n
self.age = a
def __str__(self):
return "这个人的姓名是%s, 这个人的年龄是:%s" % (self.name, self.age)
p1 = Person("sz", 18)
print(p1)
执行结果:  str():可以用于触发 __str__ 执行
class Person:
def __init__(self, n, a):
self.name = n
self.age = a
def __str__(self):
return "这个人的姓名是%s, 这个人的年龄是:%s" % (self.name, self.age)
p1 = Person("sz", 18)
s = str(p1)
print(s, type(s))
执行结果: 
2.2 repr() 与
class Person:
def __init__(self, n, a):
self.name = n
self.age = a
def __str__(self):
return "这个人的姓名是%s, 这个人的年龄是:%s" % (self.name, self.age)
p1 = Person("sz", 18)
s = str(p1)
print(s, type(s))
print(repr(p1))
执行结果:  当类内部实现 __repr__ 方法后,此时使用 repr() 方法就会的到重现实现后的结果
class Person:
def __init__(self, n, a):
self.name = n
self.age = a
def __str__(self):
return "这个人的姓名是%s, 这个人的年龄是:%s" % (self.name, self.age)
def __repr__(self):
return "reprxxxxx"
p1 = Person("sz", 18)
s = str(p1)
print(s, type(s))
print(repr(p1))
执行结果: 
class Person:
def __init__(self, n, a):
self.name = n
self.age = a
def __repr__(self):
return "reprxxxxx"
p1 = Person("sz", 18)
print(p1)
s = str(p1)
print(s, type(s))
print(repr(p1))
执行结果: 当内部没有实现 __str__ 方法时,就会去找 __repr__ 方法 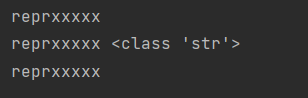
2.3 __call__方法
__call__ :可以让实例化的对象可以直接被调用
class Person:
def __call__(self, *args, **kwargs):
print("xxx", args, kwargs)
pass
p = Person()
p(123, 456, name="sz")
执行结果:加粗样式 
2.4 __call__ 方法的运用
创建很多个画笔, 画笔的类型(钢笔, 铅笔), 画笔的颜色(红, 黄色, 青色, 绿色)
print("创建了一个%s这个类型的画笔, 它是%s颜色" % ("钢笔", "红色"))
print("创建了一个%s这个类型的画笔, 它是%s颜色" % ("钢笔", "黄色"))
print("创建了一个%s这个类型的画笔, 它是%s颜色" % ("钢笔", "青色"))
print("创建了一个%s这个类型的画笔, 它是%s颜色" % ("钢笔", "绿色"))
print("创建了一个%s这个类型的画笔, 它是%s颜色" % ("钢笔", "橘色"))
执行结果: 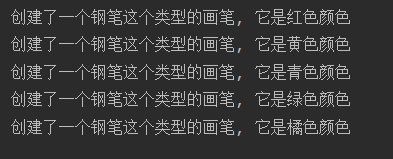
def createPen(p_color, p_type):
print("创建了一个%s这个类型的画笔, 它是%s颜色" % (p_type, p_color))
createPen("钢笔", "红色")
createPen("钢笔", "绿色")
createPen("钢笔", "黄色")
执行结果: 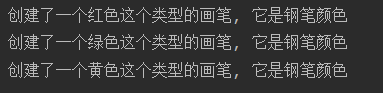
def createPen(p_color, p_type):
print("创建了一个%s这个类型的画笔, 它是%s颜色" % (p_type, p_color))
createPen("钢笔", "红色")
createPen("钢笔", "绿色")
createPen("钢笔", "黄色")
import functools
gangbiFunc = functools.partial(createPen, p_type="钢笔")
gangbiFunc("红色")
gangbiFunc("黄色")
gangbiFunc("绿色")
执行结果: 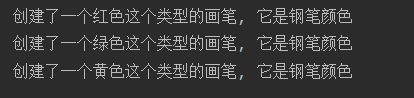
class PenFactory:
def __init__(self, p_type):
self.p_type = p_type
def __call__(self, p_color):
