1. 线性函数
线性函数用于拟合线性的曲线
2. 非线性函数
非线性函数怎么拟合呢?
- 使用非线性函数拟合
- 使用线性函数+非线性函数(激活函数去拟合)
为了和神经网络相统一,用线性函数+激活函数去拟合非线性函数
3.搭建网络
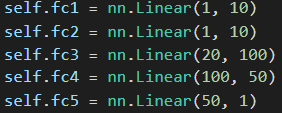 分两路进行全连接层,然后将输出拼接,再经过三个全连接层
4.代码实现
使用Tensorboard查看数据
from numpy.core.function_base import linspace
import torch
import numpy as np
import matplotlib.pyplot as plt
from torch import nn, optim
import torch.nn.functional as F
from matplotlib.animation import FuncAnimation
import threading
from torch.utils.data import Dataset, DataLoader
from torch.utils.tensorboard import SummaryWriter
xx = linspace(0, 2, 3000)
class Data(Dataset):
def __init__(self):
super(Data, self).__init__()
self.x = linspace(0, 3.14, 3000)
self.y = []
for i in self.x:
'''
# 阶梯函数
if i <=1:
self.y.append(0)
else:
self.y.append(1)
# 方波函数
if i <=0.5 or i >= 1.5:
self.y.append(0)
else:
self.y.append(1)
# 正弦函数
self.y = np.sin(self.x*2)
'''
self.y = np.sin(self.x*2)
def __len__(self):
return len(self.x)
def __getitem__(self, index):
x = self.x[index]
y = self.y[index]
x = torch.tensor(x).unsqueeze(dim=0).float()
y = torch.tensor(y).unsqueeze(dim=0).float()
return x, y
train = Data()
train_data = DataLoader(train, batch_size=3000, shuffle=False)
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(1, 10)
self.fc2 = nn.Linear(1, 10)
self.fc3 = nn.Linear(20, 100)
self.fc4 = nn.Linear(100, 50)
self.fc5 = nn.Linear(50, 1)
def forward(self, x):
x1 = F.relu(self.fc1(x))
x2 = F.relu(self.fc2(x))
x3 = torch.cat((x1, x2), dim=1)
x = self.fc3(x3)
x = F.relu(self.fc4(x))
x = self.fc5(x)
return x
net = Net()
optimizer = optim.Adam(net.parameters(), lr=0.001)
loss_fn = nn.MSELoss()
for epoch in range(10000):
for x, y in train_data:
y_pred = net(x)
loss = loss_fn(y_pred, y)
print(f"\r第{epoch}个epoch的损失为{loss.item()}", end="")
optimizer.zero_grad()
loss.backward()
optimizer.step()
if epoch%50 == 0:
fig = plt.figure()
subfig1 = fig.add_subplot(1,2,1)
subfig1.plot(x.detach().numpy(), y_pred.detach().numpy())
subfig1 = fig.add_subplot(1,2,2)
subfig1.plot(x.detach().numpy(), y.detach().numpy())
writer = SummaryWriter()
writer.add_figure('figure', fig, global_step=None, close=True, walltime=None)
writer.add_scalar('Loss', loss.item(), epoch)
使用Matplotlib查看变化动画
from numpy.core.function_base import linspace
import torch
import numpy as np
import matplotlib.pyplot as plt
from torch import nn, optim
import torch.nn.functional as F
from matplotlib.animation import FuncAnimation
import threading
from torch.utils.data import Dataset, DataLoader
from torch.utils.tensorboard import SummaryWriter
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
xx = linspace(0, 2, 3000)
class Data(Dataset):
def __init__(self):
super(Data, self).__init__()
self.id = 1
self.x = linspace(0, 3.14, 3000)
self.y = []
for i in self.x:
if self.id ==0 :
if i <=1:
self.y.append(0)
else:
self.y.append(1)
elif self.id == 1:
if i <=0.5 or i >= 1.5:
self.y.append(0)
else:
self.y.append(1)
elif self.id == 2:
self.y = np.sin(self.x*2)
self.y = np.sin(self.x*2)
def __len__(self):
return len(self.x)
def __getitem__(self, index):
x = self.x[index]
y = self.y[index]
x = torch.tensor(x).unsqueeze(dim=0).float()
y = torch.tensor(y).unsqueeze(dim=0).float()
return x, y
train = Data()
train_data = DataLoader(train, batch_size=3000, shuffle=False)
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(1, 10)
self.fc2 = nn.Linear(1, 10)
self.fc3 = nn.Linear(20, 100)
self.fc4 = nn.Linear(100, 50)
self.fc5 = nn.Linear(50, 1)
def forward(self, x):
x1 = F.relu(self.fc1(x))
x2 = F.relu(self.fc2(x))
x3 = torch.cat((x1, x2), dim=1)
x = self.fc3(x3)
x = F.relu(self.fc4(x))
x = self.fc5(x)
return x
net = Net()
optimizer = optim.Adam(net.parameters(), lr=0.001)
loss_fn = nn.MSELoss()
xx, yy, y_pred = None, None, None
def train():
global xx, yy, y_pred
for epoch in range(10000):
for x, y in train_data:
xx = x
yy = y
y_pred = net(x)
loss = loss_fn(y_pred, y)
print(f"\r第{epoch}个epoch的损失为{loss.item()}", end="")
optimizer.zero_grad()
loss.backward()
optimizer.step()
thread1 = threading.Thread(target=train)
thread1.start()
fig, ax = plt.subplots()
ln, = plt.plot([], [], 'red')
def init():
ax.set_xlim(0, np.pi)
ax.set_ylim(-1, 1)
return ln,
def update(frame):
ln.set_data(xx.detach().numpy(), y_pred.detach().numpy())
return ln,
ani = FuncAnimation(fig, update, frames=50,
init_func=init, interval=200, blit=True)
plt.show()
5.拟合效果
左侧为拟合图,右侧为实际图
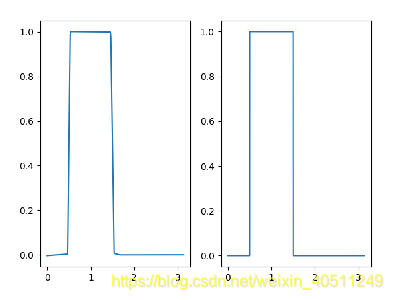
-
方波函数 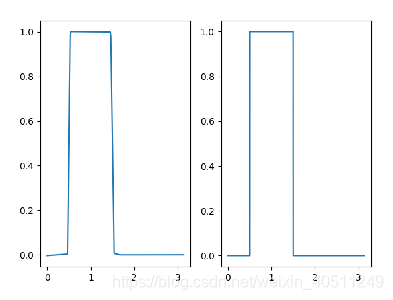 -
三角函数 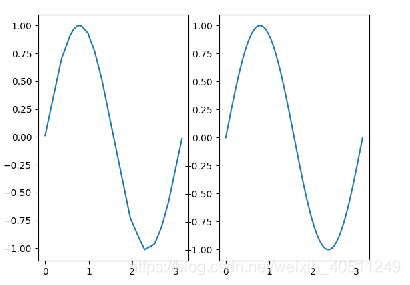
参考
PyTorch文档
|