
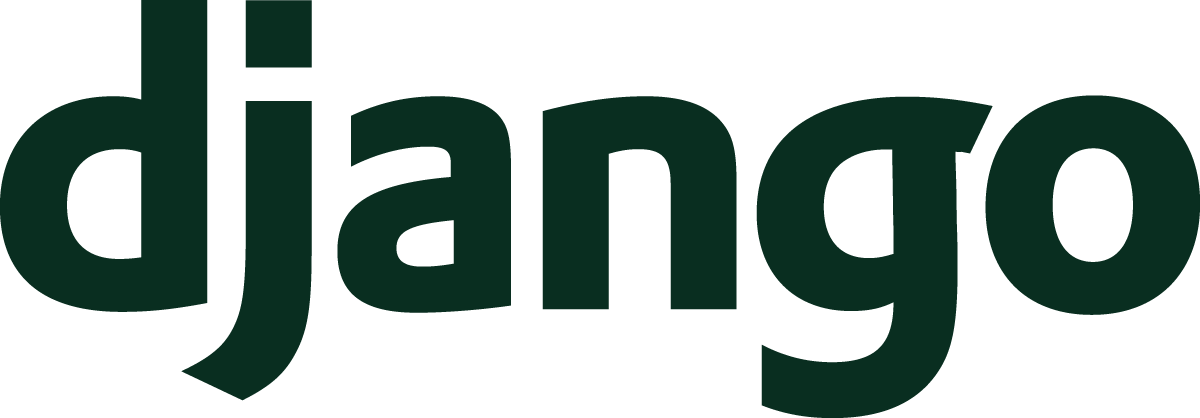
视图在接收请求并处理后,必须返回
HttpResponse 对象或?对象。
HttpRequest 对象由Django 创建,HttpResponse 对象由开发?员创建。
class HttpResponse(HttpResponseBase):
"""
An HTTP response class with a string as content.
This content that can be read, appended to, or replaced.
"""
streaming = False
def __init__(self, content=b'', *args, **kwargs):
super().__init__(*args, **kwargs)
self.content = content
def __repr__(self):
return '<%(cls)s status_code=%(status_code)d%(content_type)s>' % {
'cls': self.__class__.__name__,
'status_code': self.status_code,
'content_type': self._content_type_for_repr,
}
def serialize(self):
"""Full HTTP message, including headers, as a bytestring."""
return self.serialize_headers() + b'\r\n\r\n' + self.content
__bytes__ = serialize
@property
def content(self):
return b''.join(self._container)
@content.setter
def content(self, value):
if hasattr(value, '__iter__') and not isinstance(value, (bytes, str)):
content = b''.join(self.make_bytes(chunk) for chunk in value)
if hasattr(value, 'close'):
try:
value.close()
except Exception:
pass
else:
content = self.make_bytes(value)
self._container = [content]
def __iter__(self):
return iter(self._container)
def write(self, content):
self._container.append(self.make_bytes(content))
def tell(self):
return len(self.content)
def getvalue(self):
return self.content
def writable(self):
return True
def writelines(self, lines):
for line in lines:
self.write(line)
1. HttpResponse
可以使?django.http.HttpResponse 来构造响应对象。
HttpResponse(content=响应体, content_type=响应体数据类型, status=状态码)
也可通过HttpResponse 对象属性来设置响应体、响应体数据类型、状态码:
response = HttpResponse('bei ji de san ha !')
response['bei ji de san ha !'] = 'Python'
示例:
from django.shortcuts import render
from django.http import HttpResponse
def response(request):
return HttpResponse(content='Hello HttpResponse', content_type=str, status=400)
或者:
from django.shortcuts import render
from django.http import HttpResponse
def response(request):
response = HttpResponse('bei ji de san ha !')
