二、回归问题
内容参考来自https://github.com/dragen1860/Deep-Learning-with-TensorFlow-book开源书籍《TensorFlow2深度学习》,这只是我做的简单的学习笔记,方便以后复习。
1. 数据生成
import numpy as np
import pandas as pd
data = []
X = []
Y = []
for i in range(100):
x = np.random.uniform(3., 12.)
eps = np.random.normal(0., 0.1)
y = 1.477 * x + 0.089 + eps
X.append(x)
Y.append(y)
data.append([x, y])
data = np.array(data)
print(data.shape, data)
dataFrame = pd.DataFrame({'x': X, 'y': Y})
dataFrame.to_csv("data_jyz.csv", index=False, sep=',', header=None)
2.线性回归
import numpy as np
import matplotlib.pyplot as plt
def compute_error_for_line_given_points(b, w, points):
totalError = 0
for i in range(0, len(points)):
x = points[i, 0]
y = points[i, 1]
totalError += (y - (w * x + b)) ** 2
return totalError / float(len(points))
def step_gradient(b_current, w_current, points, learningRate):
b_gradient = 0
w_gradient = 0
N = float(len(points))
for i in range(0, len(points)):
x = points[i, 0]
y = points[i, 1]
b_gradient += (2 / N) * ((w_current * x + b_current) - y)
w_gradient += (2 / N) * x * ((w_current * x + b_current) - y)
new_b = b_current - (learningRate * b_gradient)
new_w = w_current - (learningRate * w_gradient)
return [new_b, new_w]
def gradient_descent_runner(points, starting_b, starting_w, learning_rate, num_iterations):
b = starting_b
w = starting_w
for i in range(num_iterations):
b, w = step_gradient(b, w, np.array(points), learning_rate)
return [b, w]
def showCharts(points, w, b):
points = np.array(points)
plt.scatter(points[:, 0], points[:, 1])
x_ = np.random.uniform(0, 80, 100)
y_ = w * x_ + b
plt.plot(x_, y_)
plt.show()
def run():
points = np.genfromtxt("data.csv", delimiter=",")
learning_rate = 0.0001
initial_b = 0
initial_w = 0
num_iterations = 1000
print("Starting gradient descent at b = {0}, w = {1}, error = {2}".format(initial_b, initial_w,
compute_error_for_line_given_points(
initial_b, initial_w, points)))
print("Running...")
[b, w] = gradient_descent_runner(points, initial_b, initial_w, learning_rate, num_iterations)
print("After {0} iterations b = {1}, w = {2}, error = {3}".
format(num_iterations, b, w,
compute_error_for_line_given_points(b, w, points))
)
showCharts(points, w, b)
if __name__ == '__main__':
run()
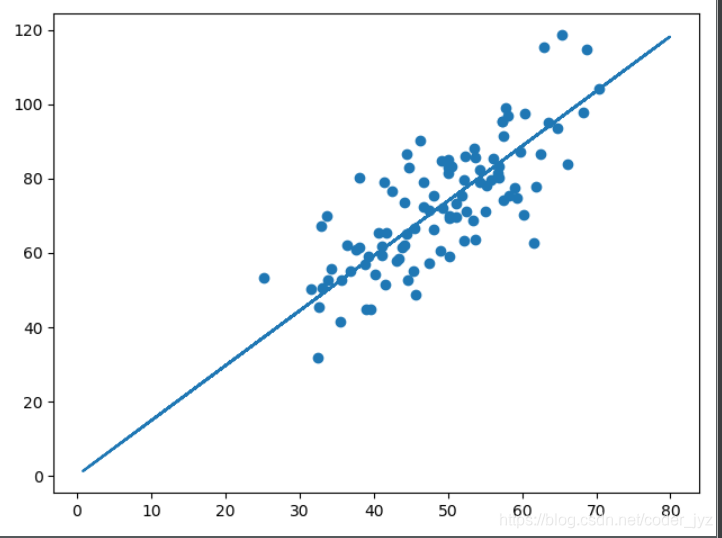
|