print("创建了一个%s这个类型的画笔, 它是%s颜色" % (self.p_type, p_color))
gangbiF = PenFactory("钢笔")
gangbiF("红色")
gangbiF("绿色")
gangbiF("黄色")
qianbiF = PenFactory("铅笔")
qianbiF("红色")
qianbiF("绿色")
qianbiF("黄色")
执行结果: 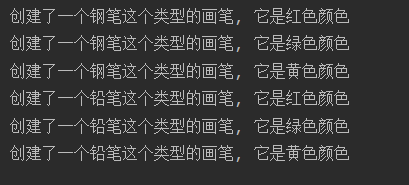
2.5 索引操作:__setitem__ 、__getitem__ 、__delitem__
class Person:
def __init__(self):
self.cache = {}
def __setitem__(self, key, value):
print("setitem", key, value)
self.cache[key] = value
def __getitem__(self, item):
print("getitem", item)
return self.cache[item]
def __delitem__(self, key):
print("delitem", key)
del self.cache[key]
p = Person()
p["name"] = "sz"
print(p["name"])
del p["name"]
print(p.cache)
执行流程: 执行 p["name"] = "sz" 时会自动调用 __setitem__ ,当执行 p["name"] 时,会自动调用 __getitem__ ,当执行 del p["name"] ,会自动调用 __delitem__ 执行结果: 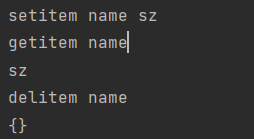
2.6 切片操作:__setitem__ 、__getitem__ 、__delitem__
l = [1, 2, 3, 4, 5]
print(l[1: 4: 2])
执行结果: 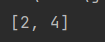
class Person:
def __init__(self):
self.items = [1, 2, 3, 4, 5, 6, 7, 8]
def __setitem__(self, key, value):
print(key, value)
print(key.start)
print(key.stop)
print(key.step)
print(value)
p = Person()
p[0: 4: 2] = ["a", "b"]
执行结果: 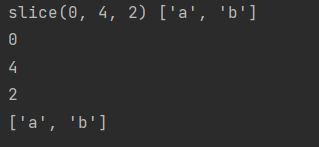
class Person:
def __init__(self):
self.items = [1, 2, 3, 4, 5, 6, 7, 8]
def __getitem__(self, item):
print("getitem", item)
p = Person()
p[0: 5: 2]
执行结果: 
class Person:
def __init__(self):
self.items = [1, 2, 3, 4, 5, 6, 7, 8]
def __setitem__(self, key, value):
if isinstance(key, slice):
self.items[key.start: key.stop: key.step] = value
def __getitem__(self, item):
print("getitem", item)
def __delitem__(self, key):
print("delitem", key)
p = Person()
p[0: 4: 2] = ["a", "b"]
print(p.items)
p[0: 5: 2]
del p[0: 5: 2]
执行结果: 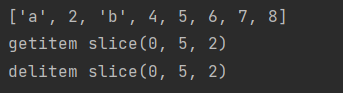
2.7 比较操作
class Person:
def __init__(self, age, height):
self.age = age
self.height = height
def __eq__(self, other):
print("eq")
return self.age == other.age
def __ne__(self, other):
pass
def __gt__(self, other):
pass
def __ge__(self, other):
pass
def __lt__(self, other):
return self.age < other.age
def __le__(self, other):
pass
p1 = Person(18, 190)
p2 = Person(19, 190)
print(p1 < p2)
print(p1 <= p2)
print(p1 == p2)
print(p1 != p2)
print(p1 <= p2)
执行结果: 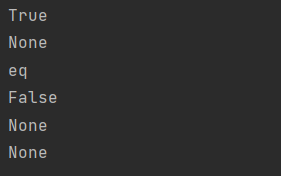
2.8 上下文环境的布尔值 :__bool__
class Person:
def __init__(self):
self.age = 20
def __bool__(self):
return self.age >= 18
pass
p = Person()
if p:
print("xx")
执行结果: 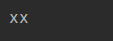
2.9 遍历操作 __iter__ 、__next__
下面的两种方法均可让对象变为一个迭代器
方式一
class Person:
def __init__(self):
self.result = 1
def __getitem__(self, item):
self.result += 1
if self.result >= 6:
raise StopIteration("停止遍历")
return self.result
pass
p = Person()
for i in p:
print(i)
执行结果: 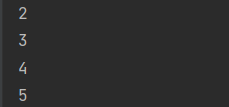
方法二
class Person:
def __init__(self):
self.result = 1
def __getitem__(self, item):
self.result += 1
if self.result >= 6:
raise StopIteration("停止遍历")
def __iter__(self):
print("iter")
self.result = 1
return self
执行结果: __iter__ 高于 __getitem__ 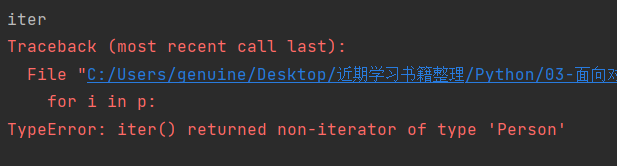 要使 一个实例能够通过 iter() 返回一个生成器,首先需要在对应的类里实现 __iter__ 方法,并返回,self ,并实现能够迭代的 __next__ 方法,缺一不可,如下:
class Person:
def __init__(self):
self.result = 1
def __iter__(self):
print("iter")
return self
执行结果: 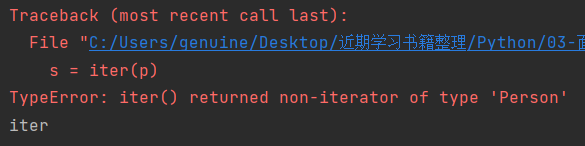
class Person:
def __init__(self):
self.result = 1
def __iter__(self):
print("iter")
return self
def __next__(self):
print(".....")
self.result += 1
if self.result >= 6:
raise StopIteration("停止遍历")
return self.result
p = Person()
s = iter(p)
print(next(p))
print(next(p))
print(next(p))
执行结果: 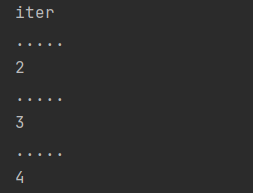
恢复迭代器初始值
- Python 迭代器 ,在这篇博客中,我介绍了迭代器只能打印一次,那么如何恢复迭代器,让其可以打印多次呢?
__iter__ 内实现了一个 self.age = 1,重置,从而下一次可以继续使用
用于判断一个对象是否为迭代器
import collections
print(isinstance(p, collections.Iterator))
print(isinstance(p, collections.Iterable))
class Person:
def __init__(self):
self.age = 1
def __getitem__(self, item):
return 1
def __iter__(self):
self.age = 1
return self
def __next__(self):
self.age += 1
if self.age >= 6:
raise StopIteration("stop")
return self.age
p = Person()
for i in p:
print(i)
print(p.age)
for i in p:
print(i)
import collections
print(isinstance(p, collections.Iterator))
print(isinstance(p, collections.Iterable))
执行结果: 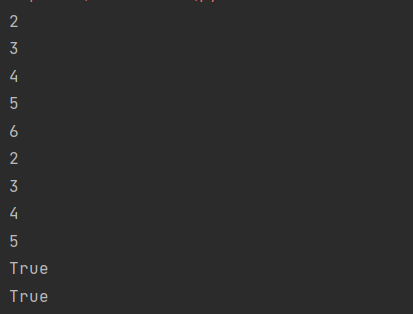
iter 函数的使用
class Person:
def __init__(self):
self.age = 1
def __iter__(self):
self.age = 1
return self
def __call__(self, *args, **kwargs):
self.age += 1
if self.age >= 6:
raise StopIteration("stop")
return self.age
p = Person()
pt = iter(p, 4)
for i in pt:
print(i)
执行结果: 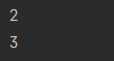
|