记录一下
import numpy as np
import math
import matplotlib.pyplot as plt
def sigmod():
x = np.arange(-10, 10, 0.1)
print(x)
y = []
for t in x:
y_1 = 1 / (1 + math.exp(-t))
y.append(y_1)
print(y)
plt.plot(x, y, label="sigmoid")
plt.xlabel("x")
plt.ylabel("y")
plt.ylim(0, 1)
plt.legend()
plt.show()
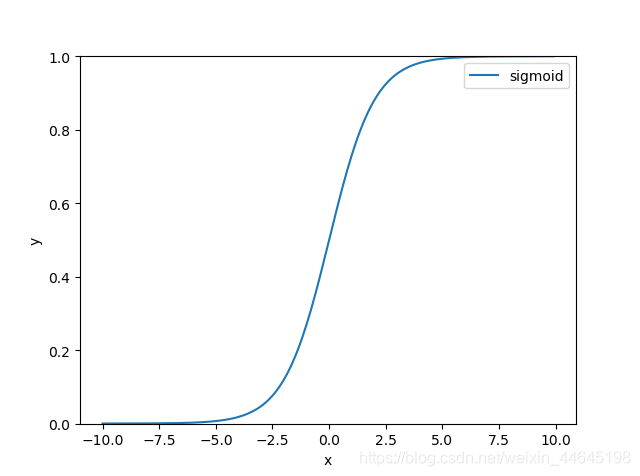
def Tanh():
x = np.arange(-5, 5, 0.1)
print(x)
y = []
for t in x:
y_1 = (1 - math.exp(-(2*t))) / (1 + math.exp(-(2*t)))
y.append(y_1)
print(y)
plt.plot(x, y, label="Tanh")
plt.xlabel("x")
plt.ylabel("y")
plt.ylim(-1, 1)
plt.legend()
plt.show()
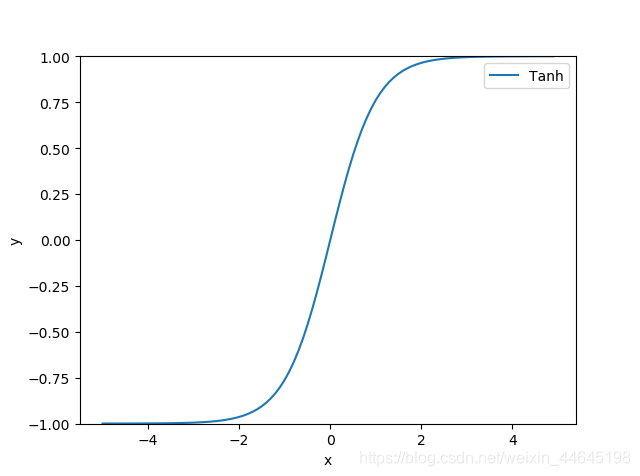
def relu():
g = lambda z: np.maximum(0, z)
start = -10
stop = 10
step = 0.01
num = (stop - start) / step
x = np.linspace(start, stop, int(num))
y = g(x)
plt.plot(x, y, label='relu')
plt.grid(False)
plt.legend()
plt.show()
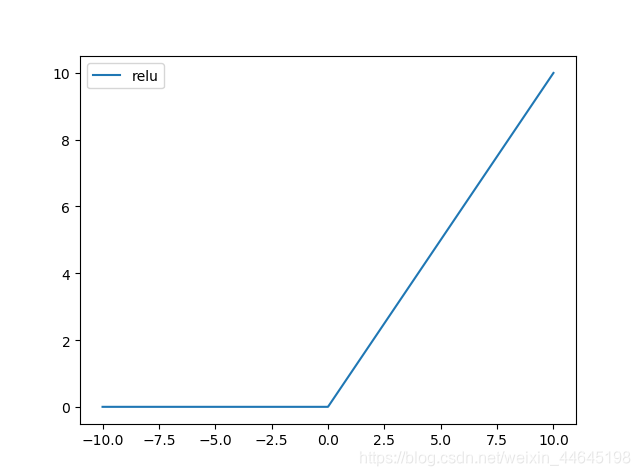
def leaky_relu():
g = lambda z: np.maximum(0.01 * z, z)
start = -150
stop = 50
step = 0.01
num = (stop - start) / step
x = np.linspace(start, stop, int(num))
y = g(x)
fig = plt.figure(1)
plt.plot(x, y, label='Leaky ReLU')
plt.grid(True)
plt.legend()
plt.show(fig)
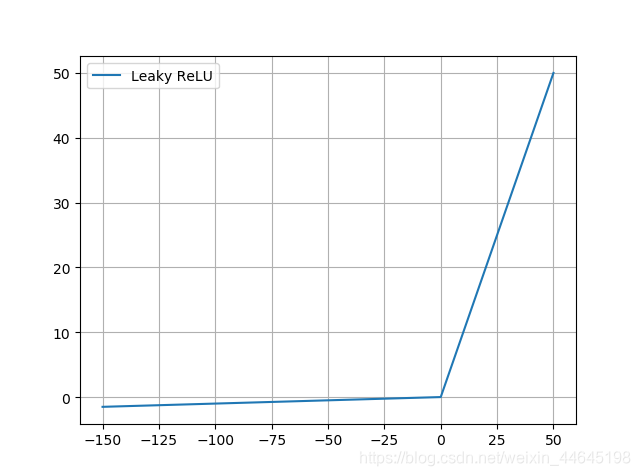
def SiLU():
x = np.arange(-10, 10, 0.1)
y = []
for t in x:
y_1 = t*(1 / (1 + math.exp(-t)))
y.append(y_1)
print(y)
plt.plot(x, y, label="SiLU")
plt.xlabel("x")
plt.ylabel("y")
plt.ylim(-1, 10)
plt.grid(True)
plt.legend()
plt.show()
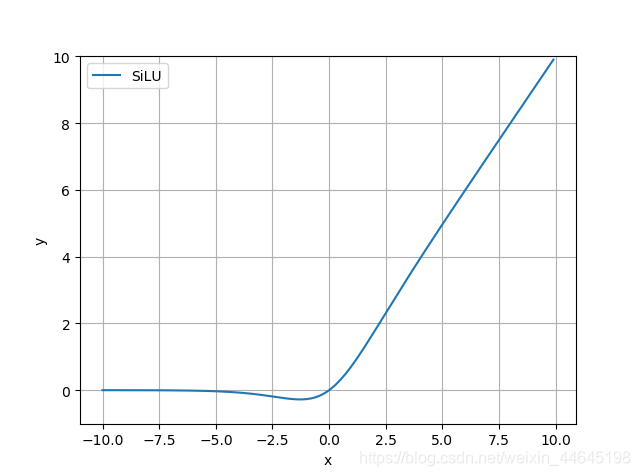
|