一、图片处理
import cv2
import matplotlib.pyplot as plt
def to_plt_color(img):
img_cp = img.copy()
return img_cp[...,(2,1,0)]
def cv_img_show(img, title=""):
if title:
plt.title(title)
plt.imshow(to_plt_color(img))
plt.show()
读取图片与显示图片
img = cv2.imread("nikou.jpg")
print(type(img))
print(img.shape)
<class 'numpy.ndarray'>
(1044, 1200, 3)
cv_img_show(img)
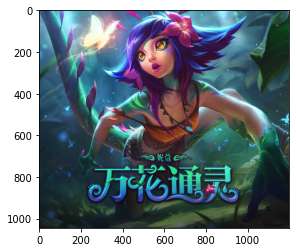
编辑与保存图片
cv2.imwrite(path, img) 保存图片
img_cp = img.copy()
def save_channel(img, channel=0):
img = img.copy()
for i in range(img.shape[-1]):
if i != channel:
img[..., i] = 0
cv2.imwrite(f"channel{channel}.jpg", img)
plt.title(f"channel_{channel}")
plt.imshow(to_plt_color(img))
plt.show()
for i in range(img_cp.shape[-1]):
save_channel(img_cp, channel=i)
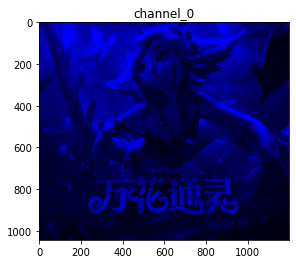
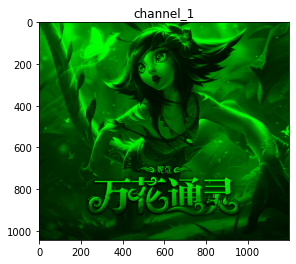
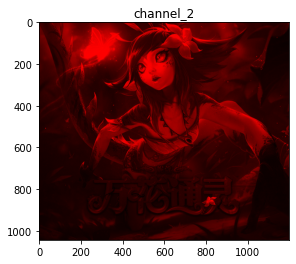
截取部分图片
img_part = img.copy()[0:400, 400:800, :]
cv_img_show(img_part)
print(img_part.shape)
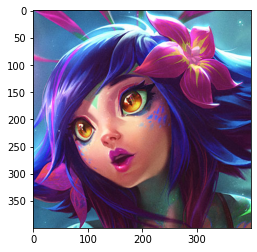
(400, 400, 3)
图片通道分离与合并
cv2.split() 通道分离 cv2.merge() 通道合并
def mark_channel(img):
l = 200
h = int(l / 2)
for i in range(3):
img[i * l: i * l + h, 0:200, i] = 255
img_cp = img.copy()
mark_channel(img_cp)
blue, green, red = cv2.split(img_cp)
print(type(blue))
print(blue.shape)
<class 'numpy.ndarray'>
(1044, 1200)
img_cp = img.copy()
mark_channel(img_cp)
cv_img_show(img_cp)
plt.imshow(blue, cmap='gray')
plt.show()
plt.imshow(green, cmap="gray")
plt.show()
plt.imshow(red, cmap="gray")
plt.show()
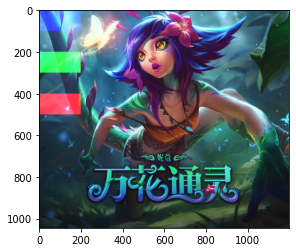
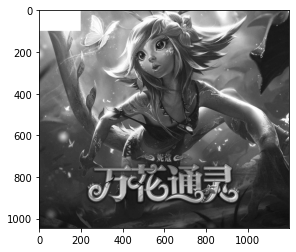
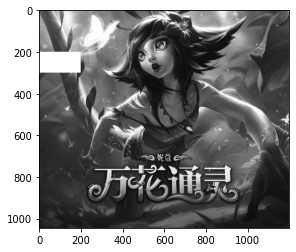
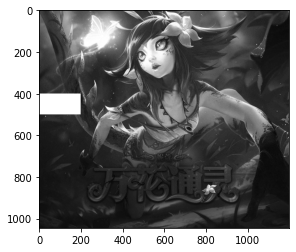
img_merge = cv2.merge([blue,green, red])
cv_img_show(img_merge)
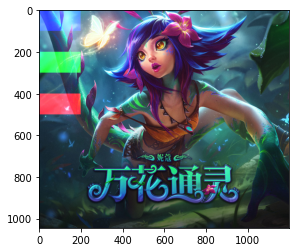
颜色模式变换
颜色模式变换:cv2.COLOR_BGR2GRAY: BGR模式变成灰度, cv2.COLOR_BGR2RGB: BGR变成 RGB 其他如 CMYK Lab模式也有对应转换
img_gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
print(img_gray.shape)
(1044, 1200)
plt.imshow(img_gray, cmap="gray")
<matplotlib.image.AxesImage at 0x23024ed6760>
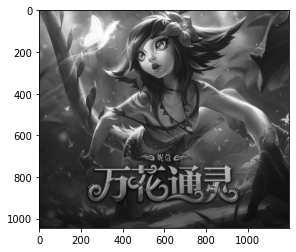
img_rgb = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
plt.subplot(1,2,1)
plt.imshow(img)
plt.subplot(1,2,2)
plt.imshow(img_rgb)
plt.show()
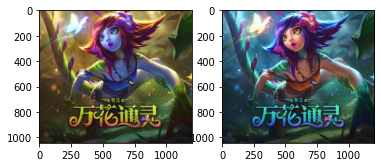
图片相加
"""
cv2.copyMakeBorder(img, top, bottom, left, right, border_type, value=None)
参数:
img: numpy.ndarray
top,bottom,left,right: 上下左右边界宽度,单位为像素值
border_type:
cv2.BORDER_CONSTANT, 带颜色的边界,需要传入另外一个颜色值
cv2.BORDER_REFLECT, 边缘元素的镜像反射做为边界
cv2.BORDER_REFLECT_101/cv2.BORDER_DEFAULT
cv2.BORDER_REPLICATE, 边缘元素的复制做为边界
CV2.BORDER_WRAP
value: borderType为cv2.BORDER_CONSTANT时,传入的边界颜色值,如[0,255,0]
"""
img2 = cv2.imread("mumu.jpg")
print(img2.shape)
img2 = cv2.copyMakeBorder(img2, 500, 0,10,900, borderType=cv2.BORDER_CONSTANT, value=(0,0,0))
img2 =cv2.resize(img2, img.shape[:2][::-1])
print(img2.shape)
cv_img_show(img2)
(523, 320, 3)
(1044, 1200, 3)
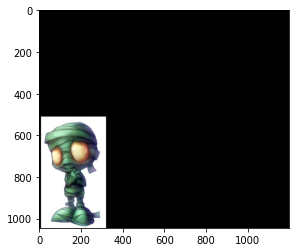
img_add = cv2.add(img, img2)
cv_img_show(img_add)
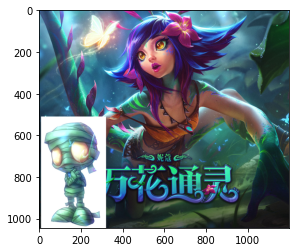
img.shape
(1044, 1200, 3)
img3 = cv2.imread("mumu2.jpg")
img3 = cv2.resize(img3, img.shape[:2][::-1])
cv_img_show(img3)
img_merge = cv2.add(img3, img)
cv_img_show(img_merge)
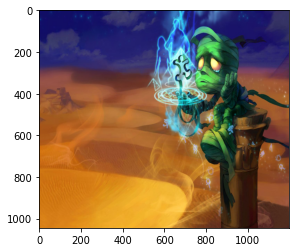
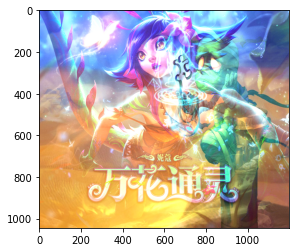
cv2.imwrite("merge.jpg",img_merge)
True
将图片保存到内存中(PIL)
某些应用场景需要序列化或者发送图片,如果将处理好的图片,先保存到硬盘,然后再读取二进制内容,这中方式速度慢。可以使用PIL直接写到BytesIO,免去磁盘IO。
import io
from PIL import Image
with io.BytesIO() as f:
img_pil = Image.fromarray(cv2.cvtColor(img_merge, cv2.COLOR_BGR2RGB))
img_pil.save(f, "JPEG")
data = f.getvalue()
print(type(data))
print(data[:10])
with open("pil_save.jpg", "wb") as f:
f.write(data)
<class 'bytes'>
b'\xff\xd8\xff\xe0\x00\x10JFIF'
|