response.status_code = 400
response['bei ji de san ha !'] = 'Python'
return respons
2. HttpResponse?类
Django 提供了?系列HttpResponse 的?类,可以快速设置状态码。
HttpResponseRedirect 301
HttpResponsePermanentRedirect 302
HttpResponseNotModified 304
HttpResponseBadRequest 400
HttpResponseNotFound 404
HttpResponseForbidden 403
HttpResponseNotAllowed 405
HttpResponseGone 410
HttpResponseServerError 500
示例:HttpResponseRedirect 301
视图函数:
from django.shortcuts import render
from django.http import HttpResponse, HttpResponseRedirect
def response(request):
return HttpResponseRedirect('/hrv/')
def httpResponseView(request):
return HttpResponse('Hello Django HttpResponse')
路由:
""""""
from django.urls import path, re_path
from papp import views
urlpatterns = [
path('response/', views.response),
path('hrv/', views.httpResponseView),
]
访问:127.0.0.1:8000/response/ 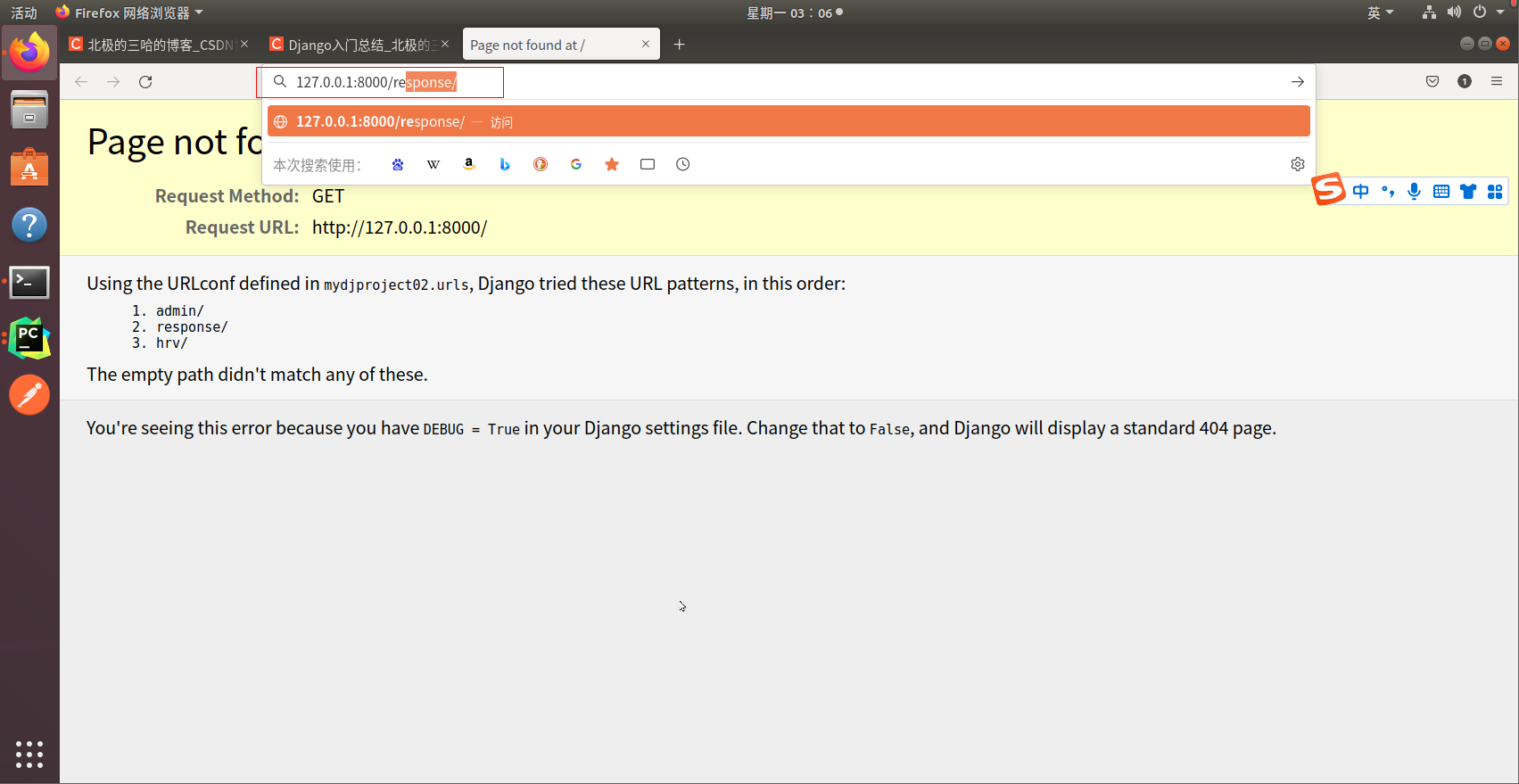
响应:http://127.0.0.1:8000/hrv/ 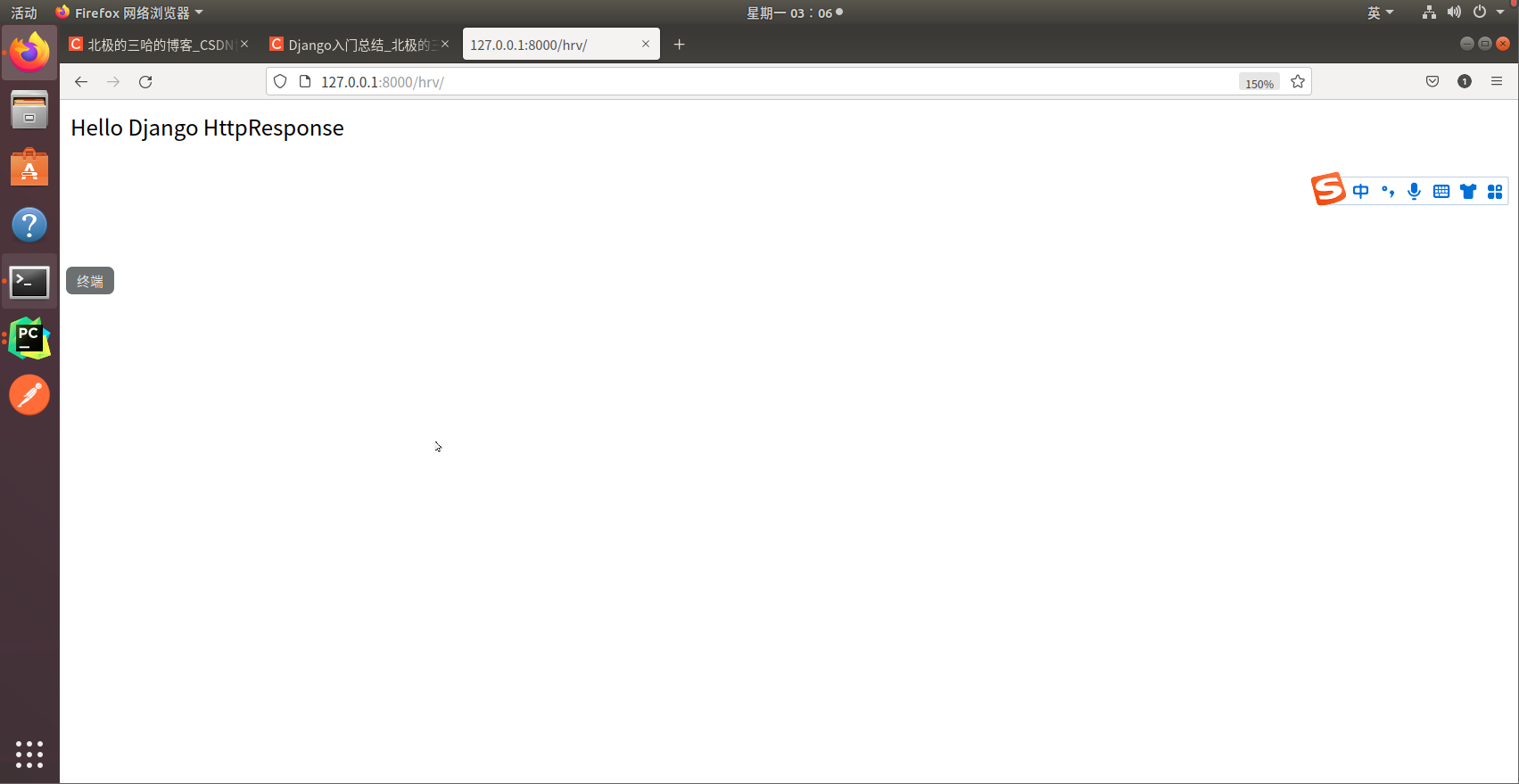
3. JsonResponse
class JsonResponse(HttpResponse):
"""
An HTTP response class that consumes data to be serialized to JSON.
:param data: Data to be dumped into json. By default only ``dict`` objects
are allowed to be passed due to a security flaw before EcmaScript 5. See
the ``safe`` parameter for more information.
:param encoder: Should be a json encoder class. Defaults to
``django.core.serializers.json.DjangoJSONEncoder``.
:param safe: Controls if only ``dict`` objects may be serialized. Defaults
to ``True``.
:param json_dumps_params: A dictionary of kwargs passed to json.dumps().
"""
def __init__(self, data, encoder=DjangoJSONEncoder, safe=True,
json_dumps_params=None, **kwargs):
if safe and not isinstance(data, dict):
raise TypeError(
'In order to allow non-dict objects to be serialized set the '
'safe parameter to False.'
)
if json_dumps_params is None:
json_dumps_params = {}
kwargs.setdefault('content_type', 'application/json')
data = json.dumps(data, cls=encoder, **json_dumps_params)
super().__init__(content=data, **kwargs)
若要返回json 数据,可以使?JsonResponse 来构造响应对象,作?:
from django.shortcuts import render
from django.http import HttpResponse, JsonResponse
def response(request):
return JsonResponse({'uname': 'san ha', 'age': 22})
访问:http://127.0.0.1:8000/response/
JSON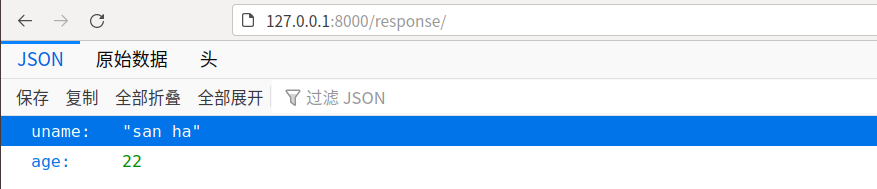
原始数据 
头 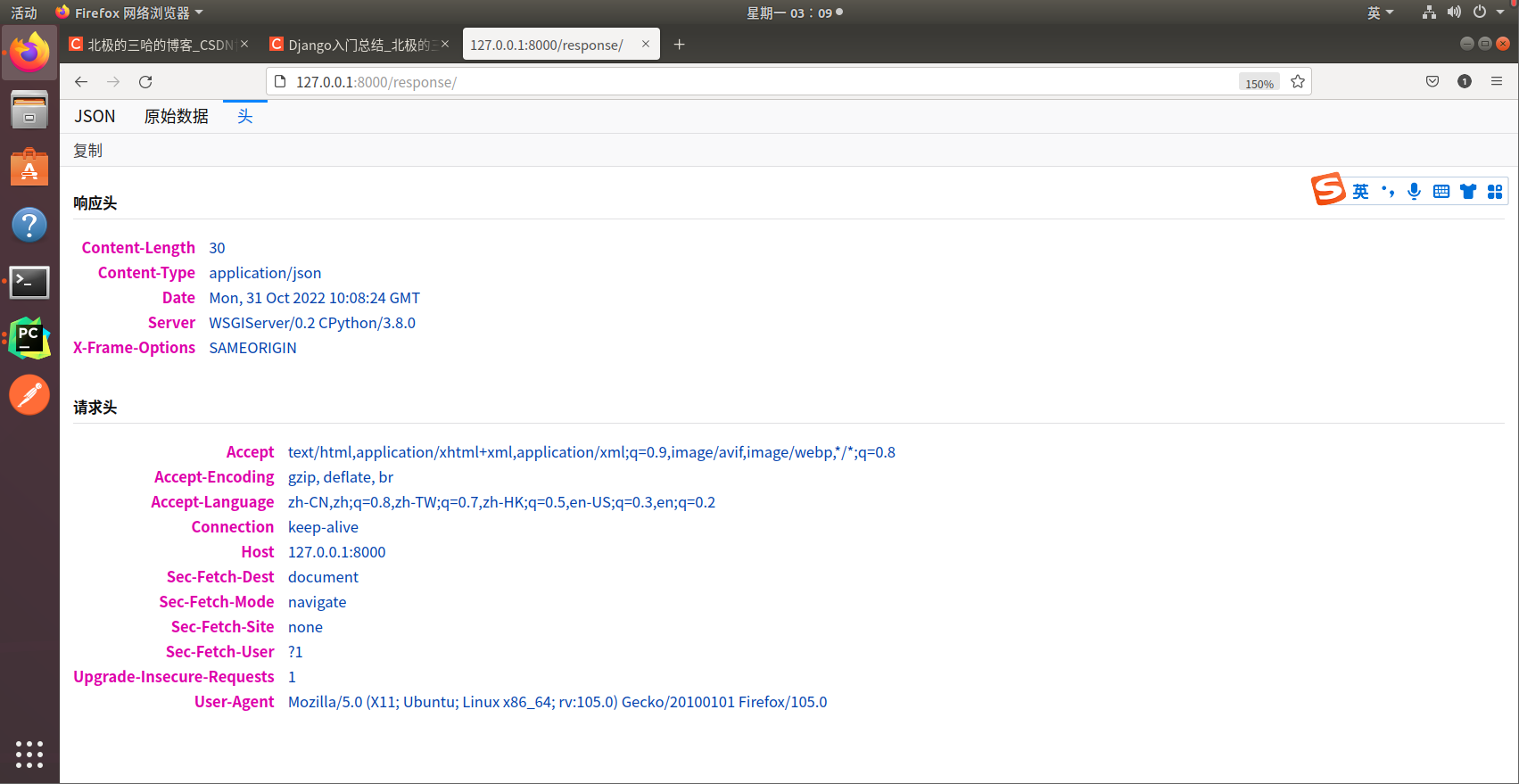
4. redirect重定向
def redirect(to, *args, permanent=False, **kwargs):
"""
Return an HttpResponseRedirect to the appropriate URL for the arguments
passed.
The arguments could be:
* A model: the model's `get_absolute_url()` function will be called.
* A view name, possibly with arguments: `urls.reverse()` will be used
to reverse-resolve the name.
* A URL, which will be used as-is for the redirect location.
Issues a temporary redirect by default; pass permanent=True to issue a
permanent redirect.
"""
redirect_class = HttpResponsePermanentRedirect if permanent else HttpResponseRedirect
return redirect_class(resolve_url(to, *args, **kwargs))
视图函数:
from django.shortcuts import render
from django.http import HttpResponse, JsonResponse, HttpResponseRedirect
from django.shortcuts import redirect
def response(request):
return redirect('/hrv/')
def httpResponseView(request):
return HttpResponse('Hello Django HttpResponse')
路由:
""""""
from django.urls import path, re_path
from papp import views
urlpatterns = [
path('response/', views.response),
path('hrv/', views.httpResponseView),
]
访问:127.0.0.1:8000/response/ 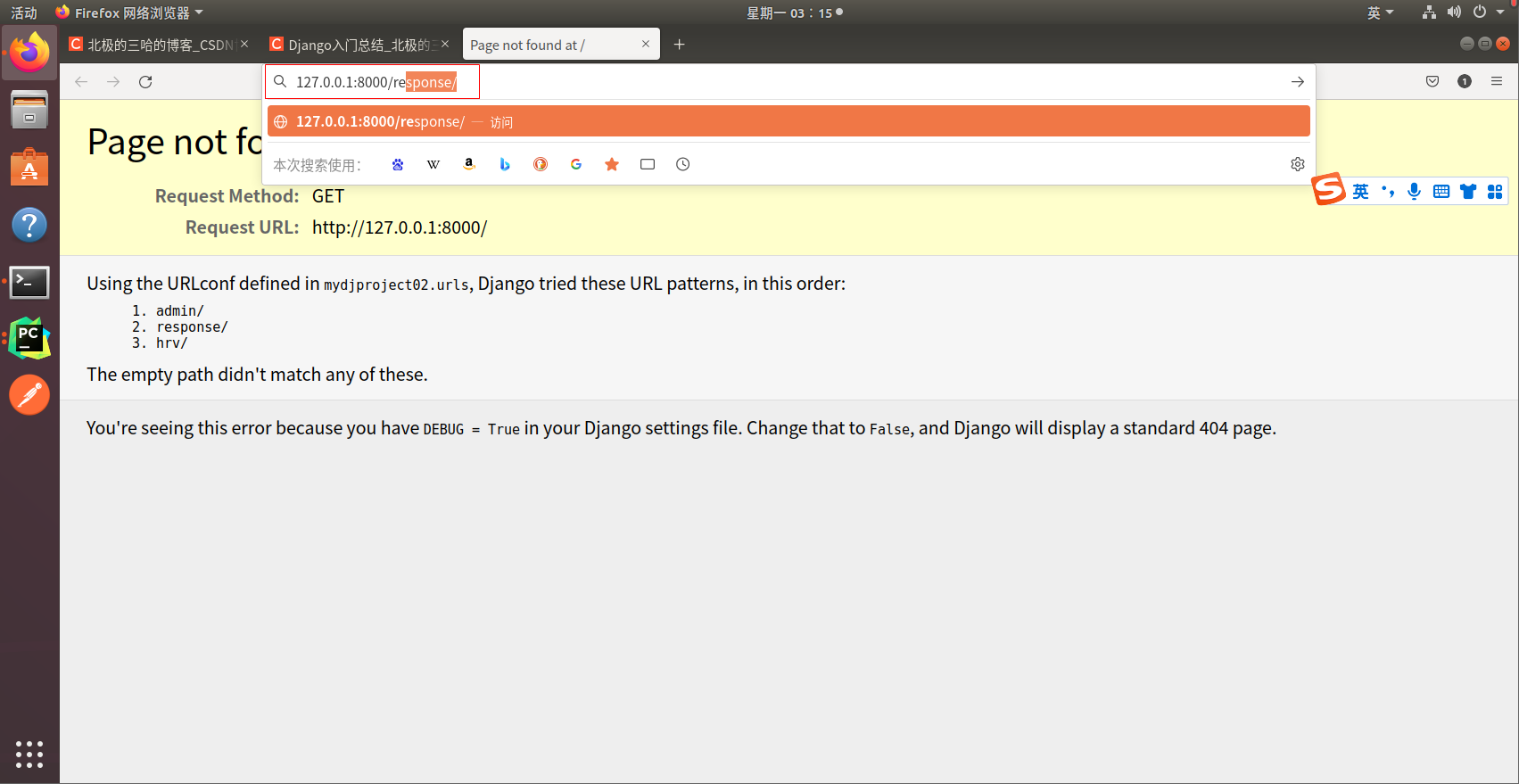
响应:http://127.0.0.1:8000/hrv/ 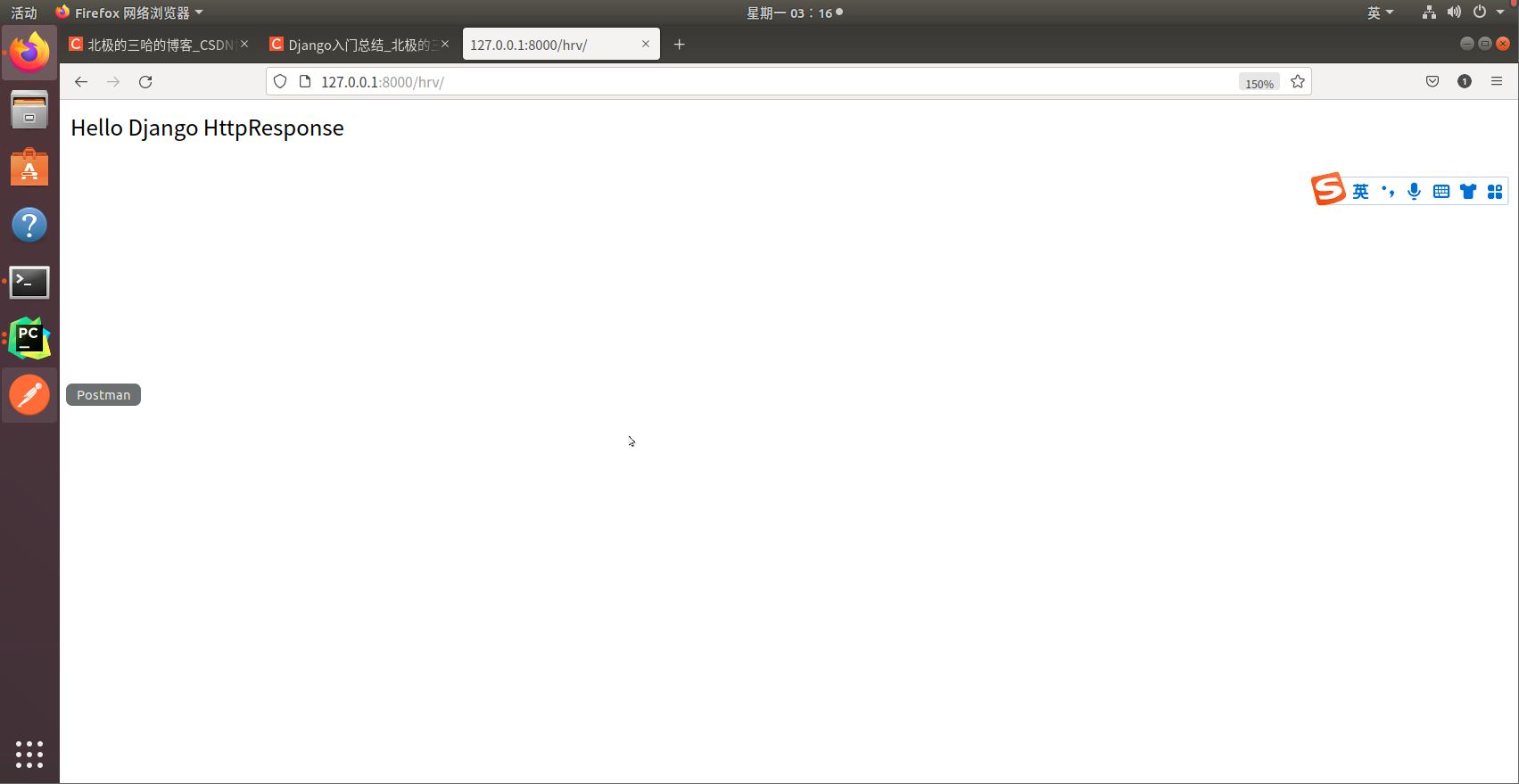
|