Mac M1环境安装参考文章
环境安装成功测试代码
import torch
import math
dtype = torch.float
device = torch.device("mps")
x = torch.linspace(-math.pi, math.pi, 2000, device=device, dtype=dtype)
y = torch.sin(x)
a = torch.randn((), device=device, dtype=dtype)
b = torch.randn((), device=device, dtype=dtype)
c = torch.randn((), device=device, dtype=dtype)
d = torch.randn((), device=device, dtype=dtype)
learning_rate = 1e-6
for t in range(2000):
y_pred = a + b * x + c * x ** 2 + d * x ** 3
loss = (y_pred - y).pow(2).sum().item()
if t % 100 == 99:
print(t, loss)
grad_y_pred = 2.0 * (y_pred - y)
grad_a = grad_y_pred.sum()
grad_b = (grad_y_pred * x).sum()
grad_c = (grad_y_pred * x ** 2).sum()
grad_d = (grad_y_pred * x ** 3).sum()
a -= learning_rate * grad_a
b -= learning_rate * grad_b
c -= learning_rate * grad_c
d -= learning_rate * grad_d
print(f'Result: y = {a.item()} + {b.item()} x + {c.item()} x^2 + {d.item()} x^3')
相关解读见下面几个点
关于MPS
苹果有自己的一套GPU实现API Metal. 而Pvtorch此次的加速就是基于Metal. 具体来说,使用苹果 的Metal Performance Shaders (MPS) 作为PyTorch的后端,可以实现加速GPU训练。MPS后端 扩展了PyTorch框架,提供了在Mac上设置和运行操作的脚本和功能。MPS通过针对每个Metal GpU 系列的独特特性进行微调的内核来优化计算性能。新设备在MPS图形框架和MPS提供的调整内核上 映射机器学习计算图形和基元。
PyTorch中linspace的详细用法
“linspace”是“linear space”的缩写,中文含义为“线性等分向量”、“线性平分矢量”、“线性平分向量”。
linspace()函数详细参数为:
函数的作用是: 返回一个一维的tensor(张量),这个张量包含了从start到end(包括端点)的等距的steps个数据点
torch.linspace(start, end, steps=100, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor
常用的几个参数含义:
start:开始值;
end:结束值;
steps:分割的点数,默认是100;
dtype:返回值(张量)的数据类型
函数的作用是: 返回一个一维的tensor(张量),这个张量包含了从start到end(包括端点)的等距的steps个数据点
示例:
import torch
print(torch.linspace(3,10,5))
#输出: tensor([ 3.0000, 4.7500, 6.5000, 8.2500, 10.0000])
type=torch.float
print(torch.linspace(-10,10,steps=6,dtype=type))
#输出: tensor([-10., -6., -2., 2., 6., 10.])
torch.linspace(-10, 10, steps=21,dtype=type)
#输出: tensor([-10., -9., -8., -7., -6., -5., -4., -3., -2., -1., 0., 1., 2., 3., 4., 5., 6., 7., 8., 9., 10.])
torch.randn()
有时我们想通过从某个特定的概率分布中随机采样来得到张量中每个元素的值。例如,当我们构造数组来作为神经网络中的参数时,我们通常会随机初始化参数的值。以下代码创建一个形状为(3, 4)的张量。其中的每个元素都从均值为0、标准差为1的标准高斯分布(正态分布)中随机采样
torch.randn(3, 4)
输出:
tensor([[-0.5582, -0.0443, 1.6146, 0.6003],
[-1.7652, 1.3074, 0.5233, 1.4372],
[ 0.2452, 2.2281, 1.3483, 0.1783]])
torch.sin()
函数说明:
返回一个新张量,包含输入input张量每个元素的正弦。
torch.sin(input, out=None) → Tensor
参数:
input (Tensor) – 输入张量
out (Tensor, optional) – 输出张量
示例:
>>> a = torch.randn(4)
>>> a
-0.6366
0.2718
0.4469
1.3122
[torch.FloatTensor of size 4]
>>> torch.sin(a)
-0.5944
0.2684
0.4322
0.9667
[torch.FloatTensor of size 4]
Python中item()和items()的用法
item()
item()的作用是取出单元素张量的元素值并返回该值,保持该元素类型不变。 听起来和使用索引来取值的作用好像一样,接下来我们看一看使用两种方法取元素值的区别: 首先定义一个张量: 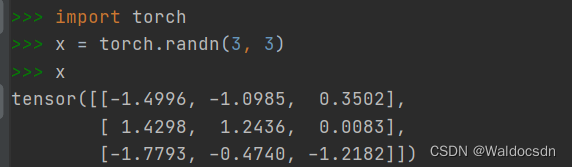 1、直接使用索引取值:  2、使用item()取出  由此可以看出使用item()函数取出的元素值的精度更高,所以在求损失函数等时我们一般用item()
items()
items()的作用是把字典中的每对key和value组成一个元组,并把这些元祖放在列表中返回。 举个例子: 
|