门户首页书籍类别显示
1.HTML拼接样式
?
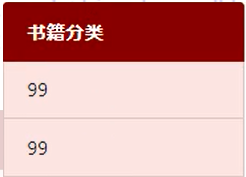
Category类:
package com.zw.entity;
public class Category {
private long id;
private String name;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Category [id=" + id + ", name=" + name + "]";
}
}
?CategoryDao:
public class CategoryDao extends BaseDao<Category>{
//查询类别
public List<Category> list( Category category, PageBean pageBean) throws Exception {
String sql="select * from t_easyui_category where 1=1 ";
long id = category.getId();
if(id != 0) {
sql += " and id =" + id;
}
return super.executeQuery(sql, Category.class, pageBean);
}
}
CategoryAction:
public class CategoryAction extends ActionSupport implements ModelDriver<Category> {
private Category category = new Category();
private CategoryDao categoryDao = new CategoryDao();
public Category getModel() {
return category;
}
// 类别下拉框
public String combobox(HttpServletRequest req, HttpServletResponse resp) throws Exception {
// 获取下拉框值
List<Category> list = categoryDao.list(category, null);
ResponseUtil.writeJson(resp, list);
return null;
}
}
配置mvc文件:
<action path="/category" type="com.zw.web.CategoryAction"></action>
js文件:
$(function(){
$.ajax({
url:$("#ctx").val()+"/category.action?methodName=combobox",
success:function(data){
var jsonArr=eval("("+data+")");
var html='';
for(var i in jsonArr){
html+='<li class="list-group-item">'+jsonArr[i].name+'</li>';
}
$(".list-group").append(html);
}
});
})
效果:
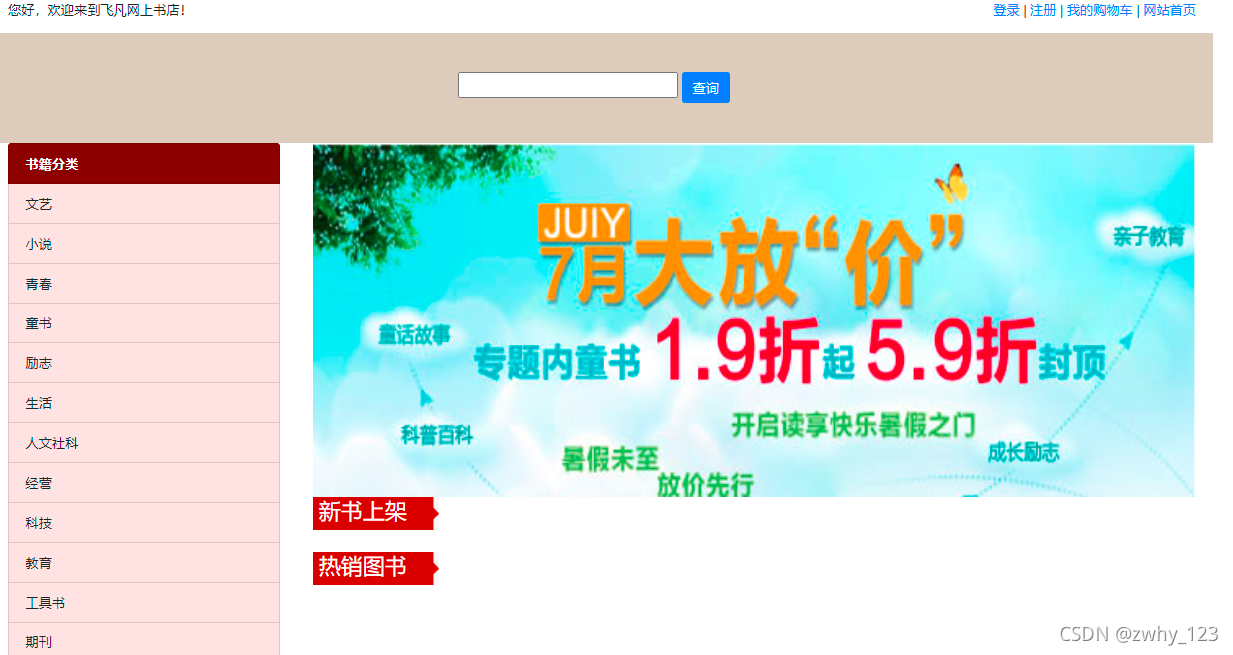
类别查询
设置点击事件:
$(function(){
$.ajax({
url:$("#ctx").val()+"/category.action?methodName=combobox",
success:function(data){
var jsonArr=eval("("+data+")");
var html='';
for(var i in jsonArr){
html+='<li class="list-group-item" οnclick="searchByType('+jsonArr[i].id+')">'+jsonArr[i].name+'</li>';
}
$(".list-group").append(html);
}
});
})
BookDao:
// 查询
public List<Book> list(Book book, PageBean pageBean) throws Exception {
String sql = "select * from t_easyui_book where 1=1 ";
String name = book.getName();
int state = book.getState();
long cid = book.getCid();
if(StringUtils.isNotBlank(name)) {
sql += " and name like '%"+name+"%'";
}
// 书籍状态
if(state != 0) {
sql+=" and state="+state;
}
// 类别
if(cid != 0) {
sql+=" and cid="+cid;
}
return super.executeQuery(sql, Book.class, pageBean);
}
?BookAction:
public String findByType(HttpServletRequest req, HttpServletResponse resp) {
try {
PageBean pageBean=new PageBean();
pageBean.setRequest(req);
List<Book> list = bd.list(book, pageBean);
req.setAttribute("books", list);
req.setAttribute("pageBean", pageBean);
} catch (Exception e) {
e.printStackTrace();
try {
ResponseUtil.writeJson(resp, 0);
} catch (Exception e1) {
e1.printStackTrace();
}
}
return "findBook";
}
配置文件:
<action path="/book" type="com.zw.web.BookAction"> ?? ?<forward name="findBook" path="/fg/findBook.jsp" redirect="false" /> </action>
效果:
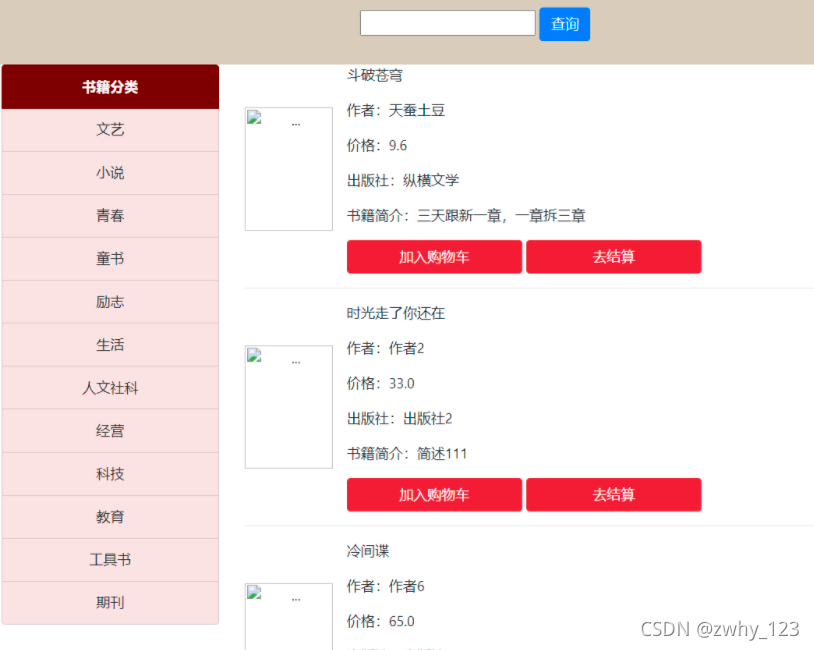
?网络图片上传
1.导入jar包:
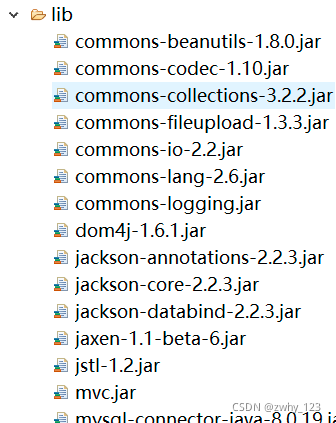
2.图片上传表单提交:
function submitForm2() {
var row = $('#dg').datagrid('getSelected');
console.log(row);
// if (row) {
// $('#ff2').attr("action", $('#ff2').attr("action") + "&id=" + row.id);
// }
$('#ff2').form('submit', {
url: '${pageContext.request.contextPath}/book.action?methodName=upload&id=' + row.id,
success: function (param) {
$('#dd2').dialog('close');
$('#dg').datagrid('reload');
$('#ff2').form('clear');
}
})
}
3.BookDao
修改:
public void editImgUrl(Book book) throws Exception { ? ? ? ? super.executeUpdate("update t_easyui_book set image=? ?where id=?",? ? ? ? ? ? ? ? ? book,new String[] {"image","id"}); }
?4.工具类:
public static String getCurrentDateStr() { // ? ? ? ?生成20210924123025这种格式的照片 ? ? ? ? SimpleDateFormat sdf=new SimpleDateFormat("yyyyMMddHHmmss"); ? ? ? ? return sdf.format(new Date()); ? ? }
5.帮助类:
public static String getValue(String key) throws Exception { ? ? ? ? Properties p=new Properties(); ? ? ? ? InputStream in = PropertiesUtil.class.getResourceAsStream("/resource.properties"); ? ? ? ? p.load(in); ? ? ? ? return p.getProperty(key); ? ? }
6.BookAaction:
public String upload(HttpServletRequest req, HttpServletResponse resp) {
try {
FileItemFactory factory = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(factory);
List<FileItem> items = upload.parseRequest(req);
Iterator<FileItem> itr = items.iterator();
HttpSession session = req.getSession();
while (itr.hasNext()) {
FileItem item = (FileItem) itr.next();
if (item.isFormField()) {
System.out.println("普通字段处理");
book.setId(Long.valueOf(req.getParameter("id")));
} else if (!"".equals(item.getName())) {
String imageName = DateUtil.getCurrentDateStr();
// 存入数据的的数据,以及浏览器访问图片的映射地址,网络映射
String serverDir = PropertiesUtil.getValue("serverDir");
// 图片真实的存放位置
String diskDir = PropertiesUtil.getValue("diskDir");
// 图片的后缀名
String subfix = item.getName().split("\\.")[1];
book.setImage(serverDir + imageName + "." + subfix);
item.write(new File(diskDir + imageName + "." + subfix));
this.bd.editImgUrl(book);
ResponseUtil.writeJson(resp, 1);
}
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
server文件:
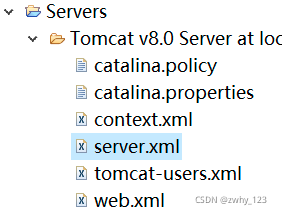
?效果:
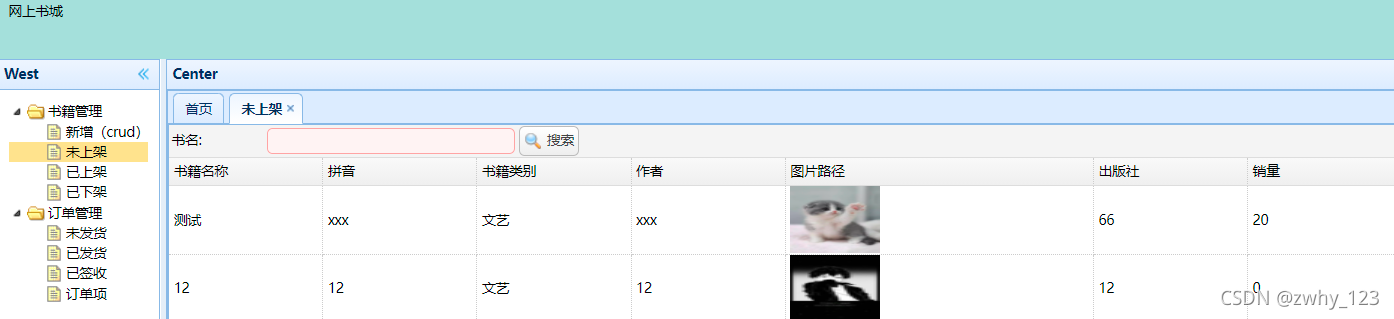
?